Common Pitfalls When Starting with GraphQL in Java
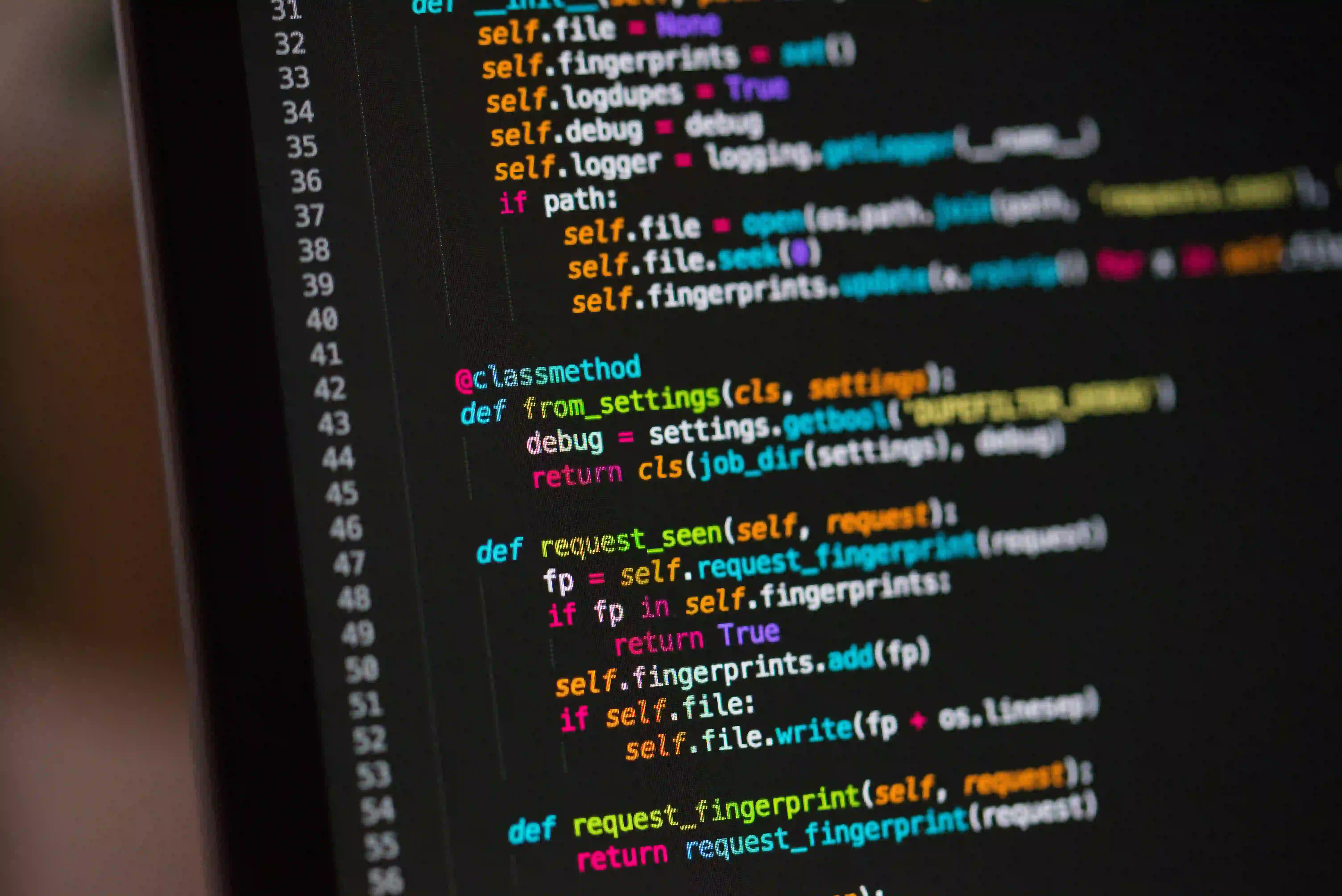
Common Pitfalls When Starting with GraphQL in Java
As the demand for efficient data interactions in modern applications rises, developers are increasingly turning to GraphQL. Originally created by Facebook, GraphQL offers a flexible and efficient alternative to REST APIs. However, while the powerful capabilities of GraphQL can be enticing, it can also introduce various pitfalls for Java developers who may be new to this paradigm. In this post, we will explore some common pitfalls when starting with GraphQL in Java and how to avoid them.
What is GraphQL?
Before diving into the pitfalls, let’s briefly recap what GraphQL is. At its core, GraphQL is a query language for APIs that allows clients to request exactly the data they need. It enables a more efficient and flexible interaction with your backend than traditional REST APIs, which often return unnecessary or overly complex data structures.
Why Use GraphQL in Java?
Java developers can benefit from using GraphQL for numerous reasons, including:
- Strongly Typed Schema: GraphQL uses a schema based definition that maps data types explicitly, enhancing both development and debugging.
- Single Endpoint: Unlike REST's multiple endpoints, a single GraphQL endpoint can handle various queries, simplifying the API structure.
- Client-Driven Queries: Clients can specify precisely what they need, reducing the amount of data transferred and processed.
Common Pitfalls When Starting with GraphQL in Java
1. Poorly Defined Schema
Description: The GraphQL schema is the backbone of your API. A poorly defined schema can lead to confusion and inefficiencies.
Solution: Spend adequate time designing your schema. Use tools like GraphiQL or Apollo Studio to visualize and iterate on your schema. The schema should represent the relationships between your data in a straightforward manner.
import graphql.schema.*;
import graphql.GraphQL;
GraphQLSchema schema = GraphQLSchema.newSchema()
.query(QueryType())
.build();
GraphQL graphQL = GraphQL.newGraphQL(schema).build();
In this code snippet, we are creating a simple GraphQL schema that includes a root query type. Ensuring that your query types are well structured will provide a solid foundation for your API.
2. Over-fetching and Under-fetching Data
Description: One of the main features of GraphQL is its ability to fetch exactly what is needed. However, mismanagement can lead to over-fetching (requesting too much data) or under-fetching (not getting enough data in one request).
Solution: Be mindful of your queries. For complex data structures, consider using batch loading with tools like Dataloader. This will help aggregate data requests effectively, allowing you to avoid these common issues.
DataLoader<Long, User> userLoader = DataLoader.newDataLoader(userBatchLoader);
public CompletableFuture<User> getUserById(Long id) {
return userLoader.load(id);
}
In the above example, Dataloader is configured to batch load user data. By doing this, you're optimizing database calls, which reduces the likelihood of unnecessary data retrieval.
3. Ignoring Error Handling
Description: GraphQL’s error messaging is often not well-integrated into Java applications by default, which can lead to incomplete or ambiguous error messages.
Solution: Implement a structured error handling strategy to ensure that clients receive clear and meaningful error messages. Use custom error classes to provide specific information about the errors.
import graphql.GraphQLException;
public class CustomExceptionHandler implements GraphQLErrorHandler {
@Override
public List<GraphQLError> processErrors(List<GraphQLError> errors) {
return errors.stream()
.map(error -> new CustomError(error.getMessage(), error.getLocations()))
.collect(Collectors.toList());
}
}
This code snippet demonstrates a custom error handler. By customizing error messages, you can help clients understand what went wrong during their API calls.
4. Neglecting Security Measures
Description: With the flexibility of GraphQL comes the risk of over-exposing your data. If not carefully managed, you might unintentionally allow access to sensitive information.
Solution: Always validate input for your queries and regularly inspect your schema to ensure you're not exposing unnecessary fields. Consider implementing middleware to handle authorization.
import graphql.schema.*;
import graphql.GraphQL;
GraphQLSchema schema = GraphQLSchema.newSchema()
.query(QueryType())
.middleware(new AuthorizationMiddleware())
.build();
In this case, middleware is used to enforce authorization on your GraphQL schema. This is vital for ensuring that data access is controlled.
5. Lack of Documentation
Description: Good documentation is crucial, yet many developers overlook this aspect when working with GraphQL.
Solution: Leverage tools like GraphQL Voyager to create visual documentation that describes your GraphQL API schema clearly.
6. Not Caching Responses
Description: GraphQL queries can be inefficient without proper caching strategies, leading to performance bottlenecks.
Solution: Implement caching at both the API and client levels. The use of caching mechanisms like Redis or similar services can drastically speed up your application.
import redis.clients.jedis.Jedis;
// Caching middleware example
public class CachingMiddleware implements Middleware {
private Jedis jedis = new Jedis("localhost");
public Object invoke(Invocation invocation) {
String cacheKey = generateKey(invocation);
if (jedis.exists(cacheKey)) {
return jedis.get(cacheKey);
}
Object result = invocation.proceed();
jedis.set(cacheKey, result);
return result;
}
}
Here, we are implementing a caching middleware using Redis. This reduces database load and improves response times for frequent queries.
7. Skipping Testing
Description: As with any development project, skipping testing can lead to faulty APIs and disrupted applications.
Solution: Make unit testing and end-to-end testing an integral part of your development process. Use frameworks like JUnit or MockMvc to ensure your GraphQL endpoints behave as expected.
import static org.springframework.test.web.servlet.request.MockMvcRequestBuilders.*;
import static org.springframework.test.web.servlet.result.MockMvcResultMatchers.*;
@Test
public void testGraphQLQuery() throws Exception {
mockMvc.perform(post("/graphql")
.contentType("application/json")
.content("{\"query\":\"query { users { id name } }\"}"))
.andExpect(status().isOk())
.andExpect(jsonPath("$.data.users").exists());
}
This snippet provides an example of using JUnit to test a GraphQL query. Skipping this step can lead to serious bugs unnoticed during development.
In Conclusion, Here is What Matters
Starting with GraphQL in Java can be daunting due to the pitfalls associated with this powerful paradigm. However, understanding these common issues and preparing for them can lead to successful implementation. Remember to define your schema clearly, handle errors properly, secure your API, document thoroughly, implement caching, and always prioritize testing. By being proactive about these elements, you can harness the full potential of GraphQL and provide a robust API experience for your users.
Stay updated on GraphQL best practices and keep delving into the vast resources available for Java and GraphQL integration. Good luck on your GraphQL journey!