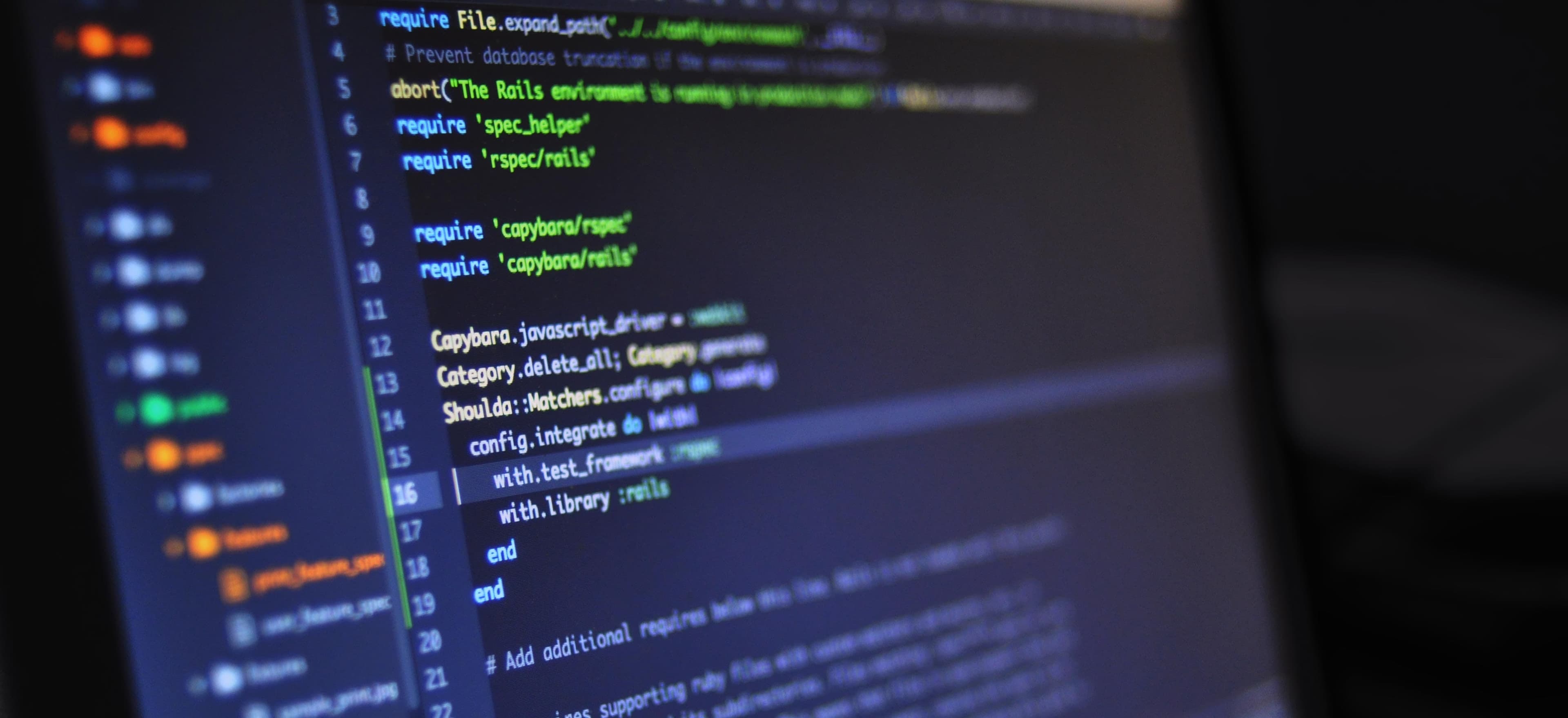
- Published on
Challenges of Managing Dependencies Outside Spring Framework
Dependency management is a critical aspect of software development. While the Spring Framework offers extensive support for managing dependencies, many developers encounter challenges when they opt to work outside this robust ecosystem. In this blog post, we'll explore the challenges of managing dependencies in Java projects without Spring, discuss best practices, and provide examples to highlight common pitfalls along with effective solutions.
Understanding Dependency Management
In software engineering, dependencies are external libraries or modules that a project requires to function correctly. Properly managing these dependencies ensures that our code is modular, testable, and maintainable. Tools like Maven and Gradle play a significant role in this process, but managing dependencies manually or with incompatible tools can lead to significant challenges.
Common Challenges of Managing Dependencies
1. Version Conflicts
One of the foremost challenges of managing dependencies is dealing with version conflicts. When multiple libraries require different versions of the same dependency, your application can fail to compile or, worse, lead to runtime errors.
Example:
Consider two libraries: LibraryA
depends on LibraryB v1.0
, while LibraryC
depends on LibraryB v2.0
. If both are included in your project, the compile-time error will surface, or undesirable behavior might occur.
Solution:
To mitigate version conflicts, always use dependency management tools. In Maven, for instance, you can manage versions systematically via a <dependencyManagement>
section. In Gradle, utilize the resolutionStrategy
to force specific dependency versions.
<dependencyManagement>
<dependencies>
<dependency>
<groupId>com.example</groupId>
<artifactId>libraryB</artifactId>
<version>2.0</version> <!-- Enforce version 2.0 -->
</dependency>
</dependencies>
</dependencyManagement>
2. Transitive Dependencies
Transitive dependencies occur when your project depends on a library that in turn depends on other libraries. Managing these transitive dependencies can become complex, especially in larger projects.
Example:
If LibraryD
depends on LibraryE
, which further depends on LibraryF
, and your project only specifies LibraryD
, it may lead to a situation where LibraryF
is not included or is the wrong version.
Solution:
Again, using Maven or Gradle can help. Both tools, by default, include transitive dependencies automatically. You can explicitly declare or exclude unnecessary transitive dependencies to maintain control over your project’s structure.
<dependency>
<groupId>com.example</groupId>
<artifactId>libraryD</artifactId>
<version>1.0</version>
<exclusions>
<exclusion>
<groupId>com.example</groupId>
<artifactId>libraryE</artifactId>
</exclusion>
</exclusions>
</dependency>
3. Classpath Issues
Classpath misconfigurations can lead to ClassNotFoundException
or NoClassDefFoundError
. These errors are often the result of not properly including all necessary libraries or including incompatible versions.
Example:
Manual inclusion of jar files without proper ordering can lead to classes not being found during runtime.
Solution:
Utilize build automation tools to automatically manage classpaths for various environments (development, testing, production). For Gradle, use the implementation
or runtimeOnly
keywords to control visibility and accessibility.
dependencies {
implementation 'com.example:libraryD:1.0' // Accessible during compilation and runtime
runtimeOnly 'com.example:libraryC:2.0' // Only available at runtime
}
4. Lack of Contextual Information
When managing dependencies manually, developers often miss out on valuable contextual information about dependencies such as licenses, documentation, and updates. This can lead to difficulties in maintaining code over time.
Solution:
Keep documentation for all dependencies updated. This can include details such as the library’s purpose, version history, and change logs. Browser-based dependency management tools allow quick access to this information.
5. Security Vulnerabilities
Using outdated or unsupported libraries can expose your application to security vulnerabilities. Libraries not maintained by an active community or that lack support can pose risks.
Solution:
Regularly monitor your dependencies for updates and security vulnerabilities. Tools like OWASP Dependency-Check can help automate this process. This tool scans your dependencies for known vulnerabilities reported in the NVD (National Vulnerability Database).
# Run OWASP Dependency-Check from command line
dependency-check.sh --project <project-name> --out <output-directory>
6. Performance Issues
Inadequate dependency management can lead to performance issues due to redundant libraries or incompatible versions that consume more resources than necessary.
Example:
A project could inadvertently include multiple logging libraries due to different dependencies, leading to increased memory usage and longer startup times.
Solution:
Conduct a periodic review of your dependencies to identify and eliminate redundancies. Ensure that you only include necessary libraries in your project, and consider shading (bundling dependencies within your own jar file) when conflicts arise.
shadowJar {
archiveClassifier.set('all') // creates a separate jar with all dependencies included
}
Best Practices for Managing Dependencies
-
Use Dependency Management Tools: Always utilize tools like Maven or Gradle to manage your dependencies and avoid manual configurations.
-
Keep Dependencies Updated: Regular updates can help fix bugs and vulnerabilities. Consider using a tool that checks for outdated dependencies.
-
Document Everything: Keep documentations for all major dependencies, including their source, purpose, and any specific version notes.
-
Automate Dependency Checks: Implement automated scripts in your CI/CD pipeline to check for dependency-related issues to minimize human errors.
-
Use Containerization: Consider using Docker to manage dependencies in your runtime environment distinctly. This can help avoid conflicts between different versions of dependencies.
The Closing Argument
Managing dependencies outside the Spring Framework can present various challenges, ranging from version conflicts to performance issues. However, by taking advantage of tools like Maven and Gradle, regularly updating libraries, and maintaining clear documentation, many of these challenges can be mitigated effectively.
For further exploration on managing Java dependencies, you can check the official Maven Documentation and Gradle User Guide. Employ these practices and tools to simplify your dependency management and bolster your application's reliability.
By understanding these challenges and embracing best practices, you will streamline your development process and enhance productivity in your Java projects. The path may be fraught with difficulties, but with the right approach and tools, dependency management can become a manageable aspect of your software development lifecycle.
Checkout our other articles