Map vs. GroupingBy: Key Differences in Java Streams
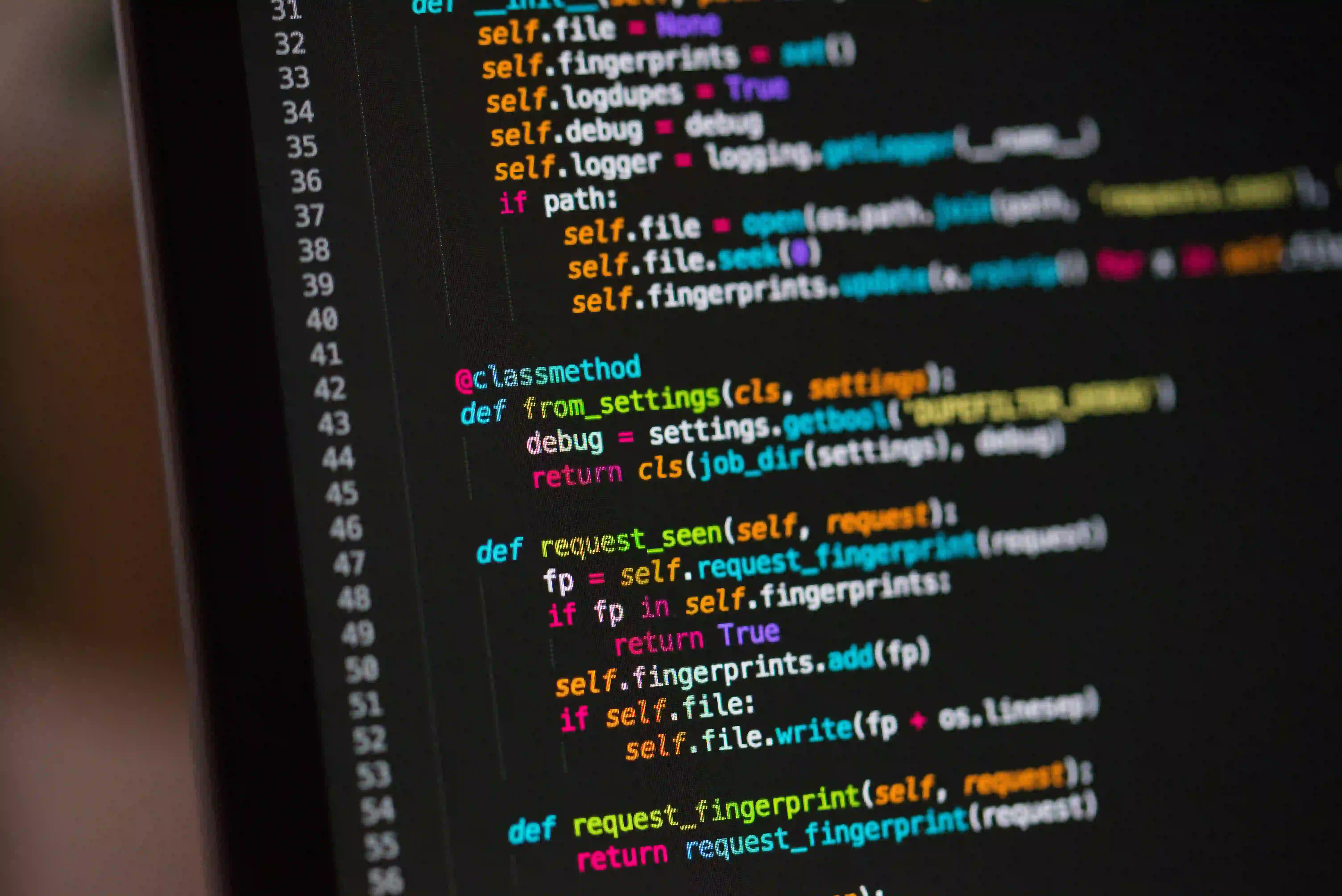
Map vs. GroupingBy: Key Differences in Java Streams
Java Streams API, introduced in Java 8, revolutionized the way we process sequences of data. Two pivotal operations provided by the Streams API are map
and groupingBy
. Understanding the key differences between these two will allow you to use them more effectively in your Java programming. This blog post will delve deep into the functionalities and use cases of both map
and groupingBy
.
What is map
?
The map
operation is primarily utilized for transforming each element in the stream. A map
operation takes a function that defines how to convert an input into an output.
Here is a simple example of the map
function in action:
import java.util.Arrays;
import java.util.List;
import java.util.stream.Collectors;
public class MapExample {
public static void main(String[] args) {
List<String> names = Arrays.asList("John", "Jane", "Jack", "Jill");
// Using map to get the lengths of names
List<Integer> nameLengths = names.stream()
.map(String::length)
.collect(Collectors.toList());
System.out.println("Lengths of names: " + nameLengths);
}
}
Why Use map
?
- Transformation: The primary use case for
map
is transforming data from one form to another. In the above snippet, we transformed a list of names into their corresponding lengths. - Stream-like Behavior: The method returns a new stream which can be further manipulated, allowing chaining of stream operations.
Understanding groupingBy
groupingBy
is a collector that performs a grouping operation on the elements of a stream. It transforms the stream into a Map
where the keys are derived from a classifier function, and the values are Lists of items.
Here is a practical example of how groupingBy
can be used:
import java.util.Arrays;
import java.util.List;
import java.util.Map;
import java.util.stream.Collectors;
public class GroupingByExample {
public static void main(String[] args) {
List<String> names = Arrays.asList("John", "Jane", "Jack", "Jill", "John");
// Using groupingBy to group names by their first letter
Map<Character, List<String>> groupedByFirstLetter = names.stream()
.collect(Collectors.groupingBy(name -> name.charAt(0)));
System.out.println("Grouped by first letter: " + groupedByFirstLetter);
}
}
Why Use groupingBy
?
- Categorization:
groupingBy
is ideal for categorizing data, which makes it perfect for business applications. - Multi-Level Grouping: The feature can have sub-grouping or multi-level grouping which can aid in detailed analytics.
Key Differences: map
vs. groupingBy
Here, we will systematically analyze the main differences between map
and groupingBy
:
1. Primary Functionality
- Map: Transforms each element in the stream, allowing you to apply a function that returns the transformed form of the input.
- GroupingBy: Aggregates the elements of the stream into groups based on a classifier function and produces a
Map
of results.
2. Output Type
- Map: The output is another stream, commonly used to carry the transformed data forward.
- GroupingBy: The output is a
Map<K, List<V>>
, where K is the key derived from the classifier function and V is the type of input elements.
3. Use Cases
- Map: Best suited for single-dimensional transformations like converting objects, extracting fields, or performing calculations.
- GroupingBy: Ideal for multi-dimensional data representation, such as grouping customers by region or employees by department.
4. Performance Considerations
While both map
and groupingBy
internally use a functional interface, performance characteristics can vary based on the size of the dataset and operations performed:
- Map: Generally has lower overhead and quicker response times for transformations.
- GroupingBy: While powerful, it could introduce latency due to mapping and subsequent classification into groups.
When to Use Each
Choosing between map
and groupingBy
can often depend on the specific requirements of your problem:
- Use map when you need to transform the data into another form without concern for grouping.
- Use groupingBy when your goal is categorizing data points based on certain attributes.
In Conclusion, Here is What Matters
In conclusion, understanding the key differences between map
and groupingBy
allows Java developers to leverage the Streams API effectively. Both methods serve distinct purposes and can be utilized together to create robust and efficient data processing pipelines. The versatility of Streams combined with the right function will enable cleaner, more manageable, and efficient code.
For further reading, you can explore the official documentation on Java Stream API for more detailed functionalities and advanced techniques.
By mastering these concepts, you’ll be well on your way to becoming a more proficient Java programmer. Consider experimenting with both methods in your projects to understand their practical differences further. Happy coding!