Managing Microservices Complexity: Key Strategies for Success
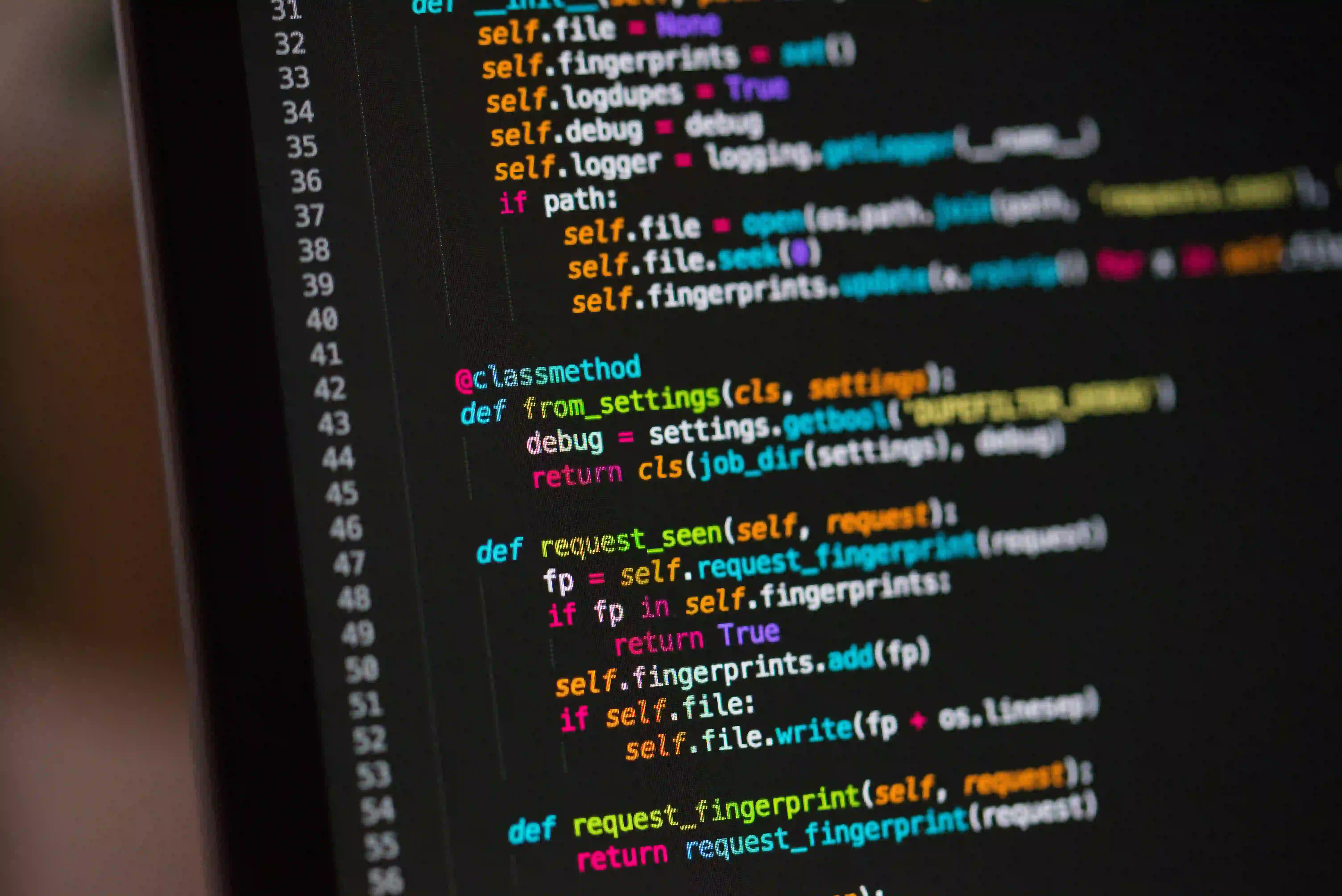
Managing Microservices Complexity: Key Strategies for Success
In recent years, microservices architecture has increasingly become the go-to solution for many organizations that focus on scalability, agility, and maintainability. However, as attractive as the prospect of microservices is, they come with a unique set of challenges—complexity being the most significant. In this blog post, we will explore various strategies for effectively managing the complexity of microservices.
Understanding the Complexity of Microservices
Before diving into the solutions, let's first understand the complexity involved in a microservices architecture. Unlike a monolithic architecture, where everything is in one place, microservices break applications into smaller, loosely coupled services; each service performs a single function. This design pattern provides flexibility but also leads to complications such as:
- Service Communication: Microservices often need to communicate with one another, and managing this communication can become complex.
- Data Management: Each service may have its own database, which can lead to data inconsistency.
- Deployment Challenges: With many independent services, deployment and monitoring become intricate.
- Security Concerns: Each service must be secured while accommodating for inter-service communication.
Key Strategies for Managing Microservices Complexity
1. Embrace Domain-Driven Design (DDD)
Why DDD?
Domain-Driven Design (DDD) helps in structuring microservices around business functionalities. By doing so, each service is responsible for a specific domain or bounded context, leading to better alignment with business needs.
public class OrderService {
private OrderRepository orderRepository;
public OrderService(OrderRepository orderRepository) {
this.orderRepository = orderRepository;
}
public void placeOrder(Order order) {
// Business logic for placing an order
orderRepository.save(order);
}
}
Commentary:
In the example above, the OrderService
class concentrates solely on the order domain. This isolation results in clear responsibilities and easier maintenance. When business requirements change, only the affected microservice requires updates, keeping the rest of the system stable.
For more insights on DDD, you can refer to Martin Fowler's blog.
2. Implement API Gateway
Why API Gateway?
An API Gateway serves as a single entry point for the whole microservices architecture. It can handle requests, route them to the appropriate microservices, and aggregate the responses.
@RestController
@RequestMapping("/api")
public class ApiGatewayController {
@Autowired
private RestTemplate restTemplate;
@GetMapping("/orders")
public ResponseEntity<?> getOrders() {
ResponseEntity<Order[]> response = restTemplate.getForEntity(
"http://order-service/orders", Order[].class);
return ResponseEntity.ok(response.getBody());
}
}
Commentary:
The ApiGatewayController
in this example uses Spring's RestTemplate
to communicate with the order service. An API Gateway consolidates multiple service calls into one and can handle cross-cutting concerns like authentication and logging, further alleviating complexity.
3. Use Service Mesh for Resilience
Why Service Mesh?
A service mesh handles service-to-service communication, providing a dedicated infrastructure layer for managing networking. This can improve observability, security, and reliability without modifying the microservices’ code.
# Sample Istio Virtual Service Configuration
apiVersion: networking.istio.io/v1alpha3
kind: VirtualService
metadata:
name: order-service
spec:
hosts:
- order-service
http:
- route:
- destination:
host: order-service
port:
number: 80
Commentary:
In this YAML configuration for Istio, we define a virtual service to route traffic to the order-service
. This abstraction allows developers to focus on business logic while the service mesh takes care of sophisticated routing, fault tolerance, and load balancing.
4. Monitor and Log Effectively
Why Monitoring and Logging?
Microservices generate considerable operational complexity, and effective monitoring can preemptively catch failures. Logging helps in tracing issues across services effectively.
- Distributed Tracing: Tools like Jaeger and Zipkin allow you to track requests as they navigate through various microservices.
Example: Using Spring Cloud Sleuth for distributed tracing
@Bean
public Sampler defaultSampler() {
return Sampler.ALWAYS_SAMPLE; // Sample all requests for tracing
}
Commentary:
By configuring the sampler, you can monitor all requests, making it more straightforward to pinpoint where issues arise within the microservices network.
5. Automate Deployment with CI/CD
Why Continuous Integration/Continuous Deployment (CI/CD)?
Automation in testing and deployment minimizes the risk of errors and enhances speed. Each microservice can be updated independently, resulting in quicker iterations and more robust software.
# Sample GitHub Actions CI/CD configuration
name: CI/CD Pipeline
on:
push:
branches:
- main
jobs:
build:
runs-on: ubuntu-latest
steps:
- name: Check out code
uses: actions/checkout@v2
- name: Build with Maven
run: mvn clean install
Commentary:
In this GitHub Actions example, the CI/CD pipeline is designed to build microservices upon code pushes, ensuring that changes are automatically tested and deployed. This automation significantly reduces the complexities that come with manual deployments.
The Last Word
While microservices offer substantial advantages, the complexity they introduce can't be overlooked. By embracing strategies like Domain-Driven Design, implementing an API Gateway, using a service mesh, monitoring effectively, and automating deployments with CI/CD, organizations can navigate these complexities.
Mastering the management of microservices will not only improve application performance but will also pave the way for a more agile and robust development process.
For a deeper dive into microservices and related technologies, be sure to check out Spring Boot documentation and Microservices Patterns by Chris Richardson.
The world of microservices is dynamic, and staying informed about best practices will keep you one step ahead in managing complexity effectively. Happy coding!