Mastering Map Markers: A Common Android Pitfall
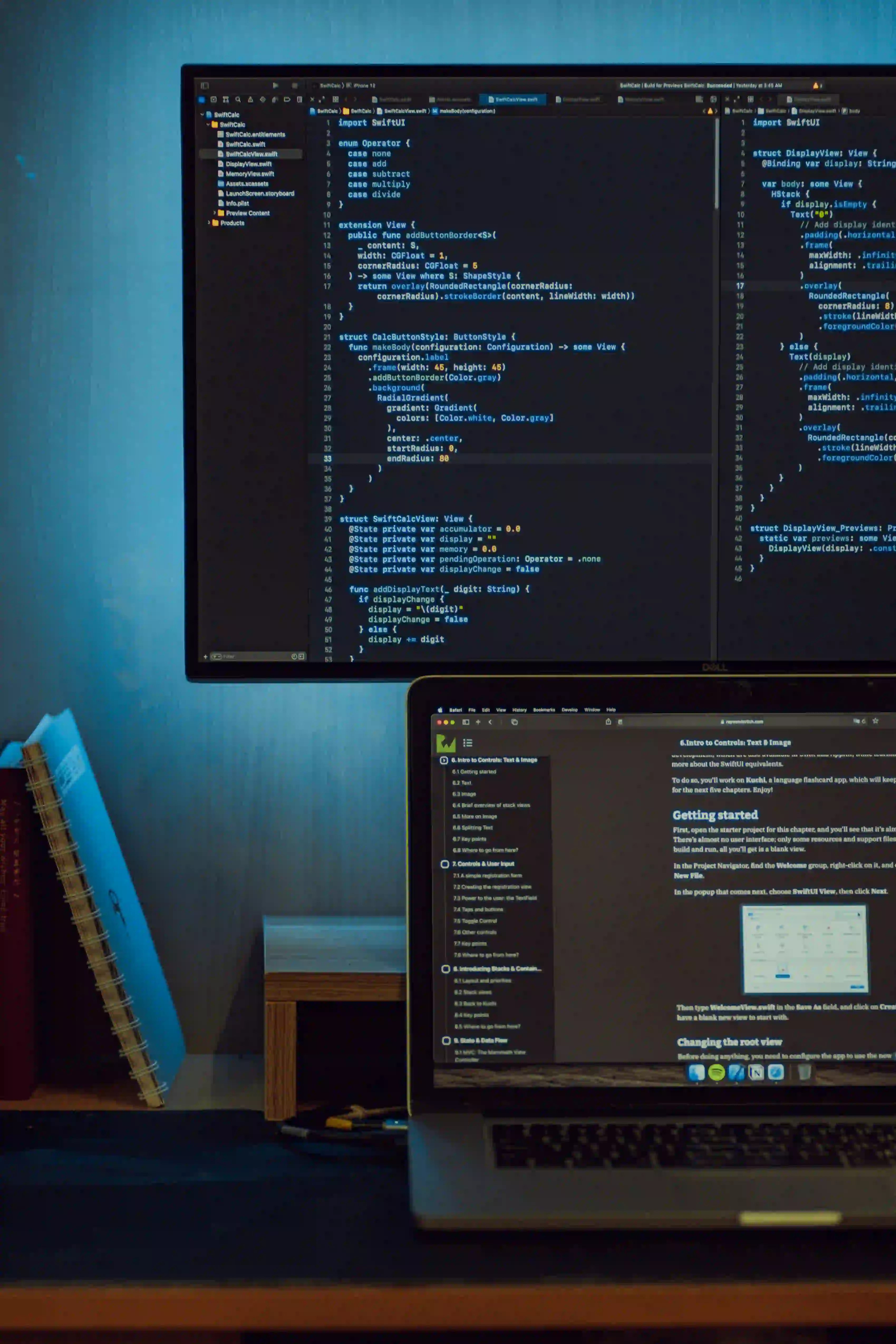
Mastering Map Markers: A Common Android Pitfall
Maps are a powerful feature in mobile applications, often utilized to provide users with visual contexts and locations. In Android development, integrating maps can sometimes come with its set of challenges. One common pitfall is correctly managing map markers. In this blog post, we’ll explore how to effectively use map markers in Android, common pitfalls, and solutions to help you streamline your map-related tasks.
Why Use Google Maps in Android?
Google Maps provides a robust framework for developers to create interactive and location-based applications. Features include geolocation, route mapping, and marker placement—which allows developers to annotate specific places on the map. If done well, these features can significantly enhance user experience. However, improper handling of map markers can lead to performance issues, cluttered interfaces, and a poor user experience.
Before delving into common pitfalls, let's take a brief look at how to set up Google Maps in your Android project.
Setting Up Google Maps
1. Adding the Dependencies
First, you need to add the relevant dependencies in your build.gradle
file:
dependencies {
implementation 'com.google.android.gms:play-services-maps:17.0.1'
}
This line imports the Google Maps library necessary to work with maps in your application.
2. Obtaining an API Key
Next, you must obtain an API key from the Google Cloud Console. This key allows your app to access Google Maps services.
3. Declaring Permissions
Don't forget to declare permissions in your AndroidManifest.xml
:
<uses-permission android:name="android.permission.ACCESS_FINE_LOCATION"/>
<uses-permission android:name="android.permission.INTERNET"/>
This allows your app to access the device's location and the internet.
4. Initializing the Map
After setting up the above, we can initialize the Map in our Activity:
public class MapActivity extends AppCompatActivity implements OnMapReadyCallback {
private GoogleMap mMap;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_map);
SupportMapFragment mapFragment = (SupportMapFragment) getSupportFragmentManager()
.findFragmentById(R.id.map);
mapFragment.getMapAsync(this);
}
@Override
public void onMapReady(GoogleMap googleMap) {
mMap = googleMap;
// Add a marker in Sydney and move the camera
LatLng sydney = new LatLng(-34, 151);
mMap.addMarker(new MarkerOptions().position(sydney).title("Marker in Sydney"));
mMap.moveCamera(CameraUpdateFactory.newLatLng(sydney));
}
}
In this code, we set up a basic map and add a marker for Sydney. Understanding the setup ensures that you're ready to tackle more complex tasks.
Common Pitfalls of Using Map Markers
Having established the fundamentals, we now can discuss common pitfalls associated with managing map markers.
1. Overcrowding the Map with Markers
Adding too many markers at once can clutter the map, making it difficult for users to focus. This issue typically arises when fetching data from a server to display, leading to performance degradation.
Solution: Clustering Markers
Using Google Maps' marker clustering feature alleviates this issue. It groups multiple markers into one when they are within close proximity, decluttering your map.
ClusterManager<MyItem> mClusterManager;
@Override
public void onMapReady(GoogleMap googleMap) {
mClusterManager = new ClusterManager<>(this, googleMap);
googleMap.setOnCameraIdleListener(mClusterManager);
// Adding items to the cluster
addItem(new LatLng(-34, 151));
addItem(new LatLng(-35, 152));
}
private void addItem(LatLng latLng) {
MyItem offsetItem = new MyItem(latLng.latitude, latLng.longitude);
mClusterManager.addItem(offsetItem);
}
The code snippet uses a ClusterManager
to group markers. This promotes a cleaner map and improves performance.
2. Not Customizing Markers
Using the default marker can diminish your app's uniqueness and branding. It does not convey additional context, which may be necessary for your users.
Solution: Custom Marker Imagery
You can easily customize the appearance of your markers by using images that represent your data type. Here’s how:
mMap.addMarker(new MarkerOptions()
.position(sydney)
.icon(BitmapDescriptorFactory.fromResource(R.drawable.custom_marker)));
This approach enhances the visual appeal of your map and reinforces your branding.
3. Failing to Handle Marker Click Events
Ignoring click events for markers can lead to missed opportunities, such as displaying relevant information or directing users to additional actions.
Solution: Handling Marker Clicks
Implement GoogleMap.OnMarkerClickListener
to enable custom actions when a marker is clicked.
mMap.setOnMarkerClickListener(new GoogleMap.OnMarkerClickListener() {
@Override
public boolean onMarkerClick(Marker marker) {
// Handle marker click
Toast.makeText(getApplicationContext(), "Clicked: " + marker.getTitle(), Toast.LENGTH_SHORT).show();
return false;
}
});
This snippet provides immediate feedback to the user, enriching their interaction with your application.
4. Poor Performance with Too Many Updates
Constantly updating the map with new markers without optimizing can lead to bad performance. Every update requires redrawing the map, leading to potential lag.
Solution: Debounce Marker Updates
When updating markers, implement a debouncing technique to limit the frequency of updates triggered in a short timeframe. Here’s an example with a timer:
private Timer timer;
private final long DELAY = 300; // milliseconds
private void scheduleMarkerUpdate(LatLng newPosition) {
if (timer != null) {
timer.cancel();
}
timer = new Timer();
timer.schedule(new TimerTask() {
@Override
public void run() {
runOnUiThread(() -> updateMarkers(newPosition));
}
}, DELAY);
}
private void updateMarkers(LatLng newPosition) {
// Logic to update markers
}
Debouncing helps optimize performance, ensuring a smoother user experience.
Best Practices for Using Map Markers
Understanding the pitfalls is crucial for avoiding them, but here are some best practices to keep in mind:
- Use Marker Clustering: This reduces visual clutter and improves performance.
- Customize Marker Icons: Use unique icons to enhance user experience and maintain your brand identity.
- Handle Clicks Effectively: Respond to user actions in a meaningful way.
- Optimize Updates: Debounce frequent updates to maintain performance.
- Coordinate System Awareness: Familiarize yourself with different coordinate systems and their peculiarities to avoid errors.
Bringing It All Together
Successfully mastering map markers in your Android application requires vigilance against common pitfalls and a basic understanding of effective practices. With the strategies highlighted above—ranging from clustering markers to customizations—you will be well on your way to creating a dynamic and user-friendly mapping experience.
If you are interested in further improving your Android skills or want more in-depth resources on Google Maps, consider visiting the Android Developers Documentation for comprehensive tutorials and guides.
By mastering these concepts, you not only create a better app but also enhance the overall user experience—a crucial aspect in today’s competitive app market. Happy coding!