Mastering Java ChronoUnit Enum for Better Date Handling
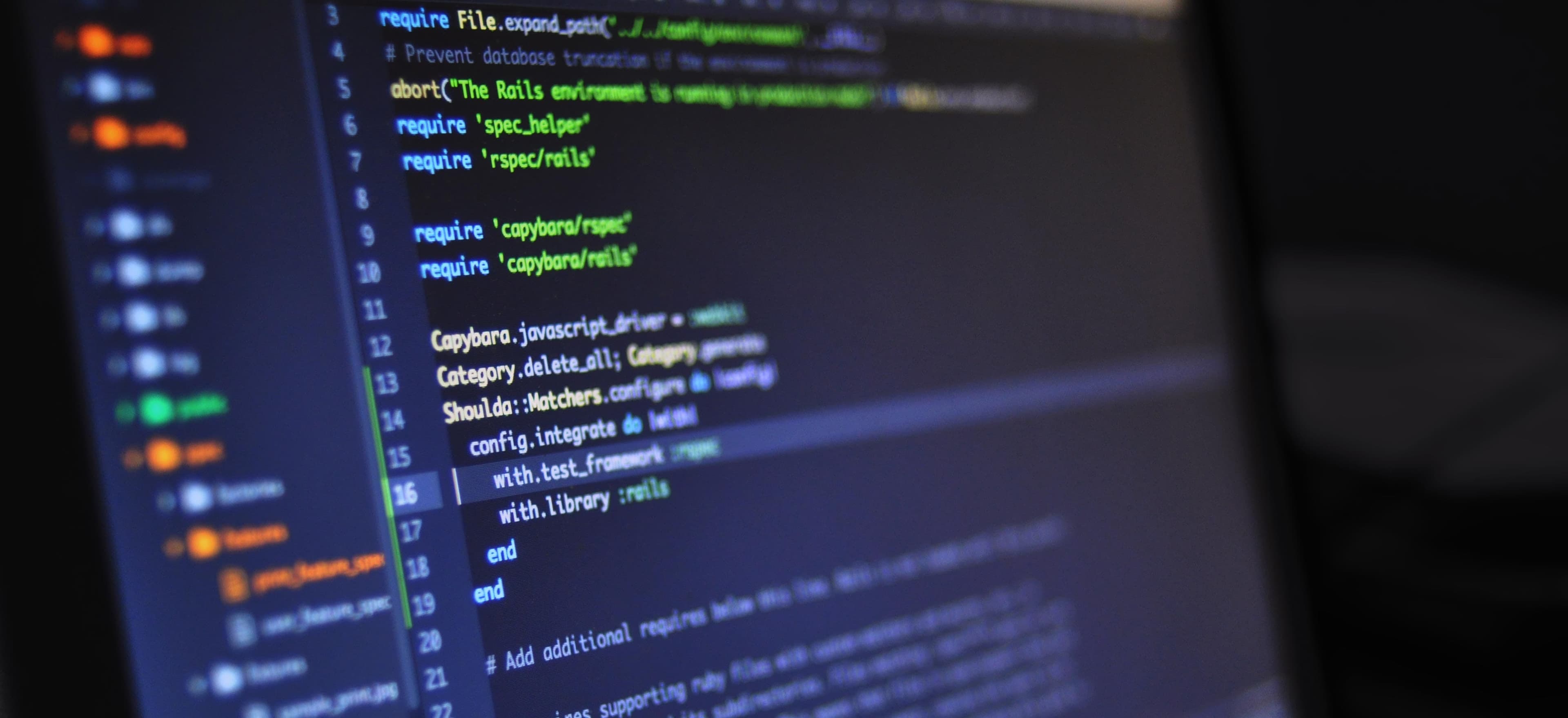
- Published on
Mastering Java ChronoUnit
Enum for Better Date Handling
Handling dates and times efficiently is a pivotal aspect of software development. In Java, the java.time
package introduced in Java 8 has revolutionized date-time handling. Among its many classes, the ChronoUnit
enum stands out as a powerful tool offering a rich API for date-time manipulations. In this post, we'll explore the ChronoUnit
enum in detail, discussing its applications, benefits, and practical examples to enhance your Java programming skills.
What is the ChronoUnit
Enum?
The ChronoUnit
enum provides a machine-readable list of standard units of time. It encompasses various time units, including DAYS
, HOURS
, MINUTES
, SECONDS
, and YEARS
, among others. This enum is a part of the new Date and Time API, introduced to overcome the limitations and complexities of the old java.util.Date
and java.util.Calendar
classes.
Why Use ChronoUnit
?
Using ChronoUnit
simplifies date manipulations by providing a clear, immutable, and type-safe way to work with time units. This approach reduces errors by avoiding magic numbers or strings that are often prevalent in date calculations.
How to Get Started with ChronoUnit
To start using ChronoUnit
, ensure you have Java 8 or later installed. Import the required classes at the beginning of your Java file:
import java.time.LocalDate;
import java.time.temporal.ChronoUnit;
Basic Usage of ChronoUnit
The primary operations provided by ChronoUnit
include adding to or subtracting from a date, comparing dates, and calculating the difference between two dates.
Adding Days to a LocalDate
Let’s begin with a practical example of adding days to a date:
public class ChronoUnitExample {
public static void main(String[] args) {
LocalDate today = LocalDate.now();
LocalDate nextWeek = today.plus(1, ChronoUnit.WEEKS);
System.out.println("Today's Date: " + today);
System.out.println("Date Next Week: " + nextWeek);
}
}
In this snippet:
- We obtain the current date using
LocalDate.now()
. - The
plus
method adds one week to the current date, leveragingChronoUnit.WEEKS
.
Subtracting Months from a LocalDate
Similarly, you can subtract months. Two scenarios often arise in date handling: scheduling and expiration. The following code snippet exemplifies how to calculate an expiration date by subtracting months:
public class DateExpiration {
public static void main(String[] args) {
LocalDate today = LocalDate.now();
LocalDate expirationDate = today.minus(3, ChronoUnit.MONTHS);
System.out.println("Today's Date: " + today);
System.out.println("Expiration Date: " + expirationDate);
}
}
In this example:
- The
minus
method efficiently removes three months from the current date.
Calculating the Difference Between Dates
A frequent requirement is to determine the time difference between two dates:
public class DateDifference {
public static void main(String[] args) {
LocalDate startDate = LocalDate.of(2023, 1, 1);
LocalDate endDate = LocalDate.now();
long daysBetween = ChronoUnit.DAYS.between(startDate, endDate);
System.out.println("Days Between: " + daysBetween);
}
}
Key takeaway:
- The
between
method fromChronoUnit
directly calculates the number of days elapsed between twoLocalDate
instances.
Additional ChronoUnit Operations
ChronoUnit
also provides several additional methods for more complex date manipulations, such as:
YEARS
: For working with years.HOURS
: For calculating hours between timestamps.MONTHS
: For month-based calculations.
Example: Working with Timestamps
Here's an example that demonstrates the use of ChronoUnit
for timestamps:
import java.time.LocalDateTime;
import java.time.temporal.ChronoUnit;
public class TimestampExample {
public static void main(String[] args) {
LocalDateTime start = LocalDateTime.now();
LocalDateTime end = start.plus(3, ChronoUnit.HOURS);
long secondsBetween = ChronoUnit.SECONDS.between(start, end);
System.out.println("Start Time: " + start);
System.out.println("End Time: " + end);
System.out.println("Seconds Between: " + secondsBetween);
}
}
Breaking Down the Example:
LocalDateTime.now()
generates the current timestamp.- We add three hours to this timestamp.
- The code uses the
between
method fromChronoUnit.SECONDS
to calculate the difference in seconds.
Advantages of Using ChronoUnit
- Readability: Clear naming conventions make the intention of the code immediately obvious.
- Type Safety: By using enums, you ensure that only valid time units can be used, minimizing errors.
- Interoperability:
ChronoUnit
works seamlessly with other Java time API components, creating a cohesive framework for date-time manipulation.
Practical Scenarios
While ChronoUnit
offers essential functionality for basic date calculations, real-world applications can involve more intricate scenarios, such as calculating fiscal quarters or setting reminders based on weeks or months.
Example: Calculating Next Quarter Start Date
import java.time.LocalDate;
import java.time.temporal.ChronoUnit;
public class FiscalQuarter {
public static void main(String[] args) {
LocalDate today = LocalDate.now();
LocalDate nextQuarterStart = today.plus(3, ChronoUnit.MONTHS).withDayOfMonth(1);
System.out.println("Next Quarter Starting Date: " + nextQuarterStart);
}
}
Key Takeaways
Mastering the ChronoUnit
enum is essential for Java developers aiming to handle dates and times efficiently. By utilizing its straightforward methods, we can perform complex date manipulations elegantly without falling prey to the common pitfalls of date handling.
With the enriched features provided by Java's new Date and Time API, including classes like LocalDate
, LocalTime
, and LocalDateTime
, along with ChronoUnit
, developers can ensure that their applications remain reliable and easy to maintain.
To dive deeper into the Java Date Time API, you may refer to the official documentation here.
Final Tips
- Always leverage
ChronoUnit
for date manipulations instead of using traditional libraries, such asjava.util.Date
, to benefit from the improvements in performance and clarity. - When working with time zones, consider using
ZonedDateTime
for better accuracy and control over your date-time objects.
Feel free to explore further and implement these concepts in your next Java project! Happy coding!
Checkout our other articles