Eliminating Rogue Spaces in CSV Headers for Neo4j
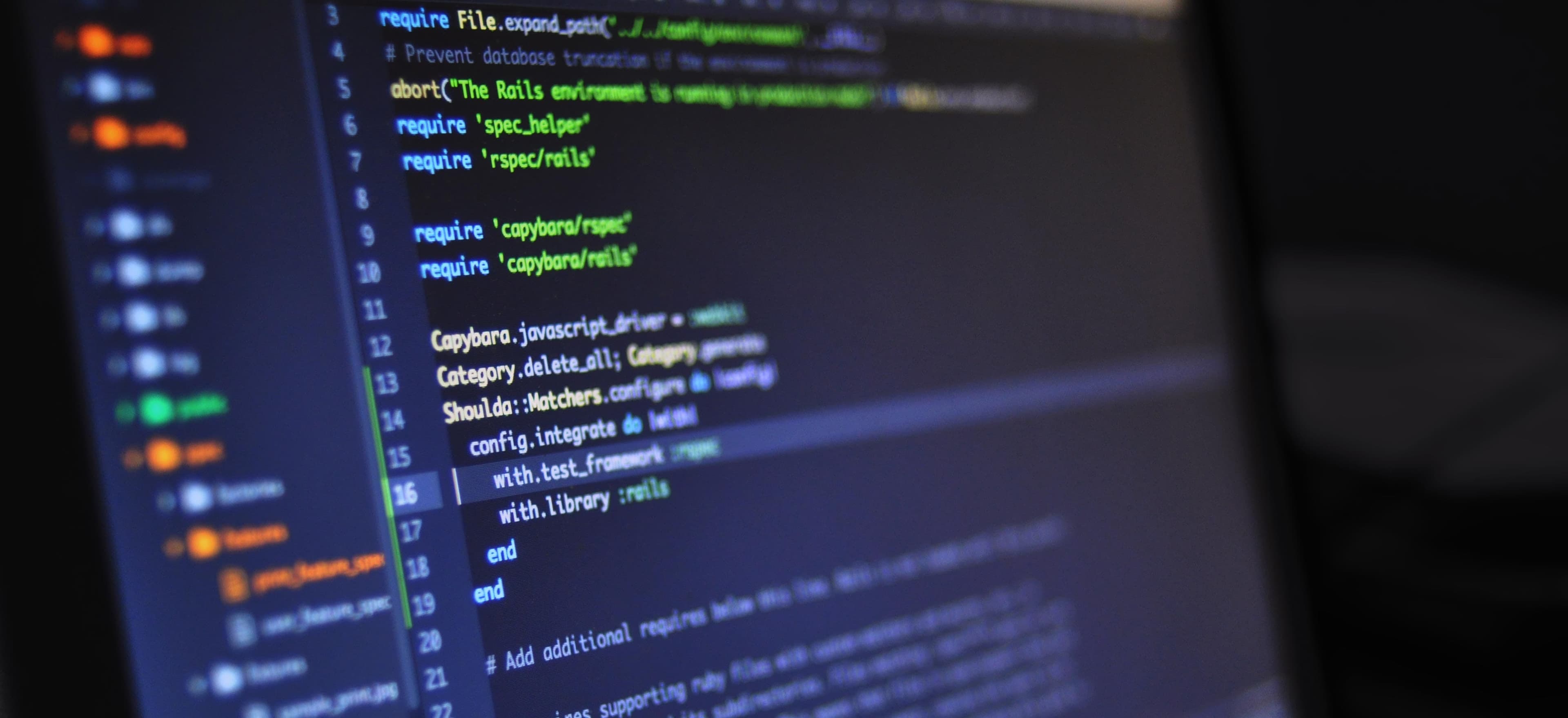
- Published on
Eliminating Rogue Spaces in CSV Headers for Neo4j
When working with CSV files to import data into Neo4j, one common issue developers face is rogue spaces in the headers. These extraneous spaces can lead to unexpected behavior during the import process, making it crucial to clean your CSV files before using them. In this blog post, we will cover methods to identify and eliminate these rogue spaces, ensuring a smooth import to your Neo4j database.
Understanding the Problem
Rogue spaces refer to undesired whitespace that precedes or follows a header name in a CSV file. For instance, a CSV with a header like " Name "
can generate confusion during querying, leading to errors in recognizing the node properties.
Consider the following sample CSV:
Name , Age , Country
Alice , 30 , USA
Bob , 25 , Canada
The additional spaces around the header names mean that any attempts to refer to the Name
property in Cypher query will require you to account for those spaces, which can introduce bugs and make the code less readable:
LOAD CSV WITH HEADERS FROM 'file:///test.csv' AS row
CREATE (:Person {name: row." Name ", age: toInteger(row." Age "), country: row." Country "})
Why Clean Your Headers
Cleaning the headers of your CSV files before import is essential for several reasons:
- Consistency: Ensures uniformity in how you reference node properties.
- Error-Free Queries: Prevents errors during Cypher queries.
- Professionalism: Producing clean data files reflects well on your work ethic.
- Performance: While minor, cleaning headers might slightly enhance performance during the import process.
Step-by-Step Approach to Eliminate Rogue Spaces
Step 1: Identify Rogue Spaces
Before eliminating rogue spaces, you need a strategy to identify them. One way is to use basic string operations in languages like Python, which grants you the ability to manipulate and inspect strings effectively.
Here’s a Python snippet that reads through a CSV file and identifies headers with rogue spaces:
import csv
def identify_rogue_spaces(filename):
with open(filename, 'r', newline='') as csvfile:
reader = csv.reader(csvfile)
headers = next(reader)
for header in headers:
if header.strip() != header:
print(f"Rogue spaces found in header: '{header}'")
identify_rogue_spaces('data.csv')
Commentary on the Code
- The function
identify_rogue_spaces
reads a CSV file. - It uses the
csv.reader
to extract headers. - The
strip()
method checks for leading or trailing spaces – a simple yet effective solution.
Step 2: Eliminate Rogue Spaces
Once rogue spaces are identified, it's time to clean them. We can build upon the previous example to achieve this:
def clean_headers(filename, output_filename):
with open(filename, 'r', newline='') as csvfile:
reader = csv.reader(csvfile)
headers = next(reader)
cleaned_headers = [header.strip() for header in headers]
with open(output_filename, 'w', newline='') as cleaned_csvfile:
writer = csv.writer(cleaned_csvfile)
writer.writerow(cleaned_headers)
for row in reader:
writer.writerow(row)
clean_headers('data.csv', 'cleaned_data.csv')
Commentary on the Code
clean_headers
reads the input CSV and processes the headers.- List comprehension is utilized for efficient cleaning of spaces.
- Finally, the cleaned headers and data get written to a new CSV.
Step 3: Validate the Cleaned CSV
After cleaning your CSV file, it is essential to validate that the headers are free of rogue spaces.
def validate_cleaned_headers(filename):
with open(filename, 'r', newline='') as csvfile:
reader = csv.reader(csvfile)
headers = next(reader)
for header in headers:
assert header.strip() == header, f"Rogue spaces still present in header: '{header}'"
print("All headers validated successfully.")
# Validate the cleaned file
validate_cleaned_headers('cleaned_data.csv')
Commentary on the Code
- The
validate_cleaned_headers
function checks the cleaned CSV. - Assertions raise exceptions if any rogue space remains, ensuring data integrity.
Importing Clean Data into Neo4j
With the cleansed CSV ready, let's move on to importing the data into Neo4j. Use the following Cypher query to create nodes based on the cleaned data:
LOAD CSV WITH HEADERS FROM 'file:///cleaned_data.csv' AS row
CREATE (:Person {name: row.Name, age: toInteger(row.Age), country: row.Country})
Commentary on the Query
- The Cypher query imports data with clean headers.
- Referencing the cleaned headers ensures that there are no errors concerning rogue spaces.
My Closing Thoughts on the Matter
Eliminating rogue spaces in CSV headers is a vital step to ensure successful data import into Neo4j. By employing Python scripts to identify, clean, and validate headers, you can enhance your data’s integrity and consistency. As a result, this paves the path for more efficient querying and management of your graph database.
For more insights into handling CSV files in programming, check Python's CSV documentation or explore Neo4j's CSV import capabilities.
Happy coding!
Checkout our other articles