Mastering JDK 12: Common Pitfalls of Switch Expressions
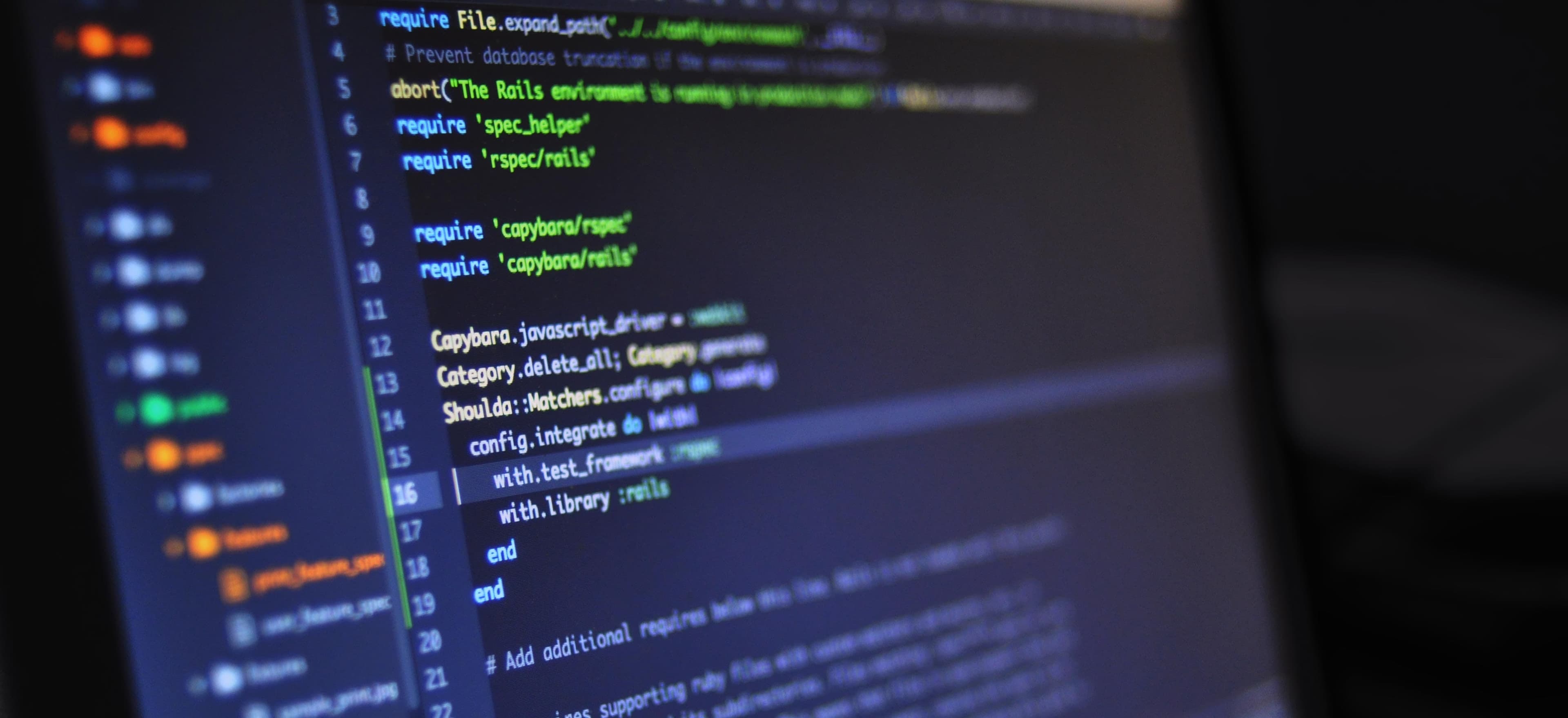
- Published on
Mastering JDK 12: Common Pitfalls of Switch Expressions
Java Development Kit (JDK) 12 brought significant enhancements to the Java programming language, one of which is the intuitive and expressive switch expressions. These expressions simplify coding and enhance the readability of conditional statements. However, like any feature, switch expressions come with their own set of pitfalls. In this blog post, we will explore these common pitfalls and how to effectively avoid them.
Understanding Switch Expressions
Before diving into the pitfalls, let's quickly review what switch expressions are. Traditionally, the switch statement in Java was limited to executing multiple cases without returning a value directly. In contrast, switch expressions allow you to use the switch construct in an expression context, returning a value.
Here’s a basic example showcasing a switch expression:
int dayOfWeek = 2;
String dayName = switch (dayOfWeek) {
case 1 -> "Monday";
case 2 -> "Tuesday";
case 3 -> "Wednesday";
case 4 -> "Thursday";
case 5 -> "Friday";
case 6 -> "Saturday";
case 7 -> "Sunday";
default -> throw new IllegalArgumentException("Invalid day: " + dayOfWeek);
};
System.out.println(dayName);
In this snippet, the switch expression evaluates dayOfWeek
and assigns the corresponding day name. This concise syntax not only simplifies coding but also eliminates the need for break statements.
Common Pitfalls
Switch expressions introduce a new syntax and behavior that can lead to several pitfalls if developers are unfamiliar with them. Below, we explore some of these common mistakes and provide clarity and solutions.
1. Forgetting to Handle All Cases
One of the significant advantages of switch expressions is that they enforce completeness. Failure to include all possible cases will lead to a compilation error. For example, omitting the default case can cause issues if a value outside the defined cases is passed.
Pitfall Example:
int dayOfWeek = 8; // Invalid case
String dayName = switch (dayOfWeek) {
case 1 -> "Monday";
case 2 -> "Tuesday";
case 3 -> "Wednesday";
// Missing cases for 4 and 5
};
The Fix: Always include a default
clause in your switch expression, or handle all possible cases to ensure reliability.
default -> throw new IllegalArgumentException("Invalid day: " + dayOfWeek);
2. Misunderstanding the Use of Yield
In JDK 12, a key keyword introduced alongside switch expressions is yield
. When using switch expressions, you should remember that yield
is the mechanism to return a value from a switch block more complex than a simple single-line statement.
Pitfall Example:
String result = switch (someValue) {
case 1 -> "One";
case 2 -> "Two";
case 3 -> {
System.out.println("Three selected");
"Three"; // Incorrect, needs 'yield'
}
};
The Fix: Use yield
to return values from a block case.
case 3 -> {
System.out.println("Three selected");
yield "Three"; // Now it's correct
}
3. Mixing Switch Statements and Switch Expressions
Switch statements and switch expressions are not interchangeable. Attempting to mix them can result in unexpected behavior, especially when reusing case
labels.
Pitfall Example:
switch (dayOfWeek) {
case 1 -> System.out.println("Monday");
case 2 -> return "Tuesday"; // Error: return statements are not valid in switch blocks
}
The Fix: Consistanly use either switch statements or switch expressions according to their design and purpose.
String dayName = switch (dayOfWeek) {
case 1 -> "Monday";
case 2 -> "Tuesday"; // Correctly returns a value
};
4. Not Taking Advantage of Multi-Case Labels
Switch expressions allow you to utilize multi-case labels neatly, improving readability. However, many still use separate cases unnecessarily.
Pitfall Example:
String status = switch (statusCode) {
case 200 -> "OK";
case 204 -> "No Content";
case 404 -> "Not Found";
case 500 -> "Server Error";
case 403 -> "Forbidden";
default -> "Unknown Status";
};
The Fix: You can group cases that yield the same result to enhance maintainability.
String status = switch (statusCode) {
case 200, 204 -> "Successful Response";
case 404, 403 -> "Client Error";
case 500 -> "Server Error";
default -> "Unknown Status";
};
This grouping facilitates easier updates if more status codes are added in the future.
5. Neglecting to Test All Cases
Just because your switch expression compiles successfully does not guarantee it functions as expected. Failing to test all possible paths can lead to unexpected runtime errors.
Pitfall Example:
If your program receives user input, and your switch expression doesn’t appropriately validate input, you could encounter issues.
The Fix: Always ensure exhaustive testing. Unit tests can help cover cases you may have missed. Here's an example of a simple unit test using JUnit:
import org.junit.jupiter.api.Test;
import static org.junit.jupiter.api.Assertions.*;
class DayOfWeekTest {
@Test
void testValidDays() {
assertEquals("Monday", getDay(1));
assertEquals("Tuesday", getDay(2));
assertEquals("Unknown Day", getDay(8));
}
String getDay(int day) {
return switch (day) {
case 1 -> "Monday";
case 2 -> "Tuesday";
case 3 -> "Wednesday";
case 4 -> "Thursday";
case 5 -> "Friday";
default -> "Unknown Day";
};
}
}
Using tests ensures that your switch expressions behave correctly and can handle unexpected input.
Wrapping Up
Switch expressions in JDK 12, while powerful, come with pitfalls that developers must be aware of to avoid errors and misunderstandings. From ensuring you handle all cases, properly utilizing yield
, maintaining a clear distinction between switch statements and expressions, to leveraging multi-case labels, these practices can greatly improve the usability and reliability of your code.
If you are looking to implement these effectively and increase your Java knowledge further, check out the official Java documentation for more on JDK enhancements and best practices in code writing.
By mastering switch expressions and avoiding these pitfalls, you'll enhance code readability, maintainability, and overall programming efficiency. Happy coding!