Transitioning from JPA to Hibernate: Identifier Challenges
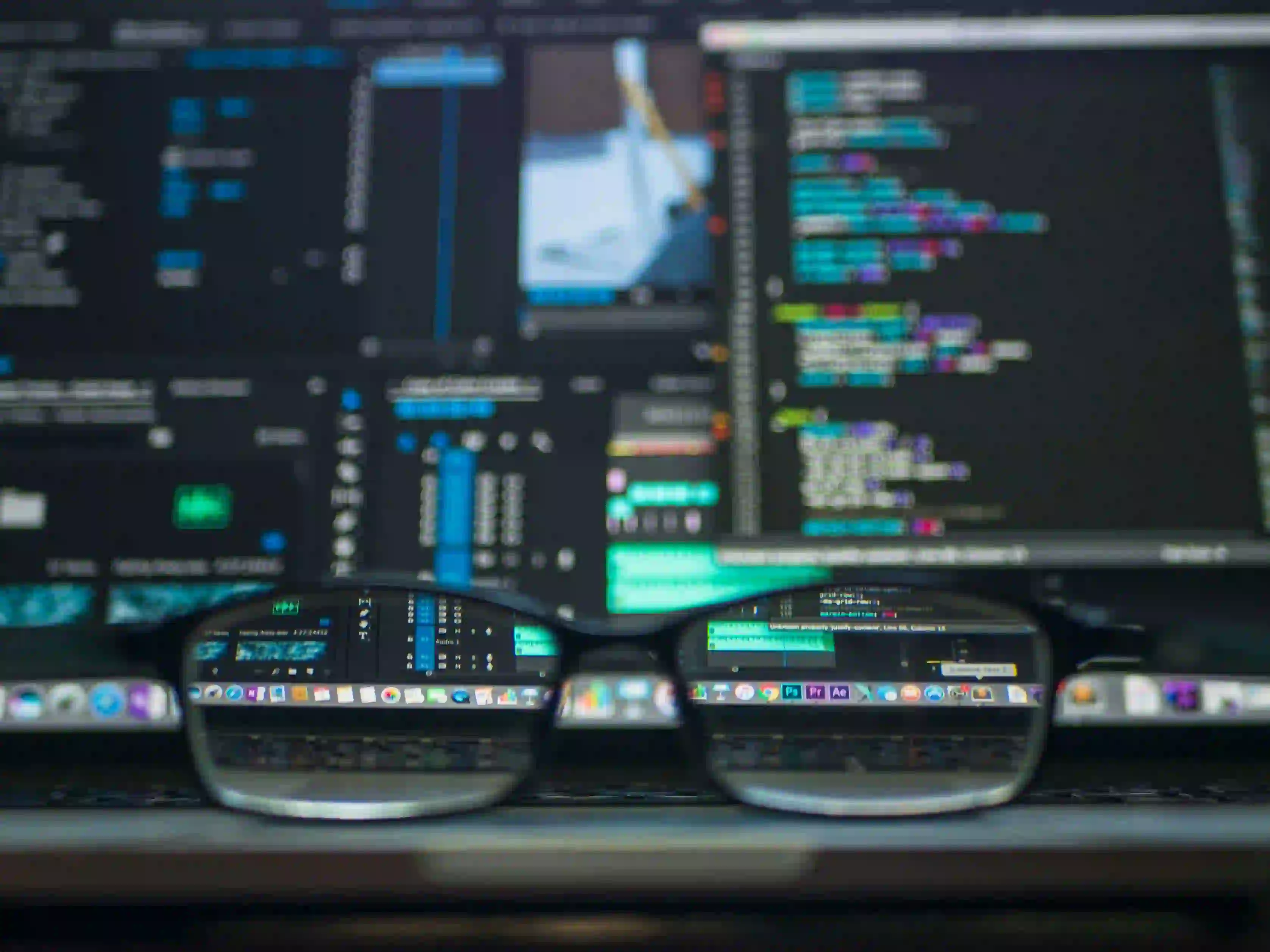
Transitioning from JPA to Hibernate: Identifier Challenges
In the world of Java persistence, JPA (Java Persistence API) and Hibernate are often used interchangeably, yet they serve distinct purposes and offer unique functionalities. While JPA is a specification that dictates how to persist and access data in Java applications, Hibernate is a widely adopted framework that implements this specification along with additional features.
When transitioning from JPA to Hibernate, developers often encounter a range of challenges, especially with identifier management. In this post, we will explore these identifier challenges, providing solutions and tips that can aid in a smoother transition.
Understanding Identifiers in JPA and Hibernate
Definition of Identifiers
In Java persistence, an identifier, or primary key, uniquely identifies an entity. It is critical for the integrity of database operations, ensuring that each row in a database table remains distinct.
JPA Annotations
In JPA, you use the @Id
annotation to specify the primary key. You can also use @GeneratedValue
to define how the primary key should be generated, for instance, using an auto-increment strategy or a UUID.
import javax.persistence.*;
@Entity
public class User {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String username;
// Constructors, getters, and setters
}
Hibernate Enhancements
Hibernate builds on JPA's capabilities by providing additional strategies for generating identifiers. For example, it supports @GenericGenerator
which can yield more customizability.
import org.hibernate.annotations.GenericGenerator;
@Entity
public class Product {
@Id
@GeneratedValue(generator = "uuid2")
@GenericGenerator(name = "uuid2", strategy = "uuid2")
private String id;
private String productName;
// Constructors, getters, and setters
}
Challenges in Identifier Transition
As you transition from JPA to Hibernate, consider the following identifier-related challenges:
-
Generation Type Differences
JPA offers several strategies for ID generation, each with its characteristics. Hibernate introduces additional strategies that are more powerful. Adjusting your entity classes to embrace Hibernate's extended capabilities can be challenging. -
Legacy Databases
When working with legacy databases, your identifier strategy may not fit perfectly within the JPA specifications. This can lead to complications when attempting to integrate Hibernate. -
Custom Identifier Strategies
Developers utilizing custom identifier generation need to ensure compatibility with JPA, which may require extra effort when transitioning to Hibernate's more versatile approach. -
Entity Relationships
When relationships exist between entities, carefully managing identifiers to maintain referential integrity becomes crucial. Understanding how both frameworks handle cascading and related entity identifiers is essential.
Addressing Identifier Challenges in Your Transition
1. Adjusting Generation Strategies
Understanding the different generation strategies is vital. In JPA, you may find yourself using:
GenerationType.AUTO
: The default generation strategy.GenerationType.IDENTITY
: Obtains the identifier automatically from the database.GenerationType.SEQUENCE
: Uses a database sequence to generate IDs.
When moving to Hibernate, you can gain flexibility by exploring Hibernate's additional options.
Example Modification
If you originally defined an identifier with GenerationType.IDENTITY
, change it to a UUID to fit a globally unique identifier:
@Entity
public class Customer {
@Id
@GeneratedValue(generator = "UUID")
@GenericGenerator(name = "UUID", strategy = "org.hibernate.id.UUIDGenerator")
private String id;
// Other fields and methods
}
2. Creating Custom Identifiers
If you need specific identifier strategies, use @GenericGenerator
. Be mindful about the implications of your specific choice.
Custom Generator
The following example illustrates creating a custom generator for a composite key:
@Embeddable
public class OrderId implements Serializable {
private Long userId;
private Long orderId;
// hashCode, equals, getters, setters
}
@Entity
public class Order {
@EmbeddedId
private OrderId id;
// Other fields and methods
}
3. Handling Legacy Databases
When dealing with legacy databases, make sure you manually define the mapping in such a way that Hibernate knows how to interact.
Integrating Legacy Ids
You may need to bypass automatic generation for existing database records:
@Entity
public class LegacyEntity {
@Id
private Long id; // ID managed by the legacy system
// Other fields and methods
}
4. Managing Relationships
Relationships between entities in JPA require you to pay particular attention during the transition. When using @ManyToOne
or @OneToMany
, ensure you handle the cascading properly.
@Entity
public class Invoice {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
@ManyToOne(cascade = CascadeType.ALL)
private User user;
// Other fields and methods
}
Testing and Validation
It's crucial to thoroughly test the changes you make during the transition phase. Use unit tests and integration tests to ensure your identifiers function as expected.
Final Thoughts
Transitioning from JPA to Hibernate involves multiple intricacies, particularly in managing identifiers. Understanding the differences, leveraging Hibernate’s advanced capabilities, and adhering to best practices will ensure a more seamless transition.
While it may seem challenging at first, embracing Hibernate allows for customizable and powerful persistence options that can significantly enhance your application architecture.
For further reading and a deeper dive into JPA and Hibernate, refer to the JPA Specification and Hibernate Documentation.
Further Reading
- Effective Java by Joshua Bloch
- Spring Data JPA
- Understanding Hibernate on Baeldung
By following the insights laid out in this article, you will build a solid foundation to tackle the challenges of transitioning from JPA to Hibernate. Happy coding!