Decoding Distributed Tracing: Common Pitfalls to Avoid
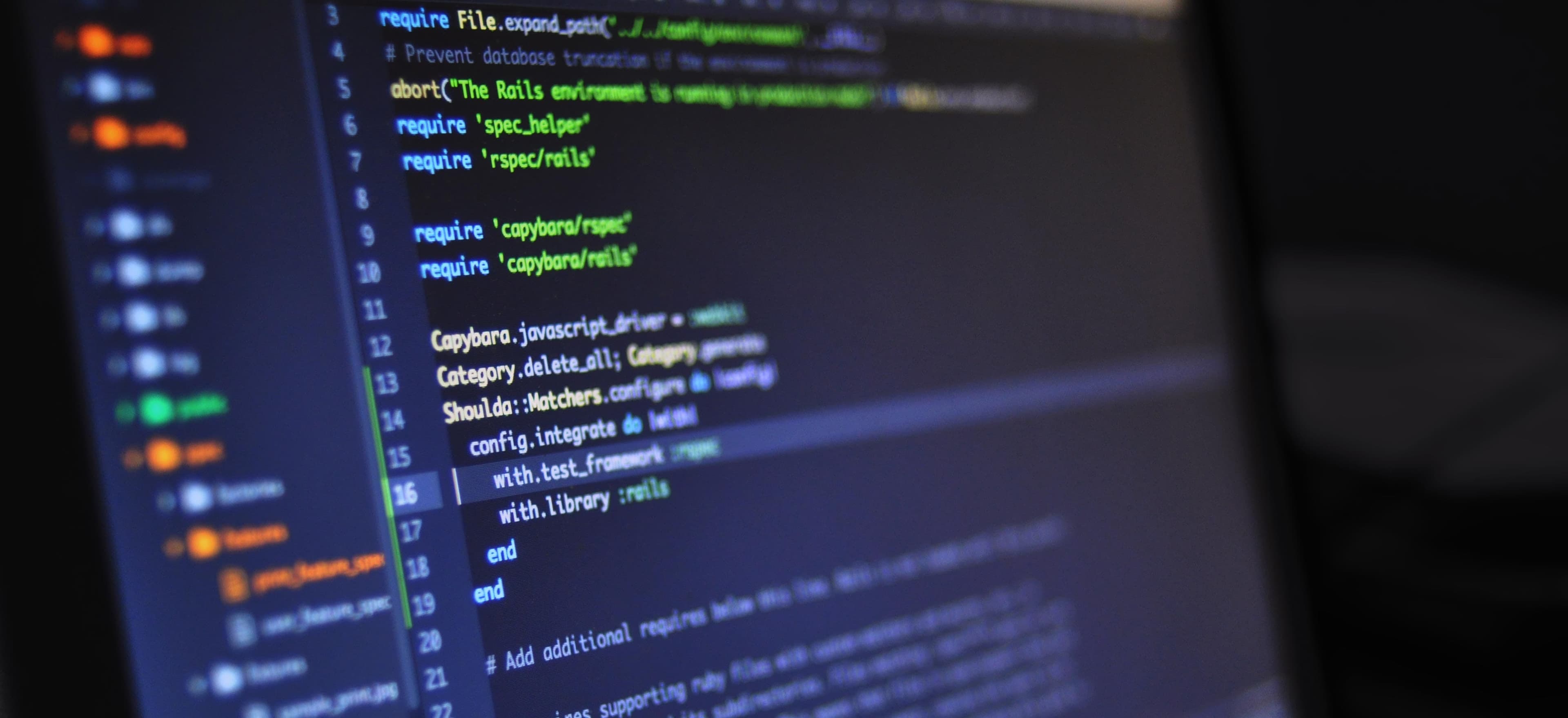
- Published on
Decoding Distributed Tracing: Common Pitfalls to Avoid
In modern software development, the microservices architecture has gained immense popularity due to its flexibility and scalability. However, this complexity can introduce significant challenges, especially in tracking and debugging requests as they flow through various services. This is where distributed tracing comes into play. This blog post aims to decode distributed tracing, shed light on its importance, and highlight common pitfalls to avoid.
What is Distributed Tracing?
Distributed tracing is a method that helps developers monitor applications built using a microservices architecture. It provides visibility into how requests propagate through multiple services, allowing teams to pinpoint where bottlenecks or failures occur. By capturing this data, organizations can improve performance, enhance user experiences, and ensure a smooth operational flow.
To get started, you might want to implement tools like Zipkin or Jaeger to set up distributed tracing in your Java application. These tools collect timing data and visualize the request flows across various services, making debugging a more straightforward process.
Why Use Distributed Tracing?
Distributed tracing offers multiple advantages:
- End-to-End Visibility: It allows developers to understand the complete picture of how data flows across services.
- Performance Optimization: By identifying latency issues, teams can optimize individual service performance.
- Fault Isolation: It helps quickly locate the service causing a failure, reducing downtime.
- Root Cause Analysis: Understanding the full request lifecycle aids in troubleshooting problems effectively.
With these benefits in mind, it's critical to implement distributed tracing thoughtfully. Below, we discuss common pitfalls developers often encounter, along with guidelines on how to avoid them.
Common Pitfalls to Avoid in Distributed Tracing
1. Inconsistency in Trace Context Propagation
One of the primary challenges in distributed tracing is ensuring that trace context is consistently propagated across microservices. Failing to pass trace IDs can result in gaps in visibility.
Solution: Always propagate the trace context through headers in your service-to-service communication. Below is a simple example using Spring Boot:
@RestController
public class SampleController {
@RequestMapping("/api/v1/resource")
public ResponseEntity<String> getResource(HttpServletRequest request) {
// Extract the trace ID from the request header
String traceId = request.getHeader("x-b3-traceid");
// Log the trace ID
log.info("Received request with trace ID: " + traceId);
// Your application logic here
return ResponseEntity.ok("Resource response");
}
}
This code snippet captures the trace ID from the incoming request headers and logs it. It serves a dual purpose: keeping track of trace IDs for monitoring and ensuring that IDs are correctly passed to downstream services.
2. Ignoring Sampling Rates
Another frequent issue is ignoring sampling rates, which can lead to performance degradation due to the sheer volume of data collected. If you trace every single request, you might produce excessive amounts of tracing data that overwhelm your logging system.
Solution: Implement a sampling strategy that makes sense for your application. For example:
public class TracingConfig {
private static final double SAMPLE_RATE = 0.1; // Sample 10% of requests
public static boolean shouldSample() {
return Math.random() < SAMPLE_RATE;
}
}
Here, the shouldSample
method determines whether a request should be traced based on a predefined sampling rate. This method helps you control the volume of tracing data generated, reducing system load while still gathering crucial insights.
3. Lack of Granular Instrumentation
Many developers fall into the trap of either over-instrumenting or under-instrumenting their applications. Over-instrumentation can clutter traces with too much information, while under-instrumentation can miss critical data required for debugging.
Solution: Balance is key. Focus on key performance indicators (KPIs) and bottlenecks in your architecture. Here’s an example of instrumenting a critical service method:
@NewSpan // Using Spring Sleuth for span creation
public void processOrder(Order order) {
log.info("Processing order with ID: " + order.getId());
// Simulate some processing logic
}
The @NewSpan
annotation creates a new span for the processOrder
method, providing crucial timing data without overloading the trace with unnecessary details.
4. Not Using Baggage for Contextual Information
Baggage items allow additional context to be passed across service calls. However, a common pitfall is neglecting to use them effectively, resulting in a loss of valuable contextual information during tracing.
Solution: Utilize baggage items judiciously. For example, when dealing with user identifiers in a request, include them in the baggage:
public void someServiceMethod(Tracer tracer, String userId) {
tracer.getBaggage("userID").set(userId);
// Proceed with any logic
}
In this case, the user ID is captured via baggage, allowing it to be associated with the trace. This can immensely help in understanding user-specific flows and issues.
5. Overlooking Tool Integration
Employing tracing without integrating it with your logging and monitoring tools often leads to a disjointed observability experience. When developers struggle to correlate traces with logs and metrics, they miss insights that could aid in debugging.
Solution: Always integrate tracing with logging and monitoring platforms. For instance, if you use ELK Stack or Prometheus, ensure your traces are linked with these tools. This integration creates a cohesive observability solution.
# Application properties for using ELK concurrent logging
logging.level.org.springframework.web=ERROR
spring.sleuth.sampler.probability=0.1
With this configuration, you can control logging levels and integrate sampling probability for a seamless flow of trace data into logging systems.
The Bottom Line
Distributed tracing is an essential practice for managing and debugging microservices architecture. With the right strategy, teams can avoid critical pitfalls while leveraging tracing to enhance performance and user experience.
- Always propagate trace context
- Implement thoughtful sampling strategies
- Focus on balanced instrumentation
- Utilize baggage for contextual information
- Ensure tool integration for a comprehensive observability suite
By recognizing and mitigating these common mistakes, organizations can build a resilient, observable system that not only tracks requests effectively but also fosters a deeper understanding of their services.
If you want more insights into distributed tracing or dive deeper into Java microservices, check out Spring Cloud Sleuth or read the documentation on OpenTelemetry for comprehensive guidance.
Happy coding!
Checkout our other articles