Mastering Mockito: Common Pitfalls in JUnit 5 Mocking
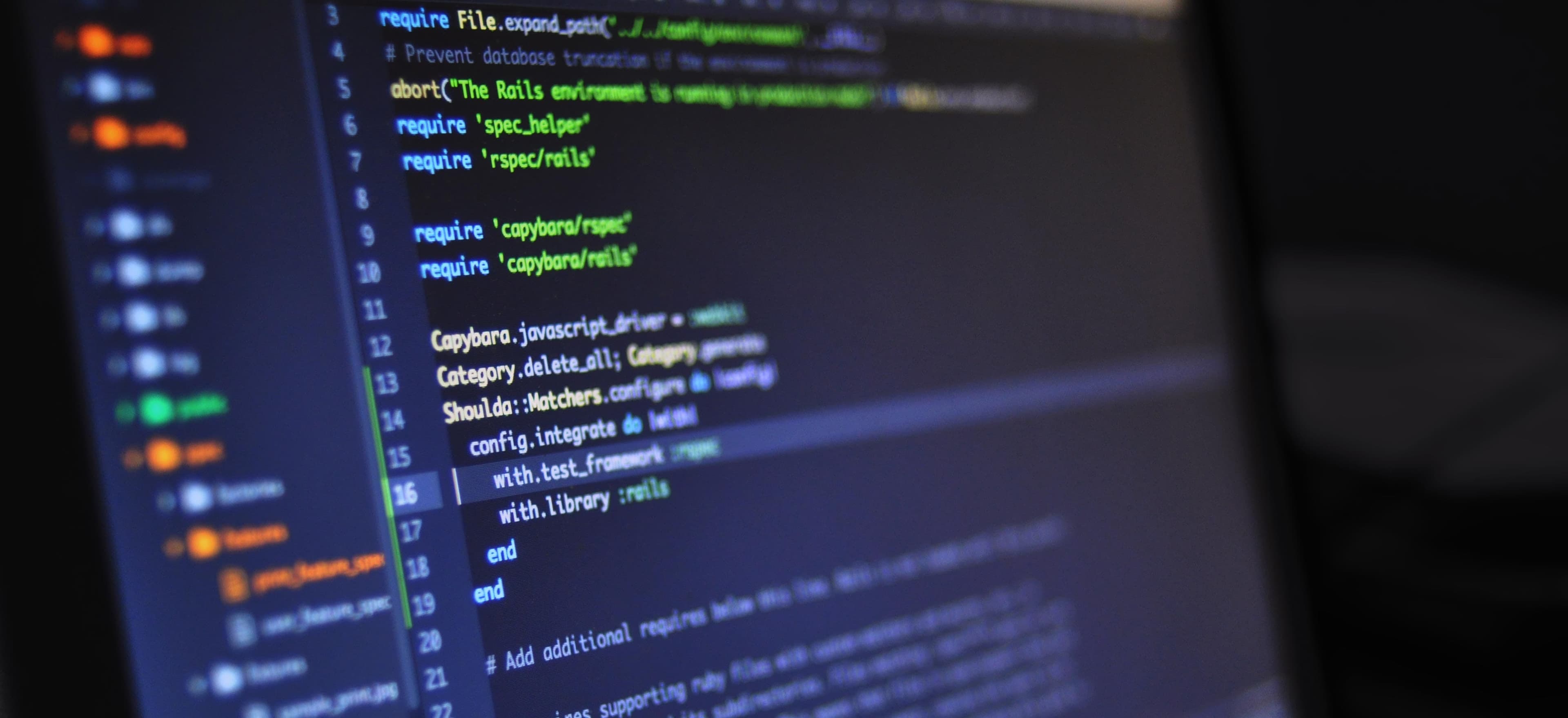
- Published on
Mastering Mockito: Common Pitfalls in JUnit 5 Mocking
In modern application development, unit testing has become an indispensable skill for ensuring software quality. Among the myriad of testing frameworks available, JUnit5 and Mockito have emerged as a powerful duo for Java developers. While both frameworks provide flexible capabilities, many developers encounter common pitfalls when using them together. This blog post explores these pitfalls, their implications, and how to navigate around them effectively.
Understanding JUnit 5 and Mockito
Before diving into pitfalls, let’s briefly highlight what JUnit 5 and Mockito are.
-
JUnit 5: This is the latest version of the widely used testing framework for Java. Its modular architecture and new features such as improved extension models and support for dynamic tests make it highly appealing.
-
Mockito: This is a powerful mocking framework that allows developers to create mock objects for unit tests efficiently. It helps isolate the code under test and simulates the behavior of dependent components.
For in-depth documentation on JUnit 5 and Mockito, be sure to check their official resources.
Common Pitfalls in Mockito with JUnit 5
Let’s delve into the common pitfalls developers may face when using Mockito with JUnit 5 and how to avoid them.
1. Not Using Annotations Properly
One common mistake is not leveraging Mockito annotations effectively. Mockito provides annotations like @Mock
, @InjectMocks
, and @ExtendWith(MockitoExtension.class)
to simplify mocking and dependency injection.
Example:
import org.junit.jupiter.api.extension.ExtendWith;
import org.mockito.InjectMocks;
import org.mockito.Mock;
import org.mockito.junit.jupiter.MockitoExtension;
@ExtendWith(MockitoExtension.class)
public class UserServiceTest {
@Mock
private UserRepository userRepository;
@InjectMocks
private UserService userService;
// Test methods go here
}
Why This Matters: Using annotations simplifies the setup of mocks and reduces boilerplate code, making your tests cleaner and more readable.
2. Misusing Mockito’s Behavior Verification
Another typical pitfall is misusing verification methods such as verify()
. It's critical to ensure that you verify the right method calls, yet developers often overlook the verification stage in their tests.
Example:
@Test
void shouldCallSaveMethod() {
User user = new User("John");
userService.saveUser(user);
verify(userRepository).save(user); // Make sure save was called
}
Why This Matters: Proper verification ensures that your code behaves as expected. By confirming method calls, you can catch unintended side effects early.
3. Ignoring Defaults of Mocks
By default, Mockito returns null
for unstubbed methods. This becomes problematic when a test fails silently due to unpredicted null returns.
Example:
@Test
void shouldReturnUserWhenExists() {
User user = new User("John");
when(userRepository.findById(1L)).thenReturn(user);
User found = userService.getUser(1L);
assertNotNull(found); // This test will pass
}
Why This Matters: Always define appropriate return values for your mocks. Use when().thenReturn()
to ensure predictable behavior, minimizing the chance of NullPointerExceptions
.
4. Over-Mocking
Another prevalent issue is over-mocking, where developers mock too many components, leading to fragile tests. It is essential to focus on the components relevant to the test case being written.
Example:
@Test
void shouldReturnUserDetails() {
when(userRepository.findById(1L)).thenReturn(new User("John"));
// Avoid mocking other unnecessary dependencies
}
Why This Matters: Over-mocking can make your tests hard to maintain and understand. Focus on mocking only what's necessary to keep your tests relevant and efficient.
5. Ignoring Argument Matchers
Using ArgumentMatchers
instead of hard-coded values offers more flexibility when verifying method calls or defining expectations. However, many developers neglect this feature.
Example:
@Test
void shouldHandleAnyUserId() {
User user = new User("John");
when(userRepository.findById(anyLong())).thenReturn(user);
User found = userService.getUser(2L); // Using anyLong() matcher
assertNotNull(found);
}
Why This Matters: Using matchers allows you to generalize and simplify your tests, reducing duplication and increasing maintainability.
6. Forgetting to Reset Mocks
In some cases, developers forget to reset mocks between tests, which can lead to state leakage and unexpected test failures.
Example:
@AfterEach
void resetMocks() {
Mockito.reset(userRepository);
}
Why This Matters: Resetting mocks ensures that each test starts with a clean slate, allowing for accurate verification of each test case.
7. Using Static Methods or Final Classes
Mockito cannot mock static methods or final classes directly without additional libraries (like PowerMock). This limitation can lead to confusion.
Example:
@Test
void shouldNotMockStaticMethod() {
// Static methods cannot be mocked by default
// Consider refactoring or using PowerMock if unavoidable
}
Why This Matters: This limitation encourages good design practices, such as dependency injection and programming to interfaces. It can guide developers to break down tightly coupled classes for better testability.
To Wrap Things Up
While Mockito and JUnit 5 provide exceptional capabilities for unit testing in Java, developers must navigate certain pitfalls to ensure effective testing. By understanding these common mistakes and taking the necessary precautions, you can significantly enhance the reliability of your tests.
From properly utilizing annotations to avoiding over-mocking, each point we've discussed is aimed at refining your testing practices. If you want to explore more advanced mocking strategies, consider delving into Mockito's cheatsheets or checking out Java's unit testing best practices.
Embrace these insights and master your unit testing skills with Mockito and JUnit 5—it’s worth the effort. Happy testing!
Checkout our other articles