Common Mistakes in Secure Software Development Practices
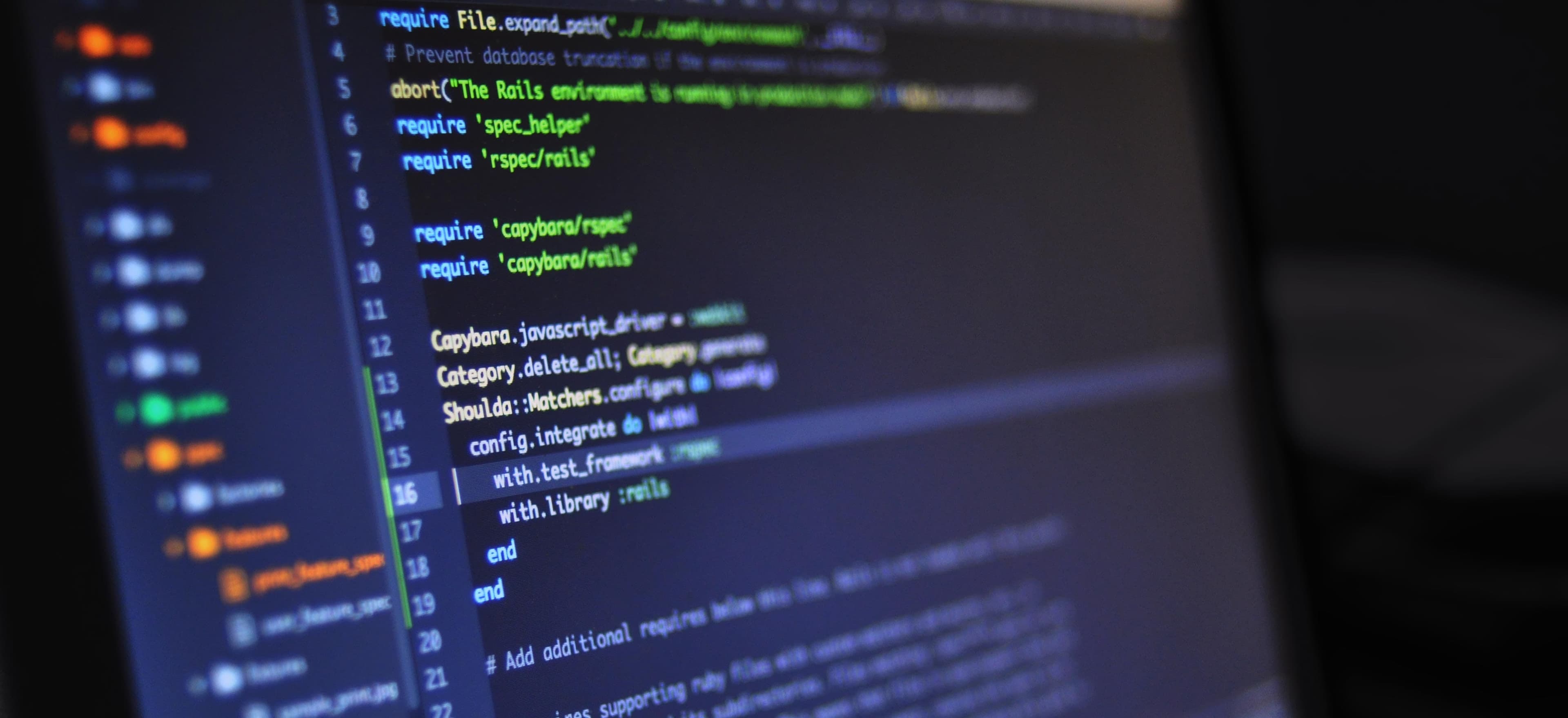
- Published on
Common Mistakes in Secure Software Development Practices
In today’s digital landscape, the importance of security in software development cannot be overstated. With an increasing number of data breaches and cyber-attacks, it has become essential to incorporate secure coding practices throughout the software development lifecycle (SDLC). However, many developers still make critical mistakes that can lead to vulnerabilities in their applications. In this blog post, we will explore common mistakes made in secure software development practices and how to avoid them.
1. Ignoring Threat Modeling
What is Threat Modeling?
Threat modeling is the process of identifying potential threats to the system and determining the security measures needed to mitigate them. It's vital for developers to consider security requirements right from the design phase.
Common Mistake
One of the most significant mistakes developers make is completely ignoring threat modeling. They jump directly into coding without assessing the risks associated with their application's architecture.
Why It Matters
Ignoring threat modeling increases the chances of security vulnerabilities later in the development process. By understanding potential threats, you can build security into the design and architecture of your application.
Example of Threat Modeling
Here is a simplified example of how you might conduct threat modeling in a web application:
1. Identify Assets (e.g., user data, financial records)
2. Create an Architecture Overview (e.g., data flow diagrams)
3. Identify Threats (e.g., data breaches, unauthorized access)
4. Determine Mitigations (e.g., access control measures)
5. Document Findings
You can learn more about threat modeling methodologies from resources provided in OWASP.
2. Poor Input Validation
Input Validation Basics
Input validation is the practice of ensuring that user input is sanitized and validated before processing it within the application. This is crucial for preventing attacks like SQL injection and Cross-Site Scripting (XSS).
Common Mistake
Developers often take a lax approach to input validation, relying on client-side checks without implementing robust server-side validation.
The Importance of Input Validation
Failing to adequately validate input leaves your application vulnerable to various attacks. For instance, an attacker can exploit poorly validated input to execute malicious code.
Example of Input Validation
Here’s a simple Java example showing how to secure user input:
import java.util.regex.Pattern;
public class InputValidator {
private static final Pattern EMAIL_PATTERN =
Pattern.compile("^[A-Za-z0-9+_.-]+@[A-Za-z0-9.-]+$");
public static boolean isValidEmail(String email) {
return email != null && EMAIL_PATTERN.matcher(email).matches();
}
}
// Usage
String userEmail = "test@example.com";
if (InputValidator.isValidEmail(userEmail)) {
System.out.println("Email is valid.");
} else {
System.out.println("Invalid email format.");
}
In this code, we utilize regular expressions to validate the email format. This helps prevent invalid data from entering the system, thereby increasing the safety of user interactions.
3. Hardcoding Secrets
Understanding Hardcoding
Hardcoding is the practice of embedding sensitive information, like passwords and API keys, directly into the source code.
Common Mistake
Many developers mistakenly hardcode sensitive credentials, believing that it won’t pose a significant risk in a local development environment.
Why It’s Dangerous
Hardcoding secrets exposes your application to substantial security risks, particularly when code is shared publicly in repositories. If anyone gains access to your source code, they might exploit it to gain unauthorized access.
Secure Secret Management Example
Here’s how you can manage secrets more securely using environment variables:
public class Config {
public static String getApiKey() {
return System.getenv("API_KEY");
}
}
// Usage
String apiKey = Config.getApiKey();
if (apiKey == null) {
System.out.println("API Key is not set.");
}
In this example, the sensitive API key is stored in an environment variable rather than hardcoded. This practice enhances security and allows for easy configuration changes.
4. Lack of Regular Security Testing
Importance of Regular Testing
Regular security testing should be a fundamental aspect of the development process. This allows developers to identify vulnerabilities before they become public knowledge.
Common Mistake
Unfortunately, many developers often neglect regular security testing, treating it as an optional step rather than a critical requirement.
The Risks of Skipping Testing
By omitting security tests, you increase the likelihood of vulnerabilities slipping through the cracks, resulting in unpatched security flaws post-deployment.
Code Example for a Simple Unit Test
Here's a basic JUnit test example for validating the security of input processing:
import static org.junit.jupiter.api.Assertions.*;
import org.junit.jupiter.api.Test;
public class InputValidatorTest {
@Test
public void testValidEmail() {
assertTrue(InputValidator.isValidEmail("test@example.com"));
}
@Test
public void testInvalidEmail() {
assertFalse(InputValidator.isValidEmail("test@.com"));
}
}
This unit test ensures that the email validation logic works correctly, thus affirming the input validation process’s integrity.
5. Not Keeping Dependencies Updated
Understanding Software Dependencies
Most applications rely on various third-party libraries and frameworks. Keeping these dependencies updated is crucial for maintaining security.
Common Mistake
A common oversight in secure software development is not regularly updating libraries or frameworks, leading to vulnerabilities present in outdated dependencies.
The Importance of Updates
Many updates address known vulnerabilities that could be exploited by attackers. Neglecting to update these libraries poses a significant security risk.
Automated Dependency Management
Using tools like Maven or Gradle can automate the process of dependency management and ensure that your libraries are up to date.
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
<version>${spring-boot.version}</version> <!-- Use property for versions -->
</dependency>
By managing your dependencies effectively, you will significantly minimize the risks associated with vulnerabilities in third-party software.
In Conclusion, Here is What Matters
Implementing secure software development practices is essential in building resilient applications that can withstand various cyber threats. By being aware of these common mistakes—including ignoring threat modeling, poor input validation, hardcoding secrets, lack of regular testing, and not keeping dependencies updated—you can take proactive measures to enhance your application's security.
To further help secure your applications, consider exploring resources such as the OWASP Secure Coding Practices which provide guidelines to follow in the development process.
Security cannot be an afterthought; it must be woven into the fabric of your software development practices from the beginning. Be vigilant, be proactive, and above all, secure your code!
Checkout our other articles