Common Pitfalls in Building a Spring MVC App with Gradle
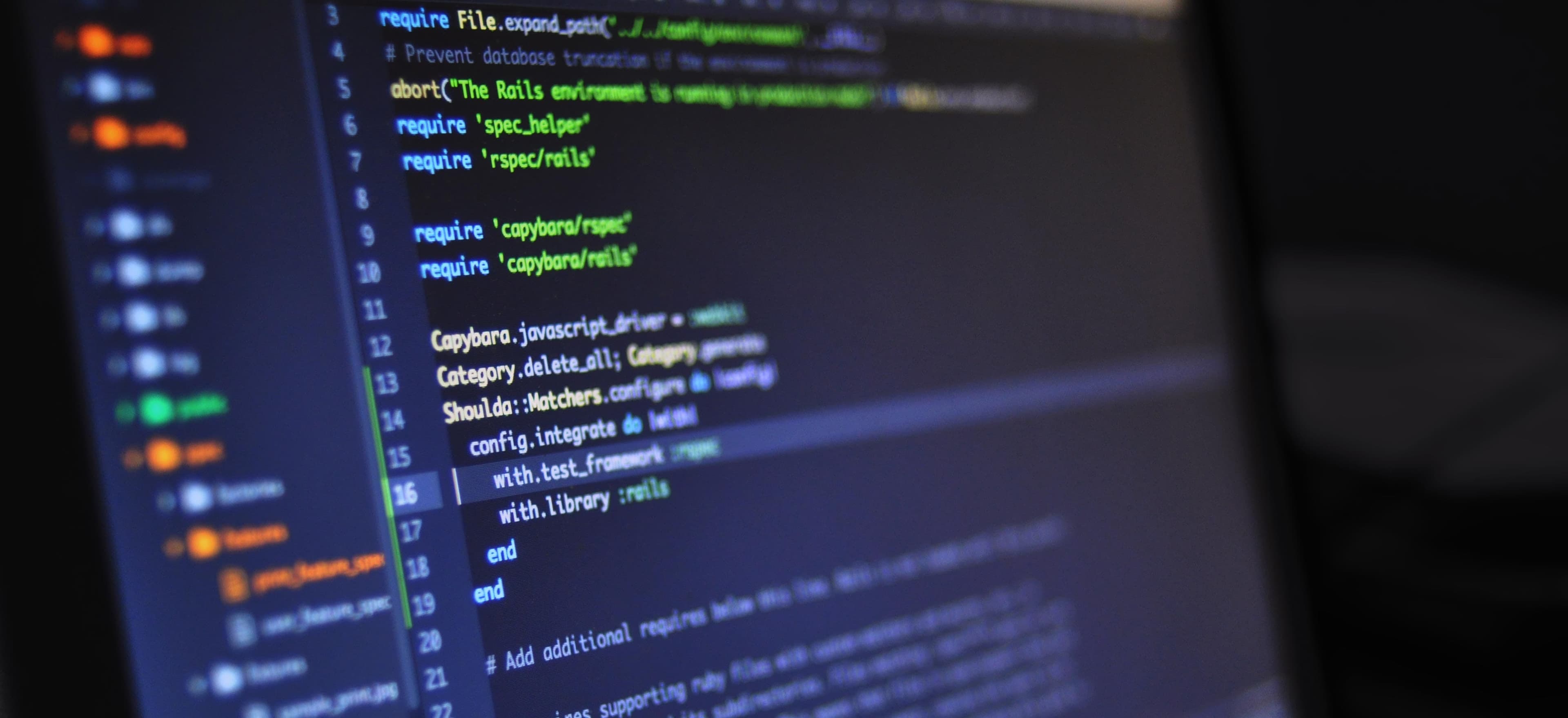
- Published on
Common Pitfalls in Building a Spring MVC App with Gradle
In the vibrant world of web development, Spring MVC stands out as a robust framework for building modern web applications. Coupled with Gradle, a powerful build automation tool, you can streamline project management significantly. However, many developers encounter pitfalls along the way that can complicate their development process. In this post, we will explore these common pitfalls, provide expert advice, and offer succinct code examples to help you develop a Spring MVC app smoothly and efficiently.
What is Spring MVC?
Spring MVC is part of the larger Spring framework, catering specifically to the design of web applications. Its features include:
- Model-View-Controller architecture: This organizes an application into three main components, enhancing separation of concerns.
- Flexible configuration: Spring MVC provides a wide range of options, such as XML or Java-based configuration.
- RESTful support: Easily build RESTful web services with its capabilities for handling HTTP requests.
By using Gradle, you can manage dependencies, streamline build processes, and automate testing.
Common Pitfalls in Building Spring MVC Apps with Gradle
Let's break down the pitfalls often encountered when building Spring MVC applications with Gradle.
1. Misconfigured Gradle Build File
The Gradle build file (build.gradle
) defines how your project is built, including dependencies and plugins. A poorly configured build file is a common issue.
Solution: Ensure that you have the correct Spring dependencies and versions specified. Here’s a basic template for a Spring MVC application:
plugins {
id 'java'
id 'org.springframework.boot' version '3.0.0'
id 'io.spring.dependency-management' version '1.0.11.RELEASE'
}
group = 'com.example'
version = '0.0.1-SNAPSHOT'
sourceCompatibility = '17'
repositories {
mavenCentral()
}
dependencies {
implementation 'org.springframework.boot:spring-boot-starter-web'
implementation 'org.springframework.boot:spring-boot-starter-thymeleaf'
testImplementation 'org.springframework.boot:spring-boot-starter-test'
}
The implementation
keyword tells Gradle to include these libraries in the build classpath.
2. Ignoring Gradle Caching
Gradle utilizes caching to optimize build performance. Ignoring caching can lead to unnecessarily slow builds.
Solution: Use the command ./gradlew build --refresh-dependencies
sparingly. Instead, trust Gradle's cache unless there’s a specific case where you know dependencies have changed.
3. Not Using Application Properties Effectively
Spring Boot allows configuration through application.properties
or application.yml
. Incorrect configurations can lead to runtime errors.
Solution: Ensure that your properties are set correctly in src/main/resources/application.properties
. Here’s an example:
server.port=8080
spring.mvc.view.prefix=/WEB-INF/views/
spring.mvc.view.suffix=.jsp
This configuration dictates where your views are located.
4. Misusing Dependency Management
Inconsistent versioning among dependencies can lead to “jar hell,” which causes conflicts and broken functionality.
Solution: Utilize the Spring Dependency Management Plugin effectively. Here’s how you can declare versions:
dependencyManagement {
imports {
mavenBom "org.springframework.boot:spring-boot-dependencies:3.0.0"
}
}
This practice is especially effective when you are managing multiple dependencies. It ensures all dependencies are aligned with the Spring Boot version.
5. Overlooking REST Controller Annotations
Spring MVC has multiple controller annotations (like @Controller
, @RestController
) that can confuse newcomers. Misusing them can lead to undesired behaviors.
Solution: Use @RestController
for RESTful services. For example:
@RestController
@RequestMapping("/api")
public class SampleController {
@GetMapping("/greeting")
public String greeting() {
return "Hello, World!";
}
}
Using @RestController
automatically applies @ResponseBody
, meaning the return value is written directly to the HTTP response body.
6. Ignoring Testing Strategies
Testing is an integral part of application development, yet many developers neglect or poorly implement it.
Solution: Leverage Spring Boot’s testing features, which provide a comprehensive testing suite. Use annotations like @SpringBootTest
for your test cases:
@SpringBootTest
public class SampleControllerTests {
@Autowired
private MockMvc mockMvc;
@Test
public void greetingShouldReturnDefaultMessage() throws Exception {
mockMvc.perform(get("/api/greeting"))
.andExpect(status().isOk())
.andExpect(content().string("Hello, World!"));
}
}
Regularly run your tests with ./gradlew test
to ensure your application remains stable throughout development.
7. Underrating Documentation
A documented API is crucial in clarifying usage and functionality. Many developers underestimate this, resulting in poor usability.
Solution: Use Swagger or Spring REST Docs to generate documentation automatically.
Here is an example of adding Swagger dependencies in your build.gradle
:
implementation 'io.springfox:springfox-boot-starter:3.0.0'
8. Ignoring Default Error Handling
Spring MVC provides default error handling, but neglecting to customize it can lead to a poor user experience.
Solution: Implement custom error pages. For example, you can create error.html
under src/main/resources/templates
:
<!DOCTYPE html>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<title>Error</title>
</head>
<body>
<h1>An error occurred</h1>
<p th:text="${message}"></p>
</body>
</html>
9. Not Leveraging Profiles
Spring profiles are a powerful feature for managing different application configurations.
Solution: Utilize profiles to manage environments (development, testing, production). In your application.properties
, you can specify different configurations:
# application-dev.properties
spring.datasource.url=jdbc:h2:mem:testdb
Activate the profile during runs with -Dspring.profiles.active=dev
.
10. Failing to Handle Exceptions Properly
Handling exceptions gracefully enhances user experience. A common mistake is to ignore exception handling, leading to vague error messages.
Solution: Define a global exception handler:
@ControllerAdvice
public class GlobalExceptionHandler {
@ExceptionHandler(Exception.class)
public ResponseEntity<Object> handleGlobalException(Exception e) {
return new ResponseEntity<>(e.getMessage(), HttpStatus.INTERNAL_SERVER_ERROR);
}
}
This handles exceptions and provides a custom response, improving the user experience.
A Final Look
Building a Spring MVC application with Gradle can be incredibly rewarding but comes with its challenges. By understanding and avoiding these common pitfalls, you can streamline your development process, enhance application performance, and provide a better experience for your users.
For further learning, refer to the Spring MVC Documentation and the Gradle User Manual.
With the right knowledge and resources, building robust Spring MVC apps can be an exciting journey. Happy coding!
Checkout our other articles