Scaling Challenges in Apache Pulsar: Overcoming Message Load
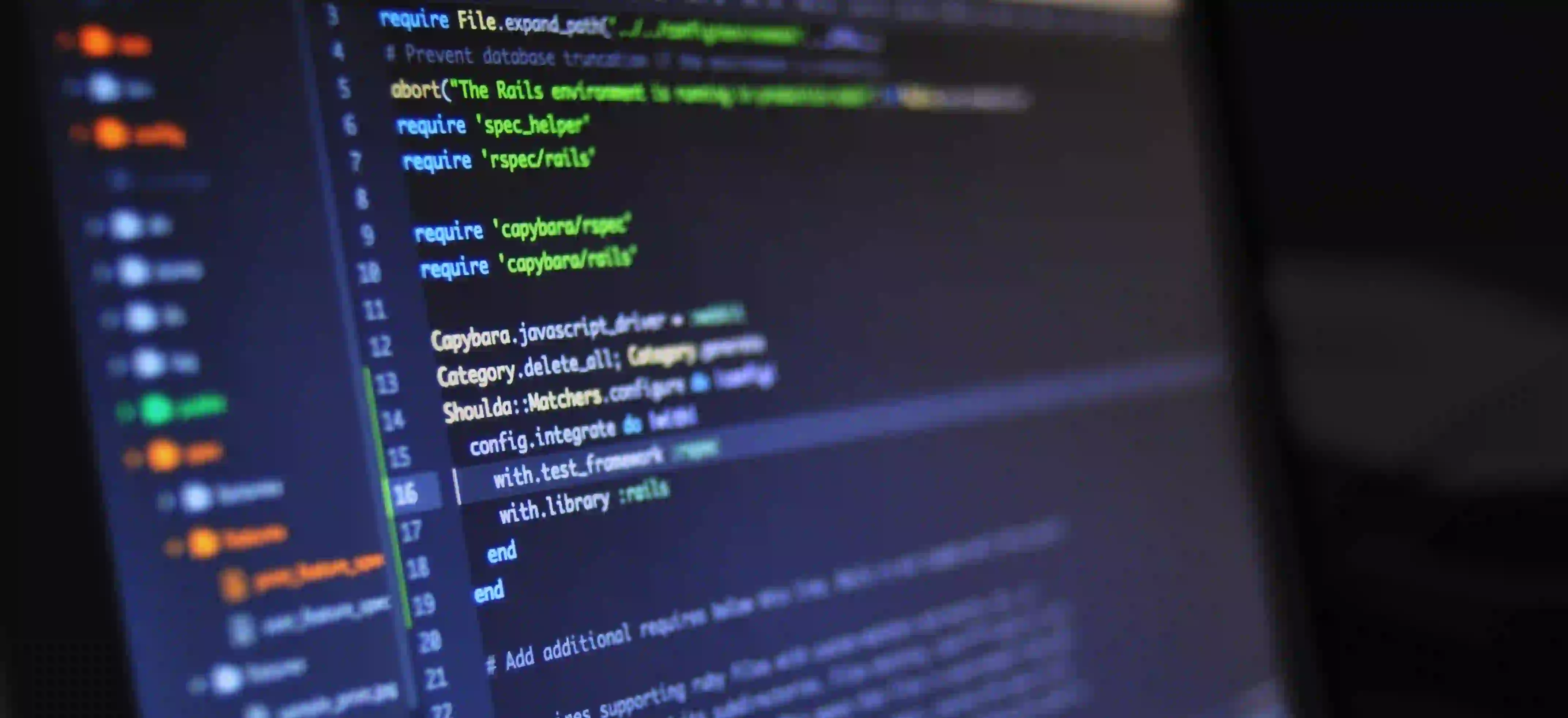
Scaling Challenges in Apache Pulsar: Overcoming Message Load
Apache Pulsar is a robust, distributed messaging system that offers great scalability and reliability for real-time data streaming. However, as with any technology, managing a growing message load can present its own set of challenges. This blog post will discuss various scaling challenges associated with Apache Pulsar and how to effectively overcome them. We will also explore best practices to optimize performance.
Understanding Apache Pulsar
Apache Pulsar is designed to handle message streaming with features like multi-tenancy, geo-replication, and low latency. However, as your application grows, you may start to experience bottlenecks that could potentially hinder performance. It becomes essential to understand these challenges better to maintain efficiency in message consumption and production.
What are the Common Scaling Challenges?
1. Message Throughput Throughput refers to the number of messages that your system can process within a given time frame. A sudden spike in message volume can overwhelm your architecture.
2. Consumer Lag As the number of messages increases, consumers may struggle to keep up with the message production rate, leading to increased consumer lag and delayed processing.
3. Storage Concerns Pulsar uses Apache BookKeeper as its storage layer, and while it is suitable for high-throughput workloads, it can struggle under heavy load if not configured properly.
4. Network Bottlenecks High throughput can put a strain on network resources, leading to performance degradation.
Strategies for Addressing Message Load
To effectively manage high message loads in Pulsar, consider these strategies:
1. Fine-Tuning Producer Configuration
When configuring producers, it's essential to focus on batching and acknowledgment settings. Acknowledgment types determine when a producer knows their message has been received. By enabling batch messages, you can significantly improve the throughput.
Example Code Snippet
import org.apache.pulsar.client.api.*;
public class PulsarProducer {
public static void main(String[] args) throws PulsarClientException {
PulsarClient client = PulsarClient.builder()
.serviceUrl("pulsar://localhost:6650")
.build();
Producer<String> producer = client.newProducer(Schema.STRING)
.topic("my-topic")
.enableBatching(true)
.batchingMaxMessages(100)
.batchingMaxPublishDelay(1, TimeUnit.MILLISECONDS)
.create();
for (int i = 0; i < 1000; i++) {
producer.send("Message " + i);
}
producer.close();
client.close();
}
}
What is happening in this code?
- enableBatching: Enables message batching to improve throughput.
- batchingMaxMessages: Limits the number of messages in a batch.
- batchingMaxPublishDelay: Reduces the wait time for sending smaller batches.
These settings will help you strike a balance between latency and throughput.
2. Scaling Consumers
To reduce consumer lag, consider increasing the number of consumers for a single subscription. Apache Pulsar's shared subscription model enables multiple consumers to read messages from the same topic concurrently.
Example Code Snippet
import org.apache.pulsar.client.api.*;
public class PulsarConsumer {
public static void main(String[] args) throws PulsarClientException {
PulsarClient client = PulsarClient.builder()
.serviceUrl("pulsar://localhost:6650")
.build();
Consumer<String> consumer = client.newConsumer(Schema.STRING)
.topic("my-topic")
.subscriptionName("my-subscription")
.subscriptionType(SubscriptionType.Shared)
.subscribe();
while (true) {
Message<String> msg = consumer.receive();
System.out.printf("Message received: %s\n", msg.getValue());
// Acknowledge the message
consumer.acknowledge(msg);
}
// Normally you would close client and consumer, but kept here for simplicity
}
}
Why use shared subscriptions?
- Improves parallelism: Multiple consumers can process messages simultaneously.
- Reduces waiting time: Ensures quicker message consumption and lowers potential lag.
3. Optimizing BookKeeper Configuration
Apache Pulsar relies on BookKeeper for storing messages. Optimizing its configuration can significantly enhance performance.
- Ledger Size: Keeping your ledger size in check can mitigate performance issues. Set the ledger size based on your message volume.
- Bookie Nodes: Consider deploying additional bookie nodes to more evenly distribute load.
4. Implementing Geo-Replication Wisely
Geo-replication allows you to replicate messages across different clusters. While it is a powerful feature, improperly configured geo-replication can lead to increased latency and impacts throughput.
- Limit Geo-Replication: Only replicate messages that need to be shared across different regions.
- Use Different Clusters: For geographically diverse clients, use nearby clusters to minimize latency.
5. Network Optimization
Network throughput can easily become a bottleneck. Here are a few tips to enhance performance:
- Network Segmentation: Use virtual LANs and traffic management solutions to ensure dedicated paths for messaging traffic.
- Monitor Traffic: Utilize tools to monitor network usage and performance. This information can guide you in making adjustments.
The Last Word
Scaling challenges in Apache Pulsar can be daunting, especially in the face of increasing message loads. However, by employing suitable strategies, such as fine-tuning producer and consumer configurations, optimizing BookKeeper settings, and correctly implementing geo-replication, you can successfully overcome these hurdles. Additionally, never underestimate the impact of network optimization in achieving a high-performing environment.
For more comprehensive insights into Apache Pulsar and its features, you might want to check the official documentation.
Further Reading:
- Message Queuing in Apache Pulsar
- Pulsar vs. Kafka: A Comparison
- Best Practices for Using Apache Pulsar
By staying informed and implementing the right strategies, you can leverage Apache Pulsar's power and scalability to meet your message processing needs.