Troubleshooting Common Issues with Spring Boot Native Builds
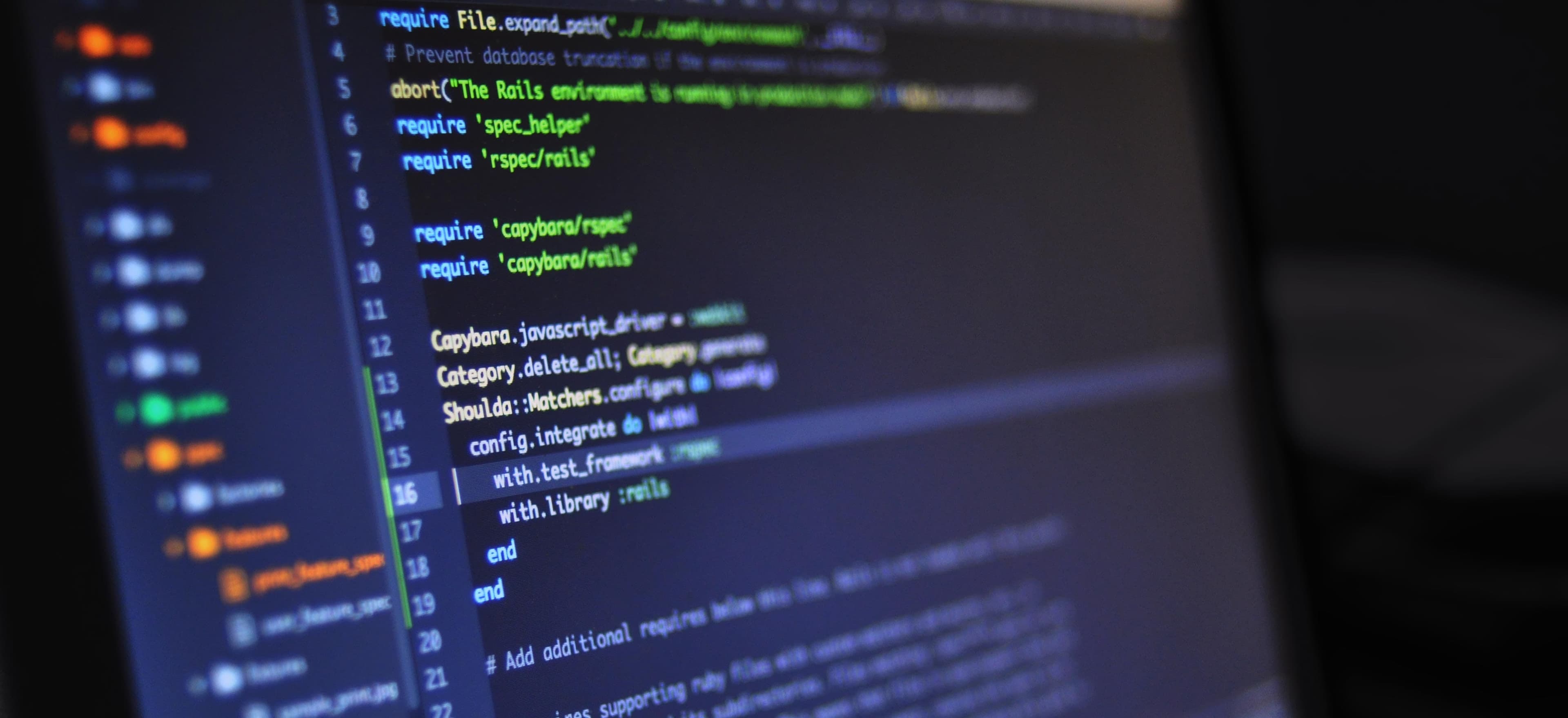
- Published on
Troubleshooting Common Issues with Spring Boot Native Builds
Spring Boot has embraced the modern demands of application deployment by introducing native compilation via GraalVM. However, transitioning to native builds can lead to a host of challenges. In this blog post, we will explore common issues developers face when working with Spring Boot native builds and how to solve them effectively.
The Roadmap to Spring Boot Native Builds
Spring Boot native builds compile Java applications to native executables. This approach significantly improves startup times and reduces runtime memory consumption. However, it comes with its own set of complexities.
Benefits of Native Builds
- Faster Startup Times: Native executables start up almost instantaneously compared to traditional JVM-based applications.
- Reduced Memory Footprint: They consume less memory during runtime, making them suitable for microservices and cloud environments.
- No JVM Dependency: The application can run independently of Java installations, simplifying deployment.
Despite these benefits, developers often encounter various issues when they start working with native builds. Let's explore some of the most common ones.
Common Issues and Troubleshooting Tips
1. Dependency Issues
One of the most frequent issues is related to dependencies that may not be compatible with native compilation. Libraries that utilize reflection or dynamic proxies can cause problems.
Solution
Always make sure that your dependencies are native-aware. You can check libraries such as Spring Native for their compatibility. Use the following ways to manage dependencies:
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-function-web</artifactId>
<version>3.0.0</version>
</dependency>
Why this code? This import declares a Spring Cloud library that is known to support native builds. Always refer to the library documentation for versions that are compatible with GraalVM.
2. Missing Resources
Certain resources that exist in your classpath may not be included in the native image. This is particularly true for things like configuration files, static content, or resources loaded via classpath.
Solution
You can include resources in your native image by specifying them in the native-image.properties
file. Here’s how you can do it:
args = --initialize-at-build-time=your.packagename.YourClass
Why this code? This argument tells GraalVM to initialize the specified class at build time, ensuring that necessary resources are included.
3. Reflection Issues
Reflection is a common approach in Java, but it can lead to complications during native image generation since the metadata required for reflection isn't automatically available.
Solution
You can provide Graal with reflection metadata by creating a reflect-config.json
. Here's an example entry:
[
{
"name": "your.packagename.YourClass",
"allDeclaredConstructors": true,
"allDeclaredMethods": true,
"allDeclaredFields": true
}
]
Why this code? This ensures that all constructors, methods, and fields of YourClass
are accessible via reflection during execution of the native image.
4. Build Configuration Issues
Sometimes the native build fails due to incorrect configuration in the Maven or Gradle build files.
Solution
Ensure you have included the necessary plugins. For Maven, it's something like:
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
<version>${spring-boot.version}</version>
<configuration>
<image>
<name>${project.groupId}/${project.artifactId}</name>
</image>
</configuration>
</plugin>
Why this code? This Maven configuration utilizes the Spring Boot Maven plugin to create a Docker image of your application.
For Gradle, make sure you include:
nativeImage {
binaries {
main { }
}
}
Why this code? This Gradle snippet specifies the main binary to include in the native build, simplifying the configuration process.
5. Configuration Properties Not Loading
Another common error is when the application fails to load properties from application.properties
or application.yml
files.
Solution
You need to specify these file locations properly for native images. Use the command line arguments while building your native image:
-Dspring.native.additional-build-args=--initialize-at-build-time=org.springframework.core.env.ConfigurableEnvironment
Why this code? This argument helps ensure that the Spring environment is initialized correctly at build time, which might resolve loading issues.
6. Native Image Size
Sometimes, developers are surprised by the large file size of their native executables. Generally, native images are larger than expected, and this can deter some from adopting the technology.
Solution
GraalVM offers options to reduce the image size. One approach is using the following argument:
--no-fallback
Why this code? This tells GraalVM not to create a fallback image, which can significantly reduce the size.
Final Thoughts
Spring Boot native builds offer extraordinary benefits, but they come with complexities and challenges. By understanding these common pitfalls and implementing the provided solutions, developers can effectively harness the power of native compilation in their applications.
If you're interested in diving deeper into this subject, consider checking the official Spring Native documentation and the GraalVM website for further insights.
By troubleshooting these common issues, you can achieve faster, more efficient applications that make the most of modern architecture. Happy coding!
Checkout our other articles