Maximize Kafka Performance with Quarkus: Common Pitfalls
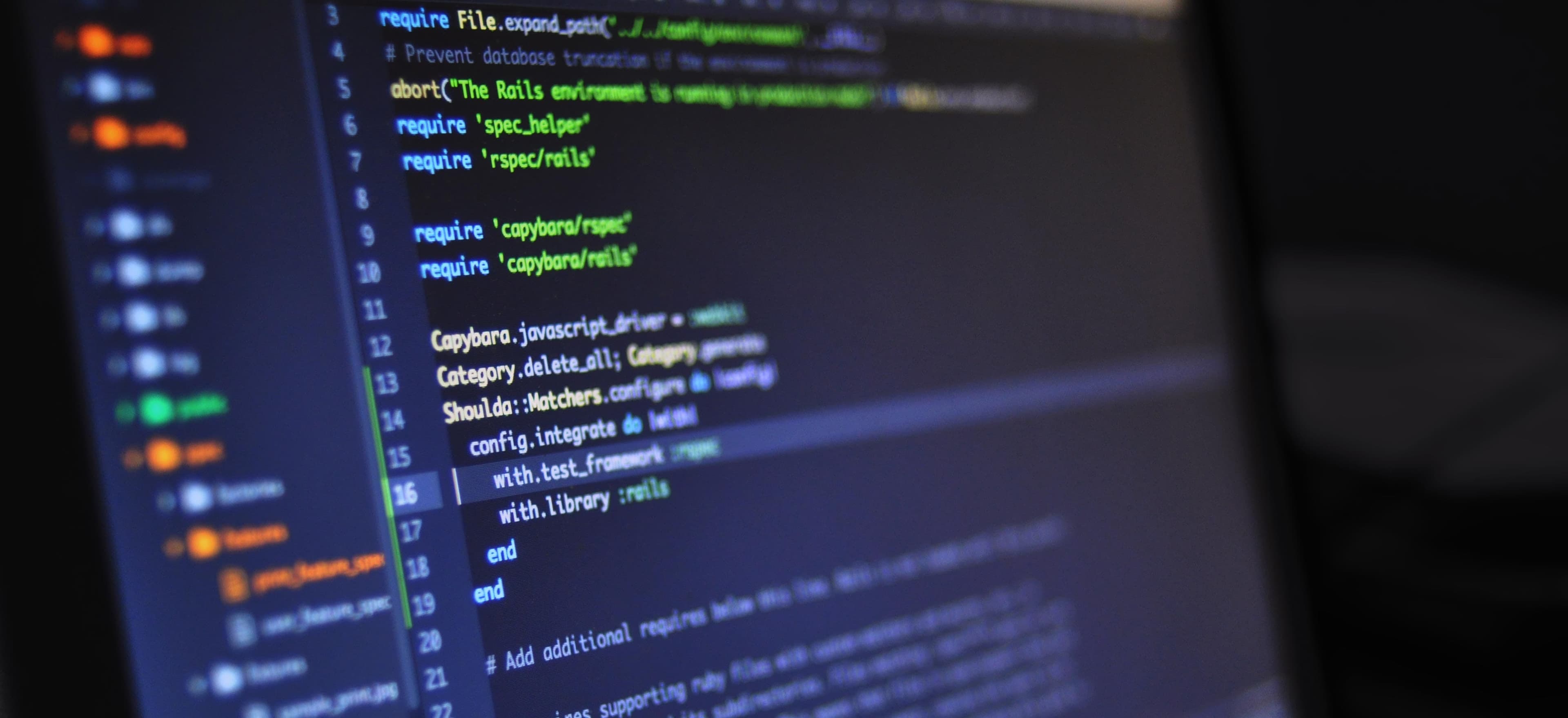
- Published on
Maximize Kafka Performance with Quarkus: Common Pitfalls
Apache Kafka is a prominent distributed event streaming platform, widely used for building real-time data pipelines and streaming applications. When combined with Quarkus, a Kubernetes-native Java framework tailored for cloud environments, developers gain a powerful toolset that improves both performance and developer experience. However, to get the most out of Kafka in Quarkus, it is crucial to avoid common pitfalls that can hinder performance.
In this post, we will explore some common performance pitfalls developers encounter while using Kafka with Quarkus and how to mitigate them.
1. Misconfigured Producer Properties
Kafka Producer configurations significantly influence performance. Here are some vital properties you should focus on:
a. Batch Size
Batch size determines how many records are sent to Kafka in a single request. A smaller batch size can result in too many requests, creating overhead. Conversely, a large batch size can increase latency.
Example Configuration:
kafka.producer.batch.size=16384 # 16KB
Why it Matters:
Increasing the batch size reduces the number of requests sent, implementing better resource utilization and lowering the acknowledgment time.
b. Acknowledgment Settings
The acks
setting in Kafka tells the producer the level of acknowledgment required from the broker. If set to all
, it guarantees all in-sync replicas acknowledge receipt before the producer continues, which can be slow for high-throughput applications.
Example Configuration:
kafka.producer.acks=1 # Only the leader node must acknowledge
Why it Matters:
Using an acknowledgment setting of 1
or 0
can dramatically increase throughput at the cost of some reliability.
2. Not Utilizing Compression
Sending large messages over the network can strain performance. Utilizing message compression is an effective way to reduce network usage.
Compression Types
Kafka supports multiple compression codecs, including Snappy, GZIP, and LZ4.
Example Configuration:
kafka.producer.compression.type=snappy
Why it Matters:
Compression can significantly reduce the size of the messages, leading to lower latency and higher throughput. It's crucial to test different codecs against your workload to choose the most suitable one.
3. Ignoring Consumer Lag Monitoring
Consumer lag is a measure of how far behind a consumer is compared to the producer. High consumer lag indicates that the consumer is unable to keep up with the incoming message rate.
Monitoring Lag
By using tools like Kafka Manager or running specific metrics in Prometheus, you can visualize and monitor consumer lag.
Why it Matters:
Monitoring consumer lag ensures your application catches up to the incoming data stream, preventing potential data loss and ensuring real-time processing.
4. Inadequate Scaling Options
One of the strengths of Kafka is its ability to handle scaling naturally. However, many developers fail to adjust topic partitions based on the workload.
Partition Configuration
More partitions can lead to better throughput but can also increase complexity. Consider balancing partition count with consumer groups.
Example Command to Increase Partitions:
kafka-topics --alter --topic your-topic --partitions 10 --bootstrap-server localhost:9092
Why it Matters:
Using an appropriate number of partitions allows you to balance load and enables parallel processing, maximizing performance.
5. Serialization Strategies
The serialization strategy applied when sending messages to Kafka affects both performance and data size.
Use Efficient Serializers
Instead of Java's default serialization, consider using lightweight alternatives like Avro or Protocol Buffers.
Example Avro Serialization:
// Create a Generic record
GenericRecord user = new GenericData.Record(userSchema);
user.put("name", "John Doe");
user.put("age", 30);
// Sending to Kafka
producer.send(new ProducerRecord<>("users", user.get("name").toString(), user));
Why it Matters:
Utilizing efficient serializers not only speeds up the serialization process but also reduces the amount of data transmitted over the network.
6. Network Tuning and Configuration
Network-related issues can severely impact Kafka performance, and tuning parameters related to communication between producers, consumers, and brokers is crucial.
Network Configurations
Look into settings such as socket buffer sizes and the number of network threads.
Example Configuration:
kafka.network.send.buffer.bytes=1048576 # 1 MB
Why it Matters:
A correctly configured network buffer size can improve the speed and reliability of message transmission.
7. Ignoring Quarkus Capabilities
Quarkus is designed to enhance resource efficiency and increase performance, but developers sometimes overlook its unique capabilities.
Using GraalVM
Quarkus allows you to compile to native executable using GraalVM, which drastically reduces startup time and decreases memory consumption.
Example Command:
mvn package -Pnative
Why it Matters:
By leveraging GraalVM, you can create highly optimized applications that consume fewer resources, leading to more cost-effective deployments.
Final Thoughts
Maximizing Kafka performance when using Quarkus involves numerous optimizations spanning configuration, monitoring, and resource management. By avoiding common pitfalls, such as misconfigured producer properties, overlooking compression, and neglecting consumer lag monitoring, you can build robust, high-performing applications capable of handling real-time data streams.
For further information and best practices, consider exploring the official Quarkus documentation or the Kafka documentation to enhance your understanding of the toolsets available to you.
Understanding the 'why' behind each choice enables developers to make informed decisions that lead to more efficient, scalable systems, whether in development or production environments. Your pursuit of performance should blend knowledge, experimentation, and ongoing monitoring for cumulative improvement and success in your applications.
By investing the time in optimizing these elements, you position your applications for greater reliability and efficiency in today’s fast-paced digital landscape.
Checkout our other articles