Understanding Immutability: Avoiding Common Pitfalls
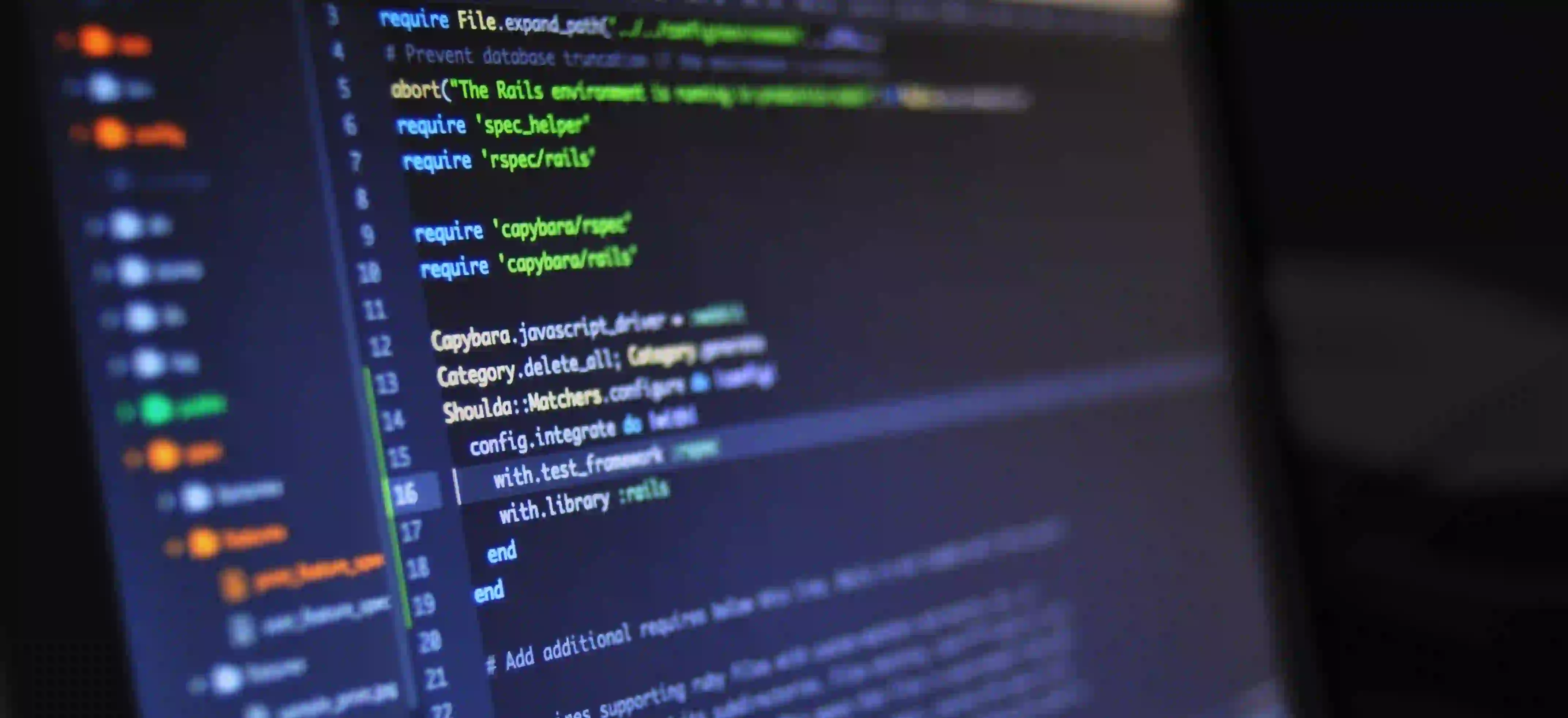
Understanding Immutability: Avoiding Common Pitfalls
Immutability is a fundamental concept in programming, particularly in languages like Java, where clear management of state can greatly enhance code quality and maintainability. It refers to the ability of an object to remain unchanged after its creation. In this blog post, we will explore immutability in Java, discuss its significance, delve into common pitfalls, and provide practical examples to illuminate best practices.
What is Immutability?
At its core, immutability signifies that once an object is created, its state or content cannot be altered. This can be beneficial for several reasons:
-
Thread-Safety: Immutable objects can be shared between threads without synchronization, eliminating the risk of concurrent modifications.
-
Ease of Understanding: As immutable objects remain constant, they are easier to reason about and understand, reducing the likelihood of side effects.
-
Caching and Optimization: Immutability can make caching strategies more efficient since the same object can be reused without fear of changes.
Example of Immutable Class
Let's start by creating a simple immutable class to illustrate the concept:
public final class Point {
private final int x;
private final int y;
public Point(int x, int y) {
this.x = x;
this.y = y;
}
public int getX() {
return x;
}
public int getY() {
return y;
}
}
Why Immutable?
The Point
class above cannot be extended (due to final
), ensuring that its behavior remains consistent. The member variables x
and y
are declared final
, meaning they must be initialized during object construction and cannot be altered afterward.
The Importance of Immutability in Java
In Java, immutability isn’t merely an option; it is often a necessity in crafting reliable applications. Below are reasons why immutability should be a core consideration:
1. Data Integrity and Consistency
When you construct a program based on immutable objects, you inherently reduce the complexity associated with data integrity. Each instance of an immutable object represents one, complete state.
2. Ease of Use and Maintenance
Immutability simplifies maintenance, as developers can safely reuse instances, knowing they are working with a known and fixed value.
3. Enhanced Performance in Functional Programming Style
Java supports a functional programming style through features such as lambdas and streams. Immutable collections (e.g., List
, Set
) can improve performance due to their straightforward usage in functional paradigms.
Common Pitfalls around Immutability
While immutability provides many benefits, it is not without its challenges. Here are some common pitfalls programmers encounter with immutable objects and how to avoid them.
Pitfall 1: Mutable Fields in Immutable Classes
One of the most frequent mistakes is including fields that can change, such as mutable objects or collections, which undermines the entire intention of immutability.
import java.util.ArrayList;
import java.util.List;
public final class Student {
private final String name;
private final List<String> grades;
public Student(String name, List<String> grades) {
this.name = name;
this.grades = new ArrayList<>(grades); // Defensive copy
}
public String getName() {
return name;
}
public List<String> getGrades() {
return new ArrayList<>(grades); // Returns a copy
}
}
Why Defensive Copies?
In this example, a defensive copy of the mutable List
ensures that the internal state of the Student
object cannot be modified externally. Whenever grades
is retrieved, a new copy is returned, thus preserving immutability.
Pitfall 2: Incomplete Implementation of Immutable Classes
An immutable class might inadvertently become mutable if not properly controlled. For instance, failing to make fields final
can lead to unintended consequences.
public final class Circle {
private final int radius;
public Circle(int radius) {
this.radius = radius; // Immutability is preserved
}
public Circle resize(int newRadius) {
return new Circle(newRadius); // Create a new Circle
}
public int getRadius() {
return radius;
}
}
Why Return New Instances?
In this Circle
class, instead of allowing changes to radius
, we create a new Circle
instance via the resize
method. This promotes immutability and clarity in managing states.
Pitfall 3: Overuse of Immutability
While immutability has its benefits, overusing it can lead to unnecessary object creation. This can be particularly harmful in performance-critical applications.
public final class LargeDataObject {
private final int[] data;
public LargeDataObject(int[] data) {
this.data = data.clone();
}
// Rather than returning a new instance, consider using a builder
}
Why Consider Performance?
If classes carry significant data payloads, creating numerous immutable instances can induce memory overhead. Sometimes, it’s more efficient to use a mutable object with controlled access.
Best Practices for Implementing Immutability
Now that we are aware of the common pitfalls, let’s explore some best practices for safely implementing immutability in your Java applications.
1. Use Final Classes
Define your classes as final
to prevent inheritance, ensuring that subclasses cannot compromise the integrity of the superclass.
2. Make Fields Private and Final
Declare all fields as private
and final
to maintain encapsulation and prevent modifications.
3. Return Copies of Mutable Objects
When accessing mutable fields, always return copies instead of the original instance.
4. Consider Builder Patterns for Complex Objects
For complex immutable objects with many parameters, consider implementing a Builder pattern to manage readability and usability more effectively.
public final class User {
private final String username;
private final String email;
private User(Builder builder) {
this.username = builder.username;
this.email = builder.email;
}
public static class Builder {
private String username;
private String email;
public Builder setUsername(String username) {
this.username = username;
return this;
}
public Builder setEmail(String email) {
this.email = email;
return this;
}
public User build() {
return new User(this);
}
}
}
Why Use Builder Pattern?
The Builder pattern allows for the creation of complex objects in a manner that is clean and understandable. It especially shines when dealing with an object that has numerous attributes.
Lessons Learned
Embracing immutability in Java can enhance code clarity, maintainability, and performance. However, it comes with its challenges. By being mindful of common pitfalls and applying best practices, developers can harness the power of immutability while avoiding unnecessary complexity.
For further reading on this topic, you might find the following resources valuable:
- Effective Java by Joshua Bloch - A staple for Java developers discussing best practices.
- The Java™ Tutorials - Objects - A comprehensive guide on Java’s object-oriented features.
Feel free to reach out with any questions or comments regarding immutability in Java or anything else programming-related! Happy coding!