Common Pitfalls When Using JCA for Cryptography in Java
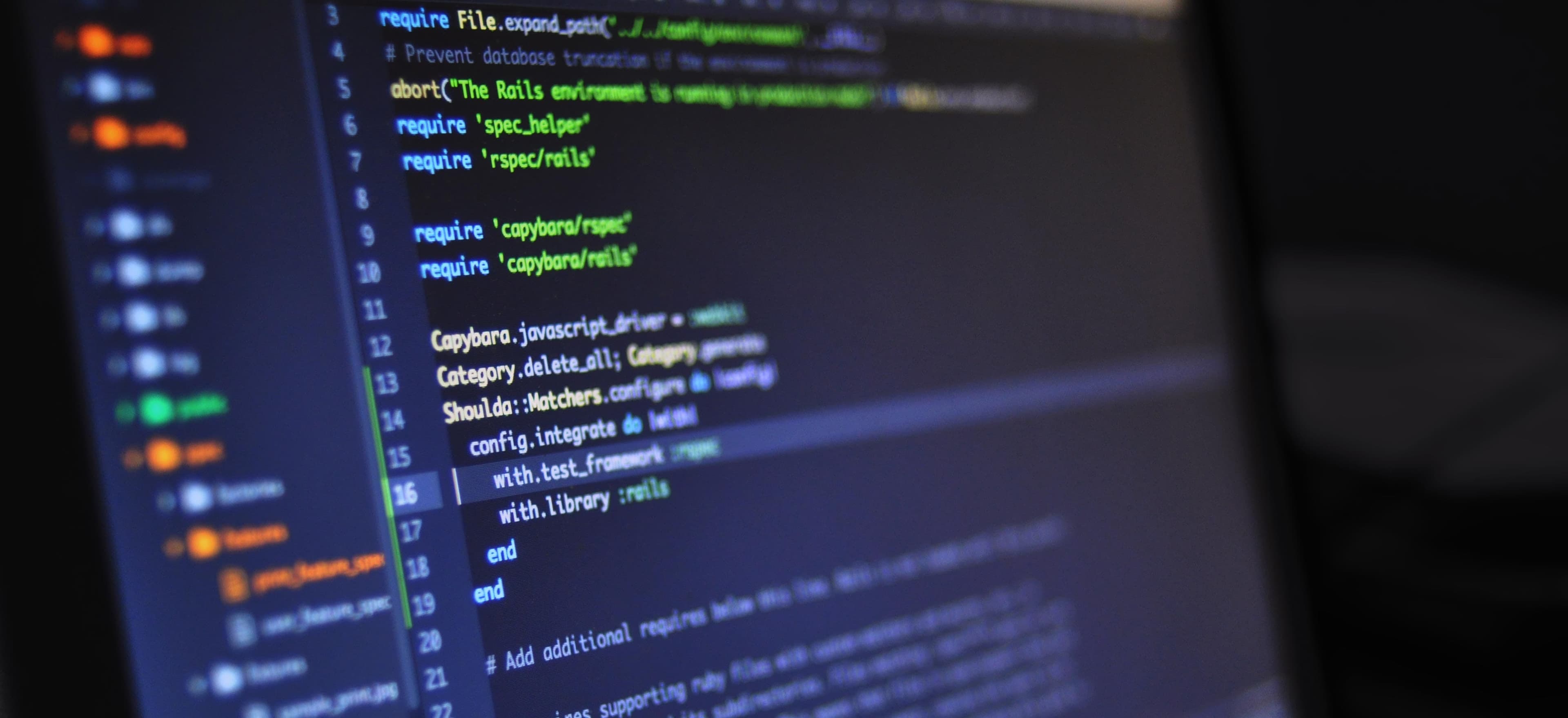
- Published on
Common Pitfalls When Using JCA for Cryptography in Java
The Java Cryptography Architecture (JCA) provides a robust framework for integrating cryptographic operations into Java applications, but it can be a minefield for developers not fully versed in its nuances. This blog post aims to navigate some of the common pitfalls associated with JCA to ensure that your implementation of cryptography in Java is both secure and efficient.
Understanding the JCA Framework
JCA is part of the Java Platform, Standard Edition (Java SE), and it provides a large set of cryptographic features including, but not limited to, encoding, hashing, and digital signatures. However, its complexity can sometimes lead developers astray.
Before diving into the pitfalls, it's important to highlight that secure coding practices in cryptography are essential. Using poorly designed algorithms, weak key sizes, or ignoring library updates can significantly compromise security.
Pitfall 1: Choosing the Wrong Algorithm
One of the fundamental mistakes developers make is selecting the wrong cryptographic algorithm. Different types of algorithms serve different purposes. Here's a brief overview:
- Symmetric Key Encryption (e.g., AES): Uses the same key for encryption and decryption.
- Asymmetric Key Encryption (e.g., RSA): Uses a pair of keys (public and private).
- Hash Functions (e.g., SHA-256): Converts data into a fixed-length string.
Example Usage
import javax.crypto.Cipher;
import javax.crypto.KeyGenerator;
import javax.crypto.SecretKey;
import javax.crypto.spec.SecretKeySpec;
public class AesEncryption {
public static void main(String[] args) throws Exception {
// Generate a new AES encryption key
KeyGenerator keyGen = KeyGenerator.getInstance("AES");
keyGen.init(256); // Key size: 128, 192, or 256 bits
SecretKey secretKey = keyGen.generateKey();
// Create a Cipher instance for AES encryption
Cipher cipher = Cipher.getInstance("AES");
String originalText = "Hello, World!";
// Encrypt
cipher.init(Cipher.ENCRYPT_MODE, secretKey);
byte[] encryptedText = cipher.doFinal(originalText.getBytes());
// Decrypt
cipher.init(Cipher.DECRYPT_MODE, secretKey);
byte[] decryptedText = cipher.doFinal(encryptedText);
System.out.println("Decrypted Text: " + new String(decryptedText));
}
}
Why This Matters
Using an outdated or insecure algorithm can expose your application to vulnerabilities. Always choose well-established algorithms and keep abreast of the latest security advisories. For in-depth guidance, check NIST's Cryptographic Standards.
Pitfall 2: Improper Key Management
Failing to manage cryptographic keys can render your encryption efforts useless. Poor key management practices can lead to keys being leaked, lost, or improperly generated, potentially compromising your entire system.
Essentials of Key Management
- Key Generation: Ensure random keys using a secure random number generator.
- Key Storage: Avoid hardcoding keys in your source code. Use a secure key storage solution, like a Hardware Security Module (HSM) or Java KeyStore (JKS).
- Key Rotation: Regularly replace keys to minimize risk.
Example of Key Generation
import java.security.SecureRandom;
public class KeyManagement {
public static void main(String[] args) throws Exception {
// Use SecureRandom for key generation
SecureRandom secureRandom = new SecureRandom();
byte[] key = new byte[32]; // 256 bits
secureRandom.nextBytes(key);
System.out.println("Generated Key (Hex): " + bytesToHex(key));
}
// Utility function to convert bytes to hexadecimal format
private static String bytesToHex(byte[] bytes) {
StringBuilder hexString = new StringBuilder();
for (byte b : bytes) {
String hex = Integer.toHexString(0xff & b);
if (hex.length() == 1) hexString.append('0');
hexString.append(hex);
}
return hexString.toString();
}
}
Why Key Management is Critical
Without solid key management, your cryptographic implementations could be effectively useless. Always audit your key management practices and follow industry best practices.
Pitfall 3: Ignoring Security Updates
The cryptographic landscape is perpetually evolving. Vulnerabilities in algorithms or libraries can emerge, making updates vital.
Keeping Libraries Up-To-Date
- Regularly check for updates to Java's security libraries.
- Follow discussions on Java security forums or repositories to stay informed.
Example Check for Updates
If you use Maven, your pom.xml
can keep track of library versions. Use the following command to check for outdated dependencies which may include your cryptographic libraries:
mvn versions:display-dependency-updates
Pitfall 4: Using Weak Random Number Generators
Random numbers play a critical role in cryptography—be it for key generation, initialization vectors (IVs), or nonces. Using non-cryptographically secure random number generators can expose your application to attacks.
Why Secure Random Generation Matters
For cryptographic operations, prefer SecureRandom
over Random
:
import java.security.SecureRandom;
public class SecureRandomDemo {
public static void main(String[] args) {
SecureRandom secureRandom = new SecureRandom();
byte[] randomBytes = new byte[16];
secureRandom.nextBytes(randomBytes);
System.out.println("Secure Random Bytes: " + bytesToHex(randomBytes));
}
}
Pitfall 5: Not Considering Padding
In block ciphers, padding is often necessary to ensure that the plaintext aligns with the block size. Incorrectly implementing padding can lead to vulnerabilities and cause decryption to fail.
Correct Padding Importance
Using proper padding schemes, such as PKCS5, helps safeguard against specific attacks:
Cipher cipher = Cipher.getInstance("AES/CBC/PKCS5Padding");
Discussing Padding Options
When designing a cryptographic system, always ensure that you've implemented proper padding schemes to protect data integrity.
The Last Word
As you can see, navigating the complexities of the Java Cryptography Architecture requires an understanding of not just how to encrypt and decrypt data, but of the underlying principles of security. By avoiding these common pitfalls and adhering to best practices, you can build a more secure Java application that harnesses the full power of JCA effectively.
For deeper insights on cryptographic practices, consider visiting the National Institute of Standards and Technology (NIST) and the Oracle Java Documentation. These resources will provide you with up-to-date and comprehensive information that can help solidify your understanding of cryptography in Java.
Happy coding, and remember: in the world of cryptography, security is a journey, not a destination!
Checkout our other articles