Optimizing OptaPlanner Logging for Better Performance
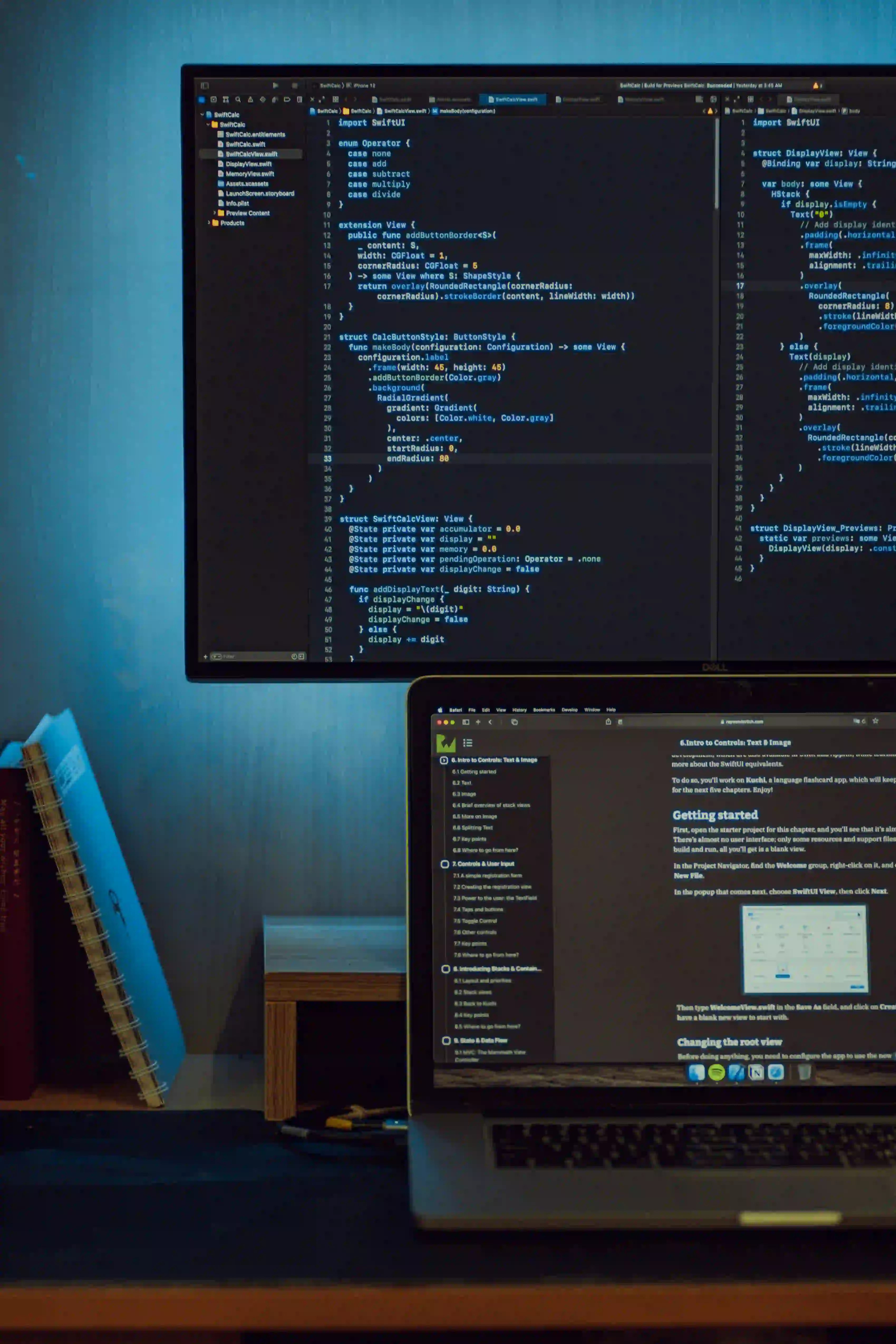
Optimizing OptaPlanner Logging for Better Performance
OptaPlanner is a popular open-source planning optimization engine in Java that enables developers to create intelligent, optimal solutions for complex planning problems. However, as with any complex system, logging can become a performance bottleneck if not managed correctly. This blog post will delve into effective strategies for optimizing OptaPlanner logging to enhance performance while maintaining useful insights into the system's behavior.
Understanding OptaPlanner Logging
Before diving into optimization techniques, let's briefly overview OptaPlanner logging. Logging plays a vital role in understanding how your application behaves under various conditions. OptaPlanner uses the SLF4J logging facade, which allows you to pipe log messages to different logging libraries (like Log4j, Logback, etc.). While logging is essential for debugging and monitoring, excessive logging can lead to increased overhead, especially in computationally intensive applications such as those using OptaPlanner.
The Cost of Logging
Logging can impact performance due to:
- I/O Overhead: Writing log messages to files or console is time-consuming.
- Memory Overhead: High-frequency logging can consume significant memory.
- Concurrency Issues: If multiple threads are writing logs simultaneously, it can lead to contention and slowdown.
To minimize the performance impact, we need to adjust our logging strategy properly.
Logging Configuration
Level Adjustment
One of the most straightforward methods to optimize logging is to adjust the logging level. OptaPlanner provides various logging levels: TRACE, DEBUG, INFO, WARN, ERROR.
- Production Environments: Set the logging level to WARN or ERROR to capture only critical issues.
- Development: Use DEBUG or TRACE for detailed outputs.
Here’s how you might configure this in your logback.xml
file for a production environment:
<configuration>
<logger name="org.optaplanner" level="WARN"/>
<root level="ERROR">
<appender-ref ref="CONSOLE"/>
</root>
</configuration>
By tuning the log level appropriately, you ensure that only essential logs are captured, reducing the overhead.
Conditional Logging
Sometimes, you may want to log specific messages based on certain conditions. Using conditional checks can prevent unnecessary logging calls in cases where logging might not be needed.
Here’s a conditional logging example:
if (logger.isDebugEnabled()) {
logger.debug("Current score: {}", score);
}
Utilizing the isDebugEnabled
method checks whether the DEBUG level is active before constructing the log message. This could save the cost of formatting the message when it won't be logged anyway.
Asynchronous Logging
If your application has high logging demands, consider asynchronous logging. This can help to offload the logging I/O operations to a separate thread, minimizing the impact on your main application threads.
Using Logback, you can implement asynchronous logging with the following configuration:
<configuration>
<appender name="ASYNC" class="ch.qos.logback.classic.AsyncAppender">
<appender-ref ref="FILE"/>
<queueSize>512</queueSize>
<discardingThreshold>0</discardingThreshold>
</appender>
<logger name="org.optaplanner" level="DEBUG" additivity="false">
<appender-ref ref="ASYNC"/>
</logger>
</configuration>
Benefits of Asynchronous Logging
- Performance: Reduces the logging latency in your application threads.
- Responsiveness: Improves application responsiveness under heavy load.
However, keep in mind that asynchronous logging can introduce slight complexity in terms of ensuring message delivery during application shutdown.
Log Sampling
In scenarios where logs can be too verbose (like performance metrics every second), log sampling can help. This means that you log only a percentage of the logs:
if ( ThreadLocalRandom.current().nextInt(100) < 5 ) {
logger.info("Current state: {}", state);
}
The above example would log only 5% of the messages, drastically reducing log volume while still keeping an overview of the application's behavior.
Enriching Logs with Context
Adding contextual information can enhance the usefulness of fewer logs. Instead of having many logs, enrich each log with context that explains the what, why, and where.
public void optimizePlan(PlanningSolution solution) {
logger.info("Starting optimization for solution ID: {}", solution.getId());
// Optimization logic
...
logger.info("Completed optimization for solution ID: {}, result: {}", solution.getId(), result);
}
Using structured logging techniques with context adds clarity without increasing log volume significantly. Consider using frameworks like Logstash for resources that promote structured logging.
Monitoring and Alerting
After optimizing logging, ensure you have monitoring in place to catch issues that may arise. Tools such as Prometheus or ELK Stack (Elasticsearch, Logstash, and Kibana) can provide insights into log usage and help ensure that your logging needs are met without flushing performance.
The Closing Argument
Optimizing OptaPlanner logging is crucial to maintaining the application's performance while ensuring critical information is captured. By adjusting log levels, considering asynchronous logging, implementing conditional checks, and enhancing logs with context, you can significantly improve performance without compromising on insights.
As a final note, always profile your application after making changes to the logging strategy. Each application is different, and the optimal configuration will depend on its unique requirements.
By following these strategies, you will set a strong foundation for effective logging within your OptaPlanner application.
For further reading about logging in Java applications, you may want to check out the SLF4J documentation and Logback for practical examples and advanced configurations.