Tackling Slow Transaction Callbacks in Spring Applications
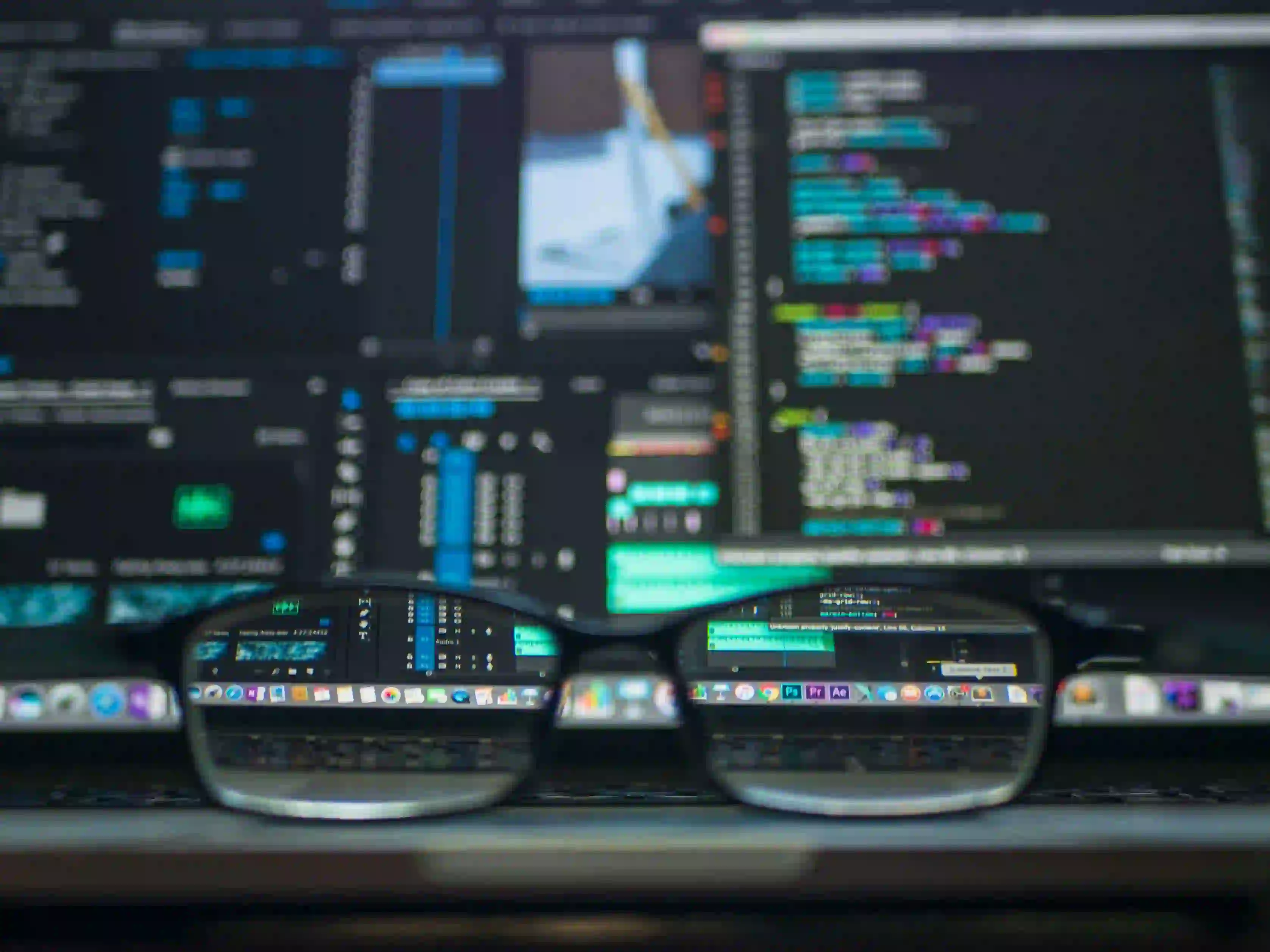
Tackling Slow Transaction Callbacks in Spring Applications
In today's fast-paced digital landscape, application performance is paramount. Slow transaction callbacks can significantly impact the user experience, potentially leading to frustration and lost customers. This blog post explores the causes of slow transaction callbacks in Spring applications and offers strategies to diagnose and resolve these issues effectively.
Understanding Spring Transaction Management
Spring Framework provides a comprehensive transaction management framework that helps developers manage transactional data with ease. It supports both programmatic and declarative transaction management, which makes it a versatile tool.
Why Transaction Management is Important
Transaction management is crucial because it ensures data integrity, allows for rollback in case of errors, and maintains consistency across multiple operations. Inadequate handling of transactions can lead to performance bottlenecks, especially when dealing with high concurrency.
Common Causes of Slow Transaction Callbacks
Before we dive into diagnosing and resolving slow transaction callbacks, it’s important to understand common causes:
- Database Locking: When multiple transactions try to access the same resource, locking mechanisms can slow down execution.
- Long-Running Queries: Inefficient queries can lead to prolonged execution times.
- Improperly Managed Transactions: Mixing different transaction management strategies can result in performance hits.
- Network Latency: Calling external services or databases can introduce latency, especially in distributed applications.
- Resource Contention: Limited resources like CPU and memory can throttle transaction performance.
Diagnosing Slow Transaction Callbacks
To tackle slow transaction callbacks, we first need to diagnose the root cause. Here are several diagnostic strategies:
1. Enable SQL Logging
Enabling SQL logging can provide insight into what queries are being executed and how long they take. In your application.properties
, add the following:
spring.jpa.show-sql=true
spring.jpa.properties.hibernate.format_sql=true
This will log all SQL statements generated by Hibernate. While this may affect performance, it is critical for diagnosing slow transactions.
2. Use a Profiler
Tools like VisualVM or Spring Boot Actuator can help you profile your application. They allow you to visualize performance metrics and identify bottlenecks.
import org.springframework.boot.actuate.metrics.MetricsEndpoint;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class MetricsController {
@Autowired
private MetricsEndpoint metricsEndpoint;
@GetMapping("/metrics")
public String getMetrics() {
return metricsEndpoint.listNames().toString();
}
}
Using Spring Boot Actuator, this endpoint allows you to check a plethora of metrics easily.
3. Analyze Application Logs
Check your application logs for any warnings or errors that occur during transaction processing. Look for recurring patterns that might indicate underlying issues, such as excessive retries or timeouts.
4. Monitor Database Performance
Utilize monitoring tools such as MySQL Performance Schema or PostgreSQL's pg_stat_statements to monitor database performance. Keep an eye on slow queries and consider adding indexes where appropriate.
Implementing Solutions for Slow Transaction Callbacks
Once you have diagnosed the issues, it's time to apply solutions. Here are some effective strategies:
1. Optimize Database Queries
Review and optimize your SQL queries. Ensure that you are only fetching what you need.
Here's an example of a poorly optimized query:
SELECT * FROM users WHERE status = 'active';
Instead, specify only the columns you need:
SELECT id, name, email FROM users WHERE status = 'active';
2. Use Transaction Propagation Wisely
Spring's transaction propagation settings (REQUIRED, REQUIRES_NEW, etc.) can significantly influence performance. Defaulting to REQUIRED
is often the best practice, but make sure it aligns with your application’s needs.
Here's how to set it:
@Transactional(propagation = Propagation.REQUIRED)
public void saveUser(User user) {
userRepository.save(user);
}
Use REQUIRES_NEW
for operations that must occur separately, but keep in mind it can lock resources longer than necessary.
3. Implement Caching
Caching frequently accessed data can reduce the number of transactions, leading to improved performance. You can use Spring’s built-in caching support.
Here's a basic example using the @Cacheable
annotation:
import org.springframework.cache.annotation.Cacheable;
@Service
public class UserService {
@Cacheable("users")
public User getUserById(Long id) {
return userRepository.findById(id).orElse(null);
}
}
Make sure to configure a caching provider, such as Ehcache or Redis.
4. Asynchronous Processing
Consider making long-running operations asynchronous, offloading them from the main transaction flow. You can use Spring's @Async
annotation for this.
import org.springframework.scheduling.annotation.Async;
import org.springframework.stereotype.Service;
@Service
public class NotificationService {
@Async
public void sendEmailNotification(String recipient) {
// Code to send email
}
}
5. Use Connection Pooling
Connection pooling can significantly enhance performance by reusing existing database connections rather than creating new ones for each transaction. Tools like HikariCP or Apache Commons DBCP can be integrated easily with Spring.
Add HikariCP to your dependencies:
<dependency>
<groupId>com.zaxxer</groupId>
<artifactId>HikariCP</artifactId>
</dependency>
And configure it in application.properties
:
spring.datasource.hikari.maximum-pool-size=10
My Closing Thoughts on the Matter
Tackling slow transaction callbacks in Spring applications requires a layered approach that involves diagnosing performance bottlenecks, optimizing queries, carefully managing transactions, and implementing caching and asynchronous processing. By keeping these best practices in mind and continuously monitoring your application's performance, you can ensure a smooth and efficient user experience.
If you're interested in learning more about Spring's transaction management, consider exploring the Spring Framework Documentation for an in-depth understanding.
Discussion Time:
What are some challenges you have faced regarding transaction management in your Spring applications? Feel free to share your insights or questions in the comments below!