Eliminating Code Duplication in Spring MVC Controllers
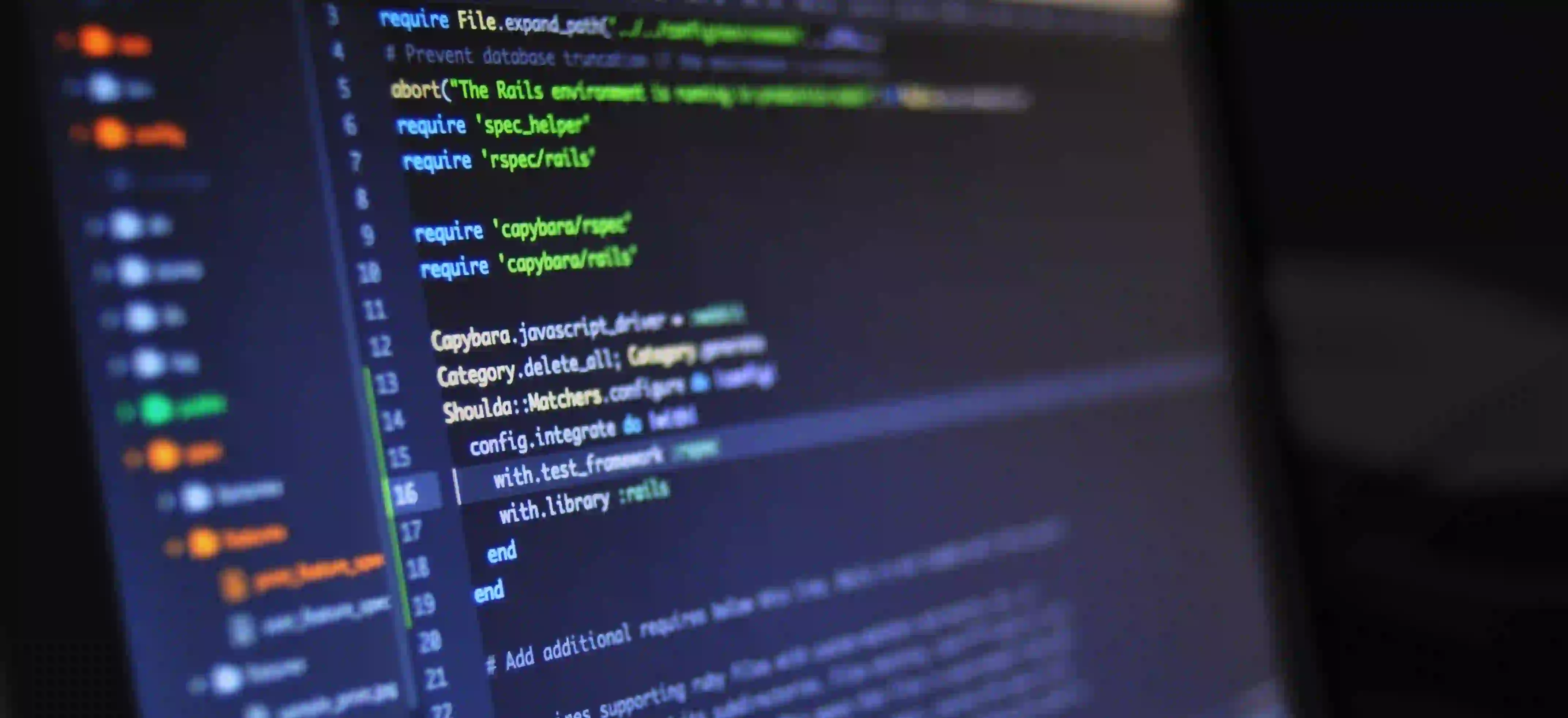
Eliminating Code Duplication in Spring MVC Controllers
In modern software development, code duplication can lead to maintenance challenges and increased potential for bugs. It's particularly troublesome in Spring MVC applications, where controller methods often mirror one another due to shared logic. In this blog post, we'll explore strategies for eliminating code duplication in Spring MVC controllers, enhancing code maintainability, readability, and reusability.
Understanding the Problem
When you have multiple controllers handling similar requests, it is common to find redundant code. For instance, multiple methods may handle validation, logging, or data transformation in the same way, leading to maintenance nightmares down the line. Let's illustrate with a simple example.
@Controller
@RequestMapping("/api/products")
public class ProductController {
@GetMapping("/{id}")
public ResponseEntity<ProductDTO> getProduct(@PathVariable("id") Long id) {
Product product = productService.findById(id);
if (product == null) {
return ResponseEntity.notFound().build();
}
return ResponseEntity.ok(ProductMapper.toDto(product));
}
@PostMapping
public ResponseEntity<ProductDTO> createProduct(@RequestBody ProductDTO productDTO) {
if (productDTO.getName() == null || productDTO.getPrice() <= 0) {
return ResponseEntity.badRequest().build();
}
Product product = ProductMapper.toEntity(productDTO);
Product createdProduct = productService.save(product);
return ResponseEntity.status(HttpStatus.CREATED).body(ProductMapper.toDto(createdProduct));
}
}
In the above example, both methods check whether the input data is valid and convert the product object to and from DTOs. This situation calls for an approach to handle these common tasks in a more elegant way.
Strategies for Eliminating Duplication
1. Use a Base Controller
One of the simplest solutions is to create a base controller with common functionality that other controllers can extend.
public abstract class BaseController {
protected ResponseEntity<ProductDTO> handleNotFound(Product product) {
if (product == null) {
return ResponseEntity.notFound().build();
}
return ResponseEntity.ok(ProductMapper.toDto(product));
}
protected ResponseEntity<ProductDTO> handleValidation(ProductDTO productDTO) {
if (productDTO.getName() == null || productDTO.getPrice() <= 0) {
return ResponseEntity.badRequest().build();
}
return null; // Indicate no validation errors
}
}
Then, your ProductController
can extend this base controller.
@Controller
@RequestMapping("/api/products")
public class ProductController extends BaseController {
@GetMapping("/{id}")
public ResponseEntity<ProductDTO> getProduct(@PathVariable("id") Long id) {
Product product = productService.findById(id);
return handleNotFound(product);
}
@PostMapping
public ResponseEntity<ProductDTO> createProduct(@RequestBody ProductDTO productDTO) {
ResponseEntity<ProductDTO> validationResponse = handleValidation(productDTO);
if (validationResponse != null) {
return validationResponse;
}
Product product = ProductMapper.toEntity(productDTO);
Product createdProduct = productService.save(product);
return ResponseEntity.status(HttpStatus.CREATED).body(ProductMapper.toDto(createdProduct));
}
}
2. Aspect-Oriented Programming (AOP)
Another powerful approach is to use Aspect-Oriented Programming (AOP) to separate cross-cutting concerns like logging, input validation, and error handling.
Logging Example
@Aspect
@Component
public class LoggingAspect {
@Before("execution(* com.example.controller..*(..))")
public void logBefore(JoinPoint joinPoint) {
System.out.println("Entering method: " + joinPoint.getSignature().getName());
}
}
Validation Example
You can create a custom annotation and target specific methods.
@Target(ElementType.METHOD)
@Retention(RetentionPolicy.RUNTIME)
public @interface ValidatedInput {}
Then, create an aspect that handles validation.
@Aspect
@Component
public class ValidationAspect {
@Before("@annotation(ValidatedInput)")
public void validateInput(JoinPoint joinPoint) {
// Obtain method arguments and implement validation logic
}
}
Incorporating these aspects keeps your controllers clean and focuses them on handling HTTP requests rather than on repetitive logic.
3. Service Layer for Business Logic
Moving business logic out of the controller into a dedicated service layer is another way to streamline your Spring MVC architecture. This separation allows your controllers to handle HTTP requests and responses while delegating business-related tasks to services.
@Service
public class ProductService {
public Product findById(Long id) {
// Business logic for finding a product
}
public Product save(Product product) {
// Business logic for saving a product
}
public void validateProduct(ProductDTO productDTO) {
if (productDTO.getName() == null || productDTO.getPrice() <= 0) {
throw new ValidationException("Invalid product data");
}
}
}
Now, in your controller, you only need to handle HTTP interactions.
@PostMapping
public ResponseEntity<ProductDTO> createProduct(@RequestBody ProductDTO productDTO) {
productService.validateProduct(productDTO);
Product product = ProductMapper.toEntity(productDTO);
Product createdProduct = productService.save(product);
return ResponseEntity.status(HttpStatus.CREATED).body(ProductMapper.toDto(createdProduct));
}
This arrangement keeps your code clean and easy to test. More about structuring service layers can be found here.
4. Utility Methods
For logic that is shared across various controllers but does not justify a new aspect or service, consider using utility methods. These methods can live in a separate class, offering reusable functions that can be called from controller methods.
public class ProductUtil {
public static boolean isValidProduct(ProductDTO productDTO) {
return productDTO.getName() != null && productDTO.getPrice() > 0;
}
}
And in your controller:
@PostMapping
public ResponseEntity<ProductDTO> createProduct(@RequestBody ProductDTO productDTO) {
if (!ProductUtil.isValidProduct(productDTO)) {
return ResponseEntity.badRequest().build();
}
Product product = ProductMapper.toEntity(productDTO);
Product createdProduct = productService.save(product);
return ResponseEntity.status(HttpStatus.CREATED).body(ProductMapper.toDto(createdProduct));
}
This practice reduces boilerplate code and encapsulates common logic without cluttering your controllers.
Wrapping Up
Eliminating code duplication in Spring MVC controllers is essential for maintainable and scalable code. By leveraging concepts such as base controllers, AOP, service layers, and utility classes, you can dramatically reduce redundancy and improve the organization of your application layers.
As you refine your approach, remember to prioritize readability and simplicity. Ultimately, the goal is to create a foundation that not only reduces duplication but also enhances the overall functionality and ease of maintenance of your application.
By implementing these strategies, your Spring MVC projects will become a model of clean design principles. For more on optimizing your Spring applications, check out Spring Framework Documentation.
Happy coding!