Streamline Your CRUD Operations in Java 8: Common Pitfalls
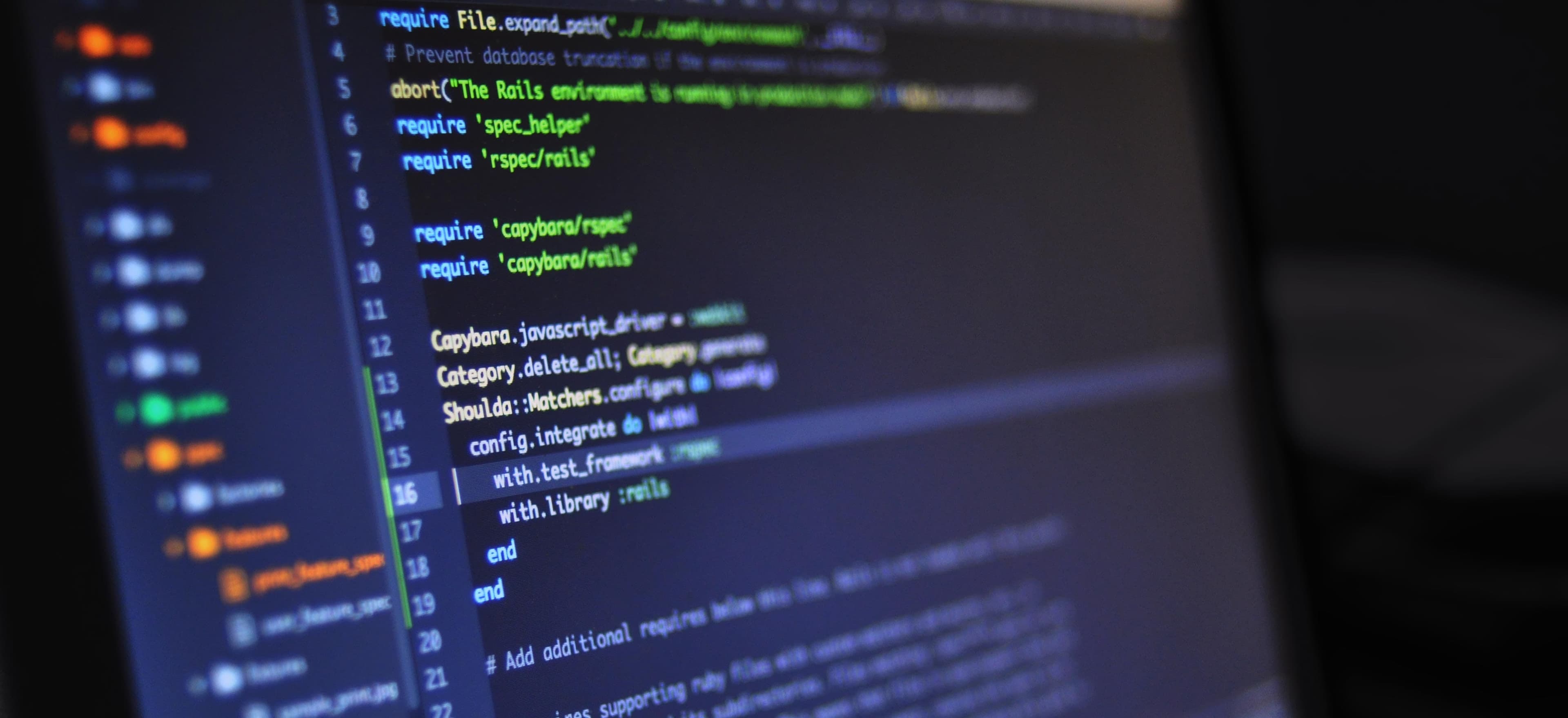
- Published on
Streamline Your CRUD Operations in Java 8: Common Pitfalls
As web applications become more advanced and data-responsive, understanding and optimizing CRUD (Create, Read, Update, Delete) operations has never been more important. Java 8 introduced a plethora of features, including lambda expressions, streams, and the optional class, that can enhance how we manage CRUD operations. In this blog post, we will explore these advantages while also shedding light on common pitfalls that developers may encounter.
Table of Contents
- Understanding CRUD Operations
- Java 8 Features for Improved CRUD
- Common Pitfalls in CRUD Operations
- Best Practices for Streamlined CRUD
- Conclusion
Understanding CRUD Operations
CRUD operations represent the essential actions performed on data in any application. These operations are foundational in data-oriented applications, allowing developers to interact seamlessly with databases and data repositories. As we implement these functions, it becomes vital to make our code clean, efficient, and maintainable.
Java 8 Features for Improved CRUD
Java 8 brings a layer of abstraction and simplicity to our CRUD operations. Let’s take a closer look at the primary features that assist in streamlining our operations.
1. Lambda Expressions
Lambda expressions are a significant addition to Java 8, offering a succinct way to express instances of single-method interfaces (functional interfaces). They reduce boilerplate code, making your CRUD operations more readable.
Example: Filtering a list with lambdas.
List<String> names = Arrays.asList("Alice", "Bob", "Charlie");
List<String> filteredNames = names.stream()
.filter(name -> name.startsWith("A"))
.collect(Collectors.toList());
System.out.println(filteredNames); // Output: [Alice]
Why: This allows you to eliminate tedious for-loops and enhances readability by expressing intentions clearly.
2. Streams API
The Streams API enables functional-style operations on collections of objects. It provides a powerful framework through which we can process sequences of elements and perform complex data manipulations concisely.
Example: Generating a list of names in upper case using streams.
List<String> names = Arrays.asList("Alice", "Bob", "Charlie");
List<String> upperCaseNames = names.stream()
.map(String::toUpperCase)
.collect(Collectors.toList());
System.out.println(upperCaseNames); // Output: [ALICE, BOB, CHARLIE]
Why: This method is highly declarative. Instead of detailing how the task is performed, you specify what you want – making the code easier to understand and maintain.
3. Optional Class
The Optional
class helps in dealing with null values gracefully. It provides a way to express that a value may be absent in a type-safe manner, reducing the chances of NullPointerExceptions
.
Example: Returning an optional user entity.
public Optional<User> findUserById(String id) {
return users.stream().filter(user -> user.getId().equals(id)).findFirst();
}
// Usage
Optional<User> user = findUserById("123");
user.ifPresent(u -> System.out.println(u.getName()));
Why: This avoids null checks throughout your codebase and allows for a cleaner approach when handling potential absence of values.
Common Pitfalls in CRUD Operations
While Java 8 brings numerous advantages for CRUD operations, it's important to be mindful of common pitfalls.
1. Not Leveraging Streams Effectively
Many developers are still accustomed to imperative programming, missing out on the expressiveness of streams. Hence, they may resort to for-loops and traditional approaches.
Solution: Practice using streams whenever applicable. Refactor existing loops into stream operations for a cleaner, more efficient implementation.
2. Overusing Optional
Despite the Optional
class being a great addition, overusing it can lead to bloated code and unnecessary complexity. If you excessively nest Optionals, you'll find yourself dealing with cumbersome code.
Solution: Use Optional
wisely. It’s best suited for method return types, especially when dealing with operations that may yield no result. Don’t convert every potential null to an Optional.
3. Ignoring Exception Handling
Error handling can often get overlooked, leading to unhandled exceptions that crash your application. When interacting with databases or APIs, it's crucial to implement proper exception handling.
Solution: Always aim to wrap your CRUD operations within try-catch blocks to handle unexpected errors and ensure they don't terminate your application.
try {
userRepository.save(user);
} catch (DatabaseException e) {
// Handle exception (logging, rethrowing, etc.)
System.err.println("Error saving user: " + e.getMessage());
}
Best Practices for Streamlined CRUD
Here are some best practices to follow in conjunction with Java 8 features:
- Keep it Simple: Don't over-engineer your solutions. Simple, straightforward methods are the easiest to read and maintain.
- Write Unit Tests: Always write tests for your CRUD operations. This ensures reliability and confidence when modifications are made.
- Use Meaningful Names: Choose clear, descriptive names for your methods and variables to enhance code readability.
- Optimize Performance: Carefully analyze the performance of your CRUD operations, especially when processing large datasets. Use parallel streams if necessary.
- Leverage Enums for Status Codes: When returning statuses from your CRUD functions, consider using enums to avoid magic strings that could lead to errors.
Lessons Learned
CRUD operations are fundamental to modern applications, and Java 8 equips us with powerful tools to streamline these processes. By leveraging lambda expressions, the Streams API, and the Optional class, developers can significantly enhance code readability and maintainability.
However, as we adopt these new practices, it’s essential to remain vigilant about common pitfalls. By following the best practices outlined in this article, you can create robust, efficient, and error-resistant CRUD functionalities in your Java applications.
For further learning, check out the Java official documentation and practice building various CRUD applications to solidify your understanding. Happy coding!