Unlock Custom Fonts in Android TextViews Easily!
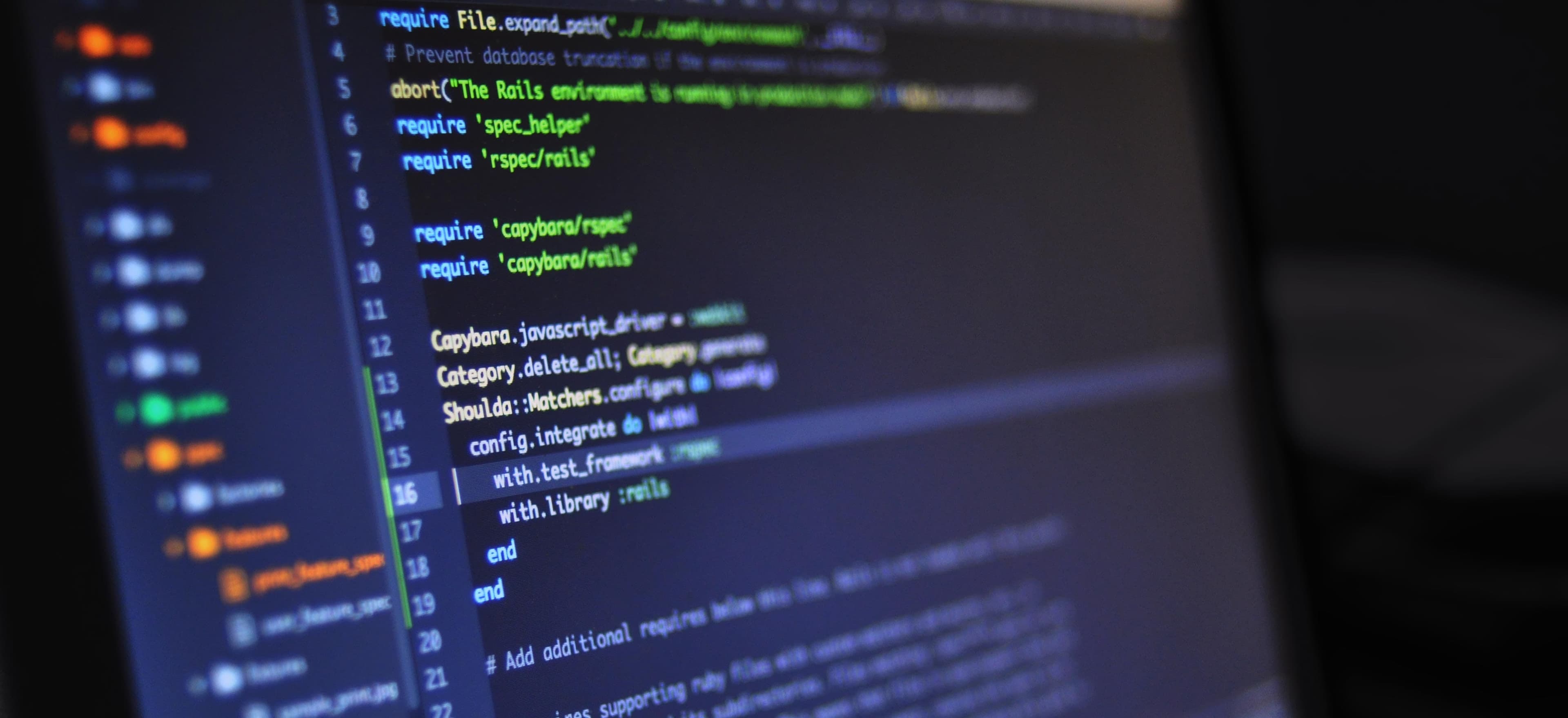
- Published on
Unlock Custom Fonts in Android TextViews Easily!
When it comes to building Android applications, fonts play a crucial role in delivering a unique user experience. Using custom fonts can help your app stand out, align with brand identity, and enhance readability. In this blog post, we will explore how to easily integrate custom fonts into TextViews within your Android application.
Why Use Custom Fonts?
Using custom fonts offers several advantages:
- Brand Identity: Custom typography can reinforce brand identity. If your brand has specific typographic guidelines, applying these in your app can ensure brand consistency.
- User Engagement: Well-chosen fonts can improve the overall aesthetics of an app, potentially increasing user engagement.
- Readability: Certain custom fonts are designed for better readability, making it easier for users to consume content.
Getting Started
Let's break down the process of integrating custom fonts into TextViews in Android. We will cover the following steps:
- Choose a custom font.
- Add the font to your project.
- Access the font programmatically.
- Applying the font to a TextView.
Step 1: Choose a Custom Font
Before we begin, you'll need to select a custom font. Websites like Google Fonts and DaFont offer a variety of fonts for personal and commercial use. For this tutorial, let's choose the "Roboto" font, which is widely used for Android applications.
Step 2: Add the Font to Your Project
After selecting your font, you need to add it to your project. Follow these steps:
- Save the downloaded font file (e.g., "Roboto-Regular.ttf").
- Navigate to your Android project structure.
- Create a new directory in
res
calledfont
(i.e.,res/font
). - Place the font file in the
font
directory.
Your project structure should look something like this:
app
└── src
└── main
└── res
└── font
└── roboto_regular.ttf
Step 3: Access the Font Programmatically
To apply the custom font to a TextView, you need to access the font programmatically. Below is an example of how you can do this:
Java Code
TextView textView = findViewById(R.id.myTextView);
Typeface typeface = ResourcesCompat.getFont(this, R.font.roboto_regular);
textView.setTypeface(typeface);
Explanation
- TextView Initialization: The
TextView
is initialized by finding its ID in the layout. - Loading the Font: The
ResourcesCompat.getFont()
method allows us to load the font we added to theres/font
directory. - Setting the Typeface: Finally, we set the typeface of the TextView via
setTypeface()
.
Using ResourcesCompat
ensures compatibility across different API levels.
Step 4: Applying the Font to a TextView in XML
If you prefer using XML, you can also apply the custom font directly in your layout XML file by using the following syntax:
<TextView
android:id="@+id/myTextView"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Hello World!"
android:fontFamily="@font/roboto_regular"/>
Additional Customizations
Beyond simply changing the font, consider applying additional attributes to TextViews for a better presentation. You can set text size, color, and style:
<TextView
android:id="@+id/myTextView"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Hello World!"
android:textSize="16sp"
android:textColor="@color/black"
android:fontFamily="@font/roboto_regular"/>
Code Snippet with Full Implementation
Here's a complete example of how your MainActivity might look:
package com.example.myapp;
import android.graphics.Typeface;
import android.os.Bundle;
import androidx.appcompat.app.AppCompatActivity;
import androidx.core.content.res.ResourcesCompat;
import android.widget.TextView;
public class MainActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
// Initialize TextView
TextView textView = findViewById(R.id.myTextView);
// Load custom typeface
Typeface typeface = ResourcesCompat.getFont(this, R.font.roboto_regular);
// Set custom font to TextView
textView.setTypeface(typeface);
}
}
To Wrap Things Up
Implementing custom fonts in Android TextViews is an easy yet significant enhancement for your application. Whether you choose to apply the font programmatically or directly in XML, this flexibility allows for a more personalized touch in your UI design.
Take your app’s aesthetics to the next level with custom fonts and improve user engagement. Experiment with different fonts and styles, and see how they fit into your app's branding.
For more detailed information, consider checking the official documentation on Custom Fonts by Android Developer.
By integrating custom fonts, you not only adopt a contemporary design trend but also create a distinct identity for your app. Start experimenting today!
If you found this blog post helpful, share it with your fellow Android developers! Have any questions? Feel free to reach out in the comments below. Happy coding!