Overcoming Common Printing Issues in JavaFX 8
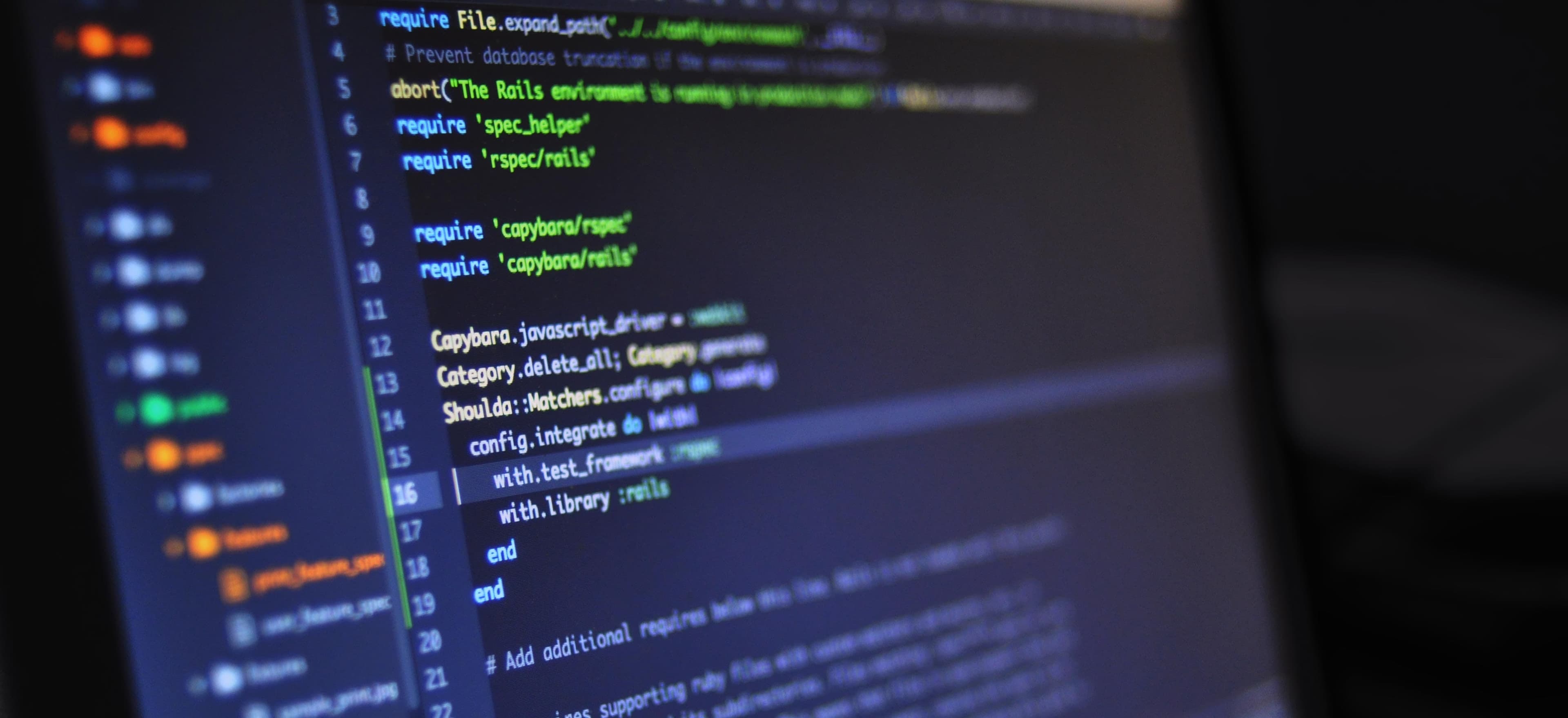
- Published on
Overcoming Common Printing Issues in JavaFX 8
JavaFX provides a powerful framework for building desktop applications in Java. As developers, we often need to incorporate functionality for printing documents, reports, or information. However, unexpected printing issues can arise. In this blog post, we'll explore some common printing issues in JavaFX 8, their causes, and how to overcome them.
Why JavaFX for Printing?
JavaFX offers several benefits:
- Rich UI Components: JavaFX supports a rich set of UI components which makes it ideal for creating visually appealing applications.
- Cross-Platform: JavaFX applications can run on multiple platforms without needing modifications, simplifying the deployment process.
- Ease of Use: The JavaFX printing API is relatively straightforward, making it easier for developers to manage print jobs.
With these advantages in mind, let’s dive into the common printing problems developers face, along with some solutions.
Common Printing Issues in JavaFX 8
1. Missing Printer Selection
Issue: Users often report that they cannot select a printer or the wrong printer is being used.
Solution: Ensure you've properly implemented the printer selection dialog.
Here is a basic example of how to display a printer setup dialog:
PrinterJob job = PrinterJob.createPrinterJob();
if (job != null && job.showPrintDialog(primaryStage)) {
// Proceed with printing
}
Explanation: This code creates a print job and opens the printer dialog. If successfully initiated, it allows users to select their preferred printer.
2. Print Job Not Initialized Correctly
Issue: Sometimes, the print job does not initialize properly, leading to exceptions or failing to print.
Solution: Always check if the printer job is valid and handle any exceptions gracefully.
Here’s how you can handle the situation:
try {
PrinterJob job = PrinterJob.createPrinterJob();
if (job != null && job.showPrintDialog(primaryStage)) {
// The content to print goes here
boolean success = job.printPage(someNode); // 'someNode' is the content to print
if (success) {
job.endJob();
} else {
System.out.println("Printing failed.");
}
}
} catch (Exception e) {
e.printStackTrace();
System.out.println("An error occurred while initializing the print job.");
}
Explanation: The code above uses a try-catch block to capture any exceptions. It ensures the print job ends properly when successful and provides feedback on failure.
3. Incorrect Page Orientation
Issue: Printed documents may come out in the wrong orientation (landscape vs. portrait).
Solution: Setting the correct page orientation before sending the print job is essential.
Example code for setting orientation:
PrinterJob job = PrinterJob.createPrinterJob();
if (job != null && job.showPrintDialog(primaryStage)) {
PageLayout layout = job.getJobSettings().getPageLayout();
job.getJobSettings().setPageLayout(layout);
job.getJobSettings().setCollate(true); // Enable collating if needed
boolean success = job.printPage(someNode);
// Handle printing as shown previously
}
Explanation: The code retrieves the current page layout and allows you to modify it if necessary. It can prevent orientation mismatches.
4. Content Not Fitting on Page
Issue: Sometimes, the content does not fit well on the printed page, causing cutoff or margins issues.
Solution: Scale the printed content to fit the page dimensions.
Here is a simple way to scale your content:
PrinterJob job = PrinterJob.createPrinterJob();
if (job != null && job.showPrintDialog(primaryStage)) {
double scaleX = job.getJobSettings().getPageLayout().getPrintableWidth() / someNode.getBoundsInParent().getWidth();
double scaleY = job.getJobSettings().getPageLayout().getPrintableHeight() / someNode.getBoundsInParent().getHeight();
someNode.setScaleX(scaleX);
someNode.setScaleY(scaleY);
job.printPage(someNode);
// Don't forget to reset the scale afterwards
}
Explanation: The scaling is based on the current dimensions of the print page and the size of the content. By modifying the scale of the node, we ensure that the entire content fits on the printed page.
5. Handling Print Dialog Issues
Issue: Users sometimes report that the print dialog fails to appear or does not respond.
Solution: Always ensure that you are using the print management functions from the JavaFX Application Thread.
Here’s an example of enforcing execution on the JavaFX thread:
Platform.runLater(() -> {
PrinterJob job = PrinterJob.createPrinterJob();
if (job != null && job.showPrintDialog(primaryStage)) {
// Proceed with printing as needed
}
});
Explanation: Wrapping the code in Platform.runLater()
ensures that it executes on the JavaFX thread. This approach can prevent common threading issues.
Best Practices for JavaFX Printing
Logging for Debugging
Developers should include logging when implementing printing functionalities. Logging can help identify where and why various issues occur. Utilize a logging framework like SLF4J or Log4j to keep track of exceptions and print job states.
Testing on Different Printers
To ensure compatibility, test your application with various printer models. Printing behavior can differ significantly from one printer to another.
Reviewing JavaFX Documentation
The official JavaFX documentation is an invaluable resource. Regularly check it for updates, especially with respect to printing and related functionalities.
User Education
Provide helpful tooltips or documentation for end-users on common tasks, including how to access the print function effectively. Clear guidance can significantly reduce user-related printing issues.
The Last Word
While printing in JavaFX 8 can present challenges, understanding these common printing issues and their solutions can facilitate a smoother user experience. By taking proactive steps to manage print jobs effectively, scaling content, and ensuring proper initialization, developers can minimize frustrations related to printing.
By leveraging the provided code snippets and best practices outlined in this article, you can empower your application with robust printing functionality, enhancing user satisfaction.
For further reading on JavaFX and its capabilities, consider checking out JavaFX Tutorial, which covers everything from the basics to advanced features.
Additional Resources
- JavaFX Print API Documentation
- Explore Java Networking and I/O
Embrace these strategies and keep advancing your JavaFX applications' printing capabilities!