Understanding Java Hoisting: Avoiding Common Pitfalls
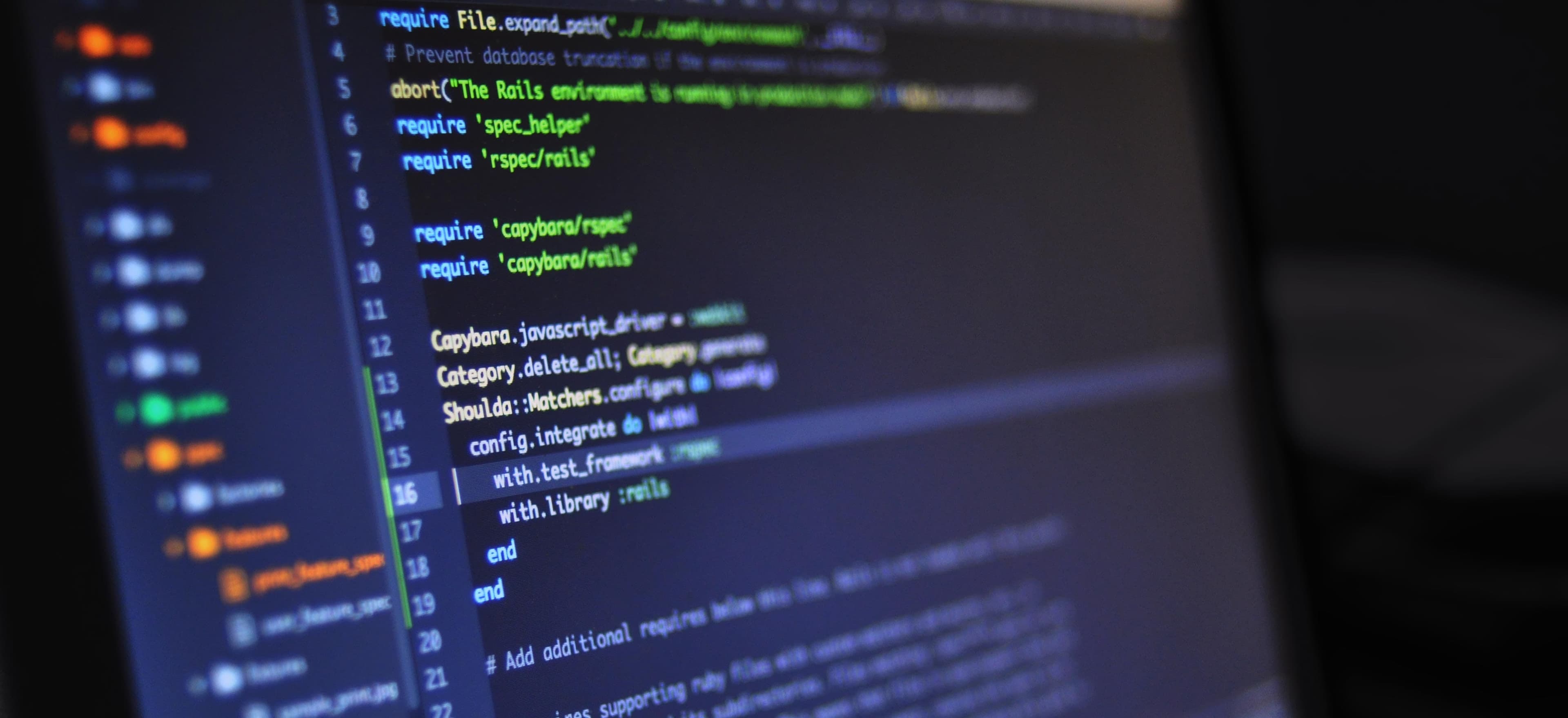
- Published on
Understanding Java Hoisting: Avoiding Common Pitfalls
When it comes to programming, especially in languages like Java, understanding variable scope and lifecycle is crucial. One topic that frequently leads to confusion is hoisting. While hoisting is a concept that's most commonly associated with JavaScript, Java has its nuances that developers should comprehend to avoid stumbling blocks. In this article, we will discuss Java hoisting, how it differs from JavaScript's behavior, and tips on circumventing common pitfalls.
What is Hoisting?
Hoisting is a behavior in programming languages that involves making variables available before their declaration in the code. While Java does not exhibit the same hoisting behavior as JavaScript, it has its own rules regarding variable declarations within different scopes, such as methods, classes, and blocks.
How Hoisting Differs in Java vs. JavaScript
In JavaScript, all variable declarations are hoisted to the top of their containing function or global scope. This means that you can access those variables even before you declare them, albeit they will be undefined
. For instance, in JavaScript:
console.log(x); // Outputs: undefined
var x = 5;
console.log(x); // Outputs: 5
In contrast, in Java, if you use a local variable before initializing it, the compiler will produce an error - you cannot access a local variable until you have both declared and initialized it:
public class HoistingExample {
public static void main(String[] args) {
System.out.println(x); // Compilation error: cannot find symbol
int x = 5; // Declaration happens here
System.out.println(x); // Outputs: 5
}
}
The above code will not compile because the variable x
is accessed before its declaration.
Scopes and Variable Types in Java
Java has different kinds of variable scopes:
- Class Variables (Static Variables) - These are shared among all instances of a class.
- Instance Variables - These are tied to a specific instance of a class.
- Local Variables - These are found within methods, constructors, or blocks.
- Parameters - Variables that are passed to methods.
Class and Instance Variables
Both class and instance variables are initialized by default. Class variables (static) start with a default value of zero for numeric types, false
for boolean, and null
for objects. Here's a quick example:
public class VariableScope {
static int classVar; // Default value: 0
int instanceVar; // Default value: 0
public static void main(String[] args) {
VariableScope example = new VariableScope();
System.out.println("Class Variable: " + classVar); // Outputs: 0
System.out.println("Instance Variable: " + example.instanceVar); // Outputs: 0
}
}
Local Variables
Local variables, however, need to be explicitly initialized before use. This is a protection mechanism in Java to ensure that programmers don't use variables that are in an undefined state. Let's see this point illustrated in the example below:
public class LocalVariableExample {
public static void main(String[] args) {
int localVar; // Declaring a local variable
// System.out.println(localVar); // Uncommenting this line will cause a compile-time error
localVar = 10; // Initialization
System.out.println(localVar); // Outputs: 10
}
}
In the code snippet above, attempting to print localVar
before its initialization results in a compilation error.
Common Pitfalls in Java Hoisting
While Java’s treatment of variable declarations can prevent certain errors that JavaScript programmers face, there are still pitfalls that can occur, especially concerning variable scope and lifecycle.
1. Using Local Variables Before Declaration
As noted earlier, referencing a local variable before it has been declared can lead to compile-time errors. Always initialize local variables before usage:
public class Main {
public static void main(String[] args) {
int result; // Declared but not initialized
// Use before initializing
// System.out.println(result); // This will produce an error
result = 20; // Initialize later
System.out.println("Result: " + result); // Outputs: Result: 20
}
}
2. Shadowing Variables
Shadowing occurs when a local variable has the same name as an instance variable, causing confusion. The local variable will have precedence, which could lead to unexpected behavior if you do not recognize this scope.
public class ShadowingExample {
int x = 100; // Instance variable
public void display(int x) { // Parameter x shadows instance variable x
System.out.println("Local x: " + x); // Outputs the parameter
System.out.println("Instance x: " + this.x); // Outputs the instance variable
}
public static void main(String[] args) {
ShadowingExample example = new ShadowingExample();
example.display(200); // Outputs Local x: 200 and Instance x: 100
}
}
3. Final Variables and Initialization
Java allows the declaration of final variables, which must be initialized exactly once. If you attempt to use final variables before assigning them, you’ll encounter errors.
public class FinalVariableExample {
final int finalVar; // Final variable
public FinalVariableExample() {
// finalVar = 10; // This is acceptable
finalVar = 20; // Correct way to initialize final variables
}
public static void main(String[] args) {
FinalVariableExample example = new FinalVariableExample();
System.out.println("Final Variable: " + example.finalVar); // Outputs: 20
}
}
Key Takeaways
Understanding hoisting in Java is fundamental for writing clean and error-free code. While Java does not have hoisting in the way JavaScript does, the proper handling of local and instance variables – along with awareness of scopes – is critical.
Navigating these concepts effectively allows you to write more robust Java applications, preventing issues that often arise from misusing variable scopes.
For a broader understanding of variable hoisting in general, you might want to take a look at JavaScript's hoisting behavior in the article "Why JavaScript Hoisting Can Break Your Code: A Deep Dive". Understanding these principles across languages can enhance your programming skills and help in avoiding common pitfalls.
Additional Resources
This comprehensive overview of hoisting in Java should arm you with the knowledge to avoid common pitfalls and write cleaner code. Happy coding!