Managing Backpressure in Java Streams for Optimal Performance
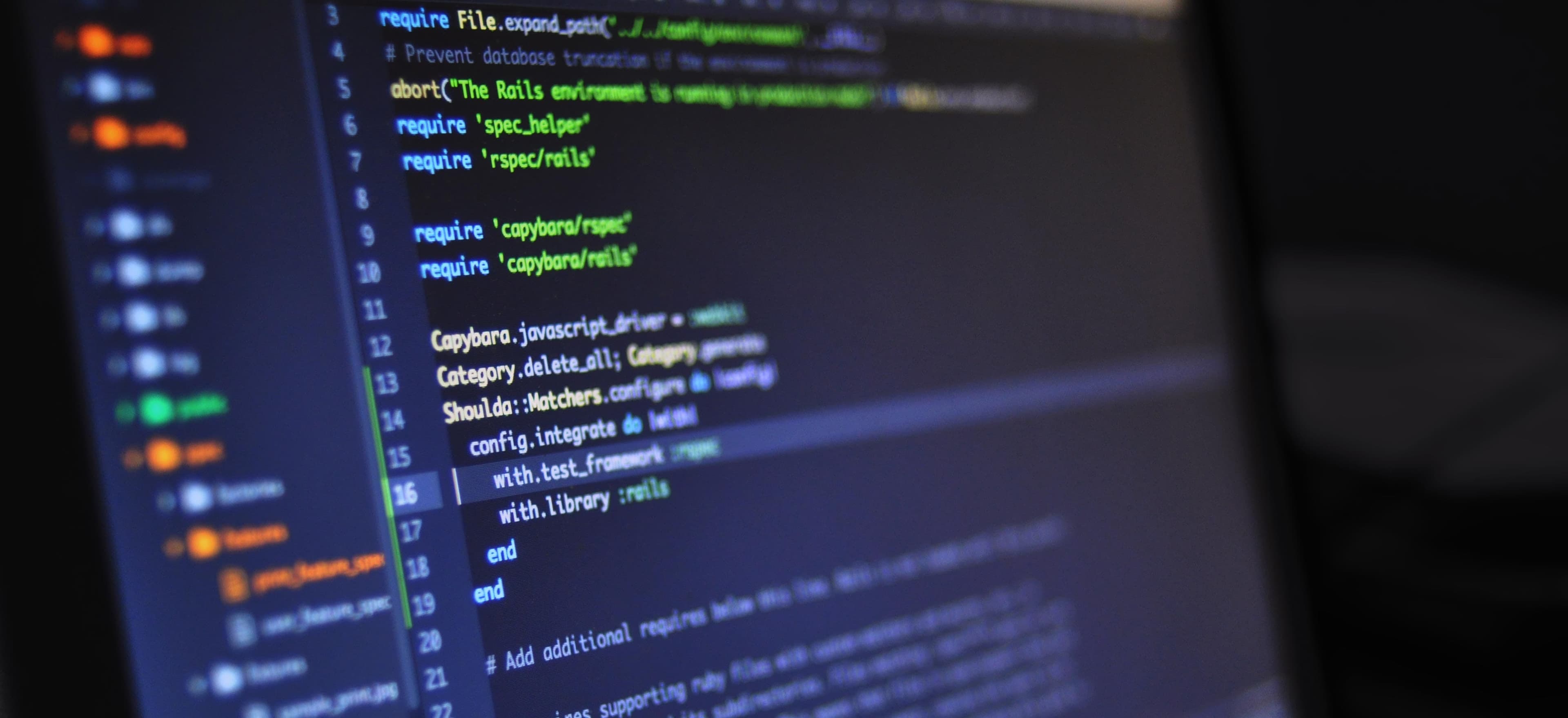
- Published on
Managing Backpressure in Java Streams for Optimal Performance
Backpressure is a crucial concept in reactive programming. It ensures that a system does not become overwhelmed when processing streams of data. As we dive into the world of Java Streams, it’s important to understand how to manage backpressure effectively to enhance application performance. This blog post discusses strategies and techniques you can implement in Java, while drawing parallels with Node.js, which has its own approach in the article Conquering Backpressure in Node.js Streams: A Guide.
What is Backpressure?
Backpressure occurs when a component in a data processing pipeline can't keep up with the incoming data rate, leading to systematic bottlenecks. Imagine a scenario where a production line produces goods faster than they can be packaged – a situation that can lead to pile-ups and inefficiencies. The same principle applies to software data processing.
In a Java context, streams provide a powerful abstraction for processing sequences of data. However, when working with streams, especially in real-time applications or data-intensive tasks, it's essential to implement backpressure mechanisms to avoid performance degradation.
Understanding Java Streams
Java Streams, introduced in Java 8, allow for functional-style operations on collections of data. Here’s a simple example:
import java.util.Arrays;
import java.util.List;
public class StreamExample {
public static void main(String[] args) {
List<String> fruits = Arrays.asList("Apple", "Banana", "Cherry");
fruits.stream()
.filter(fruit -> fruit.startsWith("A"))
.forEach(System.out::println);
}
}
Why Use Java Streams?
The primary advantage of using Java Streams is the ability to chain operations in a clear and concise manner. However, the more complex your stream operations become (with mapping, filtering, and collecting), the greater the potential for backpressure issues, especially if your data source produces data at a high rate.
Managing Backpressure in Java
1. Utilize Reactive Streams
Reactive programming frameworks like Project Reactor and RxJava offer built-in backpressure support. They allow systems to apply backpressure in a more sophisticated way than standard Java streams.
For example, in Project Reactor:
import reactor.core.publisher.Flux;
public class BackpressureExample {
public static void main(String[] args) {
Flux.range(1, 1000)
.doOnNext(data -> {
try {
Thread.sleep(10); // Simulate processing time
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
}
})
.subscribe(data -> System.out.println("Processed " + data));
}
}
Why Project Reactor?
Project Reactor uses a concept of "backpressure" that allows the downstream consumers to dictate how much data they can handle. In this snippet, the doOnNext
simulates a time-consuming operation that could introduce backpressure, indicating that the processing rate is slower than the production rate.
2. Buffering
Another strategy is to implement buffering. Buffers can temporarily store data elements until they can be processed, effectively smoothing out bursts in data production.
import java.util.concurrent.BlockingQueue;
import java.util.concurrent.LinkedBlockingQueue;
public class BufferingExample {
private static final BlockingQueue<Integer> buffer = new LinkedBlockingQueue<>(10);
public static void main(String[] args) {
// Producer Thread
new Thread(() -> {
for (int i = 0; i < 100; i++) {
try {
buffer.put(i); // Will block if buffer is full
System.out.println("Produced: " + i);
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
}
}
}).start();
// Consumer Thread
new Thread(() -> {
for (int i = 0; i < 100; i++) {
try {
int data = buffer.take(); // Will block if buffer is empty
System.out.println("Consumed: " + data);
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
}
}
}).start();
}
}
Buffering Rationale
The use of BlockingQueue
allows the producer to wait when the buffer is full and the consumer to wait when it's empty. This coordination is critical to managing data flow and preventing overflow conditions.
3. Throttling
Throttling is another strategy to control the data rate. By delaying the processing of elements in a stream, you can prevent the consumer from being flooded with too much data at once.
import reactor.core.publisher.Flux;
public class ThrottlingExample {
public static void main(String[] args) {
Flux.range(1, 100)
.delayElements(Duration.ofMillis(100)) // Throttle to handle backpressure
.subscribe(data -> System.out.println("Received: " + data));
}
}
Why Throttle?
In this example, delayElements
introduces a controlled delay between emitted items, reducing the risk of overwhelming the consumer and giving it adequate time to process.
When to Use Backpressure Management Strategies
Identifying when to implement backpressure strategies involves understanding the flow of data in your application:
- High Data Volume: If your application handles large streams of data, consider using Project Reactor or RxJava for robust backpressure support.
- Asynchronous Tasks: When tasks cannot be processed synchronously, buffering or throttling can be effective in managing load.
- Limited Resources: If your consumer has limited processing capabilities (e.g., in mobile applications), you'll need to use backpressure mechanisms to avoid crashes and slowdowns.
Key Takeaways
Managing backpressure is vital for building efficient Java applications that can handle streaming data without performance hitches. Techniques like utilizing reactive frameworks, buffering, and throttling are just the beginning. As you explore and implement these strategies, you’ll find that they not only enhance the robustness of your applications but also improve user experience significantly.
For a deeper understanding of similar concepts in other programming languages, particularly Node.js, be sure to check out Conquering Backpressure in Node.js Streams: A Guide.
Through thoughtful design and implementation of backpressure management strategies, you can optimize your Java Streams for peak performance and reliability. Embrace these techniques to create seamless data processing pipelines that remain responsive, efficient, and capable of handling the challenges of modern applications.