Mastering Backpressure Management in Java Stream API
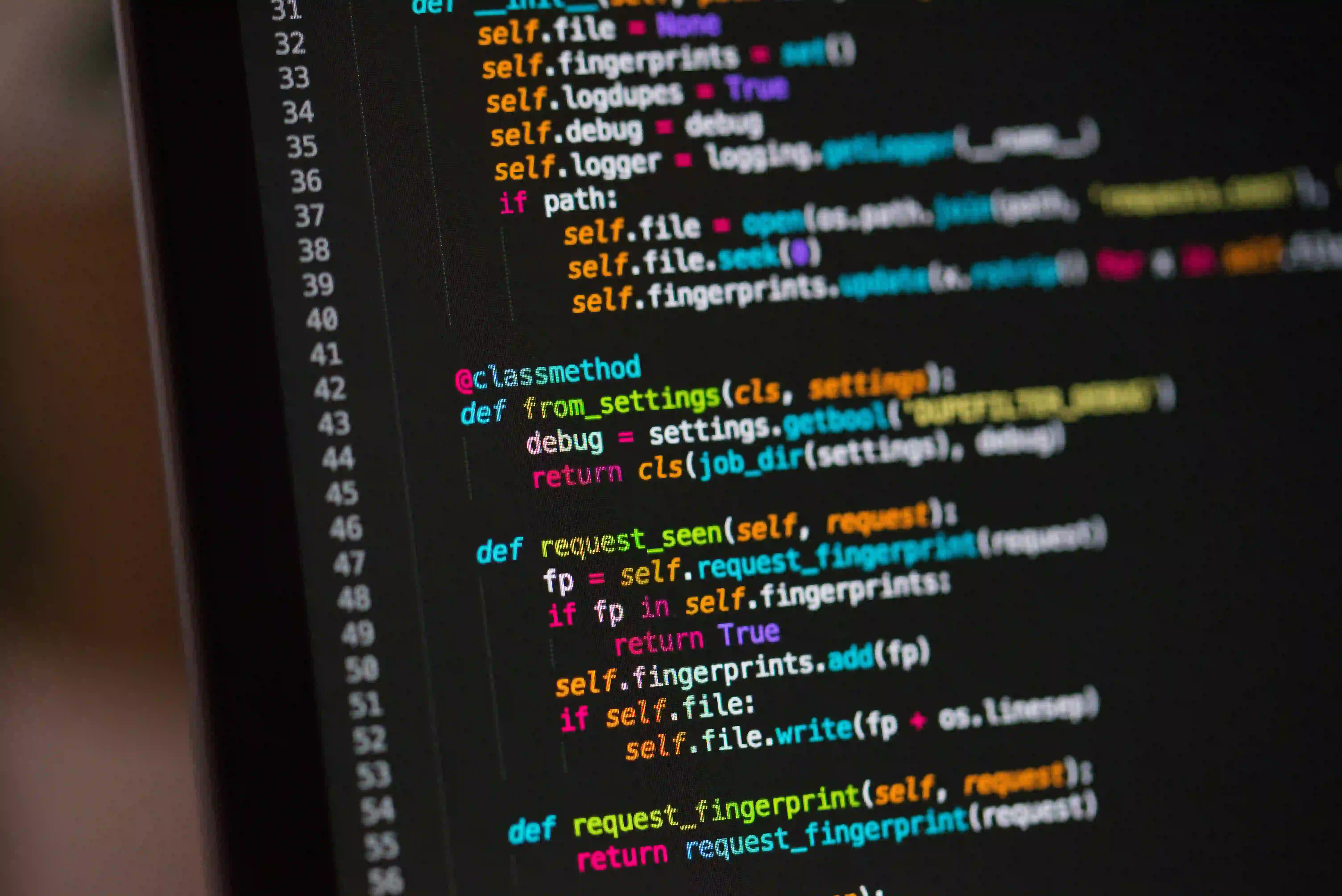
Mastering Backpressure Management in Java Stream API
In the world of data processing, efficient and effective handling of backpressure is vital for ensuring system reliability. While many developers might be familiar with Node.js and its streams, understanding how to manage backpressure in Java is equally crucial, especially as the complexity of systems grows. This blog post delves into the inner workings of the Java Stream API, exploring how backpressure comes into play when using streams for data processing.
Understanding Backpressure
Backpressure refers to a situation in stream processing where the producer of data is sending information faster than the consumer can process it. This discrepancy can lead to problems such as memory overflows, data loss, and system degradation. Backpressure mechanisms allow consumers to signal to producers to slow down or temporarily pause the flow of data, thus maintaining system stability.
In Java, the Stream API provides a means of processing sequences of elements, either in a sequential or parallel manner. However, it lacks native support for backpressure handling, which adds a layer of complexity to developers accustomed to frameworks that offer this feature, such as Node.js.
To get a better sense of managing backpressure in Node.js, it’s helpful to consult the article titled Conquering Backpressure in Node.js Streams: A Guide.
The Java Stream API: A Primer
Before diving into backpressure management, let's briefly cover the basics of the Java Stream API.
A stream represents a sequence of elements supporting sequential and parallel aggregate operations. You can obtain a stream from various data sources, such as collections, arrays, or I/O channels.
Here’s a simple example of using a Java stream to process a list of integers:
import java.util.Arrays;
import java.util.List;
public class StreamExample {
public static void main(String[] args) {
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5);
// Processing the stream
numbers.stream()
.filter(n -> n % 2 == 0)
.map(n -> n * n)
.forEach(System.out::println); // Prints the square of even numbers
}
}
Why this code?
This code snippet demonstrates how to create a stream from a list of integers, filtering the even numbers, squaring them, and printing the results. Each operation is executed in a lazy manner until the terminal operation forEach()
is called, making it essential for performance and clarity.
Handling Backpressure in Java
Though the Stream API does not offer direct support for backpressure, there are strategies you can employ to manage it effectively. Let’s explore them.
1. Use of CompletableFuture for Asynchronous Processing
By leveraging CompletableFuture
, you can manage the flow of data more gracefully. You can combine this with throttling to prevent overwhelming the consumer.
Here's an example of how you might do this:
import java.util.concurrent.CompletableFuture;
public class BackpressureExample {
public static void main(String[] args) {
Producer producer = new Producer();
Consumer consumer = new Consumer();
// Start producer and consumer threads
CompletableFuture.runAsync(producer::produce);
CompletableFuture.runAsync(consumer::consume);
}
}
class Producer {
public void produce() {
for (int i = 0; i < 10; i++) {
System.out.println("Produced: " + i);
try {
Thread.sleep(100); // Simulating variable production rate
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
}
}
}
}
class Consumer {
public void consume() {
for (int i = 0; i < 10; i++) {
System.out.println("Consumed: " + i);
try {
Thread.sleep(200); // Simulating slower consumption
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
}
}
}
}
Why this code?
In this example, the producer and consumer are run in separate threads with simulated delays. The producer creates items more quickly than the consumer can handle. This simple design showcases a basic asynchronous model, which can control backpressure through sleep intervals.
2. Adopt a Buffering Strategy
Buffering is another technique to manage backpressure effectively. It allows the consumer to process items at its speed.
Here’s how a buffering mechanism can be implemented:
import java.util.LinkedList;
import java.util.Queue;
public class BufferExample {
private final Queue<Integer> buffer = new LinkedList<>();
private final int limit = 5;
public synchronized void produce(int number) throws InterruptedException {
while (buffer.size() == limit) {
wait(); // Wait if the buffer is full
}
buffer.offer(number);
notifyAll(); // Notify consumption
}
public synchronized int consume() throws InterruptedException {
while (buffer.isEmpty()) {
wait(); // Wait if the buffer is empty
}
int number = buffer.poll();
notifyAll(); // Notify production
return number;
}
public static void main(String[] args) {
BufferExample buffer = new BufferExample();
new Thread(() -> {
try {
for (int i = 0; i < 10; i++) {
System.out.println("Producing: " + i);
buffer.produce(i);
}
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
}
}).start();
new Thread(() -> {
try {
for (int i = 0; i < 10; i++) {
int number = buffer.consume();
System.out.println("Consuming: " + number);
}
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
}
}).start();
}
}
Why this code?
In this example, we implement a simple buffer using a Queue
. The producer waits when the buffer is full, while the consumer waits when the buffer is empty. This approach effectively manages input and output flows, creating a controlled environment for data processing.
Additional Strategies for Backpressure Management
While we have covered asynchronous processing and buffering, several other strategies exist:
- Throttling API Calls: Implement rate limiting on producers or API calls to ensure data flows smoothly without overwhelming the consumer.
- Reactive Programming Libraries: Explore libraries like Reactor or RxJava that offer built-in backpressure management.
- Asynchronous Libraries: Use asynchronous libraries alongside the Stream API to manage heavy workloads effectively.
Final Considerations
While the Java Stream API does not directly address backpressure, incorporating a variety of strategies can help ensure responsive and stable applications. From using CompletableFuture
for asynchronous operations to implementing buffering techniques, developers can navigate the complexities of backpressure effectively.
For further reading and enhancement of your backpressure management skills, consider checking out Conquering Backpressure in Node.js Streams: A Guide.
By understanding these concepts and implementing these strategies, you will be well on your way to mastering the art of backpressure management in Java, leading to more robust and reliable systems. Happy coding!