Mastering Java Async: Handling Multiple Ajax Updates Efficiently
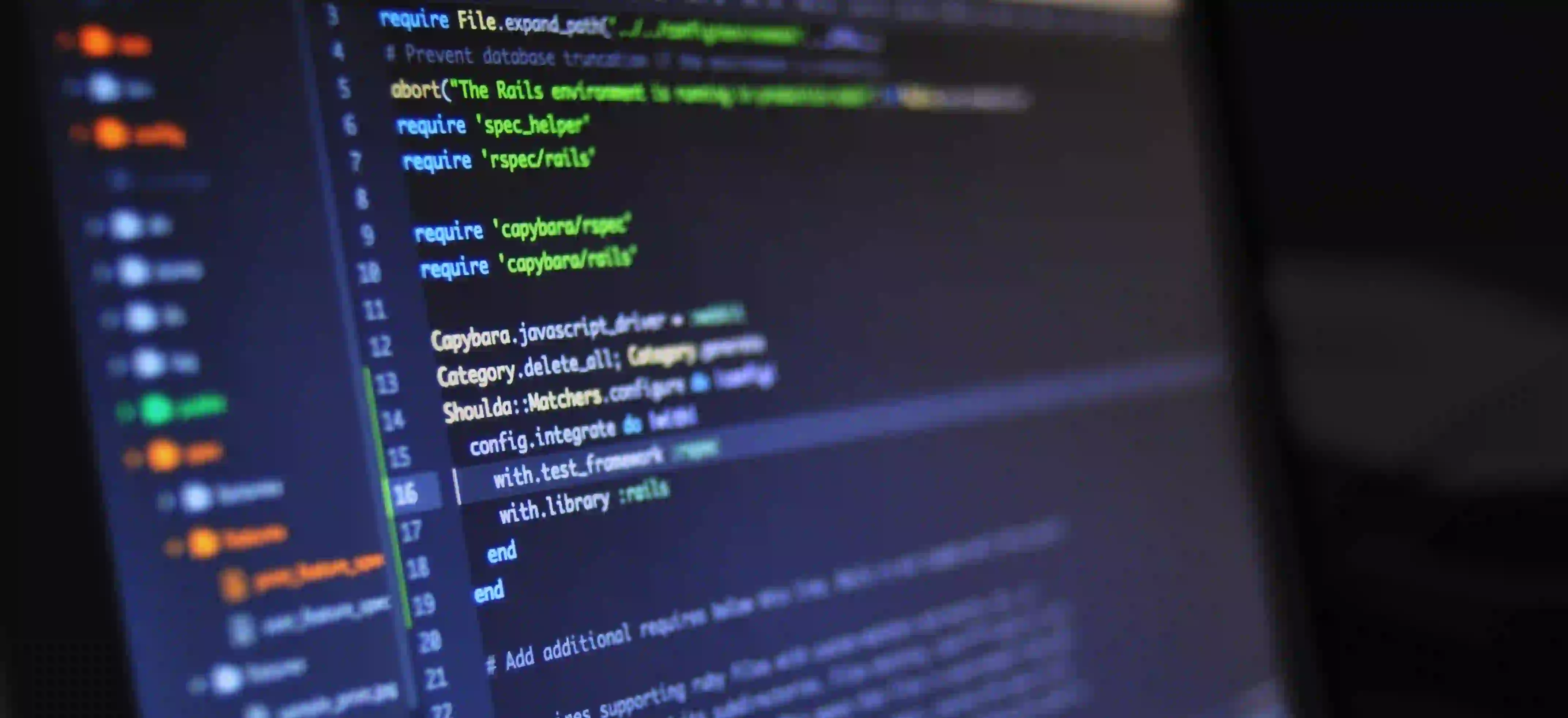
Mastering Java Async: Handling Multiple Ajax Updates Efficiently
In today's dynamic web applications, asynchronous programming plays a crucial role in enhancing user experience. Users expect real-time updates, and accommodating this demand requires efficient handling of AJAX (Asynchronous JavaScript and XML) requests. In this article, we will explore how to handle multiple AJAX updates using Java, alongside its async capabilities, particularly within the context of web applications.
Notably, if you want to dive deeper into AJAX and its behaviors, I recommend checking out the article titled Handling Multiple Ajax Status Updates on Ready State.
Understanding AJAX and its Complexity
AJAX allows web applications to send and retrieve data asynchronously without interfering with the display and behavior of the existing page. This capability is important, especially when making an application responsive to user interaction by updating sections of the webpage.
However, managing multiple simultaneous AJAX requests can become complex due to possible race conditions and the need to ensure that the responses correspond to the requests made. Java provides robust tools and frameworks for managing these challenges.
What is Java Async?
Java Async can be implemented via the CompletableFuture
API introduced in Java 8, facilitating non-blocking I/O operations. This means you can run multiple tasks asynchronously without waiting for each one to finish before starting another.
Setting Up your Java Project
Ensure that you set up your Java development environment correctly. Use an IDE like IntelliJ IDEA or Eclipse, and ensure you have Java SDK installed.
For Maven users, add the following dependency in your pom.xml
:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
This dependency will help you use Spring Boot for creating a web application and will include everything you need to handle their AJAX requests.
Creating an Asynchronous Service
Let’s create a simple asynchronous service that simulates processing multiple AJAX requests. For example, imagine a web application where users can check the status of different tasks via AJAX.
Step 1: Service Class Implementation
First, let’s create a Spring service that simulates asynchronous processing:
import org.springframework.scheduling.annotation.Async;
import org.springframework.stereotype.Service;
import java.util.concurrent.CompletableFuture;
@Service
public class TaskService {
@Async
public CompletableFuture<String> processTask(String taskName) {
try {
// Simulating complex task processing time
Thread.sleep(2000);
return CompletableFuture.completedFuture(taskName + " is completed!");
} catch (InterruptedException e) {
return CompletableFuture.completedFuture(taskName + " encountered an error!");
}
}
}
Explanation
- The
@Async
annotation ensures that this method runs on a separate thread, allowing it to process without blocking other tasks. CompletableFuture
is utilized to represent a future result of an asynchronous computation, making it straightforward to work with the result later.
Step 2: Controller to Handle Requests
Next, we need a controller to manage incoming AJAX requests and invoke our service:
import org.springframework.web.bind.annotation.*;
import java.util.List;
import java.util.stream.Collectors;
import java.util.ArrayList;
@RestController
@RequestMapping("/tasks")
public class TaskController {
private final TaskService taskService;
public TaskController(TaskService taskService) {
this.taskService = taskService;
}
@PostMapping("/start")
public List<String> startTasks(@RequestBody List<String> taskNames) {
List<CompletableFuture<String>> futures = new ArrayList<>();
for (String taskName : taskNames) {
CompletableFuture<String> future = taskService.processTask(taskName);
futures.add(future);
}
// Wait for all tasks to complete and collect the results
return futures.stream()
.map(CompletableFuture::join)
.collect(Collectors.toList());
}
}
Explanation
- This controller accepts a list of task names through a POST request.
- It invokes the
processTask
method on each task name, accumulating the futures in a list. - Finally, it collects the results of all tasks using
CompletableFuture::join
. This approach allows for concurrent execution while easily aggregating the results once they are all complete.
Step 3: AJAX Call from the Client-side
Now, let’s take a look at how you can invoke this API using AJAX in your JavaScript code:
function startTasks() {
const tasks = ["Task1", "Task2", "Task3"];
fetch('/tasks/start', {
method: 'POST',
headers: {
'Content-Type': 'application/json',
},
body: JSON.stringify(tasks)
})
.then(response => response.json())
.then(data => {
console.log(data);
})
.catch(error => {
console.error('Error:', error);
});
}
Explanation
- This snippet makes a
POST
request to the/tasks/start
endpoint with a list of tasks in JSON format. - The
fetch
API allows for chained then calls to handle the asynchronous response, making it easy to handle the output once all tasks are completed.
Exception Handling in Async Operations
Handling exceptions in asynchronous operations is crucial to ensure that your application behaves predictably. You can enhance the processTask
method by using exceptionally:
public CompletableFuture<String> processTask(String taskName) {
return CompletableFuture.supplyAsync(() -> {
try {
Thread.sleep(2000);
return taskName + " is completed!";
} catch (InterruptedException e) {
throw new RuntimeException(taskName + " encountered an error!");
}
}).exceptionally(ex -> {
// Custom exception handling
return "Error: " + ex.getMessage();
});
}
In this example, we’re catching potential errors during task execution and returning an error message to the user in case of a failure.
Key Takeaways
By utilizing Java's asynchronous capabilities, you can efficiently handle multiple AJAX updates simultaneously, manage state more effectively, and provide users with a seamless experience. As illustrated above, combining Java's powerful concurrency tools with efficient design outcomes enhances your applications significantly.
For more insights on improving AJAX interactions, explore the article on Handling Multiple Ajax Status Updates on Ready State.
With consistent updates and proper handling of asynchronous requests, you can not only meet user expectations but exceed them—creating a responsive and robust web application infrastructure capable of supporting numerous tasks without compromising performance.
Embrace Java async and empower your applications to handle the demands of modern-day web interactions effectively. Happy coding!