Mastering Ajax Calls in Java: Handle Multiple Status Updates Efficiently
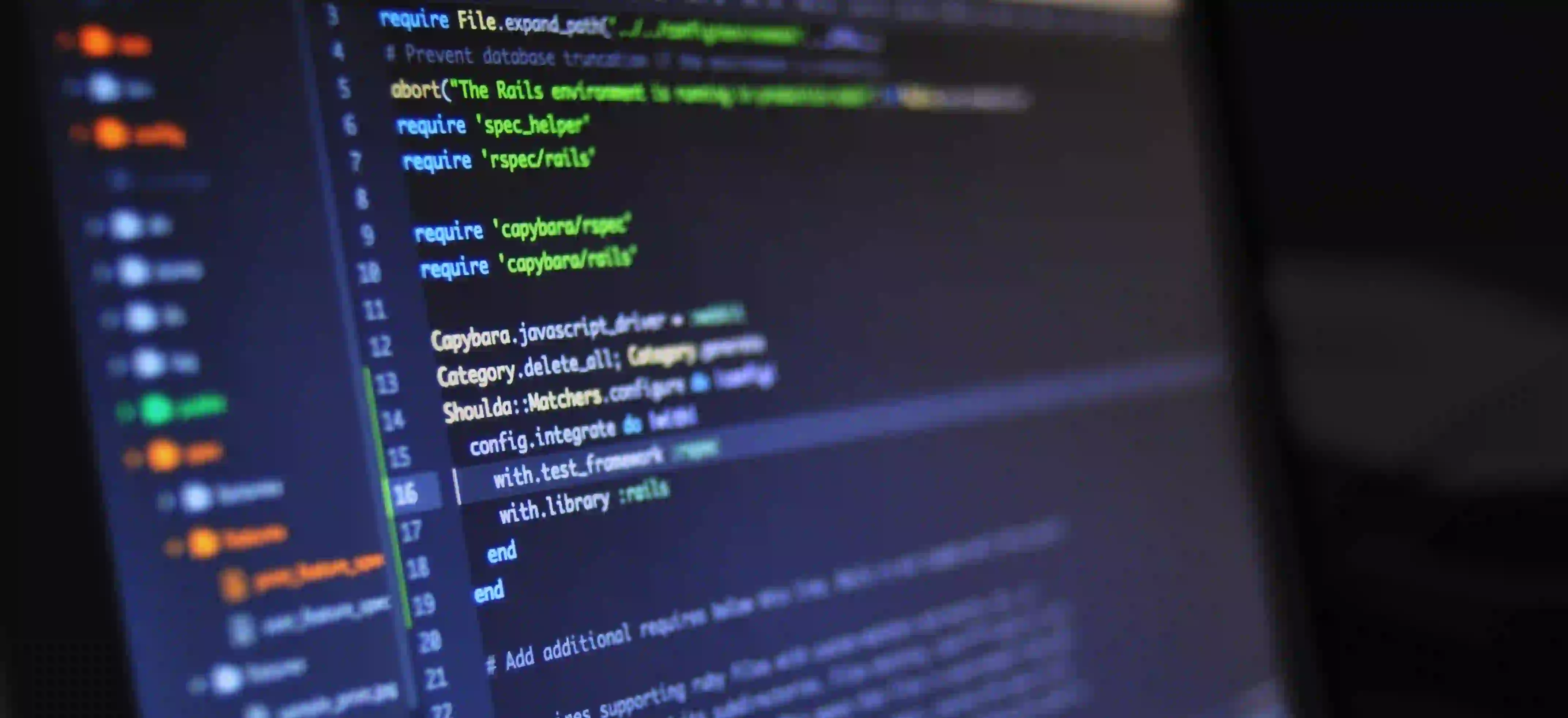
Mastering Ajax Calls in Java: Handle Multiple Status Updates Efficiently
In today's dynamic web environment, creating seamless user experiences often hinges on the effective use of Asynchronous JavaScript and XML (Ajax) calls. As a Java developer, understanding how to handle multiple Ajax status updates efficiently can greatly enhance your applications. This post will explore the intricacies of Ajax, focusing on how to manage multiple updates and ensuring smooth data exchanges without disrupting user experience.
Understanding Ajax and Its Importance
Ajax is a combination of technologies that allows web applications to send and retrieve data asynchronously from a server without interfering with the display and behavior of the existing web page. It leads to faster and more interactive web applications.
Here are key reasons why mastering Ajax is vital:
- Improved User Experience: Ajax allows for partial page updates, leading to a smoother experience.
- Fewer Server Requests: Instead of loading an entire page for minor data updates, Ajax can fetch only the necessary information.
- Increased Performance: By reducing the load on the server, Ajax can significantly speed up response times.
Ajax Calls: A Brief Overview
The core of Ajax functionality lies in the XMLHttpRequest
object. Below is a simple example of making an Ajax call:
// Create a new XMLHttpRequest object
var xhttp = new XMLHttpRequest();
// Define a callback function
xhttp.onreadystatechange = function() {
if (this.readyState == 4 && this.status == 200) {
// Handle the response
console.log(this.responseText);
}
};
// Open and send the request
xhttp.open("GET", "your_url_here", true);
xhttp.send();
Code Commentary
- XMLHttpRequest Object: This object is essential for making Ajax calls. Its
onreadystatechange
property allows you to define a function that's triggered when the ready state of the request changes. - Callback Function: The callback checks two conditions: the
readyState
should be 4 (request completed), and thestatus
should be 200 (OK).
This simple structure can be expanded upon to handle multiple Ajax calls effectively.
Handling Multiple Ajax Status Updates
One of the challenges developers face is managing multiple Ajax calls efficiently. This is crucial, especially when the response from each call could affect the user interface or overall application state.
Promises and Ajax
To tackle the challenges posed by asynchronous programming, JavaScript's Promises were introduced. Promises allow us to cleanly handle asynchronous operations like Ajax calls.
Consider the following code snippet using Promises:
function fetchData(url) {
return new Promise((resolve, reject) => {
const xhttp = new XMLHttpRequest();
xhttp.onreadystatechange = function() {
if (this.readyState == 4) {
if (this.status == 200) {
resolve(JSON.parse(this.responseText));
} else {
reject(this.status);
}
}
};
xhttp.open("GET", url, true);
xhttp.send();
});
}
// Example usage of multiple Ajax calls
Promise.all([fetchData("url1"), fetchData("url2")])
.then(responses => {
// Handle all successful responses here
console.log(responses);
})
.catch(error => {
console.error("Error:", error);
});
Code Commentary
- Promise Constructor: This pattern is used to encapsulate the asynchronous operation.
- Promise.all: This method is a powerful way to handle multiple Ajax calls. It waits for all the promises passed to it to either resolve or reject.
By utilizing Promises, you can handle multiple Ajax operations more gracefully and maintain control over your application flow.
Best Practices for Handling Multiple Ajax Calls
1. Use Async/Await
Async/await syntax can simplify the code when working with multiple Ajax calls. It allows asynchronous code to be written in a way that looks synchronous.
async function fetchDataInSequence() {
try {
const response1 = await fetchData("url1");
const response2 = await fetchData("url2");
console.log(response1, response2);
} catch (error) {
console.error("Error fetching data:", error);
}
}
2. Implement Timeout
In scenarios where Ajax calls take too long, it is beneficial to implement a timeout mechanism to enhance user experience.
function fetchDataWithTimeout(url, timeout = 5000) {
return Promise.race([
fetchData(url),
new Promise((_, reject) => setTimeout(() => reject(new Error("Request timed out")), timeout))
]);
}
3. Handle Errors Gracefully
Always anticipate potential errors, as they can impact user experience. Make error handling user-friendly.
fetchData("some_url")
.then(data => {
console.log("Data received:", data);
})
.catch(error => {
alert("An error occurred while fetching data. Please try again.");
console.error("Error details:", error);
});
Bringing It All Together
Mastering Ajax calls in Java is crucial for creating responsive, efficient, and user-friendly applications. By leveraging modern JavaScript features such as Promises, async/await, and adopting best practices, developers can handle multiple Ajax status updates with ease.
For further insights on handling Ajax calls, check out this article Handling Multiple Ajax Status Updates on Ready State. By understanding these concepts, you're well on your way to ensuring your application delivers a seamless user experience.
Remember, the efficiency of your Ajax calls can significantly impact your application, so invest the time to master them!