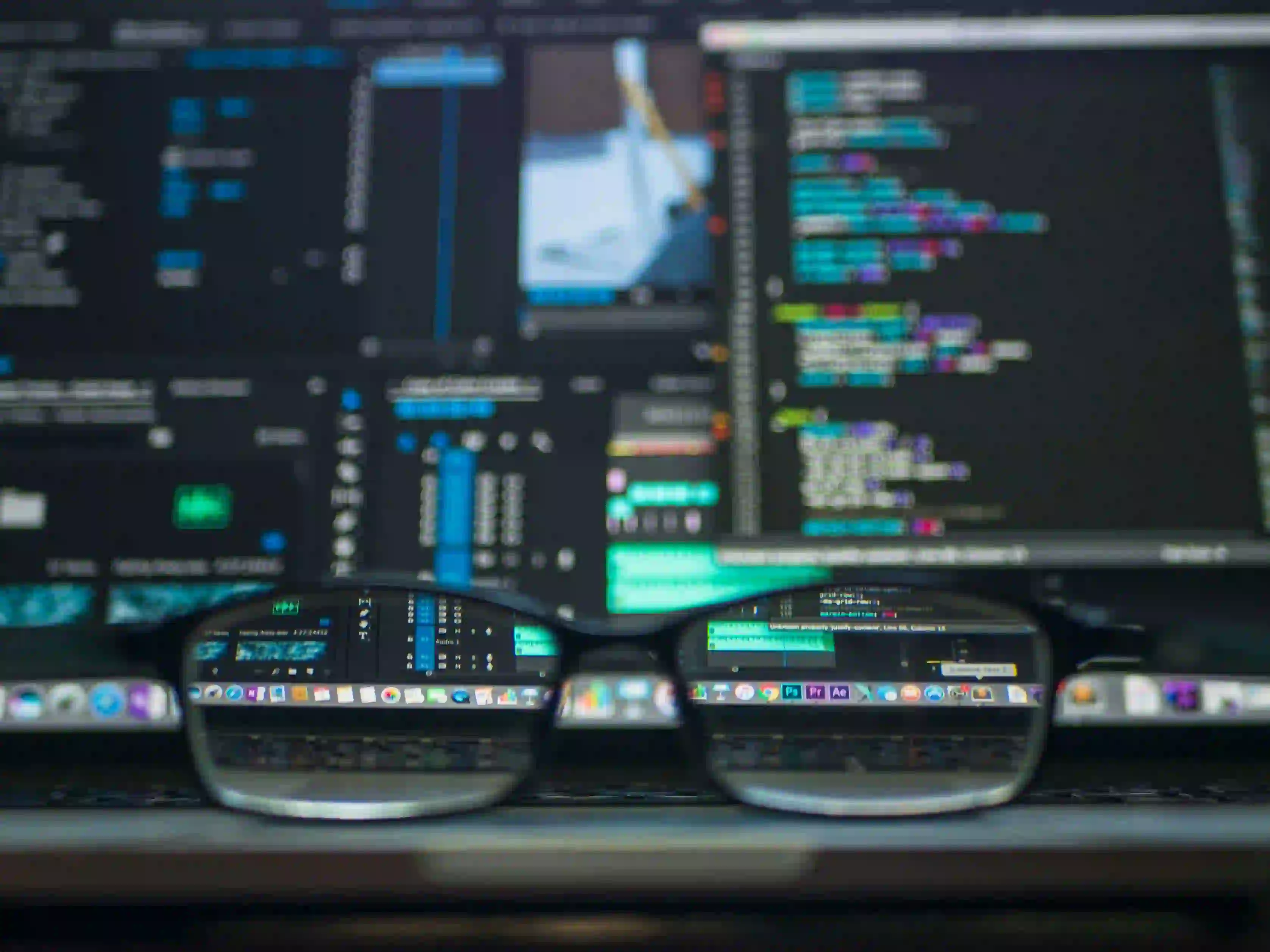
Java Web Development: Avoiding Layout Headaches with CSS
When developing web applications in Java, many developers overlook the importance of a well-structured front-end. While Java primarily handles back-end processes, the user experience is significantly influenced by how the front-end is designed. A common struggle in web development lies in achieving the right layout—especially when integrating CSS for responsive and user-friendly designs.
In this blog post, we'll explore best practices for using CSS in your Java web applications to avoid layout headaches. We'll cover essential concepts, provide examples, and even touch on common pitfalls that many developers encounter, linking to a fantastic resource for troubleshooting those issues.
Understanding the Role of CSS in Java Web Applications
CSS (Cascading Style Sheets) controls the presentation aspect of your web applications. It allows you to craft visually appealing layouts, and it's crucial to have a solid grasp of CSS, especially when integrated with Java frameworks like Spring MVC or JavaServer Faces (JSF).
Code Organization Matters
Separating CSS into different files can help maintain clarity. For instance, consider the following directory structure:
/src
/main
/java
/com
/example
/controller
/model
/service
/resources
/static
/css
styles.css
Here, having a dedicated folder for CSS makes it easier to manage styles. Within styles.css
, you can write rules that your Java web application will reference.
Example CSS File
Here’s a simple example of a CSS rule that you might include in your styles.css
file:
body {
font-family: Arial, sans-serif;
margin: 0;
padding: 0;
background-color: #f4f4f4;
}
.container {
width: 80%;
margin: 0 auto;
display: flex;
flex-direction: column;
align-items: center;
}
Why Use This Code? This layout gives your application a clean look by using a modern font and a comfortable margin. The flexbox property allows for responsive designs since it adapts automatically to varying screen sizes.
Responsive Design Tools
Using CSS frameworks like Bootstrap or Tailwind CSS can streamline your design process. Bootstrap, for instance, comes equipped with a responsive grid system. To integrate it with your Java application:
-
Include the Bootstrap CSS in your HTML head tag.
📄snippet.txt<link rel="stylesheet" href="https://stackpath.bootstrapcdn.com/bootstrap/4.5.2/css/bootstrap.min.css">
-
Utilize the Bootstrap classes in your HTML elements.
📄snippet.txt<div class="container"> <div class="row"> <div class="col-md-6">Column 1</div> <div class="col-md-6">Column 2</div> </div> </div>
Why Bootstrap? Implementing Bootstrap enables you to focus on functionality without getting bogged down by layout issues extensive styling. It ensures consistency across various devices.
Common CSS Pitfalls
Despite its benefits, CSS comes with challenges. One prevalent issue developers face is creating layouts that don’t behave as expected. A profound exploration of these problems can be found in the article Troubleshooting Sticky CSS: Common Pitfalls Explained. Notably, sticky positioning often trips developers up—leading to elements that do not stay fixed as intended.
Fixed vs. Sticky Positioning
For instance, you may want a navigation bar that remains on top while scrolling. Here’s how you can implement it:
.navbar {
position: sticky;
top: 0;
background-color: white;
z-index: 1000;
}
.content {
height: 2000px; /* Create height for scrolling */
}
Why This Code? The position: sticky
property allows the navbar to stick to the top of the viewport when scrolling. It’s essential to specify the top
property; otherwise, it won’t activate.
This implementation showcases how with proper understanding, sticky positioning can indeed work effectively to enhance your site's navigational ease.
Best Practices for CSS Management
To maintain a smooth experience across your application, consider the following best practices for CSS management:
-
Use Classes Instead of IDs: Classes are reusable and keep your styles organized.
-
Modular CSS: Break CSS into small, manageable components. Each component should encapsulate its styles.
-
Avoid Inline Styles: They can clutter your HTML and make it less readable.
-
Consistency in Units: Stick to either
px
,em
, or%
to maintain design consistency. -
Version Control: Maintain your CSS versions. Managed evolution minimizes unexpected layout shifts.
Real-World Application Example
Integrating CSS effectively into your Java web application makes a substantial difference in user experience. Here’s a mini-application showcasing a simple user registration form styled with CSS.
Sample HTML File with CSS
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>User Registration</title>
<link rel="stylesheet" href="css/styles.css">
</head>
<body>
<div class="container">
<h1>Register</h1>
<form>
<label for="username">Username:</label>
<input type="text" id="username" name="username" required>
<label for="password">Password:</label>
<input type="password" id="password" name="password" required>
<button type="submit">Register</button>
</form>
</div>
</body>
</html>
Explanation of CSS Updates
Your styles.css
might look something like this:
.container {
width: 300px;
margin: auto;
}
h1 {
text-align: center;
}
form {
display: flex;
flex-direction: column;
}
label {
margin-bottom: 5px;
}
input {
margin-bottom: 15px;
padding: 8px;
border: 1px solid #dddddd;
border-radius: 4px;
}
button {
padding: 10px;
background-color: #28a745;
color: white;
border: none;
border-radius: 4px;
cursor: pointer;
}
Why This Structure? This structure separates responsibilities—HTML handles the content while CSS creates the style. The input styles enhance usability, making the form visually appealing to users.
Key Takeaways
Achieving a seamless integration between Java back-end and CSS front-end requires understanding both paradigms. Developers should prioritize organization and modular CSS practices to mitigate common layout problems. Moreover, resources like Troubleshooting Sticky CSS: Common Pitfalls Explained can guide you through resolving specific issues that arise during development.
By applying these principles and practices to your Java web development endeavors, you’ll reduce layout headaches and build applications that are both functional and visually pleasing. Embrace CSS's power to unlock a world of design possibilities for your Java applications.
For further reading, explore resources on advanced CSS techniques and frameworks that complement Java technologies. Each step you take enhances your skills and efficiency as a developer. Happy coding!