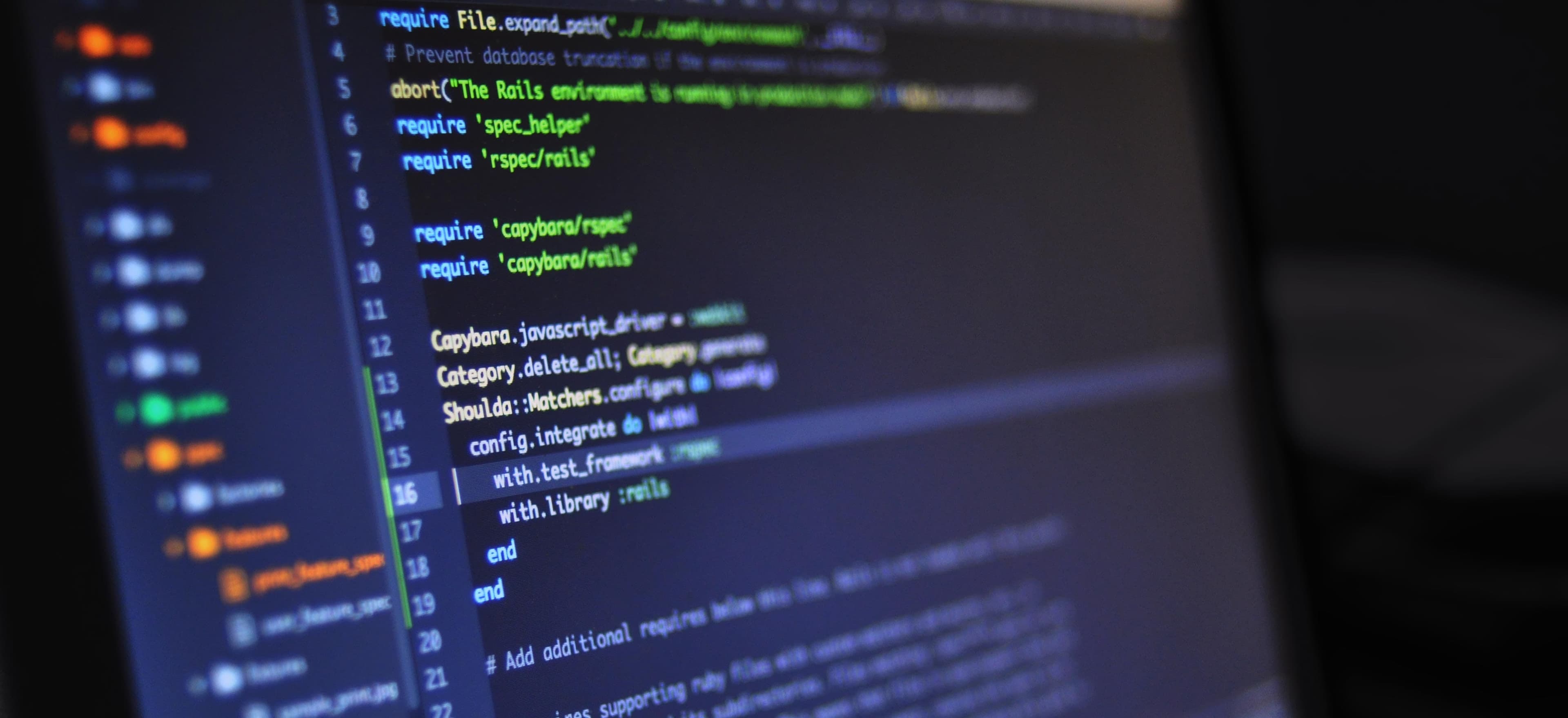
- Published on
Solving CSS Positioning Issues in Java-Based Web Apps
When developing web applications with Java, one can easily become engrossed in the complexities of server-side logic and database interactions. However, the importance of a seamless user interface cannot be understated. Problems with CSS positioning can lead to frustrating user experiences, particularly when it comes to Java-based web applications that utilize frameworks like Spring MVC or JavaServer Faces (JSF). In this blog post, we will explore common CSS positioning issues and how to solve them effectively.
Understanding CSS Positioning
CSS positioning is the way elements are arranged on a page. There are several positioning strategies in CSS, including static, relative, absolute, fixed, and sticky. Understanding how each positioning type works is crucial for creating responsive designs.
-
Static Positioning: This is the default. Elements stack normally, following the document flow.
-
Relative Positioning: The element is positioned relative to its normal position. It's useful for nudging elements.
-
Absolute Positioning: The element is removed from the document flow and positioned based on the nearest positioned ancestor (any ancestor with a position other than static).
-
Fixed Positioning: The element is positioned relative to the viewport and remains in a fixed position even when scrolling.
-
Sticky Positioning: This is a hybrid between relative and fixed positioning. Elements behave like relative until the viewport hits a defined position, where they then stick and become fixed.
Understanding these concepts is fundamental, and if you encounter issues with sticky elements specifically, you might want to check out the article "Troubleshooting Sticky CSS: Common Pitfalls Explained" at tech-snags.com/articles/troubleshooting-sticky-css-pitfalls.
Common CSS Positioning Issues in Java-Based Web Apps
1. Overlapping Elements
Overlapping elements often occur with absolute or fixed positioning. Here’s a simple example of how to create a modal window, which frequently leads to overlapping issues:
.modal {
position: fixed; // Ensures the modal stays on the screen
top: 50%; // Centers it vertically
left: 50%; // Centers it horizontally
transform: translate(-50%, -50%); // Adjusts for the width and height
z-index: 1000; // Places modal above other elements
display: none; // Hidden by default
}
Why This Code Works: The position: fixed
ensures the modal is always visible in the viewport, irrespective of scroll. The transform
property allows proper centering without needing to calculate the dimensions of the modal dynamically.
2. The Sticky Art of Positioning
Sticky positioning can be tricky. A frequent problem arises when the parent container does not have a defined height, causing sticky elements to fail at adhering to their position.
.header {
position: sticky; // Makes it sticky
top: 0; // Starts sticking at the top of the viewport
background-color: #fff; // Ensure visibility
z-index: 100; // Stays on top of other content
}
Commentary: Ensure that the parent of .header
has a defined height. If the parent is collapsing (i.e., it has no children or all children are floated), the sticky positioning will not work as expected.
.parent {
height: 100vh; // Prevents the parent from collapsing
}
3. The Issue of Responsive Design
With varying screen sizes, ensuring proper positioning can be a challenge. Using media queries becomes essential.
@media only screen and (max-width: 600px) {
.sidebar {
position: relative; // Resets to allow normal flows on small screens
display: none; // Hides sidebar for better mobile experience
}
}
Explanation: By changing the position to relative on smaller screens, we allow elements to flow naturally without fixed placements that could obscure content.
4. Debugging Techniques
When dealing with CSS issues, debugging is crucial. Here are a few methods you can employ:
-
Browser Developer Tools: Use inspector tools to view real-time changes, test CSS rules, and view the box model for your elements.
-
Avoid Over-reliance on Frameworks: Sometimes, CSS frameworks add their own styles (Bootstrap, for example) that conflict with your positioning. Always check for overrides.
-
Use Clear Specifications: Test your layout under various screen sizes and resolutions to ensure that your CSS works consistently.
5. Advanced Techniques
Sometimes you'll need to use JavaScript in conjunction with CSS to achieve the desired behavior. For example, adjusting a layout based on scroll:
window.addEventListener('scroll', function() {
const header = document.querySelector('.header');
if (window.scrollY > 100) {
header.style.position = 'fixed'; // Fix header on scroll
} else {
header.style.position = 'static'; // Revert to default
}
});
Why Use JavaScript: CSS alone may not always provide the needed flexibility, especially with dynamic layouts where conditions change based on user interaction.
To Wrap Things Up
CSS positioning can indeed feel overwhelming, especially in Java-based web applications where server-side and client-side code interconnect. However, understanding the basics of CSS and how to manipulate positions can create a seamless experience for users.
Remember to validate your designs through consistent testing, and don’t hesitate to employ JavaScript as your needs evolve. For those facing sticky CSS issues specifically, it’s useful to take a look at existing discussions on the topic, such as those found in “Troubleshooting Sticky CSS: Common Pitfalls Explained” (tech-snags.com/articles/troubleshooting-sticky-css-pitfalls).
By developing a solid grasp of CSS positioning, you can ensure your Java web applications not only function well at their core but also provide an engaging user interface that enhances the overall experience. Happy coding!
Checkout our other articles