Reducing Java App Costs: Strategies for Enterprise Efficiency
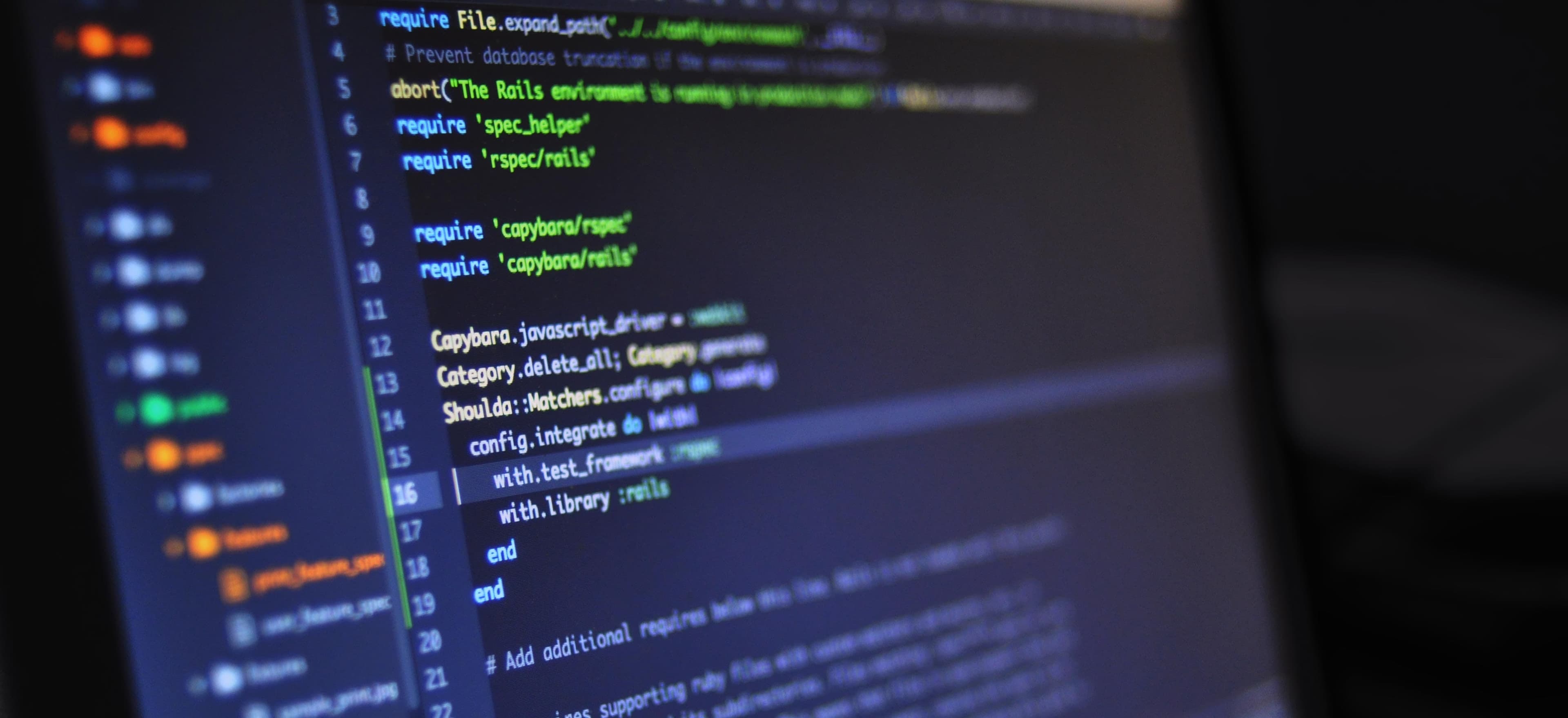
- Published on
Reducing Java App Costs: Strategies for Enterprise Efficiency
In today's competitive landscape, businesses are increasingly focused on optimizing their operations to maintain profitability. This is particularly true for enterprise applications, where substantial resources—both financial and human—can be tied up in software development and maintenance. If you're running Java applications, the good news is that there are practical strategies to reduce costs while increasing efficiency.
In this post, we will explore various techniques to enhance the performance of your Java applications, streamline processes, and ultimately cut costs. We'll also provide sample code snippets to help identify potential cost-saving opportunities. For a broader perspective on managing operational expenses, refer to the insightful article "Cutting Costs: Navigating High Expenses in Enterprise Tech" at configzen.com/blog/navigating-high-expenses-in-enterprise-tech.
1. Optimize Resource Management
Understanding Resource Allocation
One of the leading causes of inefficiency in Java applications is improper resource management. Unused resources can lead directly to escalated operational costs. Java provides garbage collection, which helps reclaim memory, but developers need to implement coding best practices to minimize leftover resources.
Code Example: Proper Resource Handling
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
public class EmployeeDatabase {
private static final String DB_URL = "jdbc:mysql://localhost:3306/employees";
private static final String USER = "root";
private static final String PASS = "password";
public void fetchEmployees() {
Connection conn = null;
PreparedStatement stmt = null;
try {
conn = DriverManager.getConnection(DB_URL, USER, PASS);
stmt = conn.prepareStatement("SELECT * FROM employee");
ResultSet rs = stmt.executeQuery();
while (rs.next()) {
// Retrieve employee details
System.out.println("Employee: " + rs.getString("name"));
}
} catch (Exception e) {
e.printStackTrace();
} finally {
try {
if (stmt != null) stmt.close();
if (conn != null) conn.close();
} catch (Exception e) {
e.printStackTrace();
}
}
}
}
Explanation
In the above code, we manage the JDBC connection and prepared statement properly—both are closed in the finally
block. This ensures that resources are always released, preventing memory leaks and reducing overhead. Efficient resource management not only decreases operational costs but also improves application performance.
2. Embrace Microservices Architecture
Why Microservices?
Switching to a microservices architecture can significantly cut costs related to deployment and scaling. Instead of managing a monolithic application, microservices allow independent development and scaling of components. This leads to using resources only where they are needed, making the overall system more efficient.
Benefits of Microservices:
- Scalability: Scale only the parts of your application that require additional resources.
- Fault Isolation: An issue in one microservice does not affect the entire system.
- Optimized Resource Usage: Spend less on infrastructure by running only the services you need.
3. Streamline Deployment with Containerization
The Power of Docker
Containerization can lead to reduced cloud costs by streamlining application deployment. With Docker, you can package your Java applications and their dependencies into containers that can be deployed anywhere. This minimizes the discrepancies between environments and reduces testing time.
Quick Example: Dockerfile for a Java App
# Use a base Java runtime image
FROM openjdk:11-jre-slim
# Set the working directory
WORKDIR /app
# Copy the Java application JAR file
COPY target/myapp.jar myapp.jar
# Command to run the application
CMD ["java", "-jar", "myapp.jar"]
Explanation
This Dockerfile showcases how to create a lightweight image for a Java application. Using a minimal JRE reduces the total image size, aiding in quicker downloads and startup times. Efficient deployment reduces the necessary infrastructure, helping you save on cloud expenses.
4. Implement Caching Mechanisms
Less is More: Caching
Reducing repetitive data requests can lead to significant performance enhancements and reduced costs. Implementing caching mechanisms to store frequently accessed data minimizes database load and improves response times.
Code Example: Simple Caching Using a HashMap
import java.util.HashMap;
public class DataCache {
private HashMap<String, String> cache = new HashMap<>();
public String getData(String key) {
if (cache.containsKey(key)) {
return cache.get(key); // Cache hit
}
// Simulate data fetch
String value = fetchDataFromDatabase(key);
cache.put(key, value); // Cache miss
return value;
}
private String fetchDataFromDatabase(String key) {
// Simulated database fetch
return "Data for " + key;
}
}
Explanation
In the DataCache
class, we use a HashMap to store data temporarily. When a request comes in, we first check the cache before attempting to fetch from the database. This approach significantly reduces database calls, leading to decreased costs and improved performance.
5. Enhance Code Efficiency
Refactoring for Performance
Another way to reduce costs is to improve the efficiency of your existing code. The less time your Java application spends processing requests, the lower the costs associated with compute resources.
Code Example: Refactoring a Loop
import java.util.Arrays;
import java.util.List;
public class CodeEfficiency {
public long sumOfSquares(List<Integer> numbers) {
return numbers.stream().mapToInt(i -> i * i).sum();
}
public static void main(String[] args) {
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5);
CodeEfficiency ce = new CodeEfficiency();
System.out.println("Sum of squares: " + ce.sumOfSquares(numbers));
}
}
Explanation
In this code snippet, we use Java Streams to calculate the sum of squares. The mapToInt
method applies a function to each element efficiently, resulting in cleaner and more maintainable code. Refactoring can lead to substantial runtime improvements, conserving computational resources.
To Wrap Things Up
By employing thoughtful strategies like resource management, switching to microservices, containerization, caching, and code refactoring, enterprises can significantly reduce costs associated with Java applications. Not only do these methods allow for monetary savings, but they also foster more efficient operations—key to survival in today's fast-paced business world.
To dive deeper into enterprise cost management, check out the article "Cutting Costs: Navigating High Expenses in Enterprise Tech" at configzen.com/blog/navigating-high-expenses-in-enterprise-tech. By refining both your strategies and your Java code, you can create a more nimble environment that adapts quickly to changing demands while keeping expenses in check.
Checkout our other articles