Cost-Effective Strategies for Java Frameworks in Enterprises
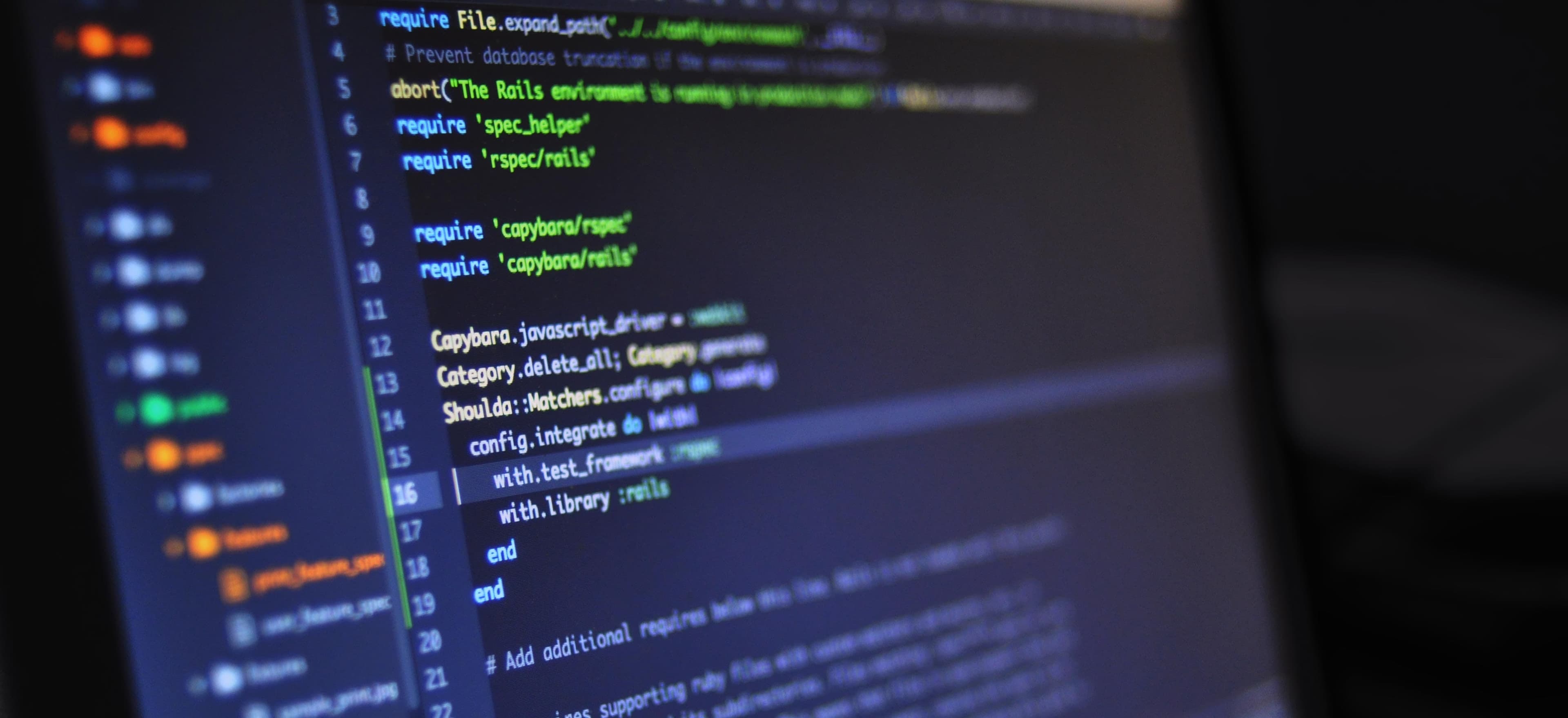
- Published on
Cost-Effective Strategies for Java Frameworks in Enterprises
As businesses increasingly rely on technology to drive growth, the discussion surrounding cost-effectiveness becomes crucial, especially when it comes to adopting frameworks in enterprise environments. While choosing Java as the programming language is often a no-brainer due to its robustness, platform independence, and vast ecosystem, the decision to select the right framework can significantly impact both costs and productivity. In this article, we’ll explore some cost-effective strategies for deploying Java frameworks in enterprises.
Why Choose Java Frameworks?
Java frameworks can accelerate the development process significantly. By utilizing predefined templates and code structures, developers can focus on building applications rather than starting from scratch. Popular Java frameworks such as Spring, Hibernate, and JavaServer Faces (JSF) offer various features, including dependency injection, data access, and user interface management.
A successful choice of a Java framework can lead to:
- Increased productivity: Developers can reuse code, which speeds up development time.
- Improved maintainability: A well-structured codebase is easier to update and debug.
- Supportive community: Established frameworks typically have a vibrant community, providing resources, plugins, and forums for support.
Understanding Your Requirements
Before diving into frameworks, businesses should clearly outline their requirements. This will prevent over-engineering and facilitate a focused approach towards framework selection. Consider the following questions:
- What kind of application are you building? (Web, enterprise, mobile)
- What is your project's scale?
- What is the skill level of your developers?
Example: Simple Requirement Analysis
public class RequirementAnalysis {
String applicationType;
int scale;
int developerExperienceLevel;
public RequirementAnalysis(String applicationType, int scale, int developerExperienceLevel) {
this.applicationType = applicationType;
this.scale = scale;
this.developerExperienceLevel = developerExperienceLevel;
}
public void analyze() {
if (applicationType.equalsIgnoreCase("web") && scale > 1000) {
System.out.println("Consider using Spring Boot for scalability.");
} else if (developerExperienceLevel < 2) {
System.out.println("A simpler framework like JSF might be more suitable.");
}
}
}
Here, the RequirementAnalysis
class helps evaluate which framework might suit your needs based on application type, scale, and developer experience. Analyzing requirements helps in choosing a framework that is aligned with your organizational goals, thus avoiding unnecessary expenses.
Choosing the Right Framework
Once you have clarity on your requirements, the next step is selecting the right Java framework.
Spring Framework
Spring is known for its flexibility and vast capabilities. Its modular nature allows developers to use only what they need, minimizing resource expenditure.
Key Features:
- Dependency Injection: Reduces boilerplate code, which focuses on business logic.
- Spring Boot: Makes it easy to create stand-alone applications.
Why Use Spring Boot?
Let's look at how to initialize a simple Spring Boot application:
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
public class MyApplication {
public static void main(String[] args) {
SpringApplication.run(MyApplication.class, args);
}
}
This straightforward code initializes a Spring Boot application. By enabling autoconfiguration, developers can reduce the time typically spent on setup.
Hibernate
When dealing with data persistence, Hibernate stands out. It removes the burden of manual data handling and reduces error rates.
Features:
- Object-Relational Mapping (ORM): Streamlines database interactions.
- Caching: Improves performance by reducing database calls.
Here's a basic Hibernate entity:
import javax.persistence.Entity;
import javax.persistence.GeneratedValue;
import javax.persistence.GenerationType;
import javax.persistence.Id;
@Entity
public class User {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String name;
// Getters and Setters
}
The User
class illustrates Hibernate's paradigm of keeping data in objects and directly mapping these to database tables. This reduces manual SQL queries and enhances maintainability.
Building a Cost-Effective Development Environment
Leverage Cloud Services
Integrating cloud-native solutions can greatly reduce infrastructure costs. Tools like AWS and Google Cloud Platform offer scalable databases and serverless solutions that save money on unused resources.
Continuous Integration/Continuous Deployment (CI/CD)
Employ automation frameworks such as Jenkins or GitLab CI to streamline your development process. Automated testing and deployment reduce human error and speed up delivery times, ultimately translating to lower project costs.
Simple CI/CD Pipeline Example:
pipeline {
agent any
stages {
stage('Build') {
steps {
sh 'mvn clean package'
}
}
stage('Deploy') {
steps {
sh 'deploy.sh'
}
}
}
}
In this Jenkins pipeline example, the project is built with Maven and deployed in a single workflow, thus ensuring consistency and reliability.
Empowering Your Team Through Training
Invest in your developers. Offering training on chosen frameworks can yield remarkable cost savings in the long run. Skilled developers can leverage frameworks effectively, reducing errors and increasing delivery speed.
- Online courses on platforms like Coursera and Udemy offer flexible training options.
- In-house workshops can be tailored to your organization's needs, enabling direct application of learned skills.
Monitor and Optimize Usage
Finally, keep an eye on resource utilization. Use profiling tools such as VisualVM or JProfiler to understand memory usage and performance bottlenecks. By continually optimizing your application, you can keep operational costs down.
Example Profiling Code
public void traceMethodExecution() {
long startTime = System.currentTimeMillis();
// method execution
long endTime = System.currentTimeMillis();
System.out.println("Execution time: " + (endTime - startTime) + " milliseconds");
}
This method tracks how long particular blocks of code take to execute. Identifying time-consuming parts can direct you to areas needing optimization.
The Last Word
Navigating the complexities of enterprise technology requires a delicate balance between functionality and cost. By understanding your requirements, selecting appropriate frameworks, and implementing best practices, you can significantly reduce expenses while still embracing the power of Java.
If you're interested in strategies for cutting costs in enterprise tech, consider reading Cutting Costs: Navigating High Expenses in Enterprise Tech for insights that may complement your Java-focused strategies.
In summary, the right approach to utilizing Java frameworks can enhance productivity, streamline costs, and ultimately lead to successful project outcomes.
Checkout our other articles