Streamlining Java Applications to Reduce Enterprise Costs
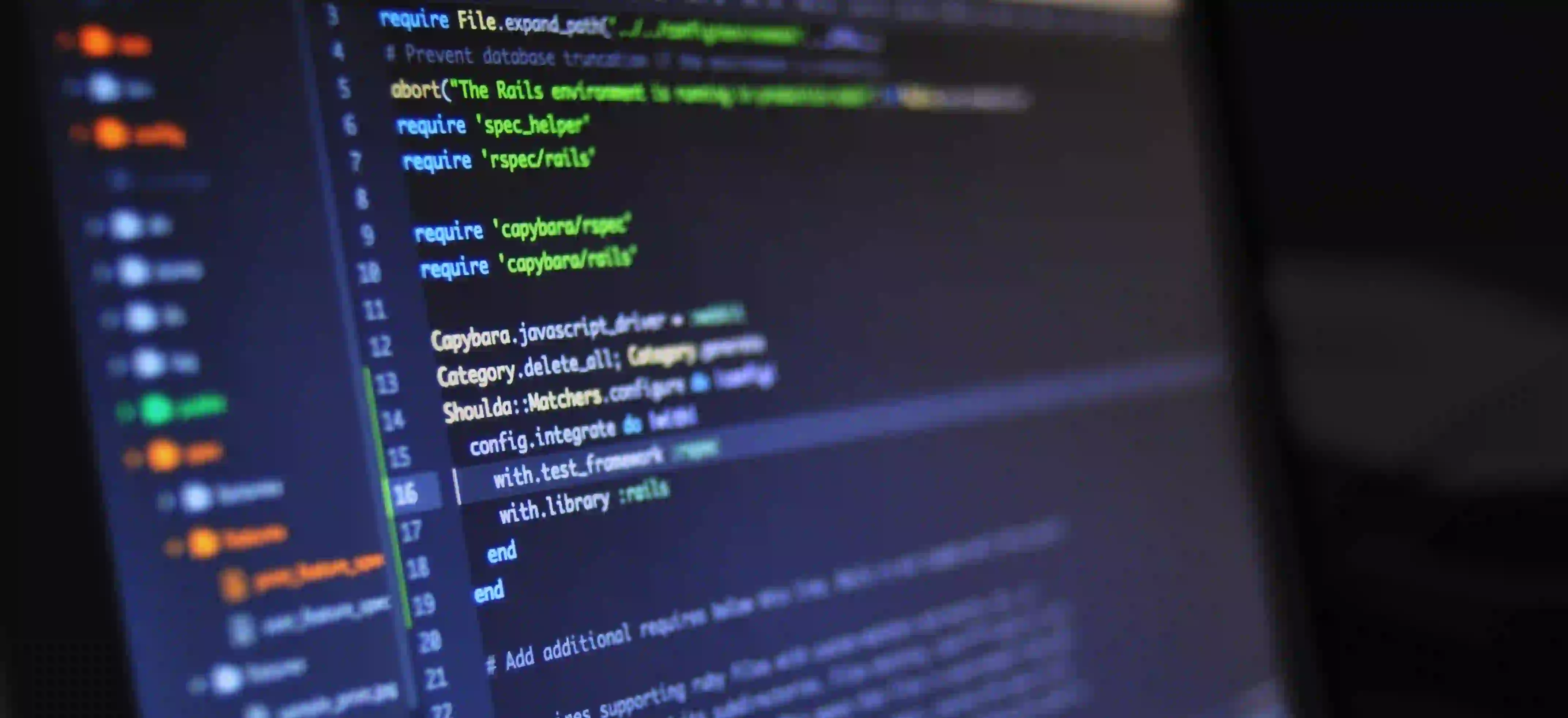
Streamlining Java Applications to Reduce Enterprise Costs
In the ever-evolving landscape of enterprise technology, companies face mounting pressure to optimize their costs while ensuring the performance and reliability of their applications. This need for efficiency is particularly pertinent in the world of Java applications, which can often be resource-intensive. Drawing on best practices, coding techniques, and architectural strategies, this article will explore how to streamline Java applications effectively.
To start, let's recognize that a well-optimized Java application is not just about cutting costs; it's about delivering better value and achieving innovation. If you are interested in understanding how enterprises can navigate high expenses in technology, consider reading the article "Cutting Costs: Navigating High Expenses in Enterprise Tech" at configzen.com/blog/navigating-high-expenses-in-enterprise-tech.
Understanding the Significance of Streamlining
Before diving into specific techniques and code optimizations, it’s essential to understand why streamlining is crucial. Here are a few compelling reasons:
- Performance Efficiency: Streamlined applications consume less memory and processing power, leading to faster response times and better user experiences.
- Cost Management: By reducing the resource footprint, companies can efficiently manage their infrastructure costs—both in cloud services and on-premises servers.
- Maintainability: More efficient code usually means simpler logic and a clearer structure, making maintenance easier and less costly over time.
Now, let’s explore actionable strategies for streamlining Java applications.
1. Optimize Code Structure and Logic
The foundation of any streamlined Java application begins with its code structure. Here are some coding principles to adhere to:
Example: Utilizing Efficient Data Structures
Using the right data structures can significantly impact memory usage and speed. For example, prefer ArrayList
over LinkedList
when fast random access is essential.
import java.util.ArrayList;
public class DataStructureExample {
public static void main(String[] args) {
ArrayList<String> names = new ArrayList<>();
// Adding elements
names.add("Alice");
names.add("Bob");
// Accessing elements – O(1) time complexity
String first = names.get(0);
System.out.println(first); // Output: Alice
}
}
Why This Matters: Using ArrayList
allows for constant-time access to elements, providing a performance boost when dealing with large datasets.
2. Leverage Caching Mechanisms
Caching is an effective way to speed up responses without repeatedly hitting the database or performing expensive calculations. Java offers several caching libraries, including EHCache and Caffeine.
Example: Simple In-memory Caching
Here's a simplified example of an in-memory cache using a Map
:
import java.util.HashMap;
import java.util.Map;
public class SimpleCache {
private Map<String, String> cache = new HashMap<>();
public String getValue(String key) {
return cache.get(key); // Return null if not found
}
public void putValue(String key, String value) {
cache.put(key, value);
}
}
Why This Matters: The time taken to retrieve cached items is substantially lower than making repeated database calls. Depending on your traffic patterns, you can significantly reduce database load and latency through effective caching.
3. Minimize Database Operations
Unoptimized database calls can drain resources and increase costs. Reduce the frequency of calls and batch transactions whenever possible.
Example: Using Batch Inserts
Batch processing in JDBC can enhance performance significantly.
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
import java.sql.SQLException;
public class BatchInsert {
public static void main(String[] args) {
String query = "INSERT INTO employees (name, role) VALUES (?, ?)";
try (Connection conn = DriverManager.getConnection("jdbc:mysql://localhost:3306/company", "user", "password");
PreparedStatement pstmt = conn.prepareStatement(query)) {
// Adding batch entries
for (int i = 0; i < 1000; i++) {
pstmt.setString(1, "Employee" + i);
pstmt.setString(2, "Role" + i);
pstmt.addBatch();
if (i % 100 == 0) { // Execute every 100 items
pstmt.executeBatch();
}
}
pstmt.executeBatch(); // Insert remaining records
} catch (SQLException e) {
e.printStackTrace();
}
}
}
Why This Matters: Executing in batches minimizes the number of transactions, significantly increasing performance and reducing load on the database server.
4. Embrace Asynchronous and Parallel Processing
Java provides robust support for asynchronous processing and parallel programming, utilizing threads or frameworks like CompletableFuture or Java Streams.
Example: Using CompletableFuture for Asynchronous Processing
import java.util.concurrent.CompletableFuture;
public class AsyncExample {
public static void main(String[] args) {
CompletableFuture<String> future = CompletableFuture.supplyAsync(() -> {
return "Hello from async processing!";
});
// Do other work while waiting for the result
future.thenAccept(result -> System.out.println(result));
}
}
Why This Matters: This approach allows your application to remain responsive while executing time-consuming tasks in parallel, leading to better resource utilization.
5. Monitor and Profile Your Applications
Regularly monitoring and profiling your applications is essential. Tools like Java VisualVM, YourKit, or JProfiler can help identify bottlenecks and memory leaks effectively.
Example: Using Java VisualVM
- Start your Java application with
-Dcom.sun.management.jmxremote
. - Launch Java VisualVM from your JDK.
- Connect to your running application and analyze its performance metrics.
Why This Matters: With continuous monitoring, you can gain insights into real-time performance issues and address them before they escalate into critical failures.
Closing Remarks
Streamlining Java applications is a strategic necessity that not only helps in reducing the operational costs but also enhances performance, user satisfaction, and overall maintainability. Implementing the strategies outlined above will take some initial investment in time and effort, but the long-term benefits far outweigh the upfront costs.
By adopting these principles and employing best practices, your organization can continue to leverage Java's capabilities while managing expenses effectively. For additional insights on navigating expenses in enterprise tech, refer to the article "Cutting Costs: Navigating High Expenses in Enterprise Tech" at configzen.com/blog/navigating-high-expenses-in-enterprise-tech.
Don't wait—start streamlining your Java applications today for a more cost-effective tomorrow!