Effortless Java Testing: Automate 150 Tests Quickly!
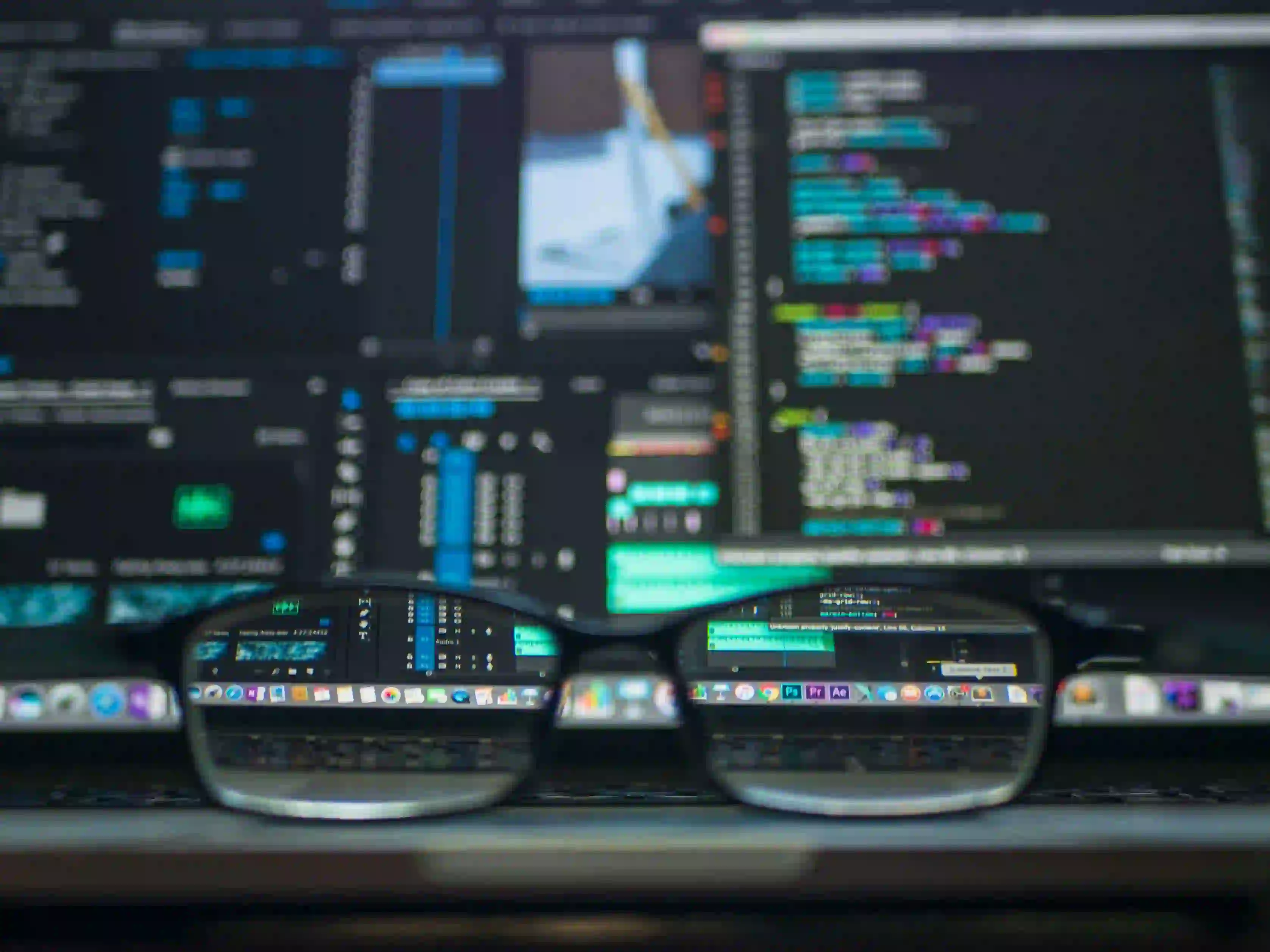
Effortless Java Testing: Automate 150 Tests Quickly!
In today's ever-evolving tech landscape, automated testing has become indispensable for delivering software faster and with fewer issues. With the right tools and planning, your Java applications can be tested quickly and efficiently, ensuring reliability. In this blog post, we'll walk you through the process of creating automated tests for Java applications and how you can perform 150 tests in just 30 minutes, reshaping your testing strategy.
For more advanced strategies, you can read the insightful article titled Mastering Quick Automation: 150 Tests in 30 Minutes!.
Why Automate Testing?
Automated testing has several advantages over manual testing:
- Speed: Tests can be executed faster since they eliminate the sluggish nature of manual testing.
- Reusability: Automated tests can be reused for any iterations in the development cycle, allowing them to save time in the long run.
- Reduced Human Error: Automated tests reduce the possibility of human error during the testing process.
- Continuous Integration: Automated tests can seamlessly integrate into a CI/CD pipeline, enhancing consistency.
Let us dive into how you can implement testing automation in Java.
Setting Up Your Testing Environment
Before we start coding our tests, you'll need to ensure the right tools are set up. Here’s what you’ll need:
- Java Development Kit (JDK): You can download the latest version from Oracle's official website.
- Maven: To manage dependencies efficiently, download Maven and set it up in your environment.
- JUnit: A widely used testing framework for Java. It allows you to create and execute tests effortlessly.
Here’s a quick way to set up JUnit in your Maven project. Add the following dependency to your pom.xml
file:
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>4.13.2</version>
<scope>test</scope>
</dependency>
Explanation
By adding this dependency, you make sure JUnit is included in your build process. You can now use its features to write and execute your tests.
Writing Your First Test
Let's create a simple test class to understand the workflow. Consider a Java class named Calculator
that we want to test:
public class Calculator {
public int add(int a, int b) {
return a + b;
}
}
Next, let's write a test class for it using JUnit:
import static org.junit.Assert.assertEquals;
import org.junit.Test;
public class CalculatorTest {
@Test
public void testAdd() {
Calculator calculator = new Calculator();
int result = calculator.add(5, 3);
assertEquals(8, result);
}
}
Explanation
- Imports: We import the necessary JUnit classes.
assertEquals
is used to verify the expected output. - @Test Annotation: This indicates that the method is a test method.
- Test Logic: It creates an instance of
Calculator
, invokes theadd
method, and usesassertEquals
to verify the outcome.
Running Your Tests
To run the tests, you can utilize the command line:
mvn test
This command will automatically compile your code and run the tests in your project.
Scaling Up: Writing More Tests
Once you're comfortable with the basics, scaling up becomes essential, especially when you aim to reach 150 tests quickly. Below are some advanced tips for efficient test creation:
1. Utilize Test Suites
Test suites allow you to group multiple test cases, making execution more manageable. Here’s an example:
import org.junit.runner.RunWith;
import org.junit.runners.Suite;
@RunWith(Suite.class)
@Suite.SuiteClasses({
CalculatorTest.class,
// add other test classes here
})
public class AllTests {
}
Explanation
The use of @RunWith(Suite.class)
indicates that this class will run all defined test classes. This setup is beneficial for organizing complex projects.
2. Parameterized Tests
You can also create parameterized tests to validate the same logic with different data sets:
import org.junit.Test;
import org.junit.runner.RunWith;
import org.junit.runners.Parameterized;
import java.util.Arrays;
import java.util.Collection;
@RunWith(Parameterized.class)
public class CalculatorParameterizedTest {
private int inputA;
private int inputB;
private int expectedResult;
public CalculatorParameterizedTest(int inputA, int inputB, int expectedResult) {
this.inputA = inputA;
this.inputB = inputB;
this.expectedResult = expectedResult;
}
@Parameterized.Parameters
public static Collection<Object[]> data() {
return Arrays.asList(new Object[][]{
{1, 1, 2},
{2, 3, 5},
{5, 5, 10},
// You can add more test cases here.
});
}
@Test
public void testAdd() {
Calculator calculator = new Calculator();
assertEquals(expectedResult, calculator.add(inputA, inputB));
}
}
Explanation
This test class verifies the add
method with multiple sets of inputs. Each parameter scenario is executed as an individual test, which can significantly increase your test count without duplicating code.
Best Practices for Automated Testing
To maximize the effectiveness of your automated tests, consider these best practices:
- Keep Tests Independent: Make sure that tests do not rely on each other; they should be able to run in any order.
- Write Readable Tests: The test code itself should be clear and easily understandable. Use descriptive names and structure.
- Test Coverage: Aim for high test coverage across your code base. Use tools such as JaCoCo to analyze coverage.
- Continuous Integration: Implement CI tools like Jenkins or Travis CI to run tests automatically upon code changes and maintain consistent quality.
Bonus: Tools to Enhance Your Testing Strategy
When your requirements grow, you may want to expand your testing strategy. Here are some essential tools:
- Selenium: For UI testing, allowing tests to interact with your application's front end.
- Mockito: A powerful mocking framework that can isolate units during tests, simplifying complex test cases.
- TestNG: An alternative to JUnit that provides more powerful test configuration options.
In Conclusion, Here is What Matters
Automating your Java tests is not just a time-saver, but a crucial operational method to ensure the reliability of your applications. By following the guidelines outlined above and incorporating industry best practices, you can efficiently scale your testing efforts and reach your goal of 150 tests in 30 minutes.
For further insights and strategies on rapid testing, do check out the comprehensive article on Mastering Quick Automation: 150 Tests in 30 Minutes!.
Happy coding and testing!