Harnessing Java's Power: Aligning Code with Your Energy Types
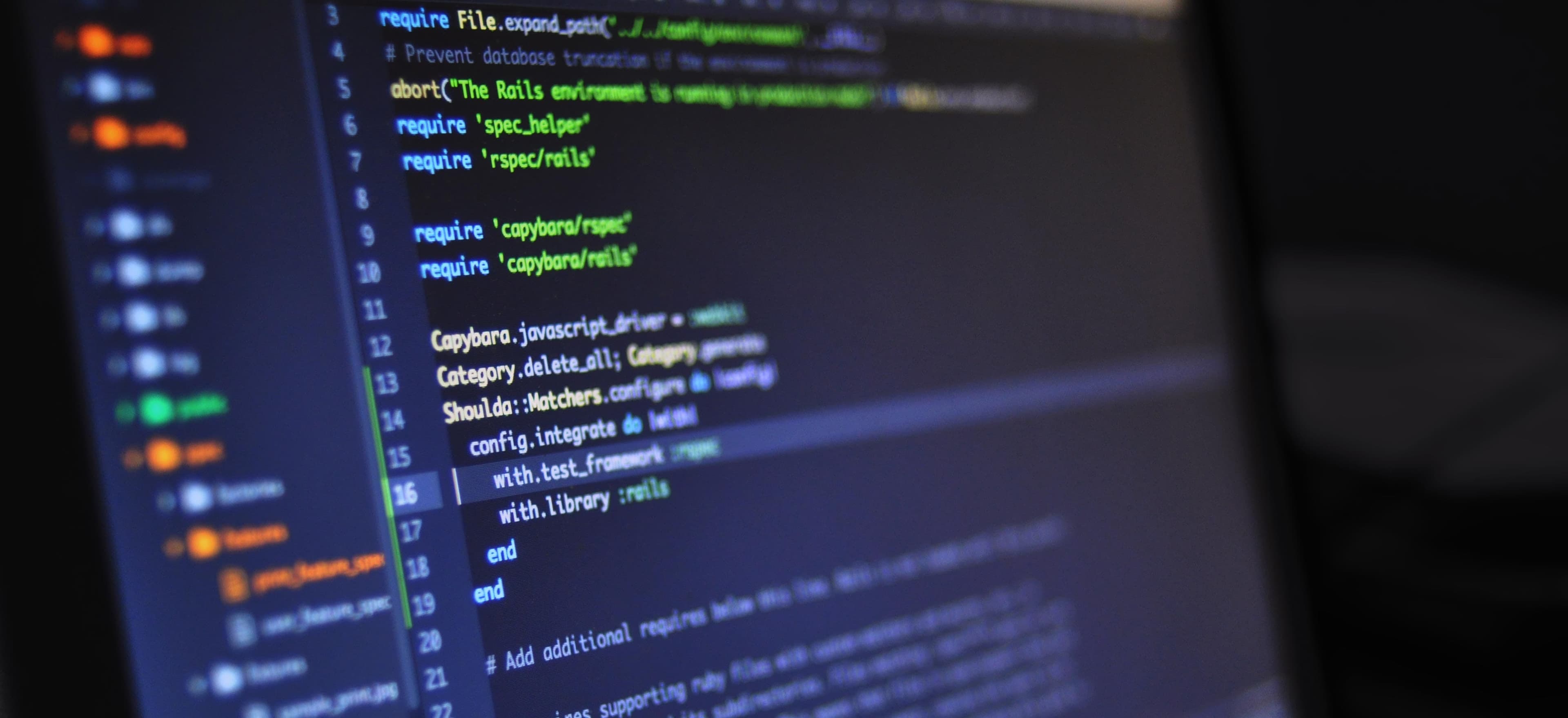
- Published on
Harnessing Java's Power: Aligning Code with Your Energy Types
In the world of programming, Java stands tall as one of the most versatile and widely-used programming languages. Known for its "write once, run anywhere" capability, Java facilitates the development of applications ranging from web services to mobile applications. However, understanding the nuances of Java can sometimes feel overwhelming. This blog post aims not only to demystify Java but also to align your Java coding practices with the metaphysical concepts discussed in the article titled "Widder: Entfessel die Kraft deiner Sternzeichen-Energie!". Just as the stars influence our energy types, how we align our code with best practices can significantly impact our productivity and experience as developers.
Setting the Scene: What Makes Java Special?
Java has an extensive environment consisting of a robust set of libraries and frameworks, which allows developers to build things quickly. Its strong community support, rich ecosystem, and stability make it the go-to choice for developers working on both large and small projects.
Key Features of Java:
- Object-Oriented: Java is built on the principles of object-oriented programming (OOP). This means it encourages better data organization and code modularity.
- Platform Independent: The ability to run Java programs on any device equipped with the Java Virtual Machine (JVM) makes it incredibly versatile.
- Rich Standard Library: Java’s extensive standard library simplifies common programming tasks and accelerates the development process.
Before plunging into code, let’s delve into why these features are essential from a developer’s energy perspective. When you write clean, organized code, you reduce complexity and enhance your flow state—essentially aligning your "energies" with your coding practices for a more productive output.
The Essence of Object-Oriented Programming in Java
Understanding Objects
At the heart of Java programming lies the concept of objects. An object is a self-contained entity that consists of attributes (data) and methods (functions). The beauty of OOP is that it allows programmers to create modules that can be reused without rewriting code. Let’s look at a simple example:
class Car {
// Attributes
String color;
String model;
// Constructor
public Car(String color, String model) {
this.color = color;
this.model = model;
}
// Method to describe the car
public void describe() {
System.out.println("This car is a " + color + " " + model + ".");
}
}
Commentary: Why Object-Oriented?
By encapsulating the properties and behaviors of a car in a single 'Car' class, we capture a real-world object and its characteristics. This modular approach aligns with the idea of focusing on energy types—you create distinct sections of your code that can interact without needing to understand the entire system at once.
When you code in Java, think of each class as a constellation in your personal star chart, reflecting aspects of your coding journey. Just as energy types can holistically influence your life, a well-structured codebase will enhance your overall programming experience.
Aligning Your Code with Best Practices
Having established a foundational understanding of Java, we will explore coding best practices that can help align your programming "energy" for enhanced efficiency.
1. Keep Your Code DRY (Don't Repeat Yourself)
Repetition weakens your code's integrity. Instead of duplicating code, use methods and classes to abstract common functionalities.
class MathUtils {
// Method to add two integers
public static int add(int a, int b) {
return a + b;
}
}
Commentary: Why DRY?
By employing the DRY principle, you simplify maintenance and debugging. If a bug arises in the addition method, fixing that method will automatically correct all instances where it’s used across your code. This mirrors the stars—though they seem distant, their gravitational pull affects everything around them.
2. Utilize Meaningful Names
Name your classes, methods, and variables descriptively. Obscure names lead to confusion and errors.
public class UserProfile {
private String username;
private String password;
public UserProfile(String username, String password) {
this.username = username;
this.password = password;
}
// Method to display user info
public void displayInfo() {
System.out.println("User: " + username);
}
}
Commentary: Why Naming Matters?
Descriptive naming is akin to understanding your astrological signs. Just as knowing the traits of your sun sign can help you navigate your life, using clear names helps you and others grasp the purpose behind your code without needing a cryptic interpretation.
Diving into Advanced Java Features
To elevate your Java programming game, let’s explore some advanced features that can further align your code with best practices.
Exception Handling
Robust applications anticipate and manage errors gracefully. Java provides a structured way to handle exceptions using try-catch
blocks.
try {
int[] numbers = {1, 2, 3};
System.out.println(numbers[3]); // This line will throw an exception
} catch (ArrayIndexOutOfBoundsException e) {
System.out.println("An error occurred: " + e.getMessage());
}
Commentary: Why Exception Handling?
Exception handling is critical for maintaining an application's stability. It’s similar to overcoming challenges in life. When you encounter an error, how you handle it can determine your future path. Thus, handling exceptions in Java prepares you for any unforeseen circumstances.
Stream API
For powerful data manipulation, the Stream API allows you to work with collections in a functional style.
List<String> names = Arrays.asList("Alice", "Bob", "Charlie");
List<String> filteredNames = names.stream()
.filter(name -> name.startsWith("A"))
.collect(Collectors.toList());
System.out.println(filteredNames); // Output: [Alice]
Commentary: Why Streams?
The Stream API enhances readability and reduces boilerplate code, allowing you to focus on the "what" instead of the "how." Much like the energy of your astrological sign, it simplifies complex operations into an elegant flow.
Closing the Chapter: Harmonizing Your Java Journey
In conclusion, Java is not just a programming language; it can serve as a reflection of your coding energy and practices. By understanding core concepts like OOP, naming conventions, and advanced features such as exception handling and streams, you create a coding environment that echoes the harmony of your personal energy type, as discussed in the article "Widder: Entfessel die Kraft deiner Sternzeichen-Energie!".
Remember, the way you write your code is not merely an act of programming—it’s a reflection of your mindset and your energy. Align these forces, and Java can be a powerful ally in your journey of innovation and creativity. Whether you’re building the next big application or refining your coding skills, always strive for clarity, efficiency, and organization in your code. Let the energies of the stars guide your development journey as you harness the power of Java!
Checkout our other articles