Java for Preppers: Building Disaster Recovery Applications
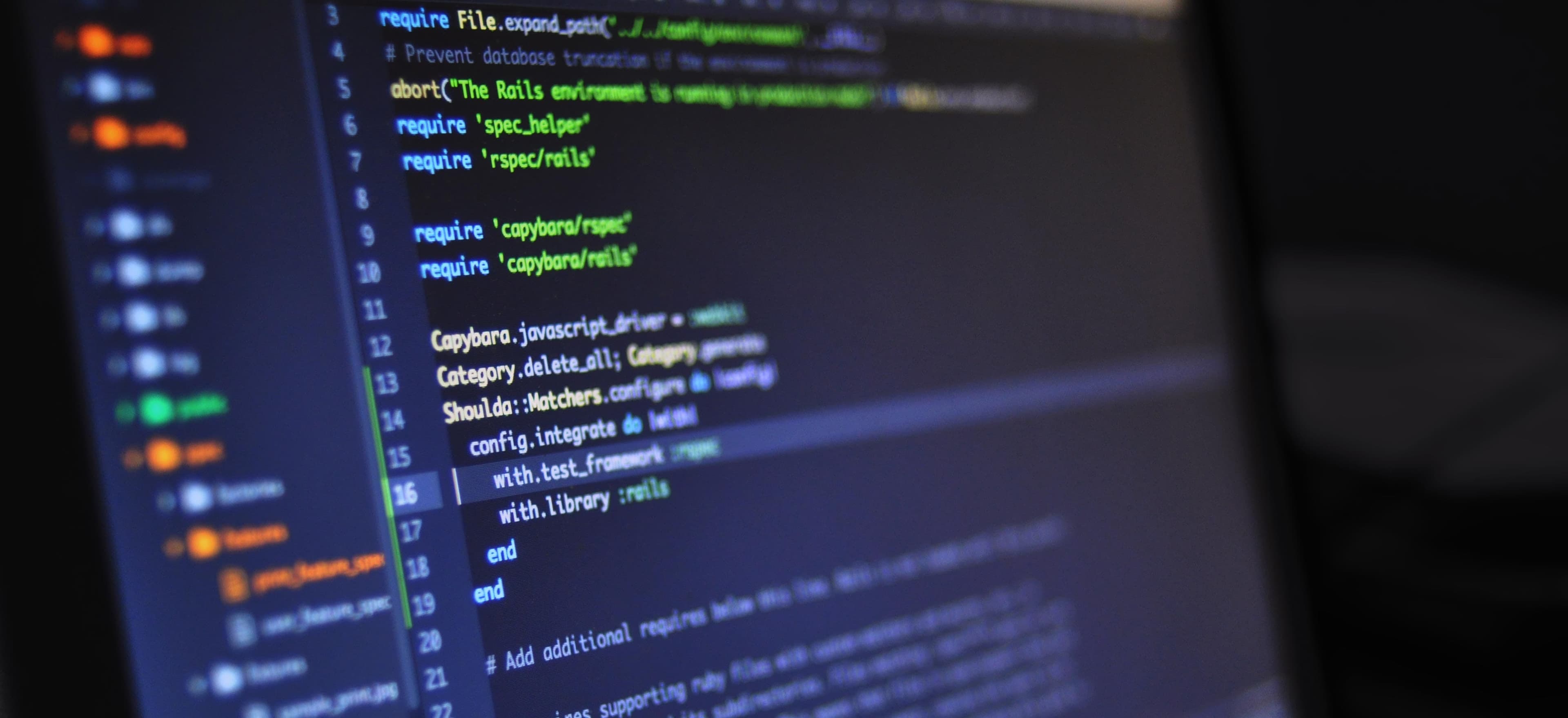
- Published on
Java for Preppers: Building Disaster Recovery Applications
In a world where uncertainties loom over daily life, the necessity for preparedness has never been more pronounced. From natural disasters to unforeseen emergencies, being ready can often make the difference between chaos and order. The recent discourse surrounding the Mormon Prepper Movement highlights the importance of having a mindset geared towards resilience and survival (you can read more about this in Survival and Faith: Navigating the Mormon Prepper Movement). A crucial part of this preparedness involves developing robust and efficient applications that can assist in disaster recovery. This is where Java, one of the most versatile and widely-used programming languages, comes into play.
Why Java?
Java is not just a language; it's a toolkit that has stood the test of time. Here are some reasons why Java is an excellent choice for building disaster recovery applications:
- Platform Independence: Java's "Write Once, Run Anywhere" philosophy ensures applications can run on various devices and platforms.
- Strong Community Support: With a vast developer community, finding resources, libraries, and frameworks is easier than ever.
- Robust Security Features: Security is crucial when dealing with sensitive data, especially during crises. Java provides a range of built-in security features.
- Multi-threading Capabilities: Java allows for performing multiple operations at once, which is essential in emergency situations.
Understanding Disaster Recovery Applications
Before diving into code, it's essential to understand the core functionalities a disaster recovery application should possess. These applications generally focus on:
- Data Backup and Restoration: Ensuring critical data can be easily retrieved.
- Real-time Monitoring: Keeping track of relevant data, such as weather conditions or emergency alerts.
- Communication Tools: Providing ways for individuals to communicate and share resources effectively.
Getting Started: Setting Up Your Environment
To start building your disaster recovery application, you need a development environment. The simplest setup for Java development includes:
- Java Development Kit (JDK): Download and install the latest JDK from the official site.
- Integrated Development Environment (IDE): Popular choices include IntelliJ IDEA, Eclipse, or NetBeans.
Sample Code: A Simple Backup Tool
Now, let's look at a simple Java application that can back up files to ensure data safety. The following code snippet demonstrates how to copy files from a source to a backup directory:
import java.io.File;
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.io.IOException;
import java.nio.file.Files;
public class FileBackup {
public static void main(String[] args) {
String sourceDir = "C:/user/data"; // Change the path according to your needs
String backupDir = "C:/user/backup";
File source = new File(sourceDir);
File backup = new File(backupDir);
// Ensure backup directory exists
if (!backup.exists()) {
backup.mkdir();
}
try {
for (File file : source.listFiles()) {
if (file.isFile()) {
backupFile(file, new File(backup, file.getName()));
}
}
} catch (IOException e) {
System.err.println("Error during file backup: " + e.getMessage());
}
}
private static void backupFile(File sourceFile, File backupFile) throws IOException {
try (FileInputStream in = new FileInputStream(sourceFile);
FileOutputStream out = new FileOutputStream(backupFile)) {
byte[] buffer = new byte[1024];
int length;
while ((length = in.read(buffer)) > 0) {
out.write(buffer, 0, length);
}
}
System.out.println("Backup completed for: " + sourceFile.getName());
}
}
Commentary on the Code
This simple backup tool does the following:
- Defines Source and Backup Directories: Change the paths according to your folder structure.
- Checks and Creates Backup Directory: If the backup directory does not exist, it creates one to house backup files.
- Copies Files: Iterates through the source directory, copying files to the backup directory.
A fundamental aspect of this code is error handling. Implementing robust error handling mechanisms, as shown, ensures your application can gracefully deal with problems.
Adding Real-time Monitoring
Another key component of disaster recovery applications is real-time monitoring. For instance, let's implement a simple system that checks the status of a server, which could be critical during a disaster scenario.
import java.io.IOException;
import java.net.HttpURLConnection;
import java.net.URL;
public class ServerMonitor {
public static void main(String[] args) {
String urlString = "http://example.com"; // Replace with actual URL to monitor
try {
URL url = new URL(urlString);
HttpURLConnection connection = (HttpURLConnection) url.openConnection();
connection.setRequestMethod("HEAD");
connection.setConnectTimeout(5000);
connection.setReadTimeout(5000);
int responseCode = connection.getResponseCode();
System.out.println("Response Code: " + responseCode);
if (responseCode == 200) {
System.out.println("Server is online.");
} else {
System.out.println("Server is down or unreachable.");
}
} catch (IOException e) {
System.err.println("Error monitoring server: " + e.getMessage());
}
}
}
Commentary on the Monitoring Code
In this example:
- HTTP Connection: Establishes a connection to a specified URL to check its status.
- Response Code Evaluation: Checks if the server is online by interpreting the HTTP response.
Understanding how to monitor services within your preparedness plan provides valuable insight during disaster recovery scenarios.
Integrating Communication Tools
Effective communication can be a lifesaver during emergencies. By integrating APIs such as Twilio for SMS notifications or Telegram Bot for alerts, you can keep individuals informed.
Consider this basic setup using Twilio to send an SMS:
import com.twilio.Twilio;
import com.twilio.rest.api.v2010.account.Message;
import com.twilio.type.PhoneNumber;
public class SmsNotification {
public static final String ACCOUNT_SID = "your_account_sid"; // Retrieve from your Twilio account
public static final String AUTH_TOKEN = "your_auth_token"; // Retrieve from your Twilio account
public static void main(String[] args) {
Twilio.init(ACCOUNT_SID, AUTH_TOKEN);
Message message = Message.creator(
new PhoneNumber("to_phone_number"), // Destination phone number
new PhoneNumber("your_twilio_number"), // Your Twilio number
"This is a test message for disaster recovery.")
.create();
System.out.println("Message sent: " + message.getSid());
}
}
Commentary on the SMS Code
- Twilio Integration: Initializes Twilio with your credentials for authenticating API requests.
- Message Creation: Sends a message notifying users about a disaster or important update.
The integration of communication features fosters community engagement and enhances personal readiness during emergencies.
Wrapping Up
Building disaster recovery applications using Java can empower communities, improve individual readiness, and simplify the recovery process. By focusing on essential features such as data backup, real-time monitoring, and communication tools, developers can create powerful solutions that balance functionality with usability.
In closing, as the landscape of preparedness continues to shift, the role of technology—especially applications like Java—becomes imperative. Remember, whether it's enhancing interpersonal communication or safeguarding data, the right tools make all the difference.
For additional reading on preparedness philosophies and strategies, check out the insightful article on Survival and Faith: Navigating the Mormon Prepper Movement.
By maintaining readiness through technology, we foster a stronger, more resilient society ready to face unexpected challenges—armed with both physical resources and digital tools.
Checkout our other articles