Java Solutions for Building Resilient Prepper Apps
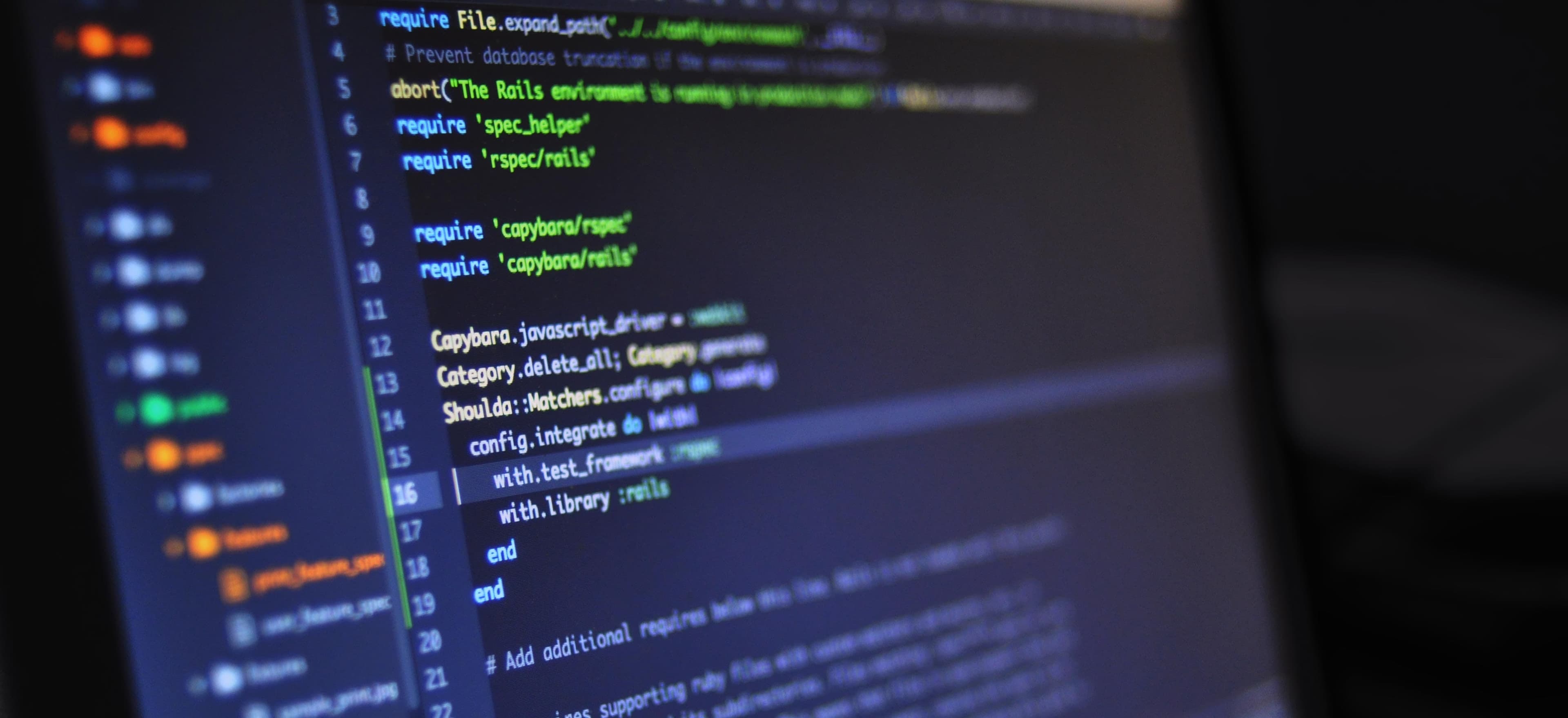
- Published on
Java Solutions for Building Resilient Prepper Apps
In the fast-paced world we live in today, preparing for unforeseen circumstances is a priority for many individuals. This is where the concept of "prepping" comes into play. As discussed in the insightful article, Survival and Faith: Navigating the Mormon Prepper Movement, prepping is not just about stockpiling supplies but also about building a community and developing skills. As technology continues to evolve, building resilient prepper applications can provide valuable tools for individuals and communities alike.
In this blog post, we'll explore how Java can be used to build robust prepper solutions. We'll delve into the essential features of these applications and provide actionable code snippets to guide you through the development process.
Understanding the Need for Prepper Apps
Before diving into the technical aspects, let’s analyze why prepper applications are necessary.
- Resource Management: Keeping track of food, water, and medical supplies is crucial in a crisis. An app can help users manage inventory effectively.
- Emergency Planning: Apps can assist in developing emergency plans, offering guidelines and checklists that users can follow.
- Community Connection: Many preppers value community and sharing skills. A platform to connect individuals can foster collaboration and support.
- Education: Providing resources like survival tips, skills training, and instructional videos is essential for beginners and seasoned preppers alike.
Key Features of a Resilient Prepper App
When building a prepper application, several core features should be considered:
1. Inventory Tracking
An efficient way to track and manage supplies is a critical component of any prepper app. Users should be able to add, edit, and delete items from their inventory, along with other relevant details such as expiration dates.
Code Example: Inventory Management
import java.util.ArrayList;
import java.util.Iterator;
import java.util.List;
public class InventoryManager {
private List<Item> inventory;
public InventoryManager() {
this.inventory = new ArrayList<>();
}
public void addItem(String name, int quantity, String expirationDate) {
inventory.add(new Item(name, quantity, expirationDate));
System.out.println("Added " + quantity + " of " + name + " to inventory.");
}
public void removeItem(String name) {
Iterator<Item> iter = inventory.iterator();
while (iter.hasNext()) {
Item item = iter.next();
if (item.getName().equals(name)) {
iter.remove();
System.out.println("Removed " + name + " from inventory.");
return;
}
}
System.out.println(name + " not found in inventory.");
}
public void displayInventory() {
System.out.println("Current inventory:");
for (Item item : inventory) {
System.out.println("- " + item.getName() + ": " + item.getQuantity() +
" (Expires on: " + item.getExpirationDate() + ")");
}
}
}
class Item {
private String name;
private int quantity;
private String expirationDate;
public Item(String name, int quantity, String expirationDate) {
this.name = name;
this.quantity = quantity;
this.expirationDate = expirationDate;
}
public String getName() { return name; }
public int getQuantity() { return quantity; }
public String getExpirationDate() { return expirationDate; }
}
Commentary
The InventoryManager
class provides functionality to manage supplies effectively. The addItem
method allows users to add items to their inventory, while the removeItem
method supports the removal of items when necessary. The displayInventory
method offers a clear overview of what supplies are on hand.
2. Emergency Plans
In a crisis, having predefined emergency plans is invaluable. Users can create and save a tailored emergency plan that outlines actions, resources, and contacts.
Code Snippet: Emergency Plan Storage
import java.util.HashMap;
import java.util.Map;
public class EmergencyPlan {
private Map<String, String> plan;
public EmergencyPlan() {
plan = new HashMap<>();
}
public void addPlanStep(String step, String details) {
plan.put(step, details);
System.out.println("Added step: " + step);
}
public void displayPlan() {
System.out.println("Emergency Plan:");
for (String step : plan.keySet()) {
System.out.println(step + ": " + plan.get(step));
}
}
}
Commentary
The EmergencyPlan
class allows users to add steps to their emergency plans. Utilizing a HashMap
make it easy to associate each step with relevant details. The displayPlan
method provides a straightforward presentation of the plan.
3. Community Features
Connecting with like-minded individuals is essential for sharing knowledge and resources. Implementing a forum or chat feature boosts community interaction within the app.
4. Educational Content
Incorporating articles, videos, and tutorials helps users learn critical survival skills. A section for educational content can be integrated using simple interfaces.
To Wrap Things Up
Java offers a robust environment for building resilient prepper applications. By implementing key features such as inventory tracking, emergency plan management, community connections, and educational content, developers can create tools that empower individuals to prepare for whatever may come their way.
Invitation to Engage
I encourage you to take these ideas and implement them in your prepper applications. The world of prepping requires adaptability and strong community ties, and a well-crafted app can bridge those gaps. For further insights into the lifestyle and the significance of community, check out the article Survival and Faith: Navigating the Mormon Prepper Movement.
Happy coding!
Checkout our other articles