Solving Java Integrations for Smooth Locomotive Scroll in Vue
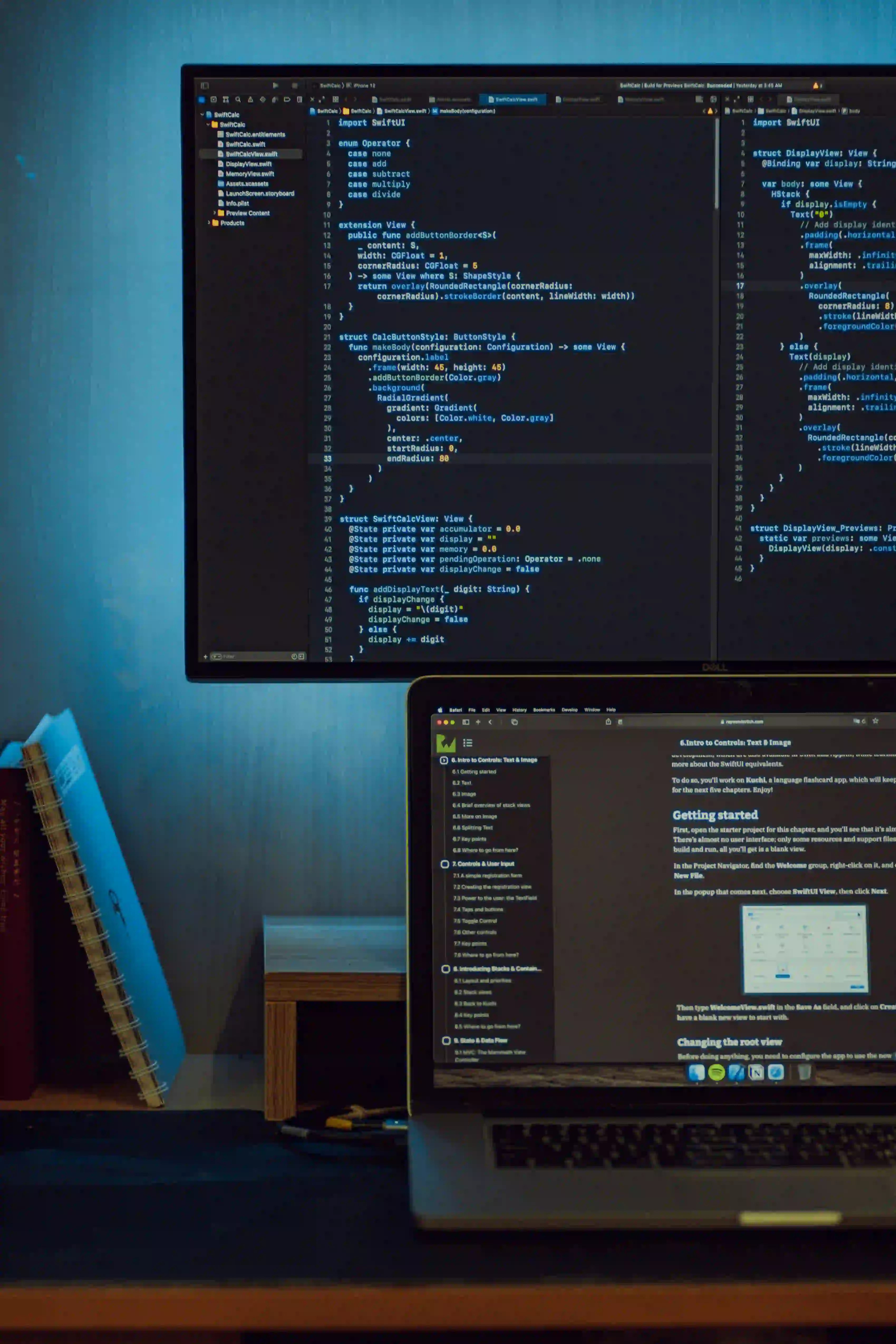
Solving Java Integrations for Smooth Locomotive Scroll in Vue
In the ever-evolving world of web development, combining various technologies to create smooth, interactive experiences is key. One popular way to enhance scrolling capabilities in JavaScript frameworks like Vue is through the use of Locomotive Scroll. This library smoothly animates scrolling by providing a silky experience, enhancing user engagement. However, when integrating Locomotive Scroll with Java backends for applications that need high performance and scalability, challenges may arise. This blog post will discuss how to solve Java integrations for achieving smooth Locomotive Scroll in Vue applications.
Understanding Locomotive Scroll
Locomotive Scroll is a powerful library designed to provide a smoother scrolling experience. It uses a parallax effect to animate elements based on scroll position and transforms the default scroll behavior. This results in a more visually appealing user interface but can pose integration challenges with backend technologies, particularly Java.
Key Features of Locomotive Scroll
- Smooth Scrolling: Reduces jank and stutter in the scrolling experience.
- Parallax Effects: Adds depth to the user interface.
- Responsive: Adapts to different screen sizes and orientations.
Why Use Java with Vue?
Java is known for its robustness and scalability, making it an excellent choice for backend development. When building applications with Vue on the front end, you can benefit from Java’s powerful data handling and complex business logic capabilities. However, to achieve seamless communication between the Java backend and the Vue front end, a well-structured integration is crucial.
Common Issues with Locomotive Scroll and Java
Integrating Locomotive Scroll in a Vue application using a Java backend can come with its own set of challenges:
- Data Fetching Bottlenecks: If the data from the Java backend is slow to load, it can impact scrolling animations.
- State Management: Managing component state between Java services and Vue can get complicated, specifically when data needs to be updated in real-time.
- Event Handling: Locomotive Scroll uses scroll and wheel events; thus, improper event handlers may lead to undesirable scroll behavior.
To handle these issues more effectively, we should look at how Java can be utilized effectively to connect with Vue.
Setting Up Your Java Backend
Before diving into code, ensure you have your Java backend set up appropriately. A typical setup will involve:
- A RESTful API to manage data retrieval.
- Spring Boot (or another framework) to enhance productivity.
- A well-defined data model to manage the interaction between your back end and front end.
Example Java Code
Here is a simple Spring Boot example for creating a RESTful API. This code retrieves data from a database and sends it to your Vue component:
@RestController
@RequestMapping("/api/data")
public class DataController {
@Autowired
private DataService dataService;
@GetMapping("/items")
public ResponseEntity<List<Item>> getItems() {
List<Item> items = dataService.getAllItems();
return new ResponseEntity<>(items, HttpStatus.OK);
}
}
Commentary
- @RestController: This annotation tells Spring Boot that this class will handle web requests.
- @GetMapping: Specifies that this method is a handler for GET requests, which is perfect for fetching data.
- ResponseEntity: Allows you to customize the HTTP response in terms of status code and response body.
Handling Data in Vue
Once you have your Java backend set up, you need to connect it to your Vue.js components. You can make HTTP requests to your Java API using libraries like Axios.
Example Vue Code
Here’s how you can fetch data from the Java backend in your Vue.js component:
<template>
<div class="item-list" v-locomotive-scroll>
<div class="item" v-for="item in items" :key="item.id">
{{ item.name }}
</div>
</div>
</template>
<script>
import axios from 'axios';
export default {
data() {
return {
items: []
};
},
mounted() {
this.fetchItems();
},
methods: {
fetchItems() {
axios.get('/api/data/items')
.then(response => {
this.items = response.data;
})
.catch(error => {
console.error("There was an error fetching the items:", error);
});
}
}
};
</script>
<style scoped>
.item-list {
/* Styling to ensure locomotion effect */
}
</style>
Commentary
- v-locomotive-scroll: This directive initializes Locomotive Scroll on this element, allowing smooth animations.
- Axios: A promise-based HTTP client that simplifies making requests to your Java backend.
- Error Handling: Always include error handling to catch issues during data fetching.
Best Practices for Smooth Integration
- Optimize API Calls: Ensure your Java backend is optimized for fast data access. This might mean leveraging caching mechanisms.
- Debounce Scroll Events: To improve performance, debounce scroll events in Vue to prevent excessive function calls while scrolling.
- Use Local Storage: Utilize local storage to save fetched data if it doesn't change frequently, reducing unnecessary API requests.
Troubleshooting Common Issues
If you experience common issues, refer to the article titled "Fixing Common Locomotive Scroll Issues in Vue Projects" for detailed insights.
This resource tackles problems like stuttering when fetching data, and gives performance tips tailored to Vue integrations.
To Wrap Things Up
By harnessing the power of both Java and Vue with Locomotive Scroll, you can create applications that are not only visually appealing but also robust and smooth in scrolling behavior. The key lies in optimizing your data flow between the backend and frontend, carefully managing component states, and addressing performance concerns.
With the techniques discussed above, you are well-equipped to solve Java integrations for smooth Locomotive Scroll in Vue. Be sure to follow best practices and troubleshoot diligently to ensure a seamless user experience.
By embracing these principles, your web applications will stand out, providing users with exceptional functionality and aesthetic appeal. Happy coding!