Resolving Java Scroll Performance in Web Frameworks
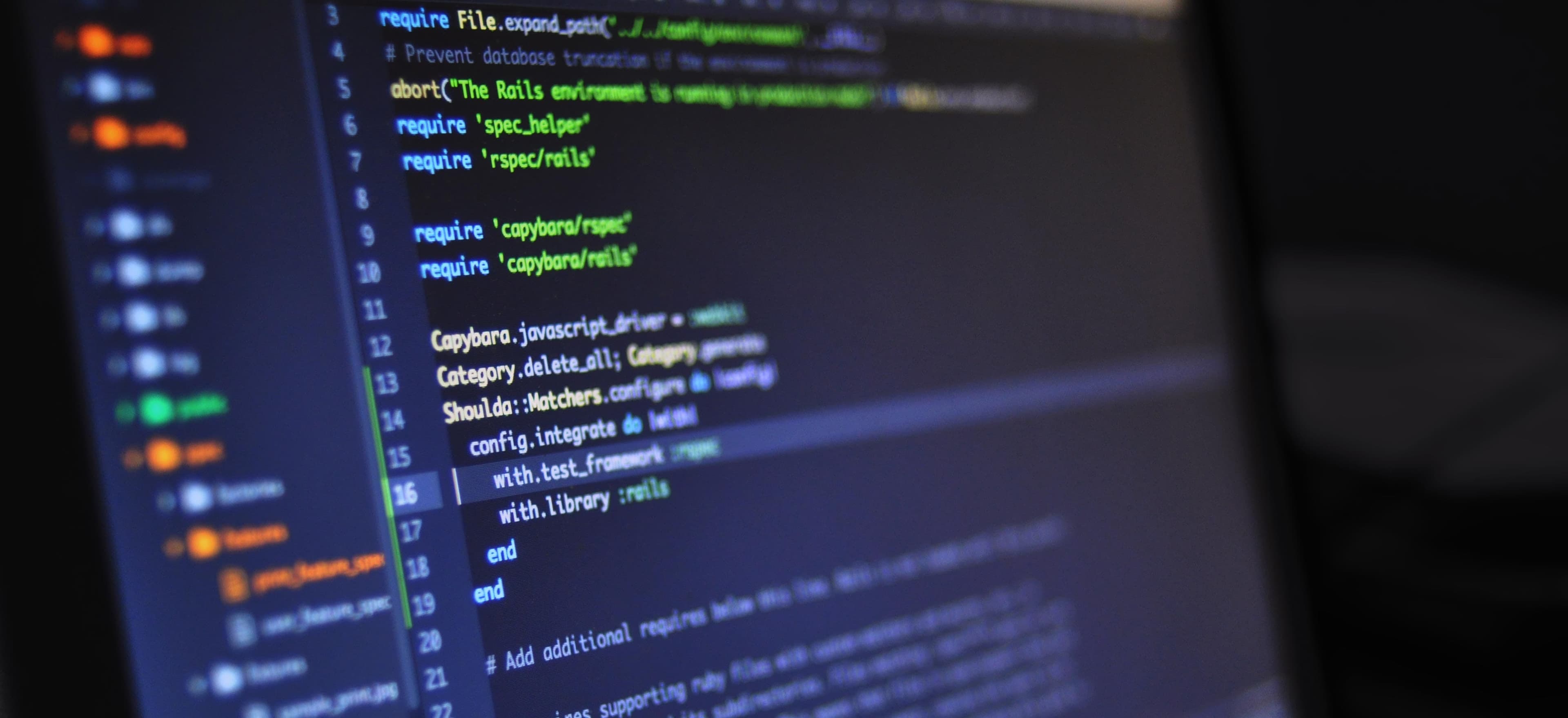
- Published on
Resolving Java Scroll Performance in Web Frameworks
Scroll performance is crucial for providing a seamless user experience in web applications. In Java-based web frameworks, optimizing scroll performance can lead to a smoother navigation experience and improved overall user satisfaction. In this blog post, we will delve into various techniques for maximizing scroll performance in Java applications.
Understanding Scroll Performance
Before diving into solutions, it's essential to understand what we mean by scroll performance. At its core, scroll performance refers to how smoothly content moves on the screen as a user scrolls through it. A lagging or jerky scroll can lead to frustration, causing users to abandon your application.
Scroll performance can be affected by a variety of factors, including:
- Rendering Load: How quickly the browser can render new content.
- JavaScript Execution: How efficiently your JavaScript runs during scroll events.
- Layout Thrashing: Frequent reflows and repaints can lead to frame drops.
Key Techniques for Improving Scroll Performance
Let's explore several strategies focusing on Java-based web frameworks for optimizing scroll performance.
1. Efficient Use of Java Server-side Rendering
Server-side rendering (SSR) can significantly enhance the initial load time of your web application. When a user requests a page, the server sends a fully rendered HTML response rather than allowing the browser to construct the page from JavaScript.
Example: Using Spring Framework for SSR
Spring MVC allows you to create server-rendered views easily. Here's a simple example of setting up a controller for rendering HTML content:
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.GetMapping;
@Controller
public class ScrollController {
@GetMapping("/scrollPage")
public String scrollPage() {
return "scrollPage"; // returns scrollPage.html
}
}
By serving the page directly from the server, you minimize the load on the client's browser, resulting in smoother scrolling.
2. Debouncing Scroll Events
One common pitfall in web applications is listening to scroll events without throttling or debouncing them. When a user scrolls, the event can fire many times per second, leading to performance degradation.
Example: Debouncing Scroll Events in JavaScript
let timeout = null;
window.addEventListener('scroll', function() {
clearTimeout(timeout);
timeout = setTimeout(function() {
// Perform actions after scrolling stops
console.log('Scrolling ended');
}, 100);
});
Debouncing ensures that your logic only runs after the user has stopped scrolling, reducing the load on your JavaScript engine.
3. Virtual Scrolling
If your application is displaying a large list of items, consider using virtual scrolling. This technique only renders the items currently visible in the viewport, drastically reducing the rendering load.
Example: Implementing Virtual Scrolling with Java
Suppose you are building a list that shows 1000 items. With virtual scrolling, you only render a handful of those items. You can use a combination of Java with client-side rendering via JavaScript:
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RestController;
import java.util.List;
@RestController
public class VirtualScrollController {
@GetMapping("/items")
public List<Item> getItems(int start, int count) {
// Fetch only the required items from the database
return itemService.fetchItems(start, count);
}
}
On the frontend, you would only request the items that are currently in view, improving load times and scroll responsiveness.
4. CSS Optimization
Another often-overlooked aspect of scroll performance is CSS. Minimizing complex styles can significantly improve scrolling smoothness.
- Avoid heavy box-shadow and filter effects.
- Minimize the use of expensive CSS properties like
position: fixed;
.
Example: Simple CSS Styles for Smooth Scrolling
body {
overflow: auto;
transition: background-color 0.3s ease; /* smooth background transition */
}
.item {
height: 100px;
margin: 10px;
border: 1px solid #ccc;
}
Making small adjustments in CSS can lead to marked improvements in rendering performance.
5. Optimize Image Loading
Images can be one of the most significant causes of slow scrolling if not managed correctly. Use responsive images and lazy loading to improve performance.
Example: Lazy Loading Images in a Java Application
You can implement lazy loading in Java-based applications using simple HTML attributes:
<img src="small-image.jpg" data-src="large-image.jpg" class="lazy" alt="Image description">
In your JavaScript, you can enable lazy loading as follows:
document.addEventListener("DOMContentLoaded", function() {
const lazyImages = document.querySelectorAll("img.lazy");
const imageObserver = new IntersectionObserver(entries => {
entries.forEach(entry => {
if (entry.isIntersecting) {
const img = entry.target;
img.src = img.dataset.src; // Load the large image
img.classList.remove('lazy');
imageObserver.unobserve(img);
}
});
});
lazyImages.forEach(image => {
imageObserver.observe(image);
});
});
This way, images are loaded only as they scroll into the viewport, optimizing performance.
Wrapping Up
Optimizing scroll performance is integral to enhancing user experience in Java-based web applications. By employing techniques such as server-side rendering, debouncing scroll events, virtual scrolling, CSS optimization, and lazy loading, you can ensure a smooth and responsive interface for your users.
For those interested in further reading on optimizing scrolling in web applications, check out the article titled Fixing Common Locomotive Scroll Issues in Vue Projects.
By implementing these strategies, developers can create robust web applications that not only retain users but also deliver an enjoyable experience. Happy coding!