Resolving Locomotive Scroll Conflicts in Java Web Apps
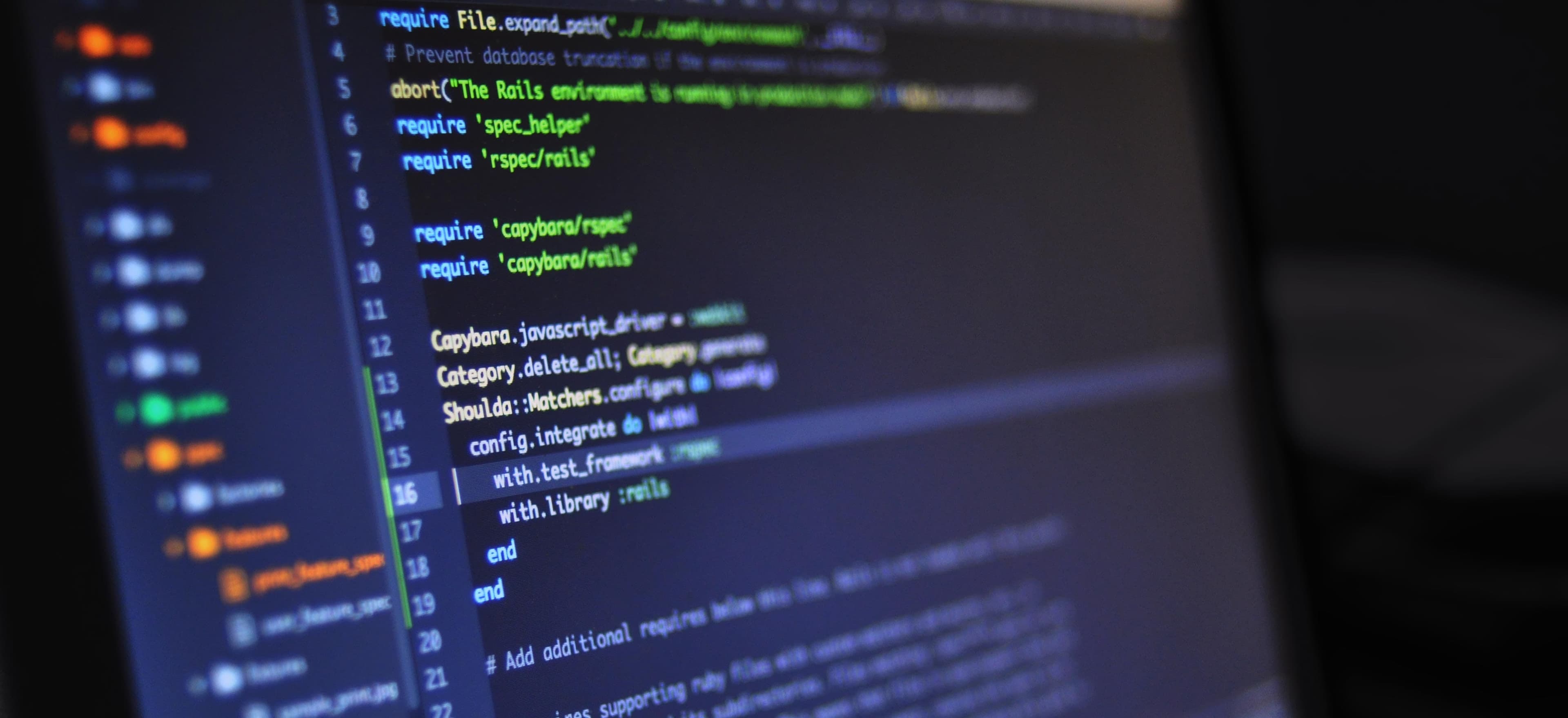
- Published on
Resolving Locomotive Scroll Conflicts in Java Web Apps
Locomotive Scroll is a powerful library that enables smooth scrolling and parallax effects in web applications. However, integrating it into Java web applications can sometimes lead to conflicts, especially when using complex frameworks like Spring or Java Server Faces (JSF). In this post, we will delve into the challenges faced when combining Locomotive Scroll with Java web apps and provide actionable solutions.
What is Locomotive Scroll?
Before diving into the conflicts, it’s essential to understand what Locomotive Scroll does. This library enhances scrolling experience by creating a unique scroll-based interaction. It assists in smooth scroll transitions and the ability to integrate parallax effects without requiring complex setups.
For more information about Locomotive Scroll and its features, you can visit the official documentation at Locomotive Scroll.
Common Issues with Locomotive Scroll in Java Web Apps
While Locomotive Scroll is robust, you might encounter several issues when integrating it into Java web applications:
- Incompatibility with other JavaScript Libraries: Java applications often incorporate multiple libraries that might interfere with Locomotive Scroll's functionality.
- DOM Handling Conflicts: When Java frameworks handle the Document Object Model (DOM) differently, issues arise regarding scroll positions and ease of event handling.
- Performance Problems: If not properly optimized, Locomotive Scroll can negatively impact the performance of Java-based web applications.
Let’s take a closer look at how to troubleshoot these issues effectively.
1. Incompatibility with Other Libraries
When Java web applications are used with multiple JavaScript libraries, compatibility issues may emerge. Here’s a general way to resolve them:
Solution: Namespace JavaScript Libraries
To avoid conflicts, it’s advisable to namespace your JavaScript functions. This approach reduces the chance of name collisions between libraries.
var MyApp = MyApp || {}; // Create a namespace
MyApp.initLocomotiveScroll = function() {
const scroll = new LocomotiveScroll({
el: document.querySelector("[data-scroll-container]"),
smooth: true
});
};
document.addEventListener("DOMContentLoaded", function() {
MyApp.initLocomotiveScroll();
});
Why: By encapsulating your JavaScript within a namespace, you can prevent other libraries from accidentally overwriting your initialization functions or requirements.
2. DOM Handling Conflicts
Java frameworks like JSF can manipulate the DOM in such a way that can conflict with Locomotive Scroll's initialization process.
Solution: Reinitialize Locomotive Scroll on DOM Changes
Whenever the DOM changes, such as Ajax updates in a JSF application, re-initialize Locomotive Scroll:
function reinitializeScroll() {
if (typeof scroll !== "undefined") {
scroll.destroy();
}
MyApp.initLocomotiveScroll();
}
// Call this function whenever you make a significant DOM change
document.addEventListener('DOMNodeInserted', reinitializeScroll);
Why: This ensures Locomotive Scroll is aware of dynamic changes, maintaining smooth scrolling even when the DOM structure is altered by the Java framework.
3. Handling Performance Issues
Performance can lag if Locomotive Scroll interacts with heavy elements or poorly optimized animations.
Solution: Optimize Animations and Throttle Scroll Events
Limit the number of elements affected by Locomotive Scroll and minimize excessive animations. Use requestAnimationFrame
to optimize scroll events:
let lastKnownScrollPosition = 0;
let ticking = false;
function doSomething(scrollPos) {
// Handle scroll logic
console.log(scrollPos);
}
document.addEventListener("scroll", function() {
lastKnownScrollPosition = window.scrollY;
if (!ticking) {
window.requestAnimationFrame(function() {
doSomething(lastKnownScrollPosition);
ticking = false;
});
ticking = true;
}
});
Why: By throttling the scroll event, we reduce performance issues and ensure that the browser doesn't get overwhelmed with too many actions at once.
Closing Remarks
Integrating Locomotive Scroll into Java web applications can enhance user experience with smooth scrolling and interactive effects. However, it is crucial to be aware of compatibility, DOM handling, and performance issues. By implementing the solutions outlined in this article, you can effectively resolve common conflicts that may arise.
For a deeper understanding of handling scrolling issues in web applications, particularly if you are working with Vue.js, you might also want to check out the article titled Fixing Common Locomotive Scroll Issues in Vue Projects. You can find it at infinitejs.com/posts/fixing-locomotive-scroll-issues-vue.
Final Thoughts
In the ever-evolving world of web development, understanding how to resolve conflicts between libraries is vital. Focusing on effective integration methods can lead to a more seamless user experience. Remember that maintaining performance and usability should always be your primary objectives.
Whether you are a beginner or a seasoned Java developer, the strategies discussed will assist in creating a smoother, more efficient application that harnesses the full potential of Locomotive Scroll. Feel free to share your thoughts or any additional tips you may have in the comments below!