Simplifying REST Endpoint Creation with Apache Camel 2.14
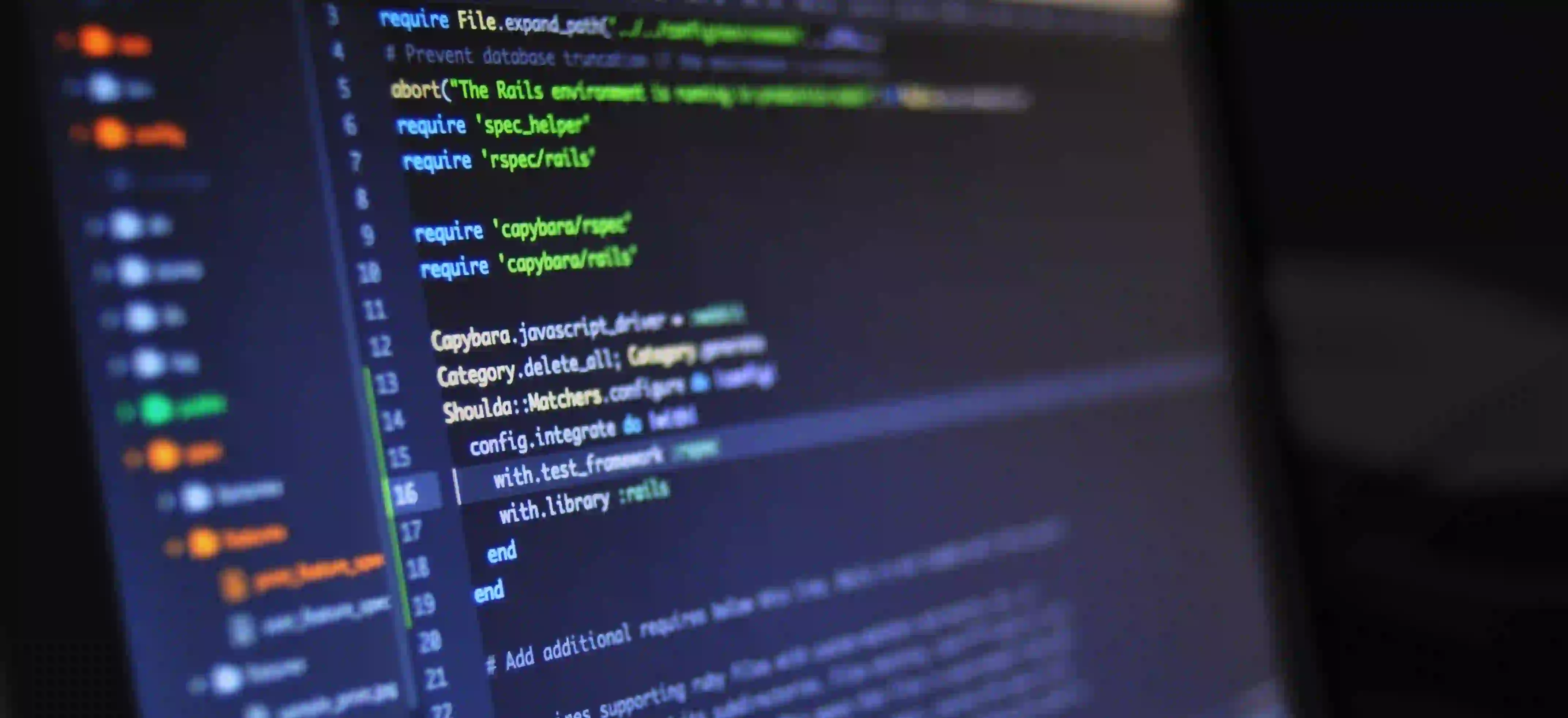
Simplifying REST Endpoint Creation with Apache Camel 2.14
Creating REST endpoints can often be a daunting task, especially when dealing with complex integrations. Fortunately, Apache Camel 2.14 has simplified this process with its added capabilities and streamlined features. This blog post will guide you through simplifying REST endpoint creation using Apache Camel, enhancing your development workflow and enabling rapid application development.
What is Apache Camel?
Apache Camel is an open-source integration framework that allows developers to create and manage integrated applications using a variety of protocols and data formats. With its consistent API and numerous components, it enables seamless integration across systems. The release of Camel 2.14 introduced many features that enhance REST endpoint creation, including better support for RESTful services and simplified configuration.
Why Use Apache Camel for REST Endpoints?
-
Simplicity: With Camel’s DSL (Domain Specific Language), you can define REST endpoints in a few simple lines of code.
-
Flexibility: It supports various protocols, including HTTP, HTTPS, and WebSocket, making it easy to integrate with different services.
-
Productivity: Rapidly create microservices with Camel's powerful routing capabilities while managing your APIs in a structured way.
-
Community and Resources: There is a vast community and extensive documentation to support developers during implementation.
REST DSL in Camel 2.14
The REST DSL in Camel 2.14 makes defining REST APIs seamless. This version provides a simple way to declare REST endpoints without intricate configuration or tedious boilerplate code. Let's take a look at how to get started with it.
Setting Up Your Project
To create a RESTful service with Apache Camel, you will first need an environment set up. You can start with a simple Maven project. Here is a brief setup guide:
- Create a new Maven project.
- Add the necessary dependencies in your
pom.xml
file:
<dependency>
<groupId>org.apache.camel</groupId>
<artifactId>camel-core</artifactId>
<version>2.14.0</version>
</dependency>
<dependency>
<groupId>org.apache.camel</groupId>
<artifactId>camel-http</artifactId>
<version>2.14.0</version>
</dependency>
<dependency>
<groupId>org.apache.camel</groupId>
<artifactId>camel-rest</artifactId>
<version>2.14.0</version>
</dependency>
<dependency>
<groupId>org.apache.camel</groupId>
<artifactId>camel-jetty</artifactId>
<version>2.14.0</version>
</dependency>
- Create a Java class to define your REST routes.
Defining REST Endpoints
Now, let’s define a simple REST API using Camel’s REST DSL. We will create a basic "Hello World" service that responds to GET requests.
import org.apache.camel.builder.RouteBuilder;
import org.apache.camel.component.rest.RestComponent;
import org.apache.camel.main.Main;
public class RestApiExample {
public static void main(String[] args) throws Exception {
Main main = new Main();
main.configure().addRoutesBuilder(new RestRouteBuilder());
main.start();
}
}
class RestRouteBuilder extends RouteBuilder {
@Override
public void configure() {
// Define REST API using REST DSL
restConfiguration()
.component("jetty")
.host("localhost")
.port(8080);
rest("/hello")
.get().to("direct:hello");
from("direct:hello")
.setBody(constant("Hello World!"));
}
}
Explanation of the Code
-
Main Application Class: We start the application with the
main
method, configuringCamel Main
to include our route builder. -
REST Configuration: The
restConfiguration()
method specifies the component (Jetty) and the host and port on which our service will run. -
Defining the REST Endpoint:
rest("/hello")
: Defines the base URI for the service..get()
: Specifies that this endpoint will respond to GET HTTP requests..to("direct:hello")
: Forwards requests to a direct component.
-
Consuming the Request: The
from("direct:hello")
listens for requests sent to "direct:hello". We set the body of the response usingsetBody(constant("Hello World!"))
, effectively returning "Hello World!" when the endpoint is called.
Testing the REST Endpoint
You can easily test the REST endpoint using tools like Postman or simply through your web browser. Accessing http://localhost:8080/hello
will return:
Hello World!
Benefits of Using REST DSL in Camel
-
Readability: The DSL syntax is clear and easy to understand.
-
Reusability: Routes and components are modular and can be reused across different projects.
-
Maintainability: Simplifying endpoint configuration reduces the worries around upkeep as your application scales.
Enhancing Your REST API
Now that you have a basic REST API, let's expand its functionality. We can add a POST endpoint that accepts a JSON body.
Updated Code for POST functionality
rest("/greet")
.post().to("direct:greet");
from("direct:greet")
.unmarshal().json(JsonLibrary.Jackson, MyRequest.class)
.process(exchange -> {
MyRequest request = exchange.getIn().getBody(MyRequest.class);
String greeting = "Hello " + request.getName() + "!";
exchange.getIn().setBody(greeting);
});
Explanation of the Additional Code
-
New REST Endpoint: The line
rest("/greet")
creates a new REST endpoint for a POST request. -
Deserialization: Using
unmarshal().json(JsonLibrary.Jackson, MyRequest.class)
allows us to convert incoming JSON into a Java object. -
Processing the Request: We then retrieve the name from the request and create a personalized greeting.
Creating the MyRequest Class
Here's a simple Java class for handling the incoming request data:
public class MyRequest {
private String name;
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
}
Testing the POST Endpoint
Using Postman, you can send a POST request to http://localhost:8080/greet
with a JSON body like:
{
"name": "Alice"
}
You should receive a response:
Hello Alice!
Lessons Learned
Apache Camel 2.14 simplifies the creation of REST endpoints, enabling developers to build microservices efficiently. This versatile framework, with its clean DSL and rich component ecosystem, is ideal for modern integration scenarios.
By applying the REST DSL to create endpoints, you reduce boilerplate code while maintaining a clear and coherent API structure. For more advanced user scenarios, referring to the official Apache Camel documentation will provide deeper insights and examples.
In summary, with just a few lines of code, you can have robust and maintainable RESTful services up and running. You can now focus on enhancing business logic rather than getting bogged down in complex integration tasks. Happy coding!
For further learning about REST design best practices, check out this guide on RESTful web services which offers valuable insights into building effective REST APIs.
Ready to build your next service with Apache Camel? Let's dive deeper together!