Overcoming Proxy Design Pattern Pitfalls in Software Development
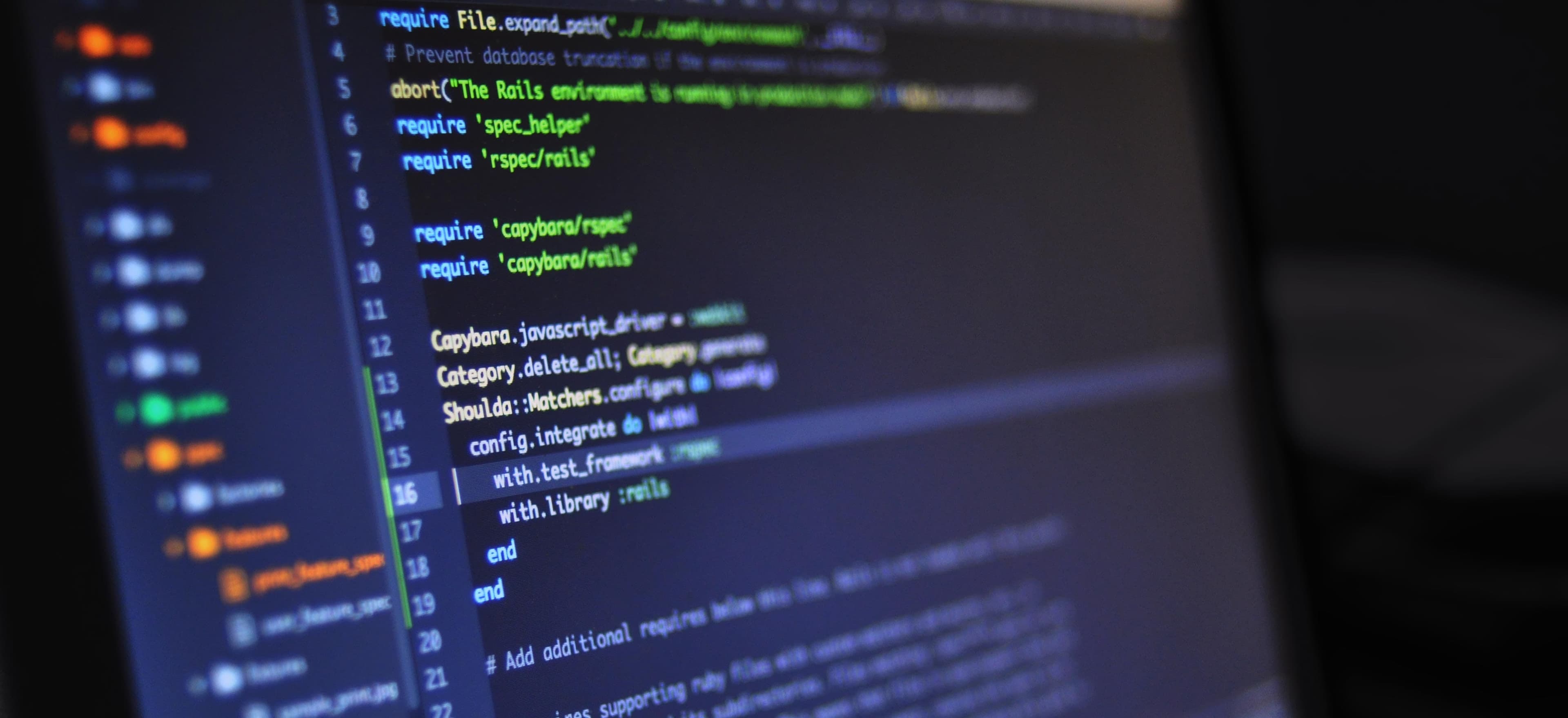
- Published on
Overcoming Proxy Design Pattern Pitfalls in Software Development
The Proxy Design Pattern is an essential construct within object-oriented programming, particularly in Java. It enables control over the actions of objects by using a placeholder or surrogate. However, like any design pattern, it presents several pitfalls that developers must navigate. In this blog post, we will dissect the Proxy Design Pattern, identify common pitfalls, and explore how to overcome these challenges effectively.
What is the Proxy Design Pattern?
The Proxy Design Pattern provides a surrogate or placeholder for another object to control access to it. This can be beneficial in various scenarios such as lazy loading, access control, logging, or even implementing remote procedure calls (RPC).
Components of the Proxy Design Pattern
- Subject Interface: This defines the common interface for RealSubject and Proxy.
- RealSubject: This represents the real object in the system.
- Proxy: This holds a reference to the RealSubject and implements the Subject interface.
Code Example
Here’s a basic implementation of the Proxy Design Pattern in Java:
// Subject Interface
public interface Image {
void display();
}
// RealSubject
public class RealImage implements Image {
private String fileName;
public RealImage(String fileName) {
this.fileName = fileName;
loadImageFromDisk();
}
private void loadImageFromDisk() {
System.out.println("Loading " + fileName);
}
@Override
public void display() {
System.out.println("Displaying " + fileName);
}
}
// Proxy
public class ProxyImage implements Image {
private RealImage realImage;
private String fileName;
public ProxyImage(String fileName) {
this.fileName = fileName;
}
@Override
public void display() {
if (realImage == null) {
realImage = new RealImage(fileName);
}
realImage.display();
}
}
Explanation
- RealImage: This class performs the heavy lifting. It loads and displays the image.
- ProxyImage: This class acts as a placeholder. It holds a reference to RealImage and controls access. It loads the RealImage only when needed.
By employing the Proxy Design Pattern, you can reduce resource consumption through lazy loading, thus enhancing performance.
Common Pitfalls of Proxy Design Pattern
With the Proxy Design Pattern comes certain embedded challenges. Below are some common pitfalls developers encounter:
1. Overhead of Proxy Objects
The introduction of Proxy objects increases the number of objects in the system. This can lead to significant memory usage. Proxies create overhead that may not always justify their benefits.
Solution
- Analyze the necessity of proxies for each scenario. Use proxies only when absolute control is required. In situations where direct access to RealSubject suffices, avoid implementing proxies.
2. Complexity
Adding proxy classes can increase the complexity of the application. This may lead to confusion, especially for new developers who might not understand the pattern immediately.
Solution
- Maintain clear documentation regarding the purpose of using Proxy and how it interacts with RealSubject. Document the flow of data and control clearly. Good documentation reduces onboarding time for new team members.
3. Performance Issues
In some cases, proxies can lead to performance degradation, especially when remote calls are involved or when excessive method calls are handled.
Solution
- Implement caching mechanisms where appropriate. This minimizes repeated calls to the RealSubject. With caching, performance can improve significantly.
4. Tight Coupling
Proxies can end up tightly coupled with their RealSubject, leading to difficulties in maintaining the code.
Solution
- Define interfaces clearly. Use dependency injection frameworks like Spring to manage lifecycles and dependencies more efficiently.
5. Violation of Liskov Substitution Principle
Not correctly implementing interfaces may lead to objects in code using the Proxy being treated differently from their RealSubject counterparts.
Solution
- Ensure that Proxy adheres to the same interface defined by the Subject. This guarantees a seamless interchangeability of the types.
Best Practices for Using the Proxy Design Pattern
In order to leverage the Proxy Design Pattern effectively, consider adopting the following best practices:
1. Define Clear Responsibilities
Break down functionalities and assign clear responsibilities. Each class should have a singular focus: the RealSubject should focus on its core functionality, while the Proxy should manage access control.
2. Implement Lazy Loading Wisely
Lazy loading is often the primary reason for adopting proxies. However, ensure that the delay in object creation does not delay critical operations or degrade user experience.
3. Utilize Design Patterns Wisely
Loading the Proxy Design Pattern with other design patterns like Decorator or Factory can create a powerful architecture. For example, using a Factory to create Proxies allows for greater flexibility.
4. Optimize Method Calls
When possible, aggregate multiple calls into a single robust call through the Proxy. This will minimize the performance hit associated with the overhead of increased object management.
Closing the Chapter
The Proxy Design Pattern is a powerful tool in software development, particularly when carefully considered and implemented. While it can introduce certain complexities and overheads, understanding the pitfalls and adhering to best practices can harness the full potential of this pattern.
By opting for well-defined interfaces, documenting design choices, and optimizing for performance, developers can effectively implement the Proxy Design Pattern while avoiding common mistakes.
For additional reading about design patterns in Java, take a look at Design Patterns: Elements of Reusable Object-Oriented Software, a foundational text in this domain.
Feel free to share your experiences and insights while working with the Proxy Design Pattern in the comments below! Your thoughts on overcoming these pitfalls could help fellow developers navigate their own developmental journeys.
Checkout our other articles