Debugging Akka: Master Actor Logging for Effective Testing
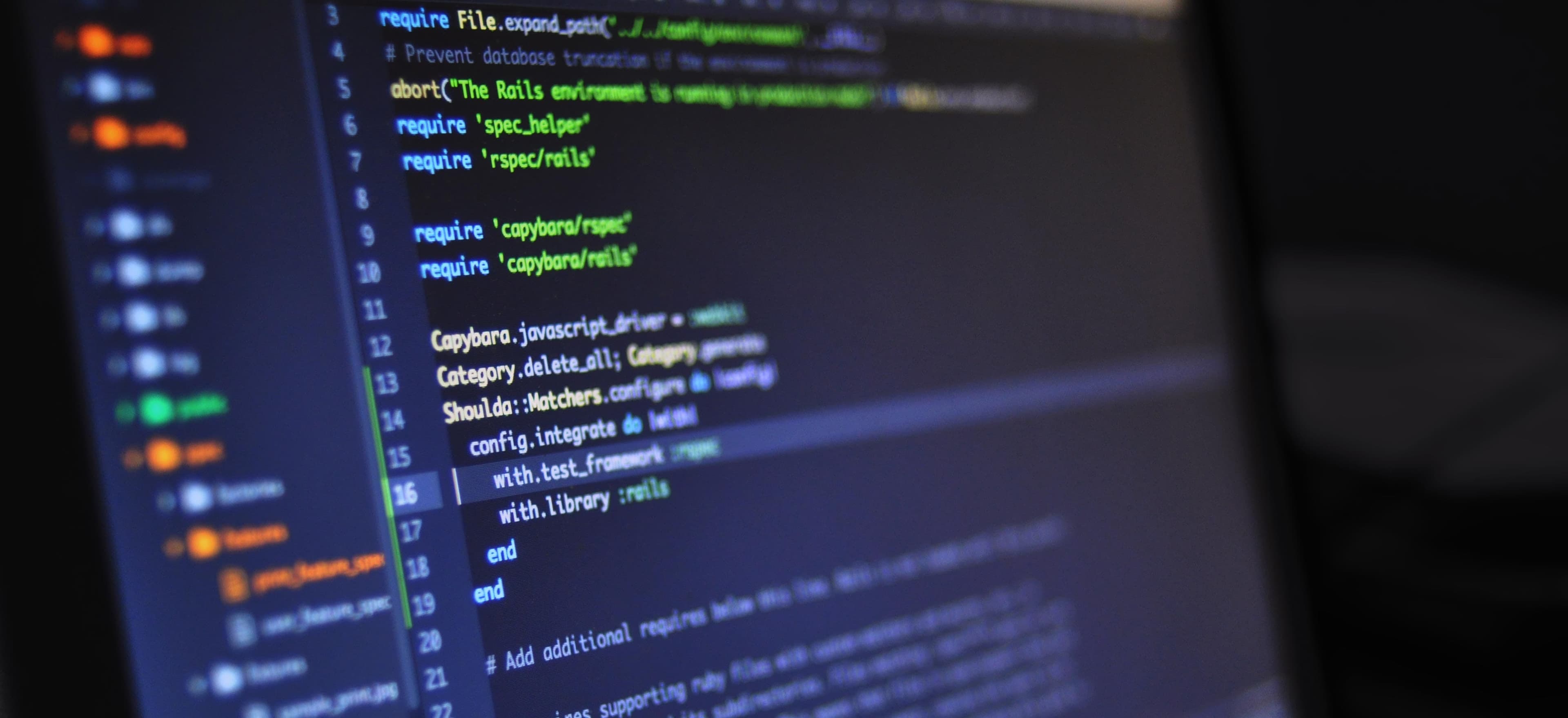
- Published on
Debugging Akka: Master Actor Logging for Effective Testing
When it comes to building concurrent applications in Java, Akka stands out as a powerful toolkit. Its actor model simplifies handling multiple tasks in a distributed environment. However, as with any complex system, debugging can be a daunting task. Thus, mastering logging in Akka is not just beneficial but essential for effective testing and debugging.
In this blog post, we will delve into the intricacies of Akka logging, how to implement it effectively, and best practices for ensuring your actor systems remain manageable and easy to debug.
Understanding Akka's Actor Model
Before we dive into logging, let’s briefly revisit the actor model in Akka. The actor model is designed for scalable and fault-tolerant systems. Each actor is an independent concurrent entity that communicates with other actors through messages.
Why Use Logging in Actors?
- State Tracing: Understanding the flow of data within an actor can illuminate faults or unexpected states.
- Error Detection: Logging exceptions that occur during message processing can help pinpoint failures.
- System Behavior: Monitoring how actors interact gives insights into the overall system performance.
Setting up Akka Logging
Akka has built-in support for logging, leveraging various logging frameworks like SLF4J, Log4j, or Logback. Below, we will use SLF4J in our examples due to its popularity and versatility.
Step 1: Adding Dependencies
First, ensure you have included necessary dependencies in your pom.xml
if you're using Maven:
<dependency>
<groupId>com.typesafe.akka</groupId>
<artifactId>akka-actor_2.12</artifactId>
<version>2.6.18</version>
</dependency>
<dependency>
<groupId>org.slf4j</groupId>
<artifactId>slf4j-api</artifactId>
<version>1.7.30</version>
</dependency>
<dependency>
<groupId>ch.qos.logback</groupId>
<artifactId>logback-classic</artifactId>
<version>1.2.3</version>
</dependency>
Note: Ensure you adapt the Scala version to match your project's needs.
Step 2: Configuring Logback for Akka
Next, configure Logback. Create a logback.xml
file in your resource directory:
<configuration>
<appender name="STDOUT" class="ch.qos.logback.core.ConsoleAppender">
<encoder>
<pattern>%d{yyyy-MM-dd HH:mm:ss} %-5level [%thread] %logger{36} - %msg%n</pattern>
</encoder>
</appender>
<root level="info">
<appender-ref ref="STDOUT" />
</root>
</configuration>
This configuration outputs log messages to the console. The chosen logging level can be adjusted according to your needs.
Implementing Logging in Actors
Example Actor Implementation
Here’s a basic actor implementation showing how to utilize logging.
import akka.actor.AbstractActor;
import akka.event.Logging;
import akka.event.LoggingAdapter;
public class MyActor extends AbstractActor {
private final LoggingAdapter log = Logging.getLogger(getContext().getSystem(), this);
@Override
public Receive createReceive() {
return receiveBuilder()
.match(String.class, message -> {
log.info("Received message: {}", message);
// simulate processing
processMessage(message);
})
.matchAny(o -> log.warning("Received unknown message"))
.build();
}
private void processMessage(String message) {
try {
// processing logic here
log.debug("Processing message...");
// Simulated potential error
if ("error".equals(message)) {
throw new RuntimeException("Simulated error processing message");
}
log.info("Successfully processed message: {}", message);
} catch (Exception e) {
log.error("Error processing message: {}", message, e);
}
}
}
Code Commentary:
-
LoggingAdapter:
LoggingAdapter
is instantiated to utilize Akka's logging capabilities. It’s passed the current actor's context. -
Logging Levels: We use
log.info()
andlog.debug()
to differentiate between normal operation messages and more granular logging for debugging. -
Error Handling: In
processMessage()
, a try-catch block captures exceptions and logs errors effectively.
Testing the Actor
To see this logging in action, you can create a simple Akka actor system and send messages.
import akka.actor.ActorRef;
import akka.actor.ActorSystem;
import akka.actor.Props;
public class Main {
public static void main(String[] args) {
ActorSystem system = ActorSystem.create("MyActorSystem");
ActorRef myActor = system.actorOf(Props.create(MyActor.class), "myActor");
myActor.tell("Hello, Akka!", ActorRef.noSender());
myActor.tell("error", ActorRef.noSender()); // Simulating an error
system.terminate();
}
}
Expected Output:
You can expect the console output to contain logs similar to:
2023-10-01 12:00:00 INFO [main] MyActor - Received message: Hello, Akka!
2023-10-01 12:00:00 DEBUG [main] MyActor - Processing message...
2023-10-01 12:00:00 INFO [main] MyActor - Successfully processed message: Hello, Akka!
2023-10-01 12:00:00 INFO [main] MyActor - Received message: error
2023-10-01 12:00:00 DEBUG [main] MyActor - Processing message...
2023-10-01 12:00:00 ERROR [main] MyActor - Error processing message: error
java.lang.RuntimeException: Simulated error processing message
...
Best Practices for Akka Logging
-
Define Logging Levels: Use different logging levels judiciously.
DEBUG
for verbose output,INFO
for operational logs,WARNING
for non-critical issues, andERROR
for failures. -
Use Mapped Parameters: Always prefer using parameters for logging rather than string concatenation to enhance performance and readability.
-
Avoid Over-Logging: Too much logging can decrease performance and make it challenging to find relevant information.
-
Structured Logging: Utilize structured logging where possible. Incorporating additional context (like user IDs or transaction IDs) can expedite troubleshooting.
-
Leverage External Tools: Tools like Akka Management can provide additional insights into the actor system's state without needing extensive manual logging.
To Wrap Things Up
Akka offers a robust framework for building concurrent applications, but effective logging is vital for debugging and testing. By mastering actor logging, you not only enhance your debugging capabilities but also gain insights that can lead to improved performance and reliability in your applications.
For further reading on Akka and logging, consider visiting the official Akka documentation or delve deeper into Scala logging techniques.
Navigating the complexities of distributed systems can be challenging, but with the right logging strategies in place, you'll be well-equipped to tackle any issue that comes your way. Happy coding!
Checkout our other articles