Unlocking Hibernate: Managing Second-Level Cache Efficiently
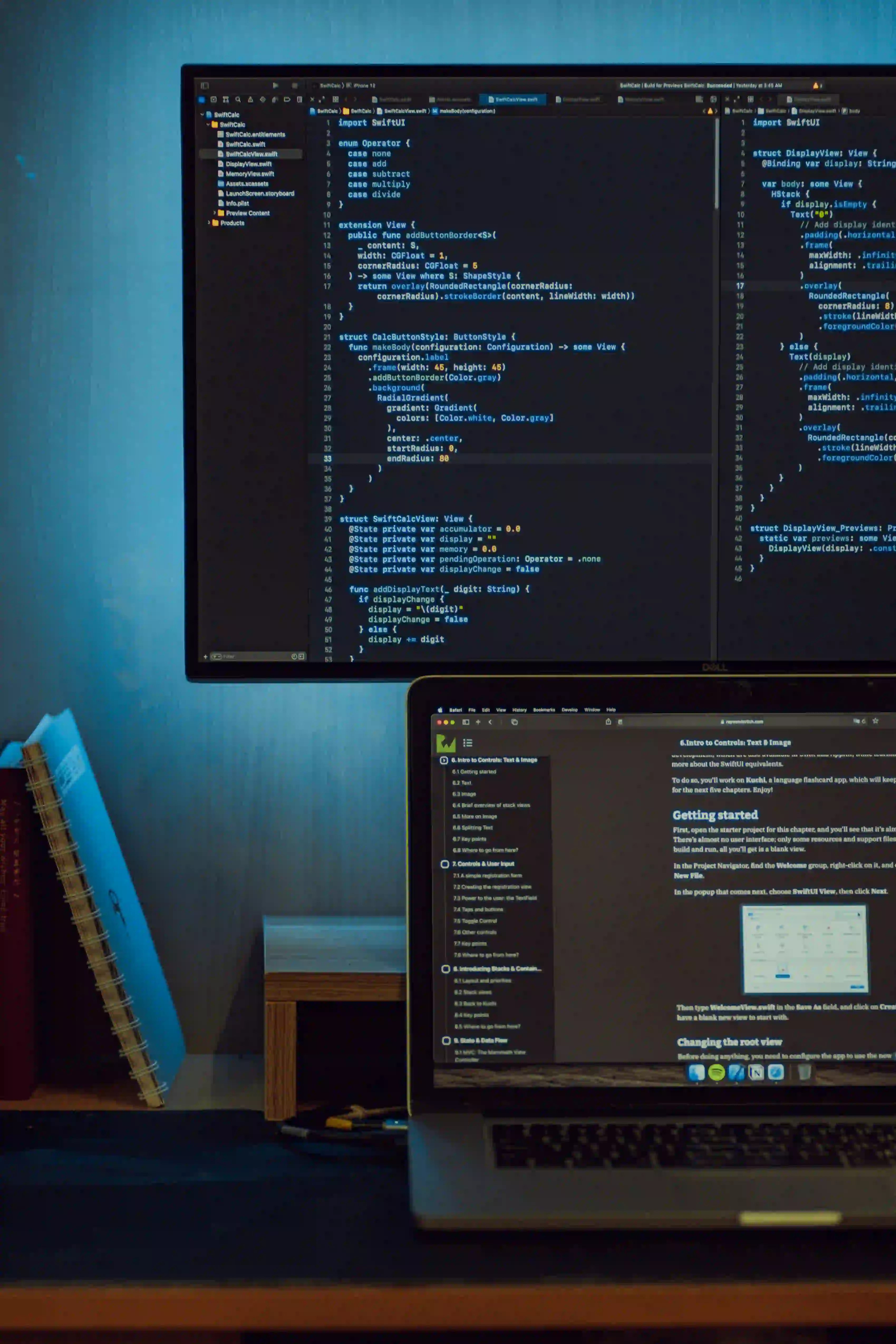
Unlocking Hibernate: Managing Second-Level Cache Efficiently
Hibernate is one of the most popular Object-Relational Mapping (ORM) frameworks in the Java ecosystem. When it comes to performance, caching is a critical feature that allows developers to optimize application speed and minimize database loads. Among the various caching strategies in Hibernate, the second-level cache stands out. This blog post explores how to manage the second-level cache effectively in Hibernate, enhancing application performance while maintaining data consistency.
What is Hibernate Second-Level Cache?
Before diving into the intricacies of second-level caching, let's clarify what it is. In Hibernate, caching is divided into two levels:
-
First-Level Cache: This is the default cache provided by Hibernate. It's session-specific, meaning that once the session is closed, all cached entities are discarded. This cache is fast and applies to a single transaction.
-
Second-Level Cache: This operates at a session factory level, allowing entities to be cached across sessions. The second-level cache can dramatically improve performance by reducing the frequency of database reads, particularly for read-heavy applications.
Why Use Second-Level Cache?
-
Performance Improvement: By caching frequently accessed data, applications can reduce the number of database queries, significantly improving response times.
-
Resource Optimization: With fewer queries hitting the database, you can optimize database resources, potentially reducing costs.
-
Scalability: Caching allows applications to handle increased loads without overburdening the database, making it easier to scale.
How to Enable Hibernate Second-Level Cache
To utilize the second-level cache effectively, developers must follow several steps. Below are the key steps involved in enabling the second-level cache in Hibernate.
1. Configure the Hibernate Properties
First, you need to configure your Hibernate settings. Here’s a sample configuration using hibernate.cfg.xml
:
<hibernate-configuration>
<session-factory>
<!-- Other configurations -->
<!-- Enable second-level cache -->
<property name="hibernate.cache.use_second_level_cache">true</property>
<!-- Specify the cache provider -->
<property name="hibernate.cache.region.factory_class">org.hibernate.cache.jcache.JCacheRegionFactory</property>
<!-- Alternatively, use Ehcache -->
<!-- <property name="hibernate.cache.region.factory_class">org.hibernate.cache.ehcache.EhCacheRegionFactory</property> -->
<!-- Configure the cache provider settings -->
<property name="hibernate.cache.use_query_cache">true</property>
</session-factory>
</hibernate-configuration>
Commentary
- The
hibernate.cache.use_second_level_cache
property enables the second-level cache. - The
hibernate.cache.region.factory_class
property allows you to specify the cache provider. In this case, you can use JCache or Ehcache depending on your needs.
2. Annotate Your Entity Classes
Once you've configured Hibernate, the next step is to annotate your entity classes to enable caching.
import javax.persistence.Cacheable;
import javax.persistence.Entity;
import javax.persistence.Id;
@Entity
@Cacheable
@org.hibernate.annotations.Cache(usage = org.hibernate.annotations.CacheConcurrencyStrategy.READ_WRITE)
public class User {
@Id
private Long id;
private String name;
// Getters and setters here
}
Commentary
- The
@Cacheable
annotation allows Hibernate to cache instances of this entity. - The
@org.hibernate.annotations.Cache
annotation specifies the caching strategy. In this example,READ_WRITE
is used, which is suitable for entities that are frequently read and occasionally updated.
3. Configuring Cache Provider
Depending on the cache provider (Ehcache, Infinispan, etc.), you'll need configuration files. If you are using Ehcache, you need an ehcache.xml
file:
<ehcache>
<defaultCache
maxElementsInMemory="10000"
eternal="false"
timeToIdleSeconds="120"
timeToLiveSeconds="120"
overflowToDisk="false" />
<cache name="com.example.User"
maxEntriesLocalHeap="1000"
eternal="false"
timeToIdleSeconds="300"
timeToLiveSeconds="600" />
</ehcache>
Commentary
- The
<defaultCache>
tag configures default caching behavior. - The
<cache>
tag specifically configures the cache for theUser
entity, which allows for more granular control over caching behavior.
Best Practices for Managing Second-Level Cache
When working with the second-level cache in Hibernate, keeping performance and consistency in mind is crucial. Here are some best practices for effective management:
1. Choose the Right Cache Strategy
Hibernate provides various caching strategies, including:
- READ_ONLY: Suitable for read-only data that will never change.
- READ_WRITE: Ideal for data that can be read and modified.
- NONSTRICT_READ_WRITE: Useful for entities that can have stale data, but do not require strict consistency.
2. Monitor Cache Effectiveness
Use monitoring tools such as JMX (Java Management Extensions) or other profiling tools to track cache hit and miss ratios. Analyzing this data helps identify caching inefficiencies and areas for improvement.
3. Implement Cache Eviction Policies
Over time, cached data can become stale. Establish a cache eviction policy that defines when data should be removed from the cache to maintain coherence. For long-lived applications, consider implementing a strategy to refresh stale entries without complete eviction.
4. Test with Different Load Patterns
Simulating various load patterns in your application can present insights into how effective your caching strategy is. Performance testing tools like JMeter can be instrumental in this regard.
5. Maintain Consistency
Caching introduces complexity in maintaining consistent data. Ensure that all applications that interact with your database are aware of any caching mechanisms to avoid unexpected behaviors.
Key Takeaways
Hibernate's second-level cache can significantly enhance the performance of your applications when configured correctly. Leveraging this feature enables scaling, reduces database load, and ultimately leads to faster response times. However, it’s crucial to implement effective strategies for cache usage. By choosing the right caching strategies, monitoring effectiveness, managing eviction policies, and ensuring consistency, developers can unlock the full potential of Hibernate's caching capabilities.
Incorporating second-level caching into your Hibernate applications may seem daunting, but with the best practices outlined, you can harness its power for better application performance.
Further Reading
For more in-depth information about Hibernate caching and configurations, consider reading the following resources:
- Hibernate ORM Documentation
- Ehcache Documentation
- JPA Caching Strategies
By implementing the strategies discussed in this blog post, you can ensure that your application remains performant and scalable, keeping user satisfaction at the forefront. Happy coding!