The Hidden Pitfalls of Using Java Enums in Large Projects
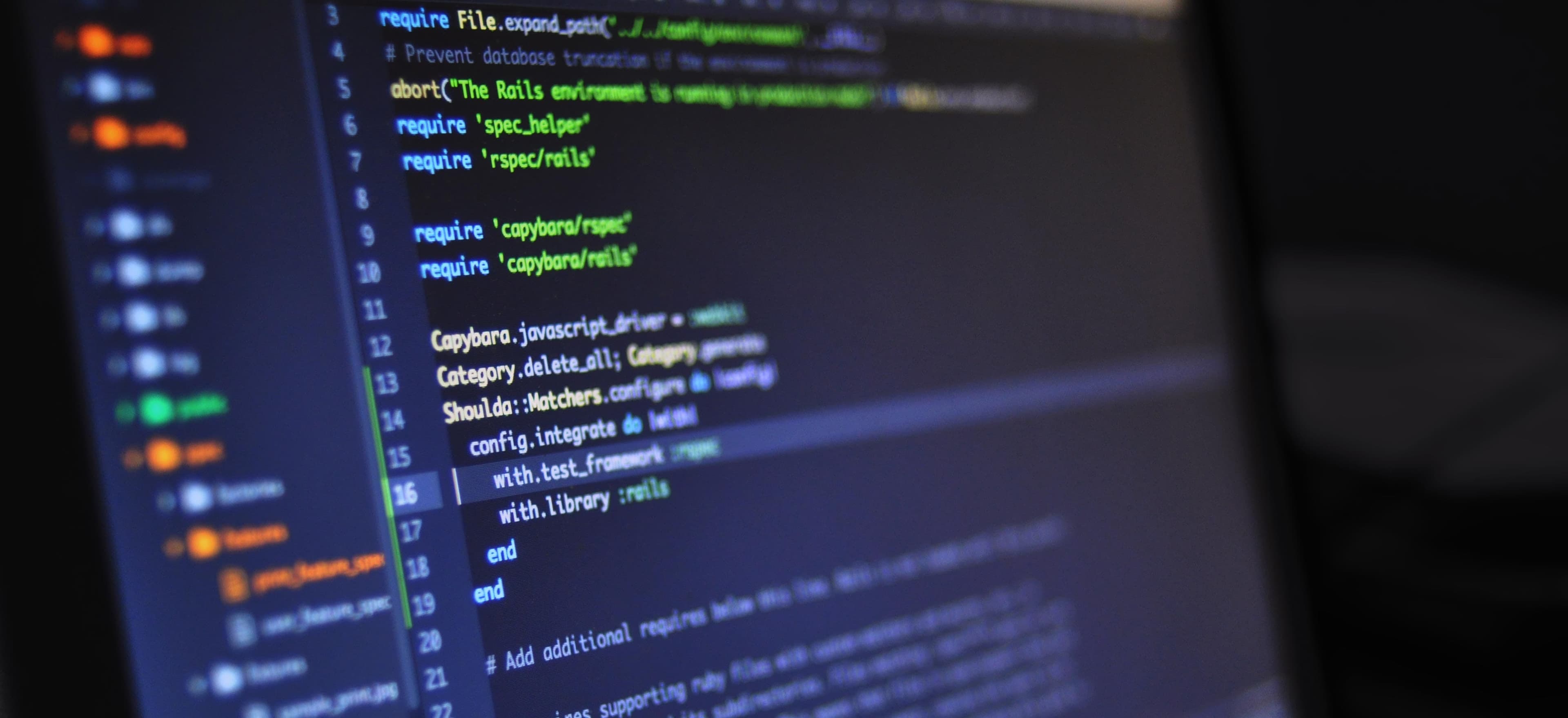
- Published on
The Hidden Pitfalls of Using Java Enums in Large Projects
Java's Enum feature, introduced in Java 5, offers a powerful way to create type-safe enumerations. Enums provide a clear and structured way to define a fixed set of constants. They improve code readability and maintainability, making them a popular choice among developers. However, as with any feature, using enums in large projects can lead to pitfalls that, if not recognized, may result in performance issues, maintenance challenges, and even design flaws.
In this blog post, we will dive deep into these pitfalls, explore the best practices for using enums, and illustrate some concepts with code snippets to provide clarity.
Understanding Enums
Before we reach the potential pitfalls, let’s quickly recap what enums are and how to use them. An enum is a special Java type used to define collections of constants. It can be defined as follows:
public enum Day {
SUNDAY, MONDAY, TUESDAY, WEDNESDAY, THURSDAY, FRIDAY, SATURDAY
}
Why Use Enums?
- Type Safety: Enums provide compile-time checking, preventing invalid values from being assigned.
- Readability: Using enums makes the code more readable and understandable.
- Methods and Fields: Enums can have methods and fields, allowing for behavior to be encapsulated within the enum itself.
However, despite these advantages, there are pitfalls that developers must be aware of.
Common Pitfalls of Using Enums in Large Projects
1. Overusing Enums for Every Constant
Enums might seem like the perfect solution for every fixed set of constants, but using them excessively or inappropriately can lead to unnecessary complexity.
Example:
Let’s say you are creating an enum for error codes:
public enum ErrorCode {
SUCCESS, FAILURE, INVALID_INPUT, TIMEOUT
}
While it works, consider whether these values change often and if they need to be centralized. A constant class may be more appropriate, especially if error codes are numerous and vary based on context.
Solution:
Use enums judiciously. Not every set of constants needs to be an enum. If the values are likely to change or expand, use constant values or another data structure, like a Map.
2. Performance Concerns
Enums in Java are more memory-intensive than simple constants. When enums are loaded, each instance is created only once, which saves space for large numbers of instances, but the overhead can be significant.
Example:
For a large set of constant values:
public enum Color {
RED, GREEN, BLUE, YELLOW, ORANGE, PURPLE, PINK // and many more...
}
The enum may increase the memory footprint of the application. Always evaluate performance needs before creating large enums.
3. Inflexibility and Evolution
Enums are static by nature. If your application evolves and the requirements change, modifying enums can be cumbersome. Adding a new constant can break existing code and create maintenance headaches.
Example:
Consider if you need to handle user roles:
public enum UserRole {
ADMIN, USER, GUEST
}
If you decide to introduce more roles down the line, it can lead to issues. Future developers may need to sift through various parts of the codebase to adjust logic that uses these roles.
Solution:
Whenever extensibility is a need, prefer using interfaces or classes to represent variants. It offers more flexibility than enums.
4. Serialization Challenges
Enums are serializable by default, which can cause complications during the serialization process, especially when enums are modified over time. This can lead to InvalidClassException
.
Example:
If your enum changes:
public enum Status {
ACTIVE, INACTIVE, SUSPENDED
}
If "SUSPENDED" is later replaced with "BLOCKED", old serialized versions will throw an exception.
Solution:
Implement a static readObject method to handle versioning properly:
private void readObject(ObjectInputStream stream) throws IOException, ClassNotFoundException {
stream.defaultReadObject();
// Custom handling to maintain backward compatibility.
}
5. Lack of Metadata
Enums do not support dynamic behavior, which can limit their effectiveness in certain applications. Suppose you want to associate metadata with enums; doing so becomes cumbersome.
Example:
Consider trying to add attributes like descriptions:
public enum Country {
USA("United States"),
CANADA("Canada");
private final String description;
Country(String description) {
this.description = description;
}
public String getDescription() {
return description;
}
}
Although it works, it creates clutter and can be difficult to manage and extend.
Solution:
For extending enum-like functionality, consider using a combination of classes/interfaces with constant fields for shared values, providing more flexibility.
6. Testing Complications
Enums can complicate testing due to the static nature of their implementation. When enums are tightly coupled with business logic, mocking them can be challenging.
Example:
Imagine you have business logic tied to an enum:
public class Order {
private Status status;
public void updateStatus(Status status) {
this.status = status;
}
}
This scenario complicates unit tests where you need to manage the specific states.
Solution:
Use dependency injection to decouple enum usage from your business logic, allowing for more manageable unit testing.
Best Practices for Using Enums
Despite these pitfalls, enums can be very useful when implemented correctly. Here are some best practices:
- Use Enums for Fixed Sets: Enums should only be used for fixed and predictable sets of values.
- Avoid Complex Logic: Refrain from placing too much logic inside enums; keep them simple.
- Document Changes: When changing enums, ensure that documentation is updated to reflect these changes.
- Consider Alternatives: Think about whether an interface or a class might be more appropriate based on your specific needs.
My Closing Thoughts on the Matter
Java enums are powerful tools that can significantly enhance code readability and provide type safety. However, when used in large projects, they come with hidden pitfalls that may affect performance, flexibility, and maintainability.
By recognizing these pitfalls and adhering to best practices, you can leverage enums effectively without falling victim to their downsides. A cautious and informed approach can lead to a well-structured, maintainable codebase, ensuring your project remains scalable and adaptable to changes.
To explore more about Java enums and to read about best coding practices, check out the official Java Enums Documentation.
Do you have any experiences or insights related to using enums in your projects? Share your thoughts in the comments below!