Exploring the Hidden Challenges of Java 9 Feature Development
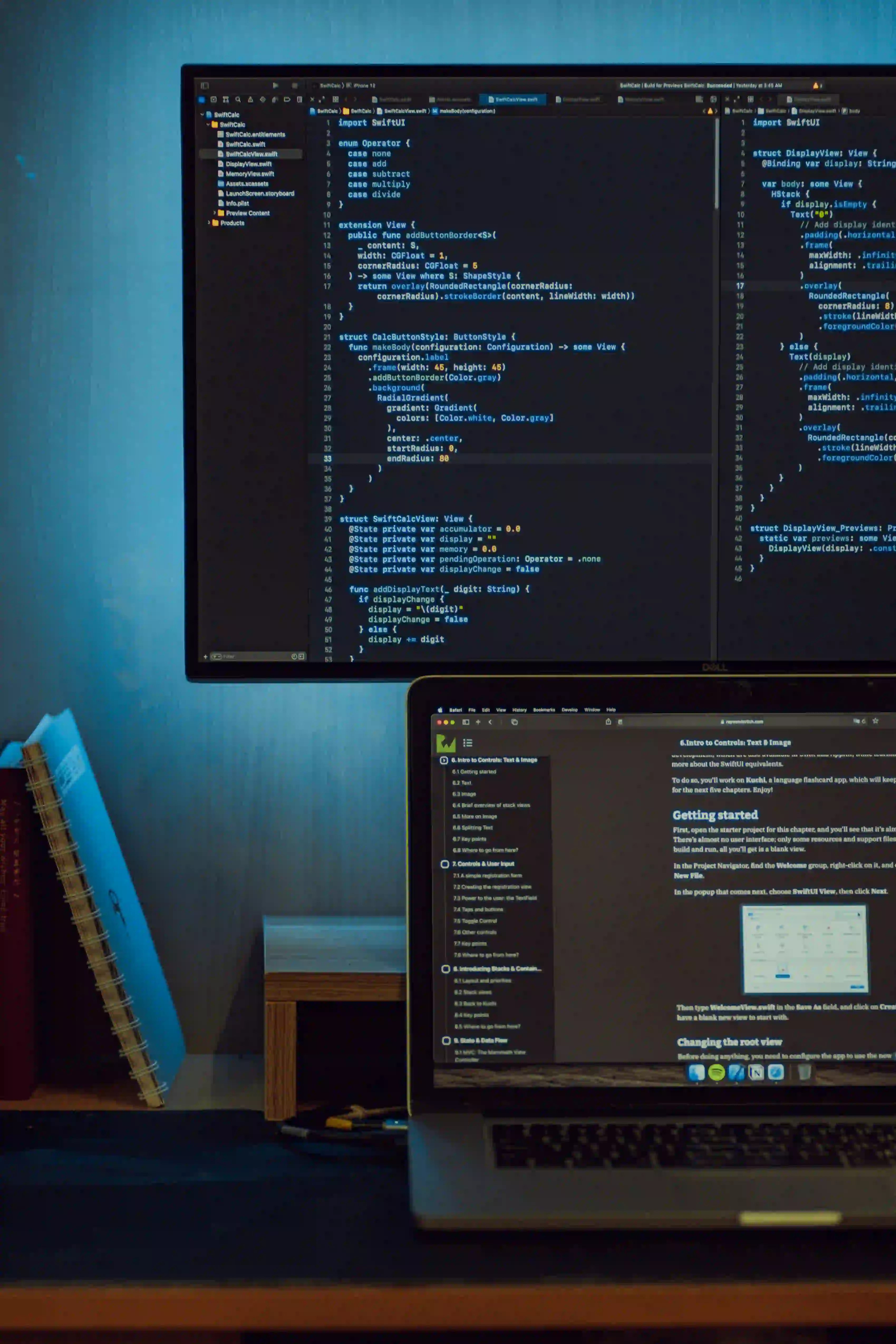
Exploring the Hidden Challenges of Java 9 Feature Development
Java, a venerable programming language, has seen numerous updates since its inception. Java 9, released in September 2017, brought forth a remarkable collection of features and enhancements. However, alongside these improvements came inherent challenges, particularly for feature development.
In this blog post, we will delve deep into these hidden challenges, examining key features, and offering practical code snippets. By the end, you should not only have a better understanding of Java 9 but also improved strategies to tackle its complexities.
What’s New in Java 9?
Before we plunge into the challenges, let’s quickly recap some of the major features introduced in Java 9:
- Modular System (Project Jigsaw)
- JShell
- Improved Javadoc
- Reactive Streams
- Stack Walking API
Modular System (Project Jigsaw)
Perhaps the most significant change was the introduction of the Java Platform Module System (JPMS) or Project Jigsaw. This modularity allows developers to create a better-organized codebase by grouping related code into modules.
Example of Creating a Module
Here’s a simple example of defining a module:
module com.example.myapp {
exports com.example.myapp.utils;
}
The exports directive shows which packages are accessible to other modules. This feature enhances encapsulation and maintenance but comes with challenges.
Challenges with Modular System
-
Refactoring Legacy Code: Transitioning existing applications to a modular format can be daunting. Legacy projects often comprise tightly coupled components, making it difficult to separate them into distinct modules.
- Tip: Start small by identifying and encapsulating the most cohesive parts of your legacy codebase.
-
Dependency Management: Managing dependencies across modules can lead to confusion. Version conflicts and visibility issues may arise if not handled properly.
- Tip: Use tools like Maven or Gradle that support modular builds to reduce dependency headaches.
-
Understanding Accessibility: You'll need to grasp the nuances of module accessibility rules. Not all packages are available by default, and mistakes can lead to runtime issues that are difficult to debug.
JShell
JShell is an interactive tool for evaluating Java expressions, allowing developers to experiment without compiling an entire program.
Example of Using JShell
$ jshell
jshell> int a = 10;
jshell> int b = 20;
jshell> System.out.println(a + b);
30
JShell is perfect for testing snippets, but its adoption can present challenges:
Challenges with JShell Usage
-
Limited to Simple Tasks: Developers tend to use JShell for lightweight testing scenarios. Exploring complex scenarios can be cumbersome since JShell doesn’t inherently support multi-file programs.
- Solution: Pair JShell with your IDE for a more enhanced experience when working on complex tasks.
-
Transitioning Back to Regular Development: Moving from JShell back to traditional Java programming can cause a cognitive shift or disconnect.
- Suggestion: Use JShell for quick ideas but avoid over-relying on it for bigger projects.
Improved Javadoc
Java 9 comes equipped with a revamped Javadoc tool, which now includes a search capability and HTML5 support. This should make it easier to generate and navigate documentation.
Example of Generating Javadoc
$ javadoc -d doc -sourcepath src -subpackages com.example
This command will generate HTML documentation in the doc
directory for all files under com.example
. However, there are challenges in utilizing the new features:
Challenges with the New Javadoc
-
Javadoc Tags: You must ensure you are well-versed with new tags and annotations introduced in Java 9. Some developers may overlook necessary documentation due to the learning curve.
- Tip: Consult the Javadoc documentation regularly for updates.
-
Third-party Compatibility: Older projects using previously structured Javadoc may face compatibility issues.
- Solution: Gradually migrate documentation formats to align with Java 9, allowing time for adjustments.
Reactive Streams
Reactive programming emerged as a necessary paradigm for asynchronous data processing. Java 9 includes the Reactive Streams API, which offers basic infrastructure for building asynchronous, non-blocking applications.
Example of a Simple Reactive Stream
import java.util.concurrent.Flow;
public class SimpleSubscriber implements Flow.Subscriber<Integer> {
@Override
public void onSubscribe(Flow.Subscription subscription) {
subscription.request(1); // Request one item at a time
}
@Override
public void onNext(Integer item) {
System.out.println(item);
}
@Override
public void onError(Throwable throwable) {
throwable.printStackTrace();
}
@Override
public void onComplete() {
System.out.println("All items processed.");
}
}
While Reactive Streams can significantly improve application responsiveness, challenges exist:
Challenges with Reactive Programming
-
Learning Curve: Developers accustomed to traditional synchronous programming may find the reactive paradigm difficult to grasp initially.
- Tip: Integrate tutorials and practice examples to build familiarity with concepts and patterns.
-
Error Handling Complexities: Managing errors in a non-blocking manner requires a different approach than standard Java error handling.
- Suggestion: Follow industry best practices for error handling in reactive systems to minimize application downtime.
Stack Walking API
The Stack Walking API provides an alternative to the standard stack trace reporting. It allows developers to construct custom stack traces and filter the method calls for better insight.
Example of Using Stack Walking API
StackWalker walker = StackWalker.getInstance();
walker.forEach(frame -> System.out.println(frame.getMethodName()));
Although powerful, the Stack Walking API is not without its challenges:
Challenges with Stack Walking
-
Performance Implications: Improper use can lead to performance downsides, especially when capturing stack traces in a high-frequency logging environment.
- Recommendation: Use this feature judiciously, profiling your application to assess any performance impacts.
-
Complex Stack Filtering: Users may struggle to apply appropriate filters, resulting in irrelevant data.
Closing Remarks
Java 9 undoubtedly introduced features designed to facilitate modern software development. However, navigating the challenges presented by these features requires a proactive approach, clear understanding, and continuous learning.
By breaking down the complexities of Project Jigsaw, JShell, Improved Javadoc, Reactive Streams, and the Stack Walking API, you empower yourself to develop better, more robust Java applications.
To stay current with Java developments, consider following official resources, community forums, or even contribute to open-source Java projects! You can explore more about Java through Oracle's official documentation or join communities like Stack Overflow to share experiences, challenges, and solutions.
Embrace the hidden challenges and transform them into opportunities for growth in your development journey. Happy coding!
Feel free to reach out if you have any questions or need further clarification on any Java 9 features or challenges.