Mastering Dead-Simple Configuration: Common Pitfalls to Avoid
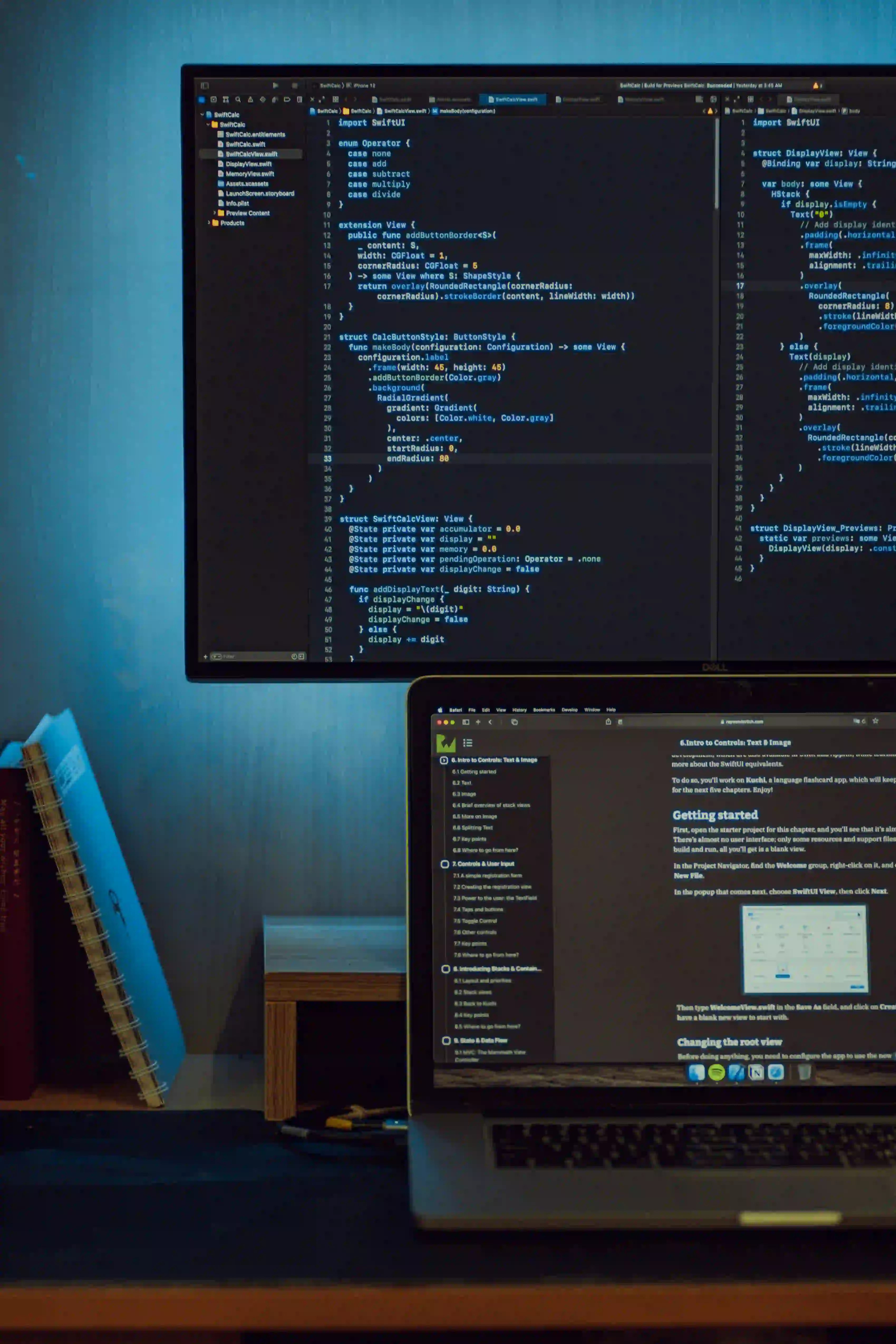
Mastering Dead-Simple Configuration: Common Pitfalls to Avoid
In the realm of software development, configuration often feels like a daunting task. But, it doesn't have to be. Java, being a robust programming language, thrives on configuration settings, which can define how applications behave in various environments. While there are many ways to configure a Java application, mastering a "dead-simple" approach can save developers both time and frustration. This blog post will explore common pitfalls in configuration management and how to avoid them, empowering you to write cleaner and more maintainable configurations.
Understanding Configuration in Java
Configuration is an essential aspect of any application. It dictates how components interact, how data is processed, and how the application behaves in different scenarios. In Java, configurations can come in various forms: properties files, XML files, or even through annotations and Java classes. Here’s a simple example of a properties file:
# server.properties
server.port=8080
server.host=localhost
logging.level=INFO
Why Use Properties Files?
Properties files are plain text files that store configuration data. They are easy to read and modify, making them a popular choice among developers. The java.util.Properties
class provides utility methods to access data stored in properties files efficiently.
Common Pitfalls in Configuration Management
When managing configurations, there are several pitfalls that developers often encounter. Addressing these early can make a significant difference in the maintainability and clarity of your application.
1. Hard-Coding Configuration Values
Hard-coding configuration values directly into your Java classes can lead to numerous issues. It limits flexibility and makes it difficult to change settings without modifying code.
Example of Hard-Coding
public class DatabaseConnection {
private final static String URL = "jdbc:mysql://localhost:3306/mydb";
private final static String USER = "root";
private final static String PASSWORD = "password";
// Database connection logic...
}
Why Avoid It?
Hard-coded values create a tight coupling between the application’s code and its configuration. This approach requires developers to recompile and redeploy code whenever a setting changes. Instead, use properties files or environment variables to externalize configuration.
Solution: Use Externalized Configuration
import java.io.InputStream;
import java.util.Properties;
public class DatabaseConfig {
private Properties properties = new Properties();
public DatabaseConfig() {
try (InputStream input = getClass().getClassLoader().getResourceAsStream("config.properties")) {
properties.load(input);
} catch (Exception e) {
e.printStackTrace();
}
}
public String getDatabaseUrl() {
return properties.getProperty("db.url");
}
}
2. Ignoring Environment-Specific Configurations
Different environments such as development, testing, and production often require distinct configurations. Failing to specify environment-specific settings can lead to deployment issues.
Example of a Uniform Configuration
# config.properties (not environment-specific)
db.url=jdbc:mysql://localhost:3306/mydb
Why Is This a Problem?
Using a single configuration file means that the same settings are applied regardless of the environment. For instance, connecting to a production database from a development environment can result in data corruption or loss.
Solution: Use Profiles or Environment Variables
Consider creating separate configuration files for each environment, or use environment variables. For example, you can define different property files like config-dev.properties
, config-test.properties
, and config-prod.properties
.
public class ConfigLoader {
private Properties properties = new Properties();
private String environment;
public ConfigLoader(String environment) {
this.environment = environment;
loadProperties();
}
private void loadProperties() {
try (InputStream input = getClass().getClassLoader().getResourceAsStream("config-" + environment + ".properties")) {
properties.load(input);
} catch (Exception e) {
e.printStackTrace();
}
}
}
3. Failing to Validate Configuration Settings
Configuration files may contain incorrect or harmful settings. Bypassing validation can lead to unexpected behavior or runtime errors.
Example of Not Validating
Imagine an application that connects to a database without validating the database URL.
Connection conn = DriverManager.getConnection(databaseConfig.getDatabaseUrl());
Why Is This Dangerous?
If the config file contains an invalid URL, your application will crash during startup or at an inopportune time. This could lead to user dissatisfaction and trust erosion.
Solution: Implement Configuration Validation
Always validate configurations before using them. You can create a simple method for common validations.
public void validateConfig() {
String dbUrl = properties.getProperty("db.url");
if (dbUrl == null || !dbUrl.startsWith("jdbc:")) {
throw new IllegalArgumentException("Invalid database URL");
}
}
4. Neglecting Comments and Documentation
Configurations may become complex over time, especially in larger applications. Neglecting to document settings can lead to confusion and time wasted.
Example of a Poorly Documented Configuration
# config.properties
db.url=jdbc:mysql://localhost:3306/mydb
db.user=admin
Why Is Documentation Important?
Without comments, it’s difficult to understand the purpose of specific settings. This obscurity makes it harder for new team members to onboard or modify the application.
Solution: Add Meaningful Comments
# config.properties
# Database connection URL
db.url=jdbc:mysql://localhost:3306/mydb
# Database user with appropriate permissions
db.user=admin
5. Not Leveraging Frameworks and Tools
Many frameworks like Spring Boot provide excellent facilities for configuration management. Ignoring these can lead to reinventing the wheel.
Example of Custom Configuration Management
Building your own configuration management from scratch can be unnecessary and error-prone.
Why Use Frameworks?
Using proven libraries allows you to leverage community support and established best practices.
Solution: Explore Spring Boot Configuration
In Spring Boot, you can use the @Value
annotation to inject configuration properties directly into your beans.
import org.springframework.beans.factory.annotation.Value;
import org.springframework.stereotype.Component;
@Component
public class DatabaseProperties {
@Value("${db.url}")
private String url;
@Value("${db.user}")
private String user;
// Further logic...
}
For more on Spring Boot's configuration capabilities, you can check the official documentation here.
Final Thoughts
Proper configuration management is crucial for the success of your Java applications. By avoiding common pitfalls like hard-coding values, neglecting validation, and disregarding documentation, you can create more maintainable and robust applications. Remember to leverage existing frameworks whenever possible to simplify your configuration management.
Stay proactive in managing your configurations, and not only will you make your application more resilient, but you’ll also ease future modifications. By mastering dead-simple configuration, you cultivate a smoother development process that benefits both you and your team.
For a deeper dive into configuration best practices across languages and frameworks, you can visit Configuration Management Best Practices.
This blog post aims to serve as a guide for both seasoned Java developers and newcomers, helping them navigate the often overlooked yet critical aspect of configuration management.