Understanding RGB Color Representation Issues in Java
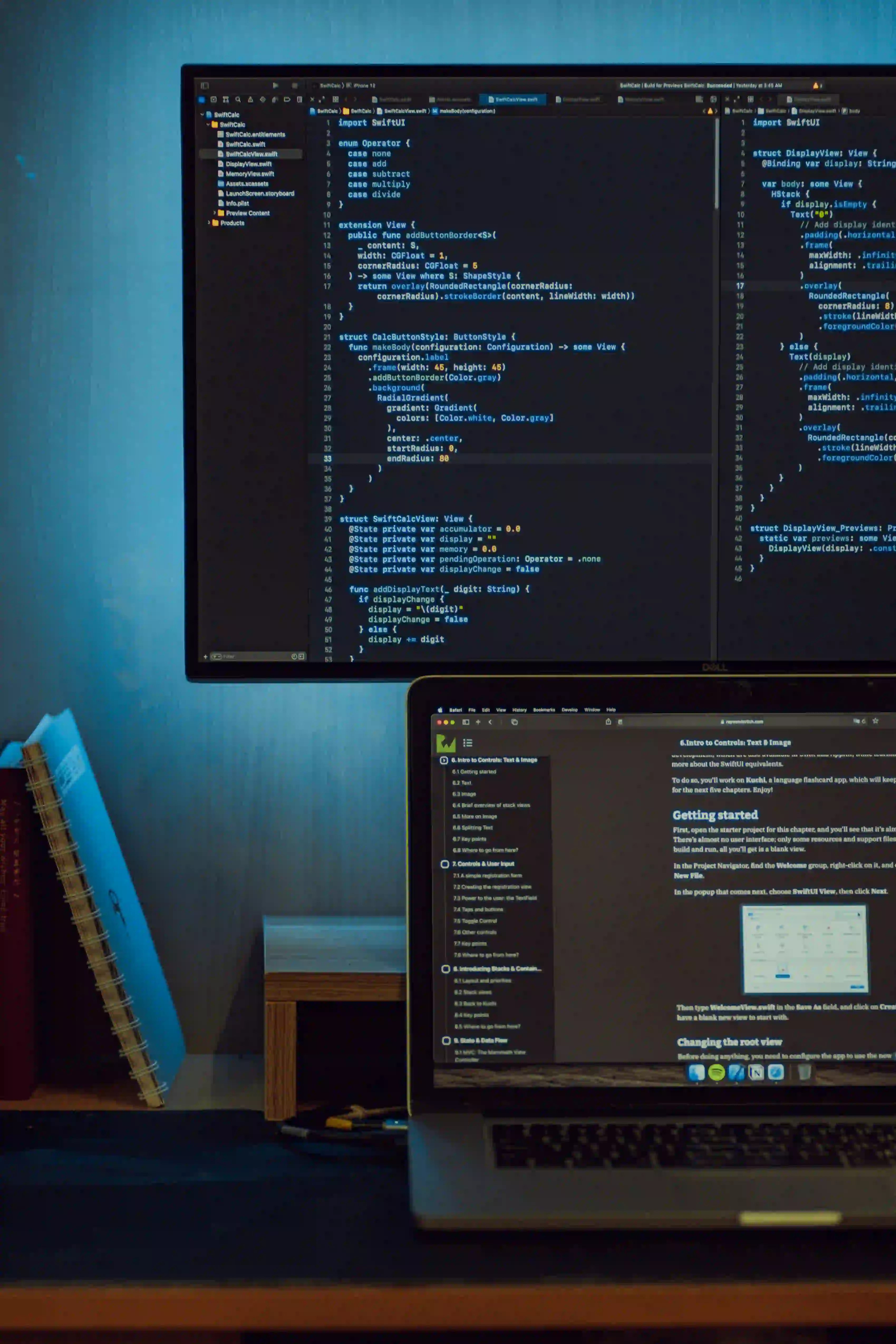
Understanding RGB Color Representation Issues in Java
Colors play a vital role in the aesthetics of applications, web designs, and user interfaces. In Java, the RGB (Red, Green, Blue) color model is a fundamental way to represent these colors. While simplistic and powerful, working with RGB values can sometimes lead to unexpected results. This blog post will delve into RGB color representation issues in Java, giving you a solid grounding in how colors are constructed and manipulated, along with best practices to ensure your applications render colors accurately.
What is RGB?
The RGB color model combines varying intensities of red, green, and blue light. This additive color model means that by adjusting the intensity of each component, you can create a broad spectrum of colors. Each color in the RGB model can typically be represented by a combination of three integer values—one for each color channel—which ranges from 0 to 255.
Example of RGB
For example:
- (255, 0, 0) represents pure red.
- (0, 255, 0) represents pure green.
- (0, 0, 255) represents pure blue.
- (255, 255, 255) is white, as all colors are combined at full intensity.
- (0, 0, 0) is black, where no colors are combined.
Java Representation of RGB
In Java, the representation of RGB colors is often handled through the java.awt.Color
class.
import java.awt.Color;
public class ColorExample {
public static void main(String[] args) {
// Create colors using RGB values
Color red = new Color(255, 0, 0);
Color green = new Color(0, 255, 0);
Color blue = new Color(0, 0, 255);
System.out.println(red); // java.awt.Color[r=255,g=0,b=0]
System.out.println(green); // java.awt.Color[r=0,g=255,b=0]
System.out.println(blue); // java.awt.Color[r=0,g=0,b=255]
}
}
This straightforward snippet creates three colors and prints their values. Using the Color
class provides additional methods for manipulating these colors, which we'll discuss later.
Understanding Common Issues with RGB Representation
Despite its simplicity, RGB representation can pose several challenges. Here are the most common issues:
1. Color Clipping
When generating colors dynamically or manipulating them, it’s easy to exceed the maximum (255) or minimum (0) values for each color channel. This is known as color clipping.
Example of Color Clipping
Color color = new Color(300, -20, 150); // Invalid values
System.out.println(color); // Outputs: java.awt.Color[r=255,g=0,b=150]
Why This Matters:
In the above example, the RGB values provided to the Color
constructor are outside the valid range. Instead of throwing an error, the color channels are clipped to their valid boundaries—resulting in full red (255) and no green (0).
2. Color Perception Differences
Not all colors appear the same on different screens due to variations in display technologies. Factors like backlighting, glass quality, and viewing angles can affect color perception.
Why This Matters: This inconsistency can lead to problems, especially in digital design work or applications where precise color matching is crucial. Always test your colors on multiple devices if your application relies heavily on visual accuracy.
3. Color Mixing Issues
When mixing colors programmatically, developers sometimes forget to account for the additive nature of the RGB model.
Example of Color Mixing
Color blue = new Color(0, 0, 255);
Color red = new Color(255, 0, 0);
Color purple = new Color(Math.min(255, red.getRed() + blue.getRed()),
Math.min(255, red.getGreen() + blue.getGreen()),
Math.min(255, red.getBlue() + blue.getBlue()));
System.out.println(purple); // Expected: java.awt.Color[r=255,g=0,b=255]
In this code, we ensure that each component is confined to the valid range. If we did not use Math.min
, we could potentially create colors that are clipped.
4. Alpha Channel Issues
RGBA (Red, Green, Blue, Alpha) represents colors along with transparency. By ignoring the alpha channel, colors can appear unexpectedly.
Example with Alpha Channel
Color semiTransparentRed = new Color(255, 0, 0, 128); // 50% transparency
Color opaqueRed = new Color(255, 0, 0); // Fully opaque
Why This Matters: With semi-transparent colors, the final display involves layering over a background, which can mix with the colors behind it. This can lead to misleading perceptions of the color.
Best Practices for Managing RGB Colors in Java
To make the most of RGB colors in Java, adhere to these best practices:
-
Validate Input Values: Always check RGB values before creating a
Color
object. Use utility methods to ensure values fall within the acceptable range. -
Understand Device Display Specifications: Familiarize yourself with various display technology specifications and test your designs across multiple devices for consistency.
-
Use Libraries for Color Management: Consider leveraging libraries such as Apache Commons Imaging to handle advanced color manipulations and conversions that go beyond basic RGB.
-
Maintain Consistency in Color Profiles: If your application is graphic heavy or manipulative in nature, understand the color profiles (like sRGB) to maintain consistency.
-
Don't Ignore Alpha: Always consider how transparency can affect color representation in your applications, especially in graphics-heavy applications.
A Final Look
RGB color representation is a robust and versatile system for managing colors in Java. By understanding its limitations and potential pitfalls, as well as using best practices, developers can achieve an accurate and aesthetically pleasing color representation in their Java applications.
For further reading on Java's color handling capabilities, check out the Java AWT Color documentation.
Thus, with a clear understanding of RGB color representation issues, developers can confidently create visually stunning applications while minimizing the risk of color inaccuracies.