The Hidden Costs of Not Using Comments in Code
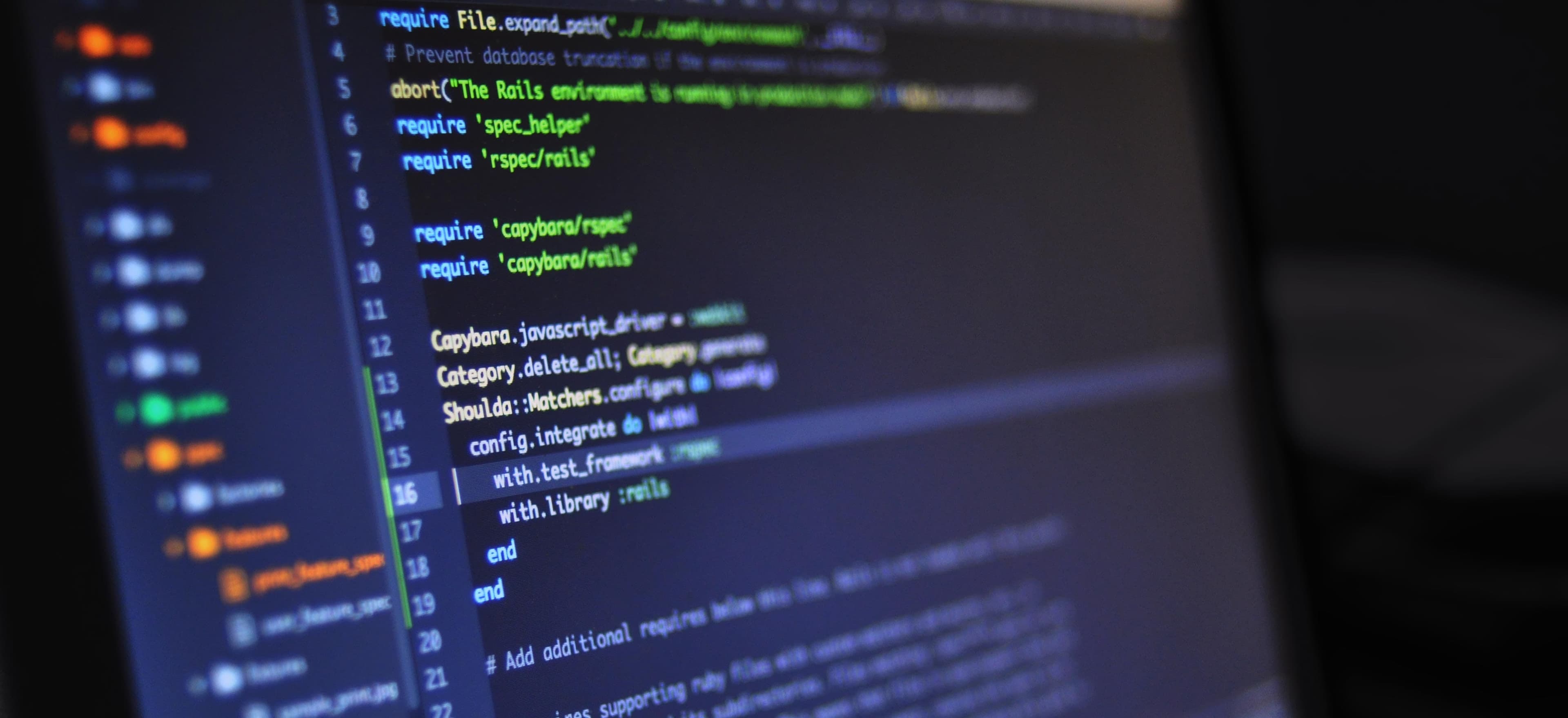
- Published on
The Hidden Costs of Not Using Comments in Code
In the realm of programming, effective communication is paramount. While code may be seen as a primary means of communication between developers and computers, its readability for other human beings is just as crucial. This is where comments play their vital role. Yet, many developers neglect this practice, assuming that code should be self-documenting. In this blog post, we'll delve into the hidden costs of not using comments in your code and explore how strategic commenting can enhance collaboration, maintenance, and overall software quality.
Why Use Comments in Code?
-
Improved Readability: Comments provide context and explanations, making the code more accessible to different audiences. Anyone new to the project will benefit from insights that might not be immediately apparent from the code itself.
-
Ease of Maintenance: Code is not set in stone. It will likely need modifications over time. Well-commented code can significantly reduce the time and effort needed to understand existing functionalities when it's time to make changes.
-
Facilitate Knowledge Transfer: Team members come and go. Comments serve as essential documentation that can help in training new developers or in providing insights when someone unfamiliar with the codebase needs to step in.
-
Enhancing Collaboration: When multiple developers work on the same codebase, comments can clarify intentions and logic. This reduces the risk of miscommunication and helps streamline project workflows.
-
Minimize Errors: Lack of comments can lead to misunderstandings. This can introduce bugs when developers misinterpret the functionality of the code.
The Costs of Neglecting Comments
Neglecting comments has substantial hidden costs that can accumulate over time.
1. Increased Time for Understanding Code
Consider this simple Java function:
public int calculateTotal(int price, int quantity) {
return price * quantity;
}
On the surface, this function is understandable. However, without comments, future developers might struggle to grasp its role in a more complex application.
Adding a comment transforms clarity:
/**
* Calculates the total price based on unit price and quantity.
* @param price The price of an individual item.
* @param quantity The number of items.
* @return The total price as an integer.
*/
public int calculateTotal(int price, int quantity) {
return price * quantity;
}
This short explanation can save another developer several minutes of deciphering the logic behind the code.
2. Increased Bug Risks
Mistakes can happen. Without comments indicating the purpose of a particular segment of code, a developer might inadvertently make changes that introduce new bugs. For instance, imagine a piece of code handling a specific case in an application:
if (userIsAdmin) {
grantAccess();
} else {
denyAccess();
}
If the context of the userIsAdmin
variable is unclear, a developer might hesitate about whether this logic is crucial. A comment here could clarify:
// Grant access only to users with admin privileges.
if (userIsAdmin) {
grantAccess();
} else {
denyAccess();
}
This context can help prevent accidental changes that alter the intended logic.
3. Higher Maintenance Costs
In the long run, unclear code leads to immense maintenance costs. According to studies, developers spend around 50-70% of their time maintaining existing code. If you do not comment on your code, the time taken to comprehend and debug it escalates.
A perfect example involves refactoring:
public void processTransaction(User user, double amount) {
// Transaction processing steps
if (user.hasCredit()) {
// Charge user
}
}
This function's purpose is not evident without context. An effective refactoring requires knowledge of the transaction process. If there were detailed comments on the transaction logic, it would save time and effort.
Effective Commenting Techniques
To avoid the hidden costs associated with poor commenting practices, here's a list of techniques to implement effective comments in your Java code:
-
Use Descriptive Comments: Rather than commenting on what the code is doing, explain why it's being done. For instance, instead of stating the obvious, provide contextual insights.
-
Document Complex Logic: For complex algorithms or unusual methods, thoroughly document your approach. This clarity can be golden for anyone trying to rework that section later.
-
Adhere to a Consistent Style: Maintain formatting and structure in your comments. Following Java's documentation style (using
/** ... */
for method comments) leads to consistency and professionalism. -
Avoid Redundant Comments: Comments that simply restate what the code does can clutter the code. For example, commenting on a
for
loop with// Loop through the array
is unnecessary. -
Update Comments Regularly: As the code changes, ensure comments are revised as well. Obsolete comments can confuse and misguide developers.
Summary
Effective commenting is an art that can significantly impact the success of a software project. Although it may seem trivial at first glance, the hidden costs of neglecting comments can harm productivity, collaboration, and maintainability.
To reinforce this concept, remember that good comments not only improve readability but also facilitate smoother transitions in teams and projects. By applying robust commenting practices, you can deliver higher quality code that stands the test of time.
Resources for Further Learning
- Effective Java by Joshua Bloch - A definitive guide that emphasizes the importance of code clarity.
- Clean Code by Robert C. Martin - Learn the qualitative aspects of writing clean, maintainable code.
By intentionally investing time into your code's clarity, you’ll save countless hours in understanding, collaboration, and maintenance down the line. Don’t let your hard work be unnoticed; make comments your ally in programming!
Checkout our other articles